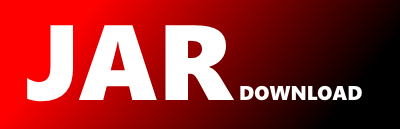
com.pulumi.azure.datadog.kotlin.inputs.MonitorDatadogOrganizationArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-kotlin Show documentation
Show all versions of pulumi-azure-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azure.datadog.kotlin.inputs
import com.pulumi.azure.datadog.inputs.MonitorDatadogOrganizationArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
*
* @property apiKey Api key associated to the Datadog organization. Changing this forces a new Datadog Monitor to be created.
* @property applicationKey Application key associated to the Datadog organization. Changing this forces a new Datadog Monitor to be created.
* @property enterpriseAppId The ID of the enterprise_app. Changing this forces a new resource to be created.
* @property id The ID of the Datadog Monitor.
* @property linkingAuthCode The auth code used to linking to an existing Datadog organization. Changing this forces a new Datadog Monitor to be created.
* @property linkingClientId The ID of the linking_client. Changing this forces a new Datadog Monitor to be created.
* @property name The name of the user that will be associated with the Datadog Monitor. Changing this forces a new Datadog Monitor to be created.
* @property redirectUri The redirect uri for linking. Changing this forces a new Datadog Monitor to be created.
*/
public data class MonitorDatadogOrganizationArgs(
public val apiKey: Output,
public val applicationKey: Output,
public val enterpriseAppId: Output? = null,
public val id: Output? = null,
public val linkingAuthCode: Output? = null,
public val linkingClientId: Output? = null,
public val name: Output? = null,
public val redirectUri: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azure.datadog.inputs.MonitorDatadogOrganizationArgs =
com.pulumi.azure.datadog.inputs.MonitorDatadogOrganizationArgs.builder()
.apiKey(apiKey.applyValue({ args0 -> args0 }))
.applicationKey(applicationKey.applyValue({ args0 -> args0 }))
.enterpriseAppId(enterpriseAppId?.applyValue({ args0 -> args0 }))
.id(id?.applyValue({ args0 -> args0 }))
.linkingAuthCode(linkingAuthCode?.applyValue({ args0 -> args0 }))
.linkingClientId(linkingClientId?.applyValue({ args0 -> args0 }))
.name(name?.applyValue({ args0 -> args0 }))
.redirectUri(redirectUri?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [MonitorDatadogOrganizationArgs].
*/
@PulumiTagMarker
public class MonitorDatadogOrganizationArgsBuilder internal constructor() {
private var apiKey: Output? = null
private var applicationKey: Output? = null
private var enterpriseAppId: Output? = null
private var id: Output? = null
private var linkingAuthCode: Output? = null
private var linkingClientId: Output? = null
private var name: Output? = null
private var redirectUri: Output? = null
/**
* @param value Api key associated to the Datadog organization. Changing this forces a new Datadog Monitor to be created.
*/
@JvmName("oaaivmixcdhrgrdh")
public suspend fun apiKey(`value`: Output) {
this.apiKey = value
}
/**
* @param value Application key associated to the Datadog organization. Changing this forces a new Datadog Monitor to be created.
*/
@JvmName("crpprglgnrrbgmgv")
public suspend fun applicationKey(`value`: Output) {
this.applicationKey = value
}
/**
* @param value The ID of the enterprise_app. Changing this forces a new resource to be created.
*/
@JvmName("vdykajrmpyjnrfjk")
public suspend fun enterpriseAppId(`value`: Output) {
this.enterpriseAppId = value
}
/**
* @param value The ID of the Datadog Monitor.
*/
@JvmName("prjkosjhwfroambs")
public suspend fun id(`value`: Output) {
this.id = value
}
/**
* @param value The auth code used to linking to an existing Datadog organization. Changing this forces a new Datadog Monitor to be created.
*/
@JvmName("bdrquslsxsvxohcy")
public suspend fun linkingAuthCode(`value`: Output) {
this.linkingAuthCode = value
}
/**
* @param value The ID of the linking_client. Changing this forces a new Datadog Monitor to be created.
*/
@JvmName("gyoesumiraucwhfl")
public suspend fun linkingClientId(`value`: Output) {
this.linkingClientId = value
}
/**
* @param value The name of the user that will be associated with the Datadog Monitor. Changing this forces a new Datadog Monitor to be created.
*/
@JvmName("rhttecsfkcoirbpj")
public suspend fun name(`value`: Output) {
this.name = value
}
/**
* @param value The redirect uri for linking. Changing this forces a new Datadog Monitor to be created.
*/
@JvmName("ytcdibxplcbiawcy")
public suspend fun redirectUri(`value`: Output) {
this.redirectUri = value
}
/**
* @param value Api key associated to the Datadog organization. Changing this forces a new Datadog Monitor to be created.
*/
@JvmName("fdqixtumaikjuulx")
public suspend fun apiKey(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.apiKey = mapped
}
/**
* @param value Application key associated to the Datadog organization. Changing this forces a new Datadog Monitor to be created.
*/
@JvmName("jrmtbhgjayctehpl")
public suspend fun applicationKey(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.applicationKey = mapped
}
/**
* @param value The ID of the enterprise_app. Changing this forces a new resource to be created.
*/
@JvmName("npurmfqykloggbjg")
public suspend fun enterpriseAppId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.enterpriseAppId = mapped
}
/**
* @param value The ID of the Datadog Monitor.
*/
@JvmName("bbgpwrdxbgvsesqj")
public suspend fun id(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.id = mapped
}
/**
* @param value The auth code used to linking to an existing Datadog organization. Changing this forces a new Datadog Monitor to be created.
*/
@JvmName("vnugptmqcqimhiow")
public suspend fun linkingAuthCode(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.linkingAuthCode = mapped
}
/**
* @param value The ID of the linking_client. Changing this forces a new Datadog Monitor to be created.
*/
@JvmName("qnkxnsweadrhuaii")
public suspend fun linkingClientId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.linkingClientId = mapped
}
/**
* @param value The name of the user that will be associated with the Datadog Monitor. Changing this forces a new Datadog Monitor to be created.
*/
@JvmName("cxlgcrafftybyhkr")
public suspend fun name(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.name = mapped
}
/**
* @param value The redirect uri for linking. Changing this forces a new Datadog Monitor to be created.
*/
@JvmName("kcyqwrilwqdtbhic")
public suspend fun redirectUri(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.redirectUri = mapped
}
internal fun build(): MonitorDatadogOrganizationArgs = MonitorDatadogOrganizationArgs(
apiKey = apiKey ?: throw PulumiNullFieldException("apiKey"),
applicationKey = applicationKey ?: throw PulumiNullFieldException("applicationKey"),
enterpriseAppId = enterpriseAppId,
id = id,
linkingAuthCode = linkingAuthCode,
linkingClientId = linkingClientId,
name = name,
redirectUri = redirectUri,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy