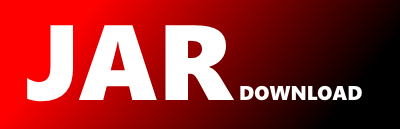
com.pulumi.azure.containerservice.kotlin.inputs.KubernetesClusterIngressApplicationGatewayArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-kotlin Show documentation
Show all versions of pulumi-azure-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azure.containerservice.kotlin.inputs
import com.pulumi.azure.containerservice.inputs.KubernetesClusterIngressApplicationGatewayArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
*
* @property effectiveGatewayId The ID of the Application Gateway associated with the ingress controller deployed to this Kubernetes Cluster.
* @property gatewayId The ID of the Application Gateway to integrate with the ingress controller of this Kubernetes Cluster. See [this](https://docs.microsoft.com/azure/application-gateway/tutorial-ingress-controller-add-on-existing) page for further details.
* @property gatewayName The name of the Application Gateway to be used or created in the Nodepool Resource Group, which in turn will be integrated with the ingress controller of this Kubernetes Cluster. See [this](https://docs.microsoft.com/azure/application-gateway/tutorial-ingress-controller-add-on-new) page for further details.
* @property ingressApplicationGatewayIdentities An `ingress_application_gateway_identity` block is exported. The exported attributes are defined below.
* @property subnetCidr The subnet CIDR to be used to create an Application Gateway, which in turn will be integrated with the ingress controller of this Kubernetes Cluster. See [this](https://docs.microsoft.com/azure/application-gateway/tutorial-ingress-controller-add-on-new) page for further details.
* @property subnetId The ID of the subnet on which to create an Application Gateway, which in turn will be integrated with the ingress controller of this Kubernetes Cluster. See [this](https://docs.microsoft.com/azure/application-gateway/tutorial-ingress-controller-add-on-new) page for further details.
* > **Note:** Exactly one of `gateway_id`, `subnet_id` or `subnet_cidr` must be specified.
* > **Note:** If specifying `ingress_application_gateway` in conjunction with `only_critical_addons_enabled`, the AGIC pod will fail to start. A separate `azure.containerservice.KubernetesClusterNodePool` is required to run the AGIC pod successfully. This is because AGIC is classed as a "non-critical addon".
*/
public data class KubernetesClusterIngressApplicationGatewayArgs(
public val effectiveGatewayId: Output? = null,
public val gatewayId: Output? = null,
public val gatewayName: Output? = null,
public val ingressApplicationGatewayIdentities:
Output>? =
null,
public val subnetCidr: Output? = null,
public val subnetId: Output? = null,
) :
ConvertibleToJava {
override fun toJava():
com.pulumi.azure.containerservice.inputs.KubernetesClusterIngressApplicationGatewayArgs =
com.pulumi.azure.containerservice.inputs.KubernetesClusterIngressApplicationGatewayArgs.builder()
.effectiveGatewayId(effectiveGatewayId?.applyValue({ args0 -> args0 }))
.gatewayId(gatewayId?.applyValue({ args0 -> args0 }))
.gatewayName(gatewayName?.applyValue({ args0 -> args0 }))
.ingressApplicationGatewayIdentities(
ingressApplicationGatewayIdentities?.applyValue({ args0 ->
args0.map({ args0 -> args0.let({ args0 -> args0.toJava() }) })
}),
)
.subnetCidr(subnetCidr?.applyValue({ args0 -> args0 }))
.subnetId(subnetId?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [KubernetesClusterIngressApplicationGatewayArgs].
*/
@PulumiTagMarker
public class KubernetesClusterIngressApplicationGatewayArgsBuilder internal constructor() {
private var effectiveGatewayId: Output? = null
private var gatewayId: Output? = null
private var gatewayName: Output? = null
private var ingressApplicationGatewayIdentities:
Output>? =
null
private var subnetCidr: Output? = null
private var subnetId: Output? = null
/**
* @param value The ID of the Application Gateway associated with the ingress controller deployed to this Kubernetes Cluster.
*/
@JvmName("opoyjhnckkqspunx")
public suspend fun effectiveGatewayId(`value`: Output) {
this.effectiveGatewayId = value
}
/**
* @param value The ID of the Application Gateway to integrate with the ingress controller of this Kubernetes Cluster. See [this](https://docs.microsoft.com/azure/application-gateway/tutorial-ingress-controller-add-on-existing) page for further details.
*/
@JvmName("vhyxwfwuhbbkfxby")
public suspend fun gatewayId(`value`: Output) {
this.gatewayId = value
}
/**
* @param value The name of the Application Gateway to be used or created in the Nodepool Resource Group, which in turn will be integrated with the ingress controller of this Kubernetes Cluster. See [this](https://docs.microsoft.com/azure/application-gateway/tutorial-ingress-controller-add-on-new) page for further details.
*/
@JvmName("bltrvhdkhywcyksw")
public suspend fun gatewayName(`value`: Output) {
this.gatewayName = value
}
/**
* @param value An `ingress_application_gateway_identity` block is exported. The exported attributes are defined below.
*/
@JvmName("bcgmbmfrdmlhuafr")
public suspend
fun ingressApplicationGatewayIdentities(`value`: Output>) {
this.ingressApplicationGatewayIdentities = value
}
@JvmName("kvkesojyoxkhymgu")
public suspend fun ingressApplicationGatewayIdentities(
vararg
values: Output,
) {
this.ingressApplicationGatewayIdentities = Output.all(values.asList())
}
/**
* @param values An `ingress_application_gateway_identity` block is exported. The exported attributes are defined below.
*/
@JvmName("cwjaslnncvvbfkuy")
public suspend
fun ingressApplicationGatewayIdentities(values: List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy