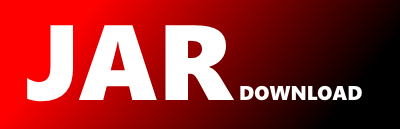
com.pulumi.azure.containerservice.kotlin.TokenPasswordArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-kotlin Show documentation
Show all versions of pulumi-azure-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azure.containerservice.kotlin
import com.pulumi.azure.containerservice.TokenPasswordArgs.builder
import com.pulumi.azure.containerservice.kotlin.inputs.TokenPasswordPassword1Args
import com.pulumi.azure.containerservice.kotlin.inputs.TokenPasswordPassword1ArgsBuilder
import com.pulumi.azure.containerservice.kotlin.inputs.TokenPasswordPassword2Args
import com.pulumi.azure.containerservice.kotlin.inputs.TokenPasswordPassword2ArgsBuilder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
* Manages a Container Registry Token Password.
* ## Example Usage
*
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azure.core.ResourceGroup;
* import com.pulumi.azure.core.ResourceGroupArgs;
* import com.pulumi.azure.containerservice.Registry;
* import com.pulumi.azure.containerservice.RegistryArgs;
* import com.pulumi.azure.containerservice.RegistryScopeMap;
* import com.pulumi.azure.containerservice.RegistryScopeMapArgs;
* import com.pulumi.azure.containerservice.RegistryToken;
* import com.pulumi.azure.containerservice.RegistryTokenArgs;
* import com.pulumi.azure.containerservice.TokenPassword;
* import com.pulumi.azure.containerservice.TokenPasswordArgs;
* import com.pulumi.azure.containerservice.inputs.TokenPasswordPassword1Args;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var example = new ResourceGroup("example", ResourceGroupArgs.builder()
* .name("example-resource-group")
* .location("West Europe")
* .build());
* var exampleRegistry = new Registry("exampleRegistry", RegistryArgs.builder()
* .name("example-registry")
* .resourceGroupName(example.name())
* .location(example.location())
* .sku("Premium")
* .adminEnabled(false)
* .georeplicationLocations(
* "East US",
* "West Europe")
* .build());
* var exampleRegistryScopeMap = new RegistryScopeMap("exampleRegistryScopeMap", RegistryScopeMapArgs.builder()
* .name("example-scope-map")
* .containerRegistryName(exampleRegistry.name())
* .resourceGroupName(example.name())
* .actions(
* "repositories/repo1/content/read",
* "repositories/repo1/content/write")
* .build());
* var exampleRegistryToken = new RegistryToken("exampleRegistryToken", RegistryTokenArgs.builder()
* .name("exampletoken")
* .containerRegistryName(exampleRegistry.name())
* .resourceGroupName(example.name())
* .scopeMapId(exampleRegistryScopeMap.id())
* .build());
* var exampleTokenPassword = new TokenPassword("exampleTokenPassword", TokenPasswordArgs.builder()
* .containerRegistryTokenId(exampleRegistryToken.id())
* .password1(TokenPasswordPassword1Args.builder()
* .expiry("2023-03-22T17:57:36+08:00")
* .build())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* example:
* type: azure:core:ResourceGroup
* properties:
* name: example-resource-group
* location: West Europe
* exampleRegistry:
* type: azure:containerservice:Registry
* name: example
* properties:
* name: example-registry
* resourceGroupName: ${example.name}
* location: ${example.location}
* sku: Premium
* adminEnabled: false
* georeplicationLocations:
* - East US
* - West Europe
* exampleRegistryScopeMap:
* type: azure:containerservice:RegistryScopeMap
* name: example
* properties:
* name: example-scope-map
* containerRegistryName: ${exampleRegistry.name}
* resourceGroupName: ${example.name}
* actions:
* - repositories/repo1/content/read
* - repositories/repo1/content/write
* exampleRegistryToken:
* type: azure:containerservice:RegistryToken
* name: example
* properties:
* name: exampletoken
* containerRegistryName: ${exampleRegistry.name}
* resourceGroupName: ${example.name}
* scopeMapId: ${exampleRegistryScopeMap.id}
* exampleTokenPassword:
* type: azure:containerservice:TokenPassword
* name: example
* properties:
* containerRegistryTokenId: ${exampleRegistryToken.id}
* password1:
* expiry: 2023-03-22T17:57:36+08:00
* ```
*
* ## Import
* Container Registry Token Passwords can be imported using the `resource id`, e.g.
* ```sh
* $ pulumi import azure:containerservice/tokenPassword:TokenPassword example /subscriptions/12345678-1234-9876-4563-123456789012/resourceGroups/group1/providers/Microsoft.ContainerRegistry/registries/registry1/tokens/token1/passwords/password
* ```
* @property containerRegistryTokenId The ID of the Container Registry Token that this Container Registry Token Password resides in. Changing this forces a new Container Registry Token Password to be created.
* @property password1 One `password` block as defined below.
* @property password2 One `password` block as defined below.
*/
public data class TokenPasswordArgs(
public val containerRegistryTokenId: Output? = null,
public val password1: Output? = null,
public val password2: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azure.containerservice.TokenPasswordArgs =
com.pulumi.azure.containerservice.TokenPasswordArgs.builder()
.containerRegistryTokenId(containerRegistryTokenId?.applyValue({ args0 -> args0 }))
.password1(password1?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.password2(password2?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) })).build()
}
/**
* Builder for [TokenPasswordArgs].
*/
@PulumiTagMarker
public class TokenPasswordArgsBuilder internal constructor() {
private var containerRegistryTokenId: Output? = null
private var password1: Output? = null
private var password2: Output? = null
/**
* @param value The ID of the Container Registry Token that this Container Registry Token Password resides in. Changing this forces a new Container Registry Token Password to be created.
*/
@JvmName("jvuuubhptchalilb")
public suspend fun containerRegistryTokenId(`value`: Output) {
this.containerRegistryTokenId = value
}
/**
* @param value One `password` block as defined below.
*/
@JvmName("lradjtjeqbvdrkcc")
public suspend fun password1(`value`: Output) {
this.password1 = value
}
/**
* @param value One `password` block as defined below.
*/
@JvmName("xnendsqwatkgcvnv")
public suspend fun password2(`value`: Output) {
this.password2 = value
}
/**
* @param value The ID of the Container Registry Token that this Container Registry Token Password resides in. Changing this forces a new Container Registry Token Password to be created.
*/
@JvmName("bwvlkivjnwiebdqa")
public suspend fun containerRegistryTokenId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.containerRegistryTokenId = mapped
}
/**
* @param value One `password` block as defined below.
*/
@JvmName("nfivoimjaesamptq")
public suspend fun password1(`value`: TokenPasswordPassword1Args?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.password1 = mapped
}
/**
* @param argument One `password` block as defined below.
*/
@JvmName("uftwjdbonritdbfv")
public suspend fun password1(argument: suspend TokenPasswordPassword1ArgsBuilder.() -> Unit) {
val toBeMapped = TokenPasswordPassword1ArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.password1 = mapped
}
/**
* @param value One `password` block as defined below.
*/
@JvmName("xpjlghsotvkpohfa")
public suspend fun password2(`value`: TokenPasswordPassword2Args?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.password2 = mapped
}
/**
* @param argument One `password` block as defined below.
*/
@JvmName("fggslsxbciqckdst")
public suspend fun password2(argument: suspend TokenPasswordPassword2ArgsBuilder.() -> Unit) {
val toBeMapped = TokenPasswordPassword2ArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.password2 = mapped
}
internal fun build(): TokenPasswordArgs = TokenPasswordArgs(
containerRegistryTokenId = containerRegistryTokenId,
password1 = password1,
password2 = password2,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy