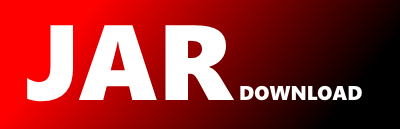
com.pulumi.azure.containerservice.kotlin.outputs.KubernetesClusterDefaultNodePoolLinuxOsConfigSysctlConfig.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-kotlin Show documentation
Show all versions of pulumi-azure-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azure.containerservice.kotlin.outputs
import kotlin.Boolean
import kotlin.Int
import kotlin.Suppress
/**
*
* @property fsAioMaxNr The sysctl setting fs.aio-max-nr. Must be between `65536` and `6553500`.
* @property fsFileMax The sysctl setting fs.file-max. Must be between `8192` and `12000500`.
* @property fsInotifyMaxUserWatches The sysctl setting fs.inotify.max_user_watches. Must be between `781250` and `2097152`.
* @property fsNrOpen The sysctl setting fs.nr_open. Must be between `8192` and `20000500`.
* @property kernelThreadsMax The sysctl setting kernel.threads-max. Must be between `20` and `513785`.
* @property netCoreNetdevMaxBacklog The sysctl setting net.core.netdev_max_backlog. Must be between `1000` and `3240000`.
* @property netCoreOptmemMax The sysctl setting net.core.optmem_max. Must be between `20480` and `4194304`.
* @property netCoreRmemDefault The sysctl setting net.core.rmem_default. Must be between `212992` and `134217728`.
* @property netCoreRmemMax The sysctl setting net.core.rmem_max. Must be between `212992` and `134217728`.
* @property netCoreSomaxconn The sysctl setting net.core.somaxconn. Must be between `4096` and `3240000`.
* @property netCoreWmemDefault The sysctl setting net.core.wmem_default. Must be between `212992` and `134217728`.
* @property netCoreWmemMax The sysctl setting net.core.wmem_max. Must be between `212992` and `134217728`.
* @property netIpv4IpLocalPortRangeMax The sysctl setting net.ipv4.ip_local_port_range max value. Must be between `32768` and `65535`.
* @property netIpv4IpLocalPortRangeMin The sysctl setting net.ipv4.ip_local_port_range min value. Must be between `1024` and `60999`.
* @property netIpv4NeighDefaultGcThresh1 The sysctl setting net.ipv4.neigh.default.gc_thresh1. Must be between `128` and `80000`.
* @property netIpv4NeighDefaultGcThresh2 The sysctl setting net.ipv4.neigh.default.gc_thresh2. Must be between `512` and `90000`.
* @property netIpv4NeighDefaultGcThresh3 The sysctl setting net.ipv4.neigh.default.gc_thresh3. Must be between `1024` and `100000`.
* @property netIpv4TcpFinTimeout The sysctl setting net.ipv4.tcp_fin_timeout. Must be between `5` and `120`.
* @property netIpv4TcpKeepaliveIntvl The sysctl setting net.ipv4.tcp_keepalive_intvl. Must be between `10` and `90`.
* @property netIpv4TcpKeepaliveProbes The sysctl setting net.ipv4.tcp_keepalive_probes. Must be between `1` and `15`.
* @property netIpv4TcpKeepaliveTime The sysctl setting net.ipv4.tcp_keepalive_time. Must be between `30` and `432000`.
* @property netIpv4TcpMaxSynBacklog The sysctl setting net.ipv4.tcp_max_syn_backlog. Must be between `128` and `3240000`.
* @property netIpv4TcpMaxTwBuckets The sysctl setting net.ipv4.tcp_max_tw_buckets. Must be between `8000` and `1440000`.
* @property netIpv4TcpTwReuse The sysctl setting net.ipv4.tcp_tw_reuse.
* @property netNetfilterNfConntrackBuckets The sysctl setting net.netfilter.nf_conntrack_buckets. Must be between `65536` and `524288`.
* @property netNetfilterNfConntrackMax The sysctl setting net.netfilter.nf_conntrack_max. Must be between `131072` and `2097152`.
* @property vmMaxMapCount The sysctl setting vm.max_map_count. Must be between `65530` and `262144`.
* @property vmSwappiness The sysctl setting vm.swappiness. Must be between `0` and `100`.
* @property vmVfsCachePressure The sysctl setting vm.vfs_cache_pressure. Must be between `0` and `100`.
*/
public data class KubernetesClusterDefaultNodePoolLinuxOsConfigSysctlConfig(
public val fsAioMaxNr: Int? = null,
public val fsFileMax: Int? = null,
public val fsInotifyMaxUserWatches: Int? = null,
public val fsNrOpen: Int? = null,
public val kernelThreadsMax: Int? = null,
public val netCoreNetdevMaxBacklog: Int? = null,
public val netCoreOptmemMax: Int? = null,
public val netCoreRmemDefault: Int? = null,
public val netCoreRmemMax: Int? = null,
public val netCoreSomaxconn: Int? = null,
public val netCoreWmemDefault: Int? = null,
public val netCoreWmemMax: Int? = null,
public val netIpv4IpLocalPortRangeMax: Int? = null,
public val netIpv4IpLocalPortRangeMin: Int? = null,
public val netIpv4NeighDefaultGcThresh1: Int? = null,
public val netIpv4NeighDefaultGcThresh2: Int? = null,
public val netIpv4NeighDefaultGcThresh3: Int? = null,
public val netIpv4TcpFinTimeout: Int? = null,
public val netIpv4TcpKeepaliveIntvl: Int? = null,
public val netIpv4TcpKeepaliveProbes: Int? = null,
public val netIpv4TcpKeepaliveTime: Int? = null,
public val netIpv4TcpMaxSynBacklog: Int? = null,
public val netIpv4TcpMaxTwBuckets: Int? = null,
public val netIpv4TcpTwReuse: Boolean? = null,
public val netNetfilterNfConntrackBuckets: Int? = null,
public val netNetfilterNfConntrackMax: Int? = null,
public val vmMaxMapCount: Int? = null,
public val vmSwappiness: Int? = null,
public val vmVfsCachePressure: Int? = null,
) {
public companion object {
public
fun toKotlin(javaType: com.pulumi.azure.containerservice.outputs.KubernetesClusterDefaultNodePoolLinuxOsConfigSysctlConfig):
KubernetesClusterDefaultNodePoolLinuxOsConfigSysctlConfig =
KubernetesClusterDefaultNodePoolLinuxOsConfigSysctlConfig(
fsAioMaxNr = javaType.fsAioMaxNr().map({ args0 -> args0 }).orElse(null),
fsFileMax = javaType.fsFileMax().map({ args0 -> args0 }).orElse(null),
fsInotifyMaxUserWatches = javaType.fsInotifyMaxUserWatches().map({ args0 -> args0 }).orElse(null),
fsNrOpen = javaType.fsNrOpen().map({ args0 -> args0 }).orElse(null),
kernelThreadsMax = javaType.kernelThreadsMax().map({ args0 -> args0 }).orElse(null),
netCoreNetdevMaxBacklog = javaType.netCoreNetdevMaxBacklog().map({ args0 -> args0 }).orElse(null),
netCoreOptmemMax = javaType.netCoreOptmemMax().map({ args0 -> args0 }).orElse(null),
netCoreRmemDefault = javaType.netCoreRmemDefault().map({ args0 -> args0 }).orElse(null),
netCoreRmemMax = javaType.netCoreRmemMax().map({ args0 -> args0 }).orElse(null),
netCoreSomaxconn = javaType.netCoreSomaxconn().map({ args0 -> args0 }).orElse(null),
netCoreWmemDefault = javaType.netCoreWmemDefault().map({ args0 -> args0 }).orElse(null),
netCoreWmemMax = javaType.netCoreWmemMax().map({ args0 -> args0 }).orElse(null),
netIpv4IpLocalPortRangeMax = javaType.netIpv4IpLocalPortRangeMax().map({ args0 ->
args0
}).orElse(null),
netIpv4IpLocalPortRangeMin = javaType.netIpv4IpLocalPortRangeMin().map({ args0 ->
args0
}).orElse(null),
netIpv4NeighDefaultGcThresh1 = javaType.netIpv4NeighDefaultGcThresh1().map({ args0 ->
args0
}).orElse(null),
netIpv4NeighDefaultGcThresh2 = javaType.netIpv4NeighDefaultGcThresh2().map({ args0 ->
args0
}).orElse(null),
netIpv4NeighDefaultGcThresh3 = javaType.netIpv4NeighDefaultGcThresh3().map({ args0 ->
args0
}).orElse(null),
netIpv4TcpFinTimeout = javaType.netIpv4TcpFinTimeout().map({ args0 -> args0 }).orElse(null),
netIpv4TcpKeepaliveIntvl = javaType.netIpv4TcpKeepaliveIntvl().map({ args0 -> args0 }).orElse(null),
netIpv4TcpKeepaliveProbes = javaType.netIpv4TcpKeepaliveProbes().map({ args0 ->
args0
}).orElse(null),
netIpv4TcpKeepaliveTime = javaType.netIpv4TcpKeepaliveTime().map({ args0 -> args0 }).orElse(null),
netIpv4TcpMaxSynBacklog = javaType.netIpv4TcpMaxSynBacklog().map({ args0 -> args0 }).orElse(null),
netIpv4TcpMaxTwBuckets = javaType.netIpv4TcpMaxTwBuckets().map({ args0 -> args0 }).orElse(null),
netIpv4TcpTwReuse = javaType.netIpv4TcpTwReuse().map({ args0 -> args0 }).orElse(null),
netNetfilterNfConntrackBuckets = javaType.netNetfilterNfConntrackBuckets().map({ args0 ->
args0
}).orElse(null),
netNetfilterNfConntrackMax = javaType.netNetfilterNfConntrackMax().map({ args0 ->
args0
}).orElse(null),
vmMaxMapCount = javaType.vmMaxMapCount().map({ args0 -> args0 }).orElse(null),
vmSwappiness = javaType.vmSwappiness().map({ args0 -> args0 }).orElse(null),
vmVfsCachePressure = javaType.vmVfsCachePressure().map({ args0 -> args0 }).orElse(null),
)
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy