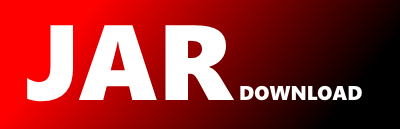
com.pulumi.azure.containerservice.kotlin.inputs.GroupInitContainerArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-kotlin Show documentation
Show all versions of pulumi-azure-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azure.containerservice.kotlin.inputs
import com.pulumi.azure.containerservice.inputs.GroupInitContainerArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Pair
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.collections.Map
import kotlin.jvm.JvmName
/**
*
* @property commands A list of commands which should be run on the container. Changing this forces a new resource to be created.
* @property environmentVariables A list of environment variables to be set on the container. Specified as a map of name/value pairs. Changing this forces a new resource to be created.
* @property image The container image name. Changing this forces a new resource to be created.
* @property name Specifies the name of the Container. Changing this forces a new resource to be created.
* @property secureEnvironmentVariables A list of sensitive environment variables to be set on the container. Specified as a map of name/value pairs. Changing this forces a new resource to be created.
* @property securities The definition of the security context for this container as documented in the `security` block below. Changing this forces a new resource to be created.
* @property volumes The definition of a volume mount for this container as documented in the `volume` block below. Changing this forces a new resource to be created.
*/
public data class GroupInitContainerArgs(
public val commands: Output>? = null,
public val environmentVariables: Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy