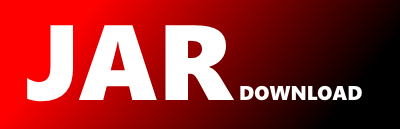
com.pulumi.azure.cosmosdb.kotlin.Account.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-kotlin Show documentation
Show all versions of pulumi-azure-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azure.cosmosdb.kotlin
import com.pulumi.azure.cosmosdb.kotlin.outputs.AccountAnalyticalStorage
import com.pulumi.azure.cosmosdb.kotlin.outputs.AccountBackup
import com.pulumi.azure.cosmosdb.kotlin.outputs.AccountCapability
import com.pulumi.azure.cosmosdb.kotlin.outputs.AccountCapacity
import com.pulumi.azure.cosmosdb.kotlin.outputs.AccountConsistencyPolicy
import com.pulumi.azure.cosmosdb.kotlin.outputs.AccountCorsRule
import com.pulumi.azure.cosmosdb.kotlin.outputs.AccountGeoLocation
import com.pulumi.azure.cosmosdb.kotlin.outputs.AccountIdentity
import com.pulumi.azure.cosmosdb.kotlin.outputs.AccountRestore
import com.pulumi.azure.cosmosdb.kotlin.outputs.AccountVirtualNetworkRule
import com.pulumi.core.Output
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.Deprecated
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.collections.Map
import com.pulumi.azure.cosmosdb.kotlin.outputs.AccountAnalyticalStorage.Companion.toKotlin as accountAnalyticalStorageToKotlin
import com.pulumi.azure.cosmosdb.kotlin.outputs.AccountBackup.Companion.toKotlin as accountBackupToKotlin
import com.pulumi.azure.cosmosdb.kotlin.outputs.AccountCapability.Companion.toKotlin as accountCapabilityToKotlin
import com.pulumi.azure.cosmosdb.kotlin.outputs.AccountCapacity.Companion.toKotlin as accountCapacityToKotlin
import com.pulumi.azure.cosmosdb.kotlin.outputs.AccountConsistencyPolicy.Companion.toKotlin as accountConsistencyPolicyToKotlin
import com.pulumi.azure.cosmosdb.kotlin.outputs.AccountCorsRule.Companion.toKotlin as accountCorsRuleToKotlin
import com.pulumi.azure.cosmosdb.kotlin.outputs.AccountGeoLocation.Companion.toKotlin as accountGeoLocationToKotlin
import com.pulumi.azure.cosmosdb.kotlin.outputs.AccountIdentity.Companion.toKotlin as accountIdentityToKotlin
import com.pulumi.azure.cosmosdb.kotlin.outputs.AccountRestore.Companion.toKotlin as accountRestoreToKotlin
import com.pulumi.azure.cosmosdb.kotlin.outputs.AccountVirtualNetworkRule.Companion.toKotlin as accountVirtualNetworkRuleToKotlin
/**
* Builder for [Account].
*/
@PulumiTagMarker
public class AccountResourceBuilder internal constructor() {
public var name: String? = null
public var args: AccountArgs = AccountArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend AccountArgsBuilder.() -> Unit) {
val builder = AccountArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): Account {
val builtJavaResource = com.pulumi.azure.cosmosdb.Account(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return Account(builtJavaResource)
}
}
/**
* Manages a CosmosDB (formally DocumentDB) Account.
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as azure from "@pulumi/azure";
* import * as random from "@pulumi/random";
* const rg = new azure.core.ResourceGroup("rg", {
* name: "sample-rg",
* location: "westus",
* });
* const ri = new random.RandomInteger("ri", {
* min: 10000,
* max: 99999,
* });
* const db = new azure.cosmosdb.Account("db", {
* name: pulumi.interpolate`tfex-cosmos-db-${ri.result}`,
* location: example.location,
* resourceGroupName: example.name,
* offerType: "Standard",
* kind: "MongoDB",
* enableAutomaticFailover: true,
* capabilities: [
* {
* name: "EnableAggregationPipeline",
* },
* {
* name: "mongoEnableDocLevelTTL",
* },
* {
* name: "MongoDBv3.4",
* },
* {
* name: "EnableMongo",
* },
* ],
* consistencyPolicy: {
* consistencyLevel: "BoundedStaleness",
* maxIntervalInSeconds: 300,
* maxStalenessPrefix: 100000,
* },
* geoLocations: [
* {
* location: "eastus",
* failoverPriority: 1,
* },
* {
* location: "westus",
* failoverPriority: 0,
* },
* ],
* });
* ```
* ```python
* import pulumi
* import pulumi_azure as azure
* import pulumi_random as random
* rg = azure.core.ResourceGroup("rg",
* name="sample-rg",
* location="westus")
* ri = random.RandomInteger("ri",
* min=10000,
* max=99999)
* db = azure.cosmosdb.Account("db",
* name=ri.result.apply(lambda result: f"tfex-cosmos-db-{result}"),
* location=example["location"],
* resource_group_name=example["name"],
* offer_type="Standard",
* kind="MongoDB",
* enable_automatic_failover=True,
* capabilities=[
* azure.cosmosdb.AccountCapabilityArgs(
* name="EnableAggregationPipeline",
* ),
* azure.cosmosdb.AccountCapabilityArgs(
* name="mongoEnableDocLevelTTL",
* ),
* azure.cosmosdb.AccountCapabilityArgs(
* name="MongoDBv3.4",
* ),
* azure.cosmosdb.AccountCapabilityArgs(
* name="EnableMongo",
* ),
* ],
* consistency_policy=azure.cosmosdb.AccountConsistencyPolicyArgs(
* consistency_level="BoundedStaleness",
* max_interval_in_seconds=300,
* max_staleness_prefix=100000,
* ),
* geo_locations=[
* azure.cosmosdb.AccountGeoLocationArgs(
* location="eastus",
* failover_priority=1,
* ),
* azure.cosmosdb.AccountGeoLocationArgs(
* location="westus",
* failover_priority=0,
* ),
* ])
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Azure = Pulumi.Azure;
* using Random = Pulumi.Random;
* return await Deployment.RunAsync(() =>
* {
* var rg = new Azure.Core.ResourceGroup("rg", new()
* {
* Name = "sample-rg",
* Location = "westus",
* });
* var ri = new Random.RandomInteger("ri", new()
* {
* Min = 10000,
* Max = 99999,
* });
* var db = new Azure.CosmosDB.Account("db", new()
* {
* Name = ri.Result.Apply(result => $"tfex-cosmos-db-{result}"),
* Location = example.Location,
* ResourceGroupName = example.Name,
* OfferType = "Standard",
* Kind = "MongoDB",
* EnableAutomaticFailover = true,
* Capabilities = new[]
* {
* new Azure.CosmosDB.Inputs.AccountCapabilityArgs
* {
* Name = "EnableAggregationPipeline",
* },
* new Azure.CosmosDB.Inputs.AccountCapabilityArgs
* {
* Name = "mongoEnableDocLevelTTL",
* },
* new Azure.CosmosDB.Inputs.AccountCapabilityArgs
* {
* Name = "MongoDBv3.4",
* },
* new Azure.CosmosDB.Inputs.AccountCapabilityArgs
* {
* Name = "EnableMongo",
* },
* },
* ConsistencyPolicy = new Azure.CosmosDB.Inputs.AccountConsistencyPolicyArgs
* {
* ConsistencyLevel = "BoundedStaleness",
* MaxIntervalInSeconds = 300,
* MaxStalenessPrefix = 100000,
* },
* GeoLocations = new[]
* {
* new Azure.CosmosDB.Inputs.AccountGeoLocationArgs
* {
* Location = "eastus",
* FailoverPriority = 1,
* },
* new Azure.CosmosDB.Inputs.AccountGeoLocationArgs
* {
* Location = "westus",
* FailoverPriority = 0,
* },
* },
* });
* });
* ```
* ```go
* package main
* import (
* "fmt"
* "github.com/pulumi/pulumi-azure/sdk/v5/go/azure/core"
* "github.com/pulumi/pulumi-azure/sdk/v5/go/azure/cosmosdb"
* "github.com/pulumi/pulumi-random/sdk/v4/go/random"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := core.NewResourceGroup(ctx, "rg", &core.ResourceGroupArgs{
* Name: pulumi.String("sample-rg"),
* Location: pulumi.String("westus"),
* })
* if err != nil {
* return err
* }
* ri, err := random.NewRandomInteger(ctx, "ri", &random.RandomIntegerArgs{
* Min: pulumi.Int(10000),
* Max: pulumi.Int(99999),
* })
* if err != nil {
* return err
* }
* _, err = cosmosdb.NewAccount(ctx, "db", &cosmosdb.AccountArgs{
* Name: ri.Result.ApplyT(func(result int) (string, error) {
* return fmt.Sprintf("tfex-cosmos-db-%v", result), nil
* }).(pulumi.StringOutput),
* Location: pulumi.Any(example.Location),
* ResourceGroupName: pulumi.Any(example.Name),
* OfferType: pulumi.String("Standard"),
* Kind: pulumi.String("MongoDB"),
* EnableAutomaticFailover: pulumi.Bool(true),
* Capabilities: cosmosdb.AccountCapabilityArray{
* &cosmosdb.AccountCapabilityArgs{
* Name: pulumi.String("EnableAggregationPipeline"),
* },
* &cosmosdb.AccountCapabilityArgs{
* Name: pulumi.String("mongoEnableDocLevelTTL"),
* },
* &cosmosdb.AccountCapabilityArgs{
* Name: pulumi.String("MongoDBv3.4"),
* },
* &cosmosdb.AccountCapabilityArgs{
* Name: pulumi.String("EnableMongo"),
* },
* },
* ConsistencyPolicy: &cosmosdb.AccountConsistencyPolicyArgs{
* ConsistencyLevel: pulumi.String("BoundedStaleness"),
* MaxIntervalInSeconds: pulumi.Int(300),
* MaxStalenessPrefix: pulumi.Int(100000),
* },
* GeoLocations: cosmosdb.AccountGeoLocationArray{
* &cosmosdb.AccountGeoLocationArgs{
* Location: pulumi.String("eastus"),
* FailoverPriority: pulumi.Int(1),
* },
* &cosmosdb.AccountGeoLocationArgs{
* Location: pulumi.String("westus"),
* FailoverPriority: pulumi.Int(0),
* },
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azure.core.ResourceGroup;
* import com.pulumi.azure.core.ResourceGroupArgs;
* import com.pulumi.random.RandomInteger;
* import com.pulumi.random.RandomIntegerArgs;
* import com.pulumi.azure.cosmosdb.Account;
* import com.pulumi.azure.cosmosdb.AccountArgs;
* import com.pulumi.azure.cosmosdb.inputs.AccountCapabilityArgs;
* import com.pulumi.azure.cosmosdb.inputs.AccountConsistencyPolicyArgs;
* import com.pulumi.azure.cosmosdb.inputs.AccountGeoLocationArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var rg = new ResourceGroup("rg", ResourceGroupArgs.builder()
* .name("sample-rg")
* .location("westus")
* .build());
* var ri = new RandomInteger("ri", RandomIntegerArgs.builder()
* .min(10000)
* .max(99999)
* .build());
* var db = new Account("db", AccountArgs.builder()
* .name(ri.result().applyValue(result -> String.format("tfex-cosmos-db-%s", result)))
* .location(example.location())
* .resourceGroupName(example.name())
* .offerType("Standard")
* .kind("MongoDB")
* .enableAutomaticFailover(true)
* .capabilities(
* AccountCapabilityArgs.builder()
* .name("EnableAggregationPipeline")
* .build(),
* AccountCapabilityArgs.builder()
* .name("mongoEnableDocLevelTTL")
* .build(),
* AccountCapabilityArgs.builder()
* .name("MongoDBv3.4")
* .build(),
* AccountCapabilityArgs.builder()
* .name("EnableMongo")
* .build())
* .consistencyPolicy(AccountConsistencyPolicyArgs.builder()
* .consistencyLevel("BoundedStaleness")
* .maxIntervalInSeconds(300)
* .maxStalenessPrefix(100000)
* .build())
* .geoLocations(
* AccountGeoLocationArgs.builder()
* .location("eastus")
* .failoverPriority(1)
* .build(),
* AccountGeoLocationArgs.builder()
* .location("westus")
* .failoverPriority(0)
* .build())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* rg:
* type: azure:core:ResourceGroup
* properties:
* name: sample-rg
* location: westus
* ri:
* type: random:RandomInteger
* properties:
* min: 10000
* max: 99999
* db:
* type: azure:cosmosdb:Account
* properties:
* name: tfex-cosmos-db-${ri.result}
* location: ${example.location}
* resourceGroupName: ${example.name}
* offerType: Standard
* kind: MongoDB
* enableAutomaticFailover: true
* capabilities:
* - name: EnableAggregationPipeline
* - name: mongoEnableDocLevelTTL
* - name: MongoDBv3.4
* - name: EnableMongo
* consistencyPolicy:
* consistencyLevel: BoundedStaleness
* maxIntervalInSeconds: 300
* maxStalenessPrefix: 100000
* geoLocations:
* - location: eastus
* failoverPriority: 1
* - location: westus
* failoverPriority: 0
* ```
*
* ## User Assigned Identity Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as azure from "@pulumi/azure";
* import * as std from "@pulumi/std";
* const example = new azure.authorization.UserAssignedIdentity("example", {
* resourceGroupName: exampleAzurermResourceGroup.name,
* location: exampleAzurermResourceGroup.location,
* name: "example-resource",
* });
* const exampleAccount = new azure.cosmosdb.Account("example", {
* name: "example-resource",
* location: exampleAzurermResourceGroup.location,
* resourceGroupName: exampleAzurermResourceGroup.name,
* defaultIdentityType: std.joinOutput({
* separator: "=",
* input: [
* "UserAssignedIdentity",
* example.id,
* ],
* }).apply(invoke => invoke.result),
* offerType: "Standard",
* kind: "MongoDB",
* capabilities: [{
* name: "EnableMongo",
* }],
* consistencyPolicy: {
* consistencyLevel: "Strong",
* },
* geoLocations: [{
* location: "westus",
* failoverPriority: 0,
* }],
* identity: {
* type: "UserAssigned",
* identityIds: [example.id],
* },
* });
* ```
* ```python
* import pulumi
* import pulumi_azure as azure
* import pulumi_std as std
* example = azure.authorization.UserAssignedIdentity("example",
* resource_group_name=example_azurerm_resource_group["name"],
* location=example_azurerm_resource_group["location"],
* name="example-resource")
* example_account = azure.cosmosdb.Account("example",
* name="example-resource",
* location=example_azurerm_resource_group["location"],
* resource_group_name=example_azurerm_resource_group["name"],
* default_identity_type=std.join_output(separator="=",
* input=[
* "UserAssignedIdentity",
* example.id,
* ]).apply(lambda invoke: invoke.result),
* offer_type="Standard",
* kind="MongoDB",
* capabilities=[azure.cosmosdb.AccountCapabilityArgs(
* name="EnableMongo",
* )],
* consistency_policy=azure.cosmosdb.AccountConsistencyPolicyArgs(
* consistency_level="Strong",
* ),
* geo_locations=[azure.cosmosdb.AccountGeoLocationArgs(
* location="westus",
* failover_priority=0,
* )],
* identity=azure.cosmosdb.AccountIdentityArgs(
* type="UserAssigned",
* identity_ids=[example.id],
* ))
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Azure = Pulumi.Azure;
* using Std = Pulumi.Std;
* return await Deployment.RunAsync(() =>
* {
* var example = new Azure.Authorization.UserAssignedIdentity("example", new()
* {
* ResourceGroupName = exampleAzurermResourceGroup.Name,
* Location = exampleAzurermResourceGroup.Location,
* Name = "example-resource",
* });
* var exampleAccount = new Azure.CosmosDB.Account("example", new()
* {
* Name = "example-resource",
* Location = exampleAzurermResourceGroup.Location,
* ResourceGroupName = exampleAzurermResourceGroup.Name,
* DefaultIdentityType = Std.Join.Invoke(new()
* {
* Separator = "=",
* Input = new[]
* {
* "UserAssignedIdentity",
* example.Id,
* },
* }).Apply(invoke => invoke.Result),
* OfferType = "Standard",
* Kind = "MongoDB",
* Capabilities = new[]
* {
* new Azure.CosmosDB.Inputs.AccountCapabilityArgs
* {
* Name = "EnableMongo",
* },
* },
* ConsistencyPolicy = new Azure.CosmosDB.Inputs.AccountConsistencyPolicyArgs
* {
* ConsistencyLevel = "Strong",
* },
* GeoLocations = new[]
* {
* new Azure.CosmosDB.Inputs.AccountGeoLocationArgs
* {
* Location = "westus",
* FailoverPriority = 0,
* },
* },
* Identity = new Azure.CosmosDB.Inputs.AccountIdentityArgs
* {
* Type = "UserAssigned",
* IdentityIds = new[]
* {
* example.Id,
* },
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-azure/sdk/v5/go/azure/authorization"
* "github.com/pulumi/pulumi-azure/sdk/v5/go/azure/cosmosdb"
* "github.com/pulumi/pulumi-std/sdk/go/std"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* example, err := authorization.NewUserAssignedIdentity(ctx, "example", &authorization.UserAssignedIdentityArgs{
* ResourceGroupName: pulumi.Any(exampleAzurermResourceGroup.Name),
* Location: pulumi.Any(exampleAzurermResourceGroup.Location),
* Name: pulumi.String("example-resource"),
* })
* if err != nil {
* return err
* }
* _, err = cosmosdb.NewAccount(ctx, "example", &cosmosdb.AccountArgs{
* Name: pulumi.String("example-resource"),
* Location: pulumi.Any(exampleAzurermResourceGroup.Location),
* ResourceGroupName: pulumi.Any(exampleAzurermResourceGroup.Name),
* DefaultIdentityType: std.JoinOutput(ctx, std.JoinOutputArgs{
* Separator: pulumi.String("="),
* Input: pulumi.StringArray{
* pulumi.String("UserAssignedIdentity"),
* example.ID(),
* },
* }, nil).ApplyT(func(invoke std.JoinResult) (*string, error) {
* return invoke.Result, nil
* }).(pulumi.StringPtrOutput),
* OfferType: pulumi.String("Standard"),
* Kind: pulumi.String("MongoDB"),
* Capabilities: cosmosdb.AccountCapabilityArray{
* &cosmosdb.AccountCapabilityArgs{
* Name: pulumi.String("EnableMongo"),
* },
* },
* ConsistencyPolicy: &cosmosdb.AccountConsistencyPolicyArgs{
* ConsistencyLevel: pulumi.String("Strong"),
* },
* GeoLocations: cosmosdb.AccountGeoLocationArray{
* &cosmosdb.AccountGeoLocationArgs{
* Location: pulumi.String("westus"),
* FailoverPriority: pulumi.Int(0),
* },
* },
* Identity: &cosmosdb.AccountIdentityArgs{
* Type: pulumi.String("UserAssigned"),
* IdentityIds: pulumi.StringArray{
* example.ID(),
* },
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azure.authorization.UserAssignedIdentity;
* import com.pulumi.azure.authorization.UserAssignedIdentityArgs;
* import com.pulumi.azure.cosmosdb.Account;
* import com.pulumi.azure.cosmosdb.AccountArgs;
* import com.pulumi.azure.cosmosdb.inputs.AccountCapabilityArgs;
* import com.pulumi.azure.cosmosdb.inputs.AccountConsistencyPolicyArgs;
* import com.pulumi.azure.cosmosdb.inputs.AccountGeoLocationArgs;
* import com.pulumi.azure.cosmosdb.inputs.AccountIdentityArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var example = new UserAssignedIdentity("example", UserAssignedIdentityArgs.builder()
* .resourceGroupName(exampleAzurermResourceGroup.name())
* .location(exampleAzurermResourceGroup.location())
* .name("example-resource")
* .build());
* var exampleAccount = new Account("exampleAccount", AccountArgs.builder()
* .name("example-resource")
* .location(exampleAzurermResourceGroup.location())
* .resourceGroupName(exampleAzurermResourceGroup.name())
* .defaultIdentityType(StdFunctions.join().applyValue(invoke -> invoke.result()))
* .offerType("Standard")
* .kind("MongoDB")
* .capabilities(AccountCapabilityArgs.builder()
* .name("EnableMongo")
* .build())
* .consistencyPolicy(AccountConsistencyPolicyArgs.builder()
* .consistencyLevel("Strong")
* .build())
* .geoLocations(AccountGeoLocationArgs.builder()
* .location("westus")
* .failoverPriority(0)
* .build())
* .identity(AccountIdentityArgs.builder()
* .type("UserAssigned")
* .identityIds(example.id())
* .build())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* example:
* type: azure:authorization:UserAssignedIdentity
* properties:
* resourceGroupName: ${exampleAzurermResourceGroup.name}
* location: ${exampleAzurermResourceGroup.location}
* name: example-resource
* exampleAccount:
* type: azure:cosmosdb:Account
* name: example
* properties:
* name: example-resource
* location: ${exampleAzurermResourceGroup.location}
* resourceGroupName: ${exampleAzurermResourceGroup.name}
* defaultIdentityType:
* fn::invoke:
* Function: std:join
* Arguments:
* separator: =
* input:
* - UserAssignedIdentity
* - ${example.id}
* Return: result
* offerType: Standard
* kind: MongoDB
* capabilities:
* - name: EnableMongo
* consistencyPolicy:
* consistencyLevel: Strong
* geoLocations:
* - location: westus
* failoverPriority: 0
* identity:
* type: UserAssigned
* identityIds:
* - ${example.id}
* ```
*
* ## Import
* CosmosDB Accounts can be imported using the `resource id`, e.g.
* ```sh
* $ pulumi import azure:cosmosdb/account:Account account1 /subscriptions/00000000-0000-0000-0000-000000000000/resourceGroups/group1/providers/Microsoft.DocumentDB/databaseAccounts/account1
* ```
*/
public class Account internal constructor(
override val javaResource: com.pulumi.azure.cosmosdb.Account,
) : KotlinCustomResource(javaResource, AccountMapper) {
public val accessKeyMetadataWritesEnabled: Output?
get() = javaResource.accessKeyMetadataWritesEnabled().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* An `analytical_storage` block as defined below.
*/
public val analyticalStorage: Output
get() = javaResource.analyticalStorage().applyValue({ args0 ->
args0.let({ args0 ->
accountAnalyticalStorageToKotlin(args0)
})
})
public val analyticalStorageEnabled: Output?
get() = javaResource.analyticalStorageEnabled().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
public val automaticFailoverEnabled: Output
get() = javaResource.automaticFailoverEnabled().applyValue({ args0 -> args0 })
public val backup: Output
get() = javaResource.backup().applyValue({ args0 ->
args0.let({ args0 ->
accountBackupToKotlin(args0)
})
})
public val capabilities: Output>
get() = javaResource.capabilities().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
accountCapabilityToKotlin(args0)
})
})
})
/**
* A `capacity` block as defined below.
*/
public val capacity: Output
get() = javaResource.capacity().applyValue({ args0 ->
args0.let({ args0 ->
accountCapacityToKotlin(args0)
})
})
@Deprecated(
message = """
This property has been superseded by the primary and secondary connection strings for sql, mongodb
and readonly and will be removed in v4.0 of the AzureRM provider
""",
)
public val connectionStrings: Output>
get() = javaResource.connectionStrings().applyValue({ args0 -> args0.map({ args0 -> args0 }) })
public val consistencyPolicy: Output
get() = javaResource.consistencyPolicy().applyValue({ args0 ->
args0.let({ args0 ->
accountConsistencyPolicyToKotlin(args0)
})
})
public val corsRule: Output?
get() = javaResource.corsRule().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
accountCorsRuleToKotlin(args0)
})
}).orElse(null)
})
/**
* The creation mode for the CosmosDB Account. Possible values are `Default` and `Restore`. Changing this forces a new resource to be created.
* > **Note:** `create_mode` can only be defined when the `backup.type` is set to `Continuous`.
*/
public val createMode: Output
get() = javaResource.createMode().applyValue({ args0 -> args0 })
/**
* The default identity for accessing Key Vault. Possible values are `FirstPartyIdentity`, `SystemAssignedIdentity` or `UserAssignedIdentity`. Defaults to `FirstPartyIdentity`.
*/
public val defaultIdentityType: Output?
get() = javaResource.defaultIdentityType().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
@Deprecated(
message = """
This property has been superseded by `automatic_failover_enabled` and will be removed in v4.0 of
the AzureRM Provider
""",
)
public val enableAutomaticFailover: Output
get() = javaResource.enableAutomaticFailover().applyValue({ args0 -> args0 })
@Deprecated(
message = """
This property has been superseded by `free_tier_enabled` and will be removed in v4.0 of the
AzureRM Provider
""",
)
public val enableFreeTier: Output
get() = javaResource.enableFreeTier().applyValue({ args0 -> args0 })
@Deprecated(
message = """
This property has been superseded by `multiple_write_locations_enabled` and will be removed in
v4.0 of the AzureRM Provider
""",
)
public val enableMultipleWriteLocations: Output
get() = javaResource.enableMultipleWriteLocations().applyValue({ args0 -> args0 })
/**
* The endpoint used to connect to the CosmosDB account.
*/
public val endpoint: Output
get() = javaResource.endpoint().applyValue({ args0 -> args0 })
public val freeTierEnabled: Output
get() = javaResource.freeTierEnabled().applyValue({ args0 -> args0 })
public val geoLocations: Output>
get() = javaResource.geoLocations().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
accountGeoLocationToKotlin(args0)
})
})
})
public val identity: Output?
get() = javaResource.identity().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
accountIdentityToKotlin(args0)
})
}).orElse(null)
})
public val ipRangeFilter: Output?
get() = javaResource.ipRangeFilter().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
public val isVirtualNetworkFilterEnabled: Output?
get() = javaResource.isVirtualNetworkFilterEnabled().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
public val keyVaultKeyId: Output?
get() = javaResource.keyVaultKeyId().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
public val kind: Output?
get() = javaResource.kind().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
public val localAuthenticationDisabled: Output?
get() = javaResource.localAuthenticationDisabled().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Specifies the supported Azure location where the resource exists. Changing this forces a new resource to be created.
*/
public val location: Output
get() = javaResource.location().applyValue({ args0 -> args0 })
/**
* Specifies the minimal TLS version for the CosmosDB account. Possible values are: `Tls`, `Tls11`, and `Tls12`. Defaults to `Tls12`.
*/
public val minimalTlsVersion: Output
get() = javaResource.minimalTlsVersion().applyValue({ args0 -> args0 })
public val mongoServerVersion: Output
get() = javaResource.mongoServerVersion().applyValue({ args0 -> args0 })
public val multipleWriteLocationsEnabled: Output
get() = javaResource.multipleWriteLocationsEnabled().applyValue({ args0 -> args0 })
/**
* Specifies the name of the CosmosDB Account. Changing this forces a new resource to be created.
*/
public val name: Output
get() = javaResource.name().applyValue({ args0 -> args0 })
public val networkAclBypassForAzureServices: Output?
get() = javaResource.networkAclBypassForAzureServices().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
public val networkAclBypassIds: Output>?
get() = javaResource.networkAclBypassIds().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 -> args0 })
}).orElse(null)
})
/**
* Specifies the Offer Type to use for this CosmosDB Account; currently, this can only be set to `Standard`.
*/
public val offerType: Output
get() = javaResource.offerType().applyValue({ args0 -> args0 })
public val partitionMergeEnabled: Output?
get() = javaResource.partitionMergeEnabled().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The Primary key for the CosmosDB Account.
*/
public val primaryKey: Output
get() = javaResource.primaryKey().applyValue({ args0 -> args0 })
/**
* Primary Mongodb connection string for the CosmosDB Account.
*/
public val primaryMongodbConnectionString: Output
get() = javaResource.primaryMongodbConnectionString().applyValue({ args0 -> args0 })
/**
* The Primary read-only Key for the CosmosDB Account.
*/
public val primaryReadonlyKey: Output
get() = javaResource.primaryReadonlyKey().applyValue({ args0 -> args0 })
/**
* Primary readonly Mongodb connection string for the CosmosDB Account.
*/
public val primaryReadonlyMongodbConnectionString: Output
get() = javaResource.primaryReadonlyMongodbConnectionString().applyValue({ args0 -> args0 })
/**
* Primary readonly SQL connection string for the CosmosDB Account.
*/
public val primaryReadonlySqlConnectionString: Output
get() = javaResource.primaryReadonlySqlConnectionString().applyValue({ args0 -> args0 })
/**
* Primary SQL connection string for the CosmosDB Account.
*/
public val primarySqlConnectionString: Output
get() = javaResource.primarySqlConnectionString().applyValue({ args0 -> args0 })
public val publicNetworkAccessEnabled: Output?
get() = javaResource.publicNetworkAccessEnabled().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* A list of read endpoints available for this CosmosDB account.
*/
public val readEndpoints: Output>
get() = javaResource.readEndpoints().applyValue({ args0 -> args0.map({ args0 -> args0 }) })
/**
* The name of the resource group in which the CosmosDB Account is created. Changing this forces a new resource to be created.
*/
public val resourceGroupName: Output
get() = javaResource.resourceGroupName().applyValue({ args0 -> args0 })
public val restore: Output?
get() = javaResource.restore().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
accountRestoreToKotlin(args0)
})
}).orElse(null)
})
/**
* The Secondary key for the CosmosDB Account.
*/
public val secondaryKey: Output
get() = javaResource.secondaryKey().applyValue({ args0 -> args0 })
/**
* Secondary Mongodb connection string for the CosmosDB Account.
*/
public val secondaryMongodbConnectionString: Output
get() = javaResource.secondaryMongodbConnectionString().applyValue({ args0 -> args0 })
/**
* The Secondary read-only key for the CosmosDB Account.
*/
public val secondaryReadonlyKey: Output
get() = javaResource.secondaryReadonlyKey().applyValue({ args0 -> args0 })
/**
* Secondary readonly Mongodb connection string for the CosmosDB Account.
*/
public val secondaryReadonlyMongodbConnectionString: Output
get() = javaResource.secondaryReadonlyMongodbConnectionString().applyValue({ args0 -> args0 })
/**
* Secondary readonly SQL connection string for the CosmosDB Account.
*/
public val secondaryReadonlySqlConnectionString: Output
get() = javaResource.secondaryReadonlySqlConnectionString().applyValue({ args0 -> args0 })
/**
* Secondary SQL connection string for the CosmosDB Account.
*/
public val secondarySqlConnectionString: Output
get() = javaResource.secondarySqlConnectionString().applyValue({ args0 -> args0 })
/**
* A mapping of tags to assign to the resource.
*/
public val tags: Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy