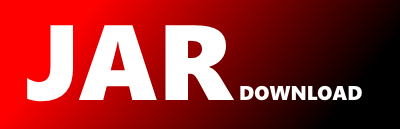
com.pulumi.azure.containerservice.kotlin.inputs.RegistryNetworkRuleSetArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-kotlin Show documentation
Show all versions of pulumi-azure-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azure.containerservice.kotlin.inputs
import com.pulumi.azure.containerservice.inputs.RegistryNetworkRuleSetArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Deprecated
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
*
* @property defaultAction The behaviour for requests matching no rules. Either `Allow` or `Deny`. Defaults to `Allow`
* @property ipRules One or more `ip_rule` blocks as defined below.
* > **NOTE:** `network_rule_set` is only supported with the `Premium` SKU at this time.
* > **NOTE:** Azure automatically configures Network Rules - to remove these you'll need to specify an `network_rule_set` block with `default_action` set to `Deny`.
* @property virtualNetworks
*/
public data class RegistryNetworkRuleSetArgs(
public val defaultAction: Output? = null,
public val ipRules: Output>? = null,
@Deprecated(
message = """
The property `virtual_network` is deprecated since this is used exclusively for service endpoints
which are being deprecated. Users are expected to use Private Endpoints instead. This property
will be removed in v4.0 of the AzureRM Provider.
""",
)
public val virtualNetworks: Output>? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azure.containerservice.inputs.RegistryNetworkRuleSetArgs =
com.pulumi.azure.containerservice.inputs.RegistryNetworkRuleSetArgs.builder()
.defaultAction(defaultAction?.applyValue({ args0 -> args0 }))
.ipRules(
ipRules?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
)
.virtualNetworks(
virtualNetworks?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
).build()
}
/**
* Builder for [RegistryNetworkRuleSetArgs].
*/
@PulumiTagMarker
public class RegistryNetworkRuleSetArgsBuilder internal constructor() {
private var defaultAction: Output? = null
private var ipRules: Output>? = null
private var virtualNetworks: Output>? = null
/**
* @param value The behaviour for requests matching no rules. Either `Allow` or `Deny`. Defaults to `Allow`
*/
@JvmName("qhmolhytpbjhgeef")
public suspend fun defaultAction(`value`: Output) {
this.defaultAction = value
}
/**
* @param value One or more `ip_rule` blocks as defined below.
* > **NOTE:** `network_rule_set` is only supported with the `Premium` SKU at this time.
* > **NOTE:** Azure automatically configures Network Rules - to remove these you'll need to specify an `network_rule_set` block with `default_action` set to `Deny`.
*/
@JvmName("iqeomhwxvvudvjks")
public suspend fun ipRules(`value`: Output>) {
this.ipRules = value
}
@JvmName("bhswrlptsxfjmvqr")
public suspend fun ipRules(vararg values: Output) {
this.ipRules = Output.all(values.asList())
}
/**
* @param values One or more `ip_rule` blocks as defined below.
* > **NOTE:** `network_rule_set` is only supported with the `Premium` SKU at this time.
* > **NOTE:** Azure automatically configures Network Rules - to remove these you'll need to specify an `network_rule_set` block with `default_action` set to `Deny`.
*/
@JvmName("gblihdwosdmottgd")
public suspend fun ipRules(values: List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy