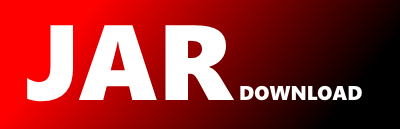
com.pulumi.azure.containerservice.kotlin.RegistryTask.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-kotlin Show documentation
Show all versions of pulumi-azure-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azure.containerservice.kotlin
import com.pulumi.azure.containerservice.kotlin.outputs.RegistryTaskAgentSetting
import com.pulumi.azure.containerservice.kotlin.outputs.RegistryTaskBaseImageTrigger
import com.pulumi.azure.containerservice.kotlin.outputs.RegistryTaskDockerStep
import com.pulumi.azure.containerservice.kotlin.outputs.RegistryTaskEncodedStep
import com.pulumi.azure.containerservice.kotlin.outputs.RegistryTaskFileStep
import com.pulumi.azure.containerservice.kotlin.outputs.RegistryTaskIdentity
import com.pulumi.azure.containerservice.kotlin.outputs.RegistryTaskPlatform
import com.pulumi.azure.containerservice.kotlin.outputs.RegistryTaskRegistryCredential
import com.pulumi.azure.containerservice.kotlin.outputs.RegistryTaskSourceTrigger
import com.pulumi.azure.containerservice.kotlin.outputs.RegistryTaskTimerTrigger
import com.pulumi.core.Output
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.collections.Map
import com.pulumi.azure.containerservice.kotlin.outputs.RegistryTaskAgentSetting.Companion.toKotlin as registryTaskAgentSettingToKotlin
import com.pulumi.azure.containerservice.kotlin.outputs.RegistryTaskBaseImageTrigger.Companion.toKotlin as registryTaskBaseImageTriggerToKotlin
import com.pulumi.azure.containerservice.kotlin.outputs.RegistryTaskDockerStep.Companion.toKotlin as registryTaskDockerStepToKotlin
import com.pulumi.azure.containerservice.kotlin.outputs.RegistryTaskEncodedStep.Companion.toKotlin as registryTaskEncodedStepToKotlin
import com.pulumi.azure.containerservice.kotlin.outputs.RegistryTaskFileStep.Companion.toKotlin as registryTaskFileStepToKotlin
import com.pulumi.azure.containerservice.kotlin.outputs.RegistryTaskIdentity.Companion.toKotlin as registryTaskIdentityToKotlin
import com.pulumi.azure.containerservice.kotlin.outputs.RegistryTaskPlatform.Companion.toKotlin as registryTaskPlatformToKotlin
import com.pulumi.azure.containerservice.kotlin.outputs.RegistryTaskRegistryCredential.Companion.toKotlin as registryTaskRegistryCredentialToKotlin
import com.pulumi.azure.containerservice.kotlin.outputs.RegistryTaskSourceTrigger.Companion.toKotlin as registryTaskSourceTriggerToKotlin
import com.pulumi.azure.containerservice.kotlin.outputs.RegistryTaskTimerTrigger.Companion.toKotlin as registryTaskTimerTriggerToKotlin
/**
* Builder for [RegistryTask].
*/
@PulumiTagMarker
public class RegistryTaskResourceBuilder internal constructor() {
public var name: String? = null
public var args: RegistryTaskArgs = RegistryTaskArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend RegistryTaskArgsBuilder.() -> Unit) {
val builder = RegistryTaskArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): RegistryTask {
val builtJavaResource = com.pulumi.azure.containerservice.RegistryTask(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return RegistryTask(builtJavaResource)
}
}
/**
* Manages a Container Registry Task.
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as azure from "@pulumi/azure";
* const example = new azure.core.ResourceGroup("example", {
* name: "example-rg",
* location: "West Europe",
* });
* const exampleRegistry = new azure.containerservice.Registry("example", {
* name: "example",
* resourceGroupName: example.name,
* location: example.location,
* sku: "Basic",
* });
* const exampleRegistryTask = new azure.containerservice.RegistryTask("example", {
* name: "example-task",
* containerRegistryId: exampleRegistry.id,
* platform: {
* os: "Linux",
* },
* dockerStep: {
* dockerfilePath: "Dockerfile",
* contextPath: "https://github.com//#:",
* contextAccessToken: "",
* imageNames: ["helloworld:{{.Run.ID}}"],
* },
* });
* ```
* ```python
* import pulumi
* import pulumi_azure as azure
* example = azure.core.ResourceGroup("example",
* name="example-rg",
* location="West Europe")
* example_registry = azure.containerservice.Registry("example",
* name="example",
* resource_group_name=example.name,
* location=example.location,
* sku="Basic")
* example_registry_task = azure.containerservice.RegistryTask("example",
* name="example-task",
* container_registry_id=example_registry.id,
* platform=azure.containerservice.RegistryTaskPlatformArgs(
* os="Linux",
* ),
* docker_step=azure.containerservice.RegistryTaskDockerStepArgs(
* dockerfile_path="Dockerfile",
* context_path="https://github.com//#:",
* context_access_token="",
* image_names=["helloworld:{{.Run.ID}}"],
* ))
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Azure = Pulumi.Azure;
* return await Deployment.RunAsync(() =>
* {
* var example = new Azure.Core.ResourceGroup("example", new()
* {
* Name = "example-rg",
* Location = "West Europe",
* });
* var exampleRegistry = new Azure.ContainerService.Registry("example", new()
* {
* Name = "example",
* ResourceGroupName = example.Name,
* Location = example.Location,
* Sku = "Basic",
* });
* var exampleRegistryTask = new Azure.ContainerService.RegistryTask("example", new()
* {
* Name = "example-task",
* ContainerRegistryId = exampleRegistry.Id,
* Platform = new Azure.ContainerService.Inputs.RegistryTaskPlatformArgs
* {
* Os = "Linux",
* },
* DockerStep = new Azure.ContainerService.Inputs.RegistryTaskDockerStepArgs
* {
* DockerfilePath = "Dockerfile",
* ContextPath = "https://github.com//#:",
* ContextAccessToken = "",
* ImageNames = new[]
* {
* "helloworld:{{.Run.ID}}",
* },
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-azure/sdk/v5/go/azure/containerservice"
* "github.com/pulumi/pulumi-azure/sdk/v5/go/azure/core"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* example, err := core.NewResourceGroup(ctx, "example", &core.ResourceGroupArgs{
* Name: pulumi.String("example-rg"),
* Location: pulumi.String("West Europe"),
* })
* if err != nil {
* return err
* }
* exampleRegistry, err := containerservice.NewRegistry(ctx, "example", &containerservice.RegistryArgs{
* Name: pulumi.String("example"),
* ResourceGroupName: example.Name,
* Location: example.Location,
* Sku: pulumi.String("Basic"),
* })
* if err != nil {
* return err
* }
* _, err = containerservice.NewRegistryTask(ctx, "example", &containerservice.RegistryTaskArgs{
* Name: pulumi.String("example-task"),
* ContainerRegistryId: exampleRegistry.ID(),
* Platform: &containerservice.RegistryTaskPlatformArgs{
* Os: pulumi.String("Linux"),
* },
* DockerStep: &containerservice.RegistryTaskDockerStepArgs{
* DockerfilePath: pulumi.String("Dockerfile"),
* ContextPath: pulumi.String("https://github.com//#:"),
* ContextAccessToken: pulumi.String(""),
* ImageNames: pulumi.StringArray{
* pulumi.String("helloworld:{{.Run.ID}}"),
* },
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azure.core.ResourceGroup;
* import com.pulumi.azure.core.ResourceGroupArgs;
* import com.pulumi.azure.containerservice.Registry;
* import com.pulumi.azure.containerservice.RegistryArgs;
* import com.pulumi.azure.containerservice.RegistryTask;
* import com.pulumi.azure.containerservice.RegistryTaskArgs;
* import com.pulumi.azure.containerservice.inputs.RegistryTaskPlatformArgs;
* import com.pulumi.azure.containerservice.inputs.RegistryTaskDockerStepArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var example = new ResourceGroup("example", ResourceGroupArgs.builder()
* .name("example-rg")
* .location("West Europe")
* .build());
* var exampleRegistry = new Registry("exampleRegistry", RegistryArgs.builder()
* .name("example")
* .resourceGroupName(example.name())
* .location(example.location())
* .sku("Basic")
* .build());
* var exampleRegistryTask = new RegistryTask("exampleRegistryTask", RegistryTaskArgs.builder()
* .name("example-task")
* .containerRegistryId(exampleRegistry.id())
* .platform(RegistryTaskPlatformArgs.builder()
* .os("Linux")
* .build())
* .dockerStep(RegistryTaskDockerStepArgs.builder()
* .dockerfilePath("Dockerfile")
* .contextPath("https://github.com//#:")
* .contextAccessToken("")
* .imageNames("helloworld:{{.Run.ID}}")
* .build())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* example:
* type: azure:core:ResourceGroup
* properties:
* name: example-rg
* location: West Europe
* exampleRegistry:
* type: azure:containerservice:Registry
* name: example
* properties:
* name: example
* resourceGroupName: ${example.name}
* location: ${example.location}
* sku: Basic
* exampleRegistryTask:
* type: azure:containerservice:RegistryTask
* name: example
* properties:
* name: example-task
* containerRegistryId: ${exampleRegistry.id}
* platform:
* os: Linux
* dockerStep:
* dockerfilePath: Dockerfile
* contextPath: https://github.com//#:
* contextAccessToken:
* imageNames:
* - helloworld:{{.Run.ID}}
* ```
*
* ## Import
* Container Registry Tasks can be imported using the `resource id`, e.g.
* ```sh
* $ pulumi import azure:containerservice/registryTask:RegistryTask example /subscriptions/12345678-1234-9876-4563-123456789012/resourceGroups/group1/providers/Microsoft.ContainerRegistry/registries/registry1/tasks/task1
* ```
*/
public class RegistryTask internal constructor(
override val javaResource: com.pulumi.azure.containerservice.RegistryTask,
) : KotlinCustomResource(javaResource, RegistryTaskMapper) {
/**
* The name of the dedicated Container Registry Agent Pool for this Container Registry Task.
*/
public val agentPoolName: Output?
get() = javaResource.agentPoolName().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* A `agent_setting` block as defined below.
*/
public val agentSetting: Output?
get() = javaResource.agentSetting().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
registryTaskAgentSettingToKotlin(args0)
})
}).orElse(null)
})
/**
* A `base_image_trigger` block as defined below.
*/
public val baseImageTrigger: Output?
get() = javaResource.baseImageTrigger().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> registryTaskBaseImageTriggerToKotlin(args0) })
}).orElse(null)
})
/**
* The ID of the Container Registry that this Container Registry Task resides in. Changing this forces a new Container Registry Task to be created.
*/
public val containerRegistryId: Output
get() = javaResource.containerRegistryId().applyValue({ args0 -> args0 })
/**
* A `docker_step` block as defined below.
*/
public val dockerStep: Output?
get() = javaResource.dockerStep().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
registryTaskDockerStepToKotlin(args0)
})
}).orElse(null)
})
/**
* Should this Container Registry Task be enabled? Defaults to `true`.
*/
public val enabled: Output?
get() = javaResource.enabled().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* A `encoded_step` block as defined below.
*/
public val encodedStep: Output?
get() = javaResource.encodedStep().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
registryTaskEncodedStepToKotlin(args0)
})
}).orElse(null)
})
/**
* A `file_step` block as defined below.
* > **NOTE:** For non-system task (when `is_system_task` is set to `false`), one and only one of the `docker_step`, `encoded_step` and `file_step` should be specified.
*/
public val fileStep: Output?
get() = javaResource.fileStep().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
registryTaskFileStepToKotlin(args0)
})
}).orElse(null)
})
/**
* An `identity` block as defined below.
*/
public val identity: Output?
get() = javaResource.identity().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
registryTaskIdentityToKotlin(args0)
})
}).orElse(null)
})
/**
* Whether this Container Registry Task is a system task. Changing this forces a new Container Registry Task to be created. Defaults to `false`.
*/
public val isSystemTask: Output?
get() = javaResource.isSystemTask().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
public val logTemplate: Output?
get() = javaResource.logTemplate().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The name which should be used for this Container Registry Task. Changing this forces a new Container Registry Task to be created.
*/
public val name: Output
get() = javaResource.name().applyValue({ args0 -> args0 })
/**
* A `platform` block as defined below.
* > **NOTE:** The `platform` is required for non-system task (when `is_system_task` is set to `false`).
*/
public val platform: Output?
get() = javaResource.platform().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
registryTaskPlatformToKotlin(args0)
})
}).orElse(null)
})
public val registryCredential: Output?
get() = javaResource.registryCredential().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> registryTaskRegistryCredentialToKotlin(args0) })
}).orElse(null)
})
/**
* One or more `source_trigger` blocks as defined below.
*/
public val sourceTriggers: Output>?
get() = javaResource.sourceTriggers().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
registryTaskSourceTriggerToKotlin(args0)
})
})
}).orElse(null)
})
public val tags: Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy