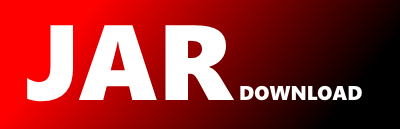
com.pulumi.azure.containerservice.kotlin.inputs.RegistryTaskFileStepArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-kotlin Show documentation
Show all versions of pulumi-azure-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azure.containerservice.kotlin.inputs
import com.pulumi.azure.containerservice.inputs.RegistryTaskFileStepArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Pair
import kotlin.String
import kotlin.Suppress
import kotlin.collections.Map
import kotlin.jvm.JvmName
/**
*
* @property contextAccessToken The token (Git PAT or SAS token of storage account blob) associated with the context for this step.
* @property contextPath The URL (absolute or relative) of the source context for this step.
* @property secretValues Specifies a map of secret values that can be passed when running a task.
* @property taskFilePath The task template file path relative to the source context.
* @property valueFilePath The parameters file path relative to the source context.
* @property values Specifies a map of values that can be passed when running a task.
*/
public data class RegistryTaskFileStepArgs(
public val contextAccessToken: Output? = null,
public val contextPath: Output? = null,
public val secretValues: Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy