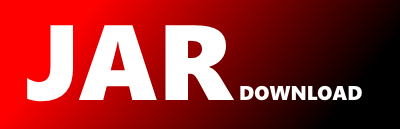
com.pulumi.azure.datafactory.kotlin.DatafactoryFunctions.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-kotlin Show documentation
Show all versions of pulumi-azure-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azure.datafactory.kotlin
import com.pulumi.azure.datafactory.DatafactoryFunctions.getFactoryPlain
import com.pulumi.azure.datafactory.DatafactoryFunctions.getTriggerSchedulePlain
import com.pulumi.azure.datafactory.DatafactoryFunctions.getTriggerSchedulesPlain
import com.pulumi.azure.datafactory.kotlin.inputs.GetFactoryPlainArgs
import com.pulumi.azure.datafactory.kotlin.inputs.GetFactoryPlainArgsBuilder
import com.pulumi.azure.datafactory.kotlin.inputs.GetTriggerSchedulePlainArgs
import com.pulumi.azure.datafactory.kotlin.inputs.GetTriggerSchedulePlainArgsBuilder
import com.pulumi.azure.datafactory.kotlin.inputs.GetTriggerSchedulesPlainArgs
import com.pulumi.azure.datafactory.kotlin.inputs.GetTriggerSchedulesPlainArgsBuilder
import com.pulumi.azure.datafactory.kotlin.outputs.GetFactoryResult
import com.pulumi.azure.datafactory.kotlin.outputs.GetTriggerScheduleResult
import com.pulumi.azure.datafactory.kotlin.outputs.GetTriggerSchedulesResult
import kotlinx.coroutines.future.await
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import com.pulumi.azure.datafactory.kotlin.outputs.GetFactoryResult.Companion.toKotlin as getFactoryResultToKotlin
import com.pulumi.azure.datafactory.kotlin.outputs.GetTriggerScheduleResult.Companion.toKotlin as getTriggerScheduleResultToKotlin
import com.pulumi.azure.datafactory.kotlin.outputs.GetTriggerSchedulesResult.Companion.toKotlin as getTriggerSchedulesResultToKotlin
public object DatafactoryFunctions {
/**
* Use this data source to access information about an existing Azure Data Factory (Version 2).
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as azure from "@pulumi/azure";
* const example = azure.datafactory.getFactory({
* name: "existing-adf",
* resourceGroupName: "existing-rg",
* });
* export const id = example.then(example => example.id);
* ```
* ```python
* import pulumi
* import pulumi_azure as azure
* example = azure.datafactory.get_factory(name="existing-adf",
* resource_group_name="existing-rg")
* pulumi.export("id", example.id)
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Azure = Pulumi.Azure;
* return await Deployment.RunAsync(() =>
* {
* var example = Azure.DataFactory.GetFactory.Invoke(new()
* {
* Name = "existing-adf",
* ResourceGroupName = "existing-rg",
* });
* return new Dictionary
* {
* ["id"] = example.Apply(getFactoryResult => getFactoryResult.Id),
* };
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-azure/sdk/v5/go/azure/datafactory"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* example, err := datafactory.LookupFactory(ctx, &datafactory.LookupFactoryArgs{
* Name: "existing-adf",
* ResourceGroupName: "existing-rg",
* }, nil)
* if err != nil {
* return err
* }
* ctx.Export("id", example.Id)
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azure.datafactory.DatafactoryFunctions;
* import com.pulumi.azure.datafactory.inputs.GetFactoryArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* final var example = DatafactoryFunctions.getFactory(GetFactoryArgs.builder()
* .name("existing-adf")
* .resourceGroupName("existing-rg")
* .build());
* ctx.export("id", example.applyValue(getFactoryResult -> getFactoryResult.id()));
* }
* }
* ```
* ```yaml
* variables:
* example:
* fn::invoke:
* Function: azure:datafactory:getFactory
* Arguments:
* name: existing-adf
* resourceGroupName: existing-rg
* outputs:
* id: ${example.id}
* ```
*
* @param argument A collection of arguments for invoking getFactory.
* @return A collection of values returned by getFactory.
*/
public suspend fun getFactory(argument: GetFactoryPlainArgs): GetFactoryResult =
getFactoryResultToKotlin(getFactoryPlain(argument.toJava()).await())
/**
* @see [getFactory].
* @param name The name of this Azure Data Factory.
* @param resourceGroupName The name of the Resource Group where the Azure Data Factory exists.
* @return A collection of values returned by getFactory.
*/
public suspend fun getFactory(name: String, resourceGroupName: String): GetFactoryResult {
val argument = GetFactoryPlainArgs(
name = name,
resourceGroupName = resourceGroupName,
)
return getFactoryResultToKotlin(getFactoryPlain(argument.toJava()).await())
}
/**
* @see [getFactory].
* @param argument Builder for [com.pulumi.azure.datafactory.kotlin.inputs.GetFactoryPlainArgs].
* @return A collection of values returned by getFactory.
*/
public suspend fun getFactory(argument: suspend GetFactoryPlainArgsBuilder.() -> Unit):
GetFactoryResult {
val builder = GetFactoryPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getFactoryResultToKotlin(getFactoryPlain(builtArgument.toJava()).await())
}
/**
* Use this data source to access information about a trigger schedule in Azure Data Factory.
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as azure from "@pulumi/azure";
* const example = azure.datafactory.getTriggerSchedule({
* name: "example_trigger",
* dataFactoryId: "/subscriptions/00000000-0000-0000-0000-000000000000/resourceGroups/rg1/providers/Microsoft.DataFactory/factories/datafactory1",
* });
* export const id = example.then(example => example.id);
* ```
* ```python
* import pulumi
* import pulumi_azure as azure
* example = azure.datafactory.get_trigger_schedule(name="example_trigger",
* data_factory_id="/subscriptions/00000000-0000-0000-0000-000000000000/resourceGroups/rg1/providers/Microsoft.DataFactory/factories/datafactory1")
* pulumi.export("id", example.id)
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Azure = Pulumi.Azure;
* return await Deployment.RunAsync(() =>
* {
* var example = Azure.DataFactory.GetTriggerSchedule.Invoke(new()
* {
* Name = "example_trigger",
* DataFactoryId = "/subscriptions/00000000-0000-0000-0000-000000000000/resourceGroups/rg1/providers/Microsoft.DataFactory/factories/datafactory1",
* });
* return new Dictionary
* {
* ["id"] = example.Apply(getTriggerScheduleResult => getTriggerScheduleResult.Id),
* };
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-azure/sdk/v5/go/azure/datafactory"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* example, err := datafactory.LookupTriggerSchedule(ctx, &datafactory.LookupTriggerScheduleArgs{
* Name: "example_trigger",
* DataFactoryId: "/subscriptions/00000000-0000-0000-0000-000000000000/resourceGroups/rg1/providers/Microsoft.DataFactory/factories/datafactory1",
* }, nil)
* if err != nil {
* return err
* }
* ctx.Export("id", example.Id)
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azure.datafactory.DatafactoryFunctions;
* import com.pulumi.azure.datafactory.inputs.GetTriggerScheduleArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* final var example = DatafactoryFunctions.getTriggerSchedule(GetTriggerScheduleArgs.builder()
* .name("example_trigger")
* .dataFactoryId("/subscriptions/00000000-0000-0000-0000-000000000000/resourceGroups/rg1/providers/Microsoft.DataFactory/factories/datafactory1")
* .build());
* ctx.export("id", example.applyValue(getTriggerScheduleResult -> getTriggerScheduleResult.id()));
* }
* }
* ```
* ```yaml
* variables:
* example:
* fn::invoke:
* Function: azure:datafactory:getTriggerSchedule
* Arguments:
* name: example_trigger
* dataFactoryId: /subscriptions/00000000-0000-0000-0000-000000000000/resourceGroups/rg1/providers/Microsoft.DataFactory/factories/datafactory1
* outputs:
* id: ${example.id}
* ```
*
* @param argument A collection of arguments for invoking getTriggerSchedule.
* @return A collection of values returned by getTriggerSchedule.
*/
public suspend fun getTriggerSchedule(argument: GetTriggerSchedulePlainArgs):
GetTriggerScheduleResult =
getTriggerScheduleResultToKotlin(getTriggerSchedulePlain(argument.toJava()).await())
/**
* @see [getTriggerSchedule].
* @param dataFactoryId The ID of the Azure Data Factory to fetch trigger schedule from.
* @param name The name of the trigger schedule.
* @return A collection of values returned by getTriggerSchedule.
*/
public suspend fun getTriggerSchedule(dataFactoryId: String, name: String):
GetTriggerScheduleResult {
val argument = GetTriggerSchedulePlainArgs(
dataFactoryId = dataFactoryId,
name = name,
)
return getTriggerScheduleResultToKotlin(getTriggerSchedulePlain(argument.toJava()).await())
}
/**
* @see [getTriggerSchedule].
* @param argument Builder for [com.pulumi.azure.datafactory.kotlin.inputs.GetTriggerSchedulePlainArgs].
* @return A collection of values returned by getTriggerSchedule.
*/
public suspend
fun getTriggerSchedule(argument: suspend GetTriggerSchedulePlainArgsBuilder.() -> Unit):
GetTriggerScheduleResult {
val builder = GetTriggerSchedulePlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getTriggerScheduleResultToKotlin(getTriggerSchedulePlain(builtArgument.toJava()).await())
}
/**
* Use this data source to access information about all existing trigger schedules in Azure Data Factory.
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as azure from "@pulumi/azure";
* const example = azure.datafactory.getTriggerSchedules({
* dataFactoryId: "/subscriptions/00000000-0000-0000-0000-000000000000/resourceGroups/rg1/providers/Microsoft.DataFactory/factories/datafactory1",
* });
* export const items = example.then(example => example.items);
* ```
* ```python
* import pulumi
* import pulumi_azure as azure
* example = azure.datafactory.get_trigger_schedules(data_factory_id="/subscriptions/00000000-0000-0000-0000-000000000000/resourceGroups/rg1/providers/Microsoft.DataFactory/factories/datafactory1")
* pulumi.export("items", example.items)
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Azure = Pulumi.Azure;
* return await Deployment.RunAsync(() =>
* {
* var example = Azure.DataFactory.GetTriggerSchedules.Invoke(new()
* {
* DataFactoryId = "/subscriptions/00000000-0000-0000-0000-000000000000/resourceGroups/rg1/providers/Microsoft.DataFactory/factories/datafactory1",
* });
* return new Dictionary
* {
* ["items"] = example.Apply(getTriggerSchedulesResult => getTriggerSchedulesResult.Items),
* };
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-azure/sdk/v5/go/azure/datafactory"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* example, err := datafactory.GetTriggerSchedules(ctx, &datafactory.GetTriggerSchedulesArgs{
* DataFactoryId: "/subscriptions/00000000-0000-0000-0000-000000000000/resourceGroups/rg1/providers/Microsoft.DataFactory/factories/datafactory1",
* }, nil)
* if err != nil {
* return err
* }
* ctx.Export("items", example.Items)
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azure.datafactory.DatafactoryFunctions;
* import com.pulumi.azure.datafactory.inputs.GetTriggerSchedulesArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* final var example = DatafactoryFunctions.getTriggerSchedules(GetTriggerSchedulesArgs.builder()
* .dataFactoryId("/subscriptions/00000000-0000-0000-0000-000000000000/resourceGroups/rg1/providers/Microsoft.DataFactory/factories/datafactory1")
* .build());
* ctx.export("items", example.applyValue(getTriggerSchedulesResult -> getTriggerSchedulesResult.items()));
* }
* }
* ```
* ```yaml
* variables:
* example:
* fn::invoke:
* Function: azure:datafactory:getTriggerSchedules
* Arguments:
* dataFactoryId: /subscriptions/00000000-0000-0000-0000-000000000000/resourceGroups/rg1/providers/Microsoft.DataFactory/factories/datafactory1
* outputs:
* items: ${example.items}
* ```
*
* @param argument A collection of arguments for invoking getTriggerSchedules.
* @return A collection of values returned by getTriggerSchedules.
*/
public suspend fun getTriggerSchedules(argument: GetTriggerSchedulesPlainArgs):
GetTriggerSchedulesResult =
getTriggerSchedulesResultToKotlin(getTriggerSchedulesPlain(argument.toJava()).await())
/**
* @see [getTriggerSchedules].
* @param dataFactoryId The ID of the Azure Data Factory to fetch trigger schedules from.
* @return A collection of values returned by getTriggerSchedules.
*/
public suspend fun getTriggerSchedules(dataFactoryId: String): GetTriggerSchedulesResult {
val argument = GetTriggerSchedulesPlainArgs(
dataFactoryId = dataFactoryId,
)
return getTriggerSchedulesResultToKotlin(getTriggerSchedulesPlain(argument.toJava()).await())
}
/**
* @see [getTriggerSchedules].
* @param argument Builder for [com.pulumi.azure.datafactory.kotlin.inputs.GetTriggerSchedulesPlainArgs].
* @return A collection of values returned by getTriggerSchedules.
*/
public suspend
fun getTriggerSchedules(argument: suspend GetTriggerSchedulesPlainArgsBuilder.() -> Unit):
GetTriggerSchedulesResult {
val builder = GetTriggerSchedulesPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getTriggerSchedulesResultToKotlin(getTriggerSchedulesPlain(builtArgument.toJava()).await())
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy