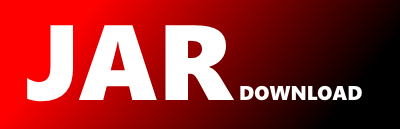
com.pulumi.azure.datafactory.kotlin.IntegrationRuntimeSsisArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-kotlin Show documentation
Show all versions of pulumi-azure-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azure.datafactory.kotlin
import com.pulumi.azure.datafactory.IntegrationRuntimeSsisArgs.builder
import com.pulumi.azure.datafactory.kotlin.inputs.IntegrationRuntimeSsisCatalogInfoArgs
import com.pulumi.azure.datafactory.kotlin.inputs.IntegrationRuntimeSsisCatalogInfoArgsBuilder
import com.pulumi.azure.datafactory.kotlin.inputs.IntegrationRuntimeSsisCopyComputeScaleArgs
import com.pulumi.azure.datafactory.kotlin.inputs.IntegrationRuntimeSsisCopyComputeScaleArgsBuilder
import com.pulumi.azure.datafactory.kotlin.inputs.IntegrationRuntimeSsisCustomSetupScriptArgs
import com.pulumi.azure.datafactory.kotlin.inputs.IntegrationRuntimeSsisCustomSetupScriptArgsBuilder
import com.pulumi.azure.datafactory.kotlin.inputs.IntegrationRuntimeSsisExpressCustomSetupArgs
import com.pulumi.azure.datafactory.kotlin.inputs.IntegrationRuntimeSsisExpressCustomSetupArgsBuilder
import com.pulumi.azure.datafactory.kotlin.inputs.IntegrationRuntimeSsisExpressVnetIntegrationArgs
import com.pulumi.azure.datafactory.kotlin.inputs.IntegrationRuntimeSsisExpressVnetIntegrationArgsBuilder
import com.pulumi.azure.datafactory.kotlin.inputs.IntegrationRuntimeSsisPackageStoreArgs
import com.pulumi.azure.datafactory.kotlin.inputs.IntegrationRuntimeSsisPackageStoreArgsBuilder
import com.pulumi.azure.datafactory.kotlin.inputs.IntegrationRuntimeSsisPipelineExternalComputeScaleArgs
import com.pulumi.azure.datafactory.kotlin.inputs.IntegrationRuntimeSsisPipelineExternalComputeScaleArgsBuilder
import com.pulumi.azure.datafactory.kotlin.inputs.IntegrationRuntimeSsisProxyArgs
import com.pulumi.azure.datafactory.kotlin.inputs.IntegrationRuntimeSsisProxyArgsBuilder
import com.pulumi.azure.datafactory.kotlin.inputs.IntegrationRuntimeSsisVnetIntegrationArgs
import com.pulumi.azure.datafactory.kotlin.inputs.IntegrationRuntimeSsisVnetIntegrationArgsBuilder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
* Manages a Data Factory Azure-SSIS Integration Runtime.
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as azure from "@pulumi/azure";
* const example = new azure.core.ResourceGroup("example", {
* name: "example-resources",
* location: "West Europe",
* });
* const exampleFactory = new azure.datafactory.Factory("example", {
* name: "example",
* location: example.location,
* resourceGroupName: example.name,
* });
* const exampleIntegrationRuntimeSsis = new azure.datafactory.IntegrationRuntimeSsis("example", {
* name: "example",
* dataFactoryId: exampleFactory.id,
* location: example.location,
* nodeSize: "Standard_D8_v3",
* });
* ```
* ```python
* import pulumi
* import pulumi_azure as azure
* example = azure.core.ResourceGroup("example",
* name="example-resources",
* location="West Europe")
* example_factory = azure.datafactory.Factory("example",
* name="example",
* location=example.location,
* resource_group_name=example.name)
* example_integration_runtime_ssis = azure.datafactory.IntegrationRuntimeSsis("example",
* name="example",
* data_factory_id=example_factory.id,
* location=example.location,
* node_size="Standard_D8_v3")
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Azure = Pulumi.Azure;
* return await Deployment.RunAsync(() =>
* {
* var example = new Azure.Core.ResourceGroup("example", new()
* {
* Name = "example-resources",
* Location = "West Europe",
* });
* var exampleFactory = new Azure.DataFactory.Factory("example", new()
* {
* Name = "example",
* Location = example.Location,
* ResourceGroupName = example.Name,
* });
* var exampleIntegrationRuntimeSsis = new Azure.DataFactory.IntegrationRuntimeSsis("example", new()
* {
* Name = "example",
* DataFactoryId = exampleFactory.Id,
* Location = example.Location,
* NodeSize = "Standard_D8_v3",
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-azure/sdk/v5/go/azure/core"
* "github.com/pulumi/pulumi-azure/sdk/v5/go/azure/datafactory"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* example, err := core.NewResourceGroup(ctx, "example", &core.ResourceGroupArgs{
* Name: pulumi.String("example-resources"),
* Location: pulumi.String("West Europe"),
* })
* if err != nil {
* return err
* }
* exampleFactory, err := datafactory.NewFactory(ctx, "example", &datafactory.FactoryArgs{
* Name: pulumi.String("example"),
* Location: example.Location,
* ResourceGroupName: example.Name,
* })
* if err != nil {
* return err
* }
* _, err = datafactory.NewIntegrationRuntimeSsis(ctx, "example", &datafactory.IntegrationRuntimeSsisArgs{
* Name: pulumi.String("example"),
* DataFactoryId: exampleFactory.ID(),
* Location: example.Location,
* NodeSize: pulumi.String("Standard_D8_v3"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azure.core.ResourceGroup;
* import com.pulumi.azure.core.ResourceGroupArgs;
* import com.pulumi.azure.datafactory.Factory;
* import com.pulumi.azure.datafactory.FactoryArgs;
* import com.pulumi.azure.datafactory.IntegrationRuntimeSsis;
* import com.pulumi.azure.datafactory.IntegrationRuntimeSsisArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var example = new ResourceGroup("example", ResourceGroupArgs.builder()
* .name("example-resources")
* .location("West Europe")
* .build());
* var exampleFactory = new Factory("exampleFactory", FactoryArgs.builder()
* .name("example")
* .location(example.location())
* .resourceGroupName(example.name())
* .build());
* var exampleIntegrationRuntimeSsis = new IntegrationRuntimeSsis("exampleIntegrationRuntimeSsis", IntegrationRuntimeSsisArgs.builder()
* .name("example")
* .dataFactoryId(exampleFactory.id())
* .location(example.location())
* .nodeSize("Standard_D8_v3")
* .build());
* }
* }
* ```
* ```yaml
* resources:
* example:
* type: azure:core:ResourceGroup
* properties:
* name: example-resources
* location: West Europe
* exampleFactory:
* type: azure:datafactory:Factory
* name: example
* properties:
* name: example
* location: ${example.location}
* resourceGroupName: ${example.name}
* exampleIntegrationRuntimeSsis:
* type: azure:datafactory:IntegrationRuntimeSsis
* name: example
* properties:
* name: example
* dataFactoryId: ${exampleFactory.id}
* location: ${example.location}
* nodeSize: Standard_D8_v3
* ```
*
* ## Import
* Data Factory Azure-SSIS Integration Runtimes can be imported using the `resource id`, e.g.
* ```sh
* $ pulumi import azure:datafactory/integrationRuntimeSsis:IntegrationRuntimeSsis example /subscriptions/00000000-0000-0000-0000-000000000000/resourceGroups/example/providers/Microsoft.DataFactory/factories/example/integrationruntimes/example
* ```
* @property catalogInfo A `catalog_info` block as defined below.
* @property copyComputeScale One `copy_compute_scale` block as defined below.
* @property credentialName The name of a Data Factory Credential that the SSIS integration will use to access data sources. For example, `azure.datafactory.CredentialUserManagedIdentity`
* > **NOTE** If `credential_name` is omitted, the integration runtime will use the Data Factory assigned identity.
* @property customSetupScript A `custom_setup_script` block as defined below.
* @property dataFactoryId The Data Factory ID in which to associate the Linked Service with. Changing this forces a new resource.
* @property description Integration runtime description.
* @property edition The Azure-SSIS Integration Runtime edition. Valid values are `Standard` and `Enterprise`. Defaults to `Standard`.
* @property expressCustomSetup An `express_custom_setup` block as defined below.
* @property expressVnetIntegration A `express_vnet_integration` block as defined below.
* @property licenseType The type of the license that is used. Valid values are `LicenseIncluded` and `BasePrice`. Defaults to `LicenseIncluded`.
* @property location Specifies the supported Azure location where the resource exists. Changing this forces a new resource to be created.
* @property maxParallelExecutionsPerNode Defines the maximum parallel executions per node. Defaults to `1`. Max is `1`.
* @property name Specifies the name of the Azure-SSIS Integration Runtime. Changing this forces a new resource to be created. Must be globally unique. See the [Microsoft documentation](https://docs.microsoft.com/azure/data-factory/naming-rules) for all restrictions.
* @property nodeSize The size of the nodes on which the Azure-SSIS Integration Runtime runs. Valid values are: `Standard_D2_v3`, `Standard_D4_v3`, `Standard_D8_v3`, `Standard_D16_v3`, `Standard_D32_v3`, `Standard_D64_v3`, `Standard_E2_v3`, `Standard_E4_v3`, `Standard_E8_v3`, `Standard_E16_v3`, `Standard_E32_v3`, `Standard_E64_v3`, `Standard_D1_v2`, `Standard_D2_v2`, `Standard_D3_v2`, `Standard_D4_v2`, `Standard_A4_v2` and `Standard_A8_v2`
* @property numberOfNodes Number of nodes for the Azure-SSIS Integration Runtime. Max is `10`. Defaults to `1`.
* @property packageStores One or more `package_store` block as defined below.
* @property pipelineExternalComputeScale One `pipeline_external_compute_scale` block as defined below.
* @property proxy A `proxy` block as defined below.
* @property vnetIntegration A `vnet_integration` block as defined below.
*/
public data class IntegrationRuntimeSsisArgs(
public val catalogInfo: Output? = null,
public val copyComputeScale: Output? = null,
public val credentialName: Output? = null,
public val customSetupScript: Output? = null,
public val dataFactoryId: Output? = null,
public val description: Output? = null,
public val edition: Output? = null,
public val expressCustomSetup: Output? = null,
public val expressVnetIntegration: Output? =
null,
public val licenseType: Output? = null,
public val location: Output? = null,
public val maxParallelExecutionsPerNode: Output? = null,
public val name: Output? = null,
public val nodeSize: Output? = null,
public val numberOfNodes: Output? = null,
public val packageStores: Output>? = null,
public val pipelineExternalComputeScale:
Output? = null,
public val proxy: Output? = null,
public val vnetIntegration: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azure.datafactory.IntegrationRuntimeSsisArgs =
com.pulumi.azure.datafactory.IntegrationRuntimeSsisArgs.builder()
.catalogInfo(catalogInfo?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.copyComputeScale(copyComputeScale?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.credentialName(credentialName?.applyValue({ args0 -> args0 }))
.customSetupScript(customSetupScript?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.dataFactoryId(dataFactoryId?.applyValue({ args0 -> args0 }))
.description(description?.applyValue({ args0 -> args0 }))
.edition(edition?.applyValue({ args0 -> args0 }))
.expressCustomSetup(
expressCustomSetup?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.expressVnetIntegration(
expressVnetIntegration?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.licenseType(licenseType?.applyValue({ args0 -> args0 }))
.location(location?.applyValue({ args0 -> args0 }))
.maxParallelExecutionsPerNode(maxParallelExecutionsPerNode?.applyValue({ args0 -> args0 }))
.name(name?.applyValue({ args0 -> args0 }))
.nodeSize(nodeSize?.applyValue({ args0 -> args0 }))
.numberOfNodes(numberOfNodes?.applyValue({ args0 -> args0 }))
.packageStores(
packageStores?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
)
.pipelineExternalComputeScale(
pipelineExternalComputeScale?.applyValue({ args0 ->
args0.let({ args0 -> args0.toJava() })
}),
)
.proxy(proxy?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.vnetIntegration(
vnetIntegration?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
).build()
}
/**
* Builder for [IntegrationRuntimeSsisArgs].
*/
@PulumiTagMarker
public class IntegrationRuntimeSsisArgsBuilder internal constructor() {
private var catalogInfo: Output? = null
private var copyComputeScale: Output? = null
private var credentialName: Output? = null
private var customSetupScript: Output? = null
private var dataFactoryId: Output? = null
private var description: Output? = null
private var edition: Output? = null
private var expressCustomSetup: Output? = null
private var expressVnetIntegration: Output? =
null
private var licenseType: Output? = null
private var location: Output? = null
private var maxParallelExecutionsPerNode: Output? = null
private var name: Output? = null
private var nodeSize: Output? = null
private var numberOfNodes: Output? = null
private var packageStores: Output>? = null
private var pipelineExternalComputeScale:
Output? = null
private var proxy: Output? = null
private var vnetIntegration: Output? = null
/**
* @param value A `catalog_info` block as defined below.
*/
@JvmName("pynqcududopfhlmx")
public suspend fun catalogInfo(`value`: Output) {
this.catalogInfo = value
}
/**
* @param value One `copy_compute_scale` block as defined below.
*/
@JvmName("yqslwalewkfbycfr")
public suspend fun copyComputeScale(`value`: Output) {
this.copyComputeScale = value
}
/**
* @param value The name of a Data Factory Credential that the SSIS integration will use to access data sources. For example, `azure.datafactory.CredentialUserManagedIdentity`
* > **NOTE** If `credential_name` is omitted, the integration runtime will use the Data Factory assigned identity.
*/
@JvmName("xypjkkfsbhpvtpcb")
public suspend fun credentialName(`value`: Output) {
this.credentialName = value
}
/**
* @param value A `custom_setup_script` block as defined below.
*/
@JvmName("ycgrctanehjdsmcg")
public suspend
fun customSetupScript(`value`: Output) {
this.customSetupScript = value
}
/**
* @param value The Data Factory ID in which to associate the Linked Service with. Changing this forces a new resource.
*/
@JvmName("ncyudkotqhpchpnh")
public suspend fun dataFactoryId(`value`: Output) {
this.dataFactoryId = value
}
/**
* @param value Integration runtime description.
*/
@JvmName("jjogrwkiijegetuk")
public suspend fun description(`value`: Output) {
this.description = value
}
/**
* @param value The Azure-SSIS Integration Runtime edition. Valid values are `Standard` and `Enterprise`. Defaults to `Standard`.
*/
@JvmName("wqssmcpeymkpbcqt")
public suspend fun edition(`value`: Output) {
this.edition = value
}
/**
* @param value An `express_custom_setup` block as defined below.
*/
@JvmName("kccvyjtteqnnmxhh")
public suspend
fun expressCustomSetup(`value`: Output) {
this.expressCustomSetup = value
}
/**
* @param value A `express_vnet_integration` block as defined below.
*/
@JvmName("ughmwbxkbsadmyqo")
public suspend
fun expressVnetIntegration(`value`: Output) {
this.expressVnetIntegration = value
}
/**
* @param value The type of the license that is used. Valid values are `LicenseIncluded` and `BasePrice`. Defaults to `LicenseIncluded`.
*/
@JvmName("muupdsnfqvpxoxcw")
public suspend fun licenseType(`value`: Output) {
this.licenseType = value
}
/**
* @param value Specifies the supported Azure location where the resource exists. Changing this forces a new resource to be created.
*/
@JvmName("qcwpnncjhabphnlj")
public suspend fun location(`value`: Output) {
this.location = value
}
/**
* @param value Defines the maximum parallel executions per node. Defaults to `1`. Max is `1`.
*/
@JvmName("cokmjviaxxmkjrkc")
public suspend fun maxParallelExecutionsPerNode(`value`: Output) {
this.maxParallelExecutionsPerNode = value
}
/**
* @param value Specifies the name of the Azure-SSIS Integration Runtime. Changing this forces a new resource to be created. Must be globally unique. See the [Microsoft documentation](https://docs.microsoft.com/azure/data-factory/naming-rules) for all restrictions.
*/
@JvmName("tarfmlsdbxhihgwq")
public suspend fun name(`value`: Output) {
this.name = value
}
/**
* @param value The size of the nodes on which the Azure-SSIS Integration Runtime runs. Valid values are: `Standard_D2_v3`, `Standard_D4_v3`, `Standard_D8_v3`, `Standard_D16_v3`, `Standard_D32_v3`, `Standard_D64_v3`, `Standard_E2_v3`, `Standard_E4_v3`, `Standard_E8_v3`, `Standard_E16_v3`, `Standard_E32_v3`, `Standard_E64_v3`, `Standard_D1_v2`, `Standard_D2_v2`, `Standard_D3_v2`, `Standard_D4_v2`, `Standard_A4_v2` and `Standard_A8_v2`
*/
@JvmName("ptrvrpwcqlteffli")
public suspend fun nodeSize(`value`: Output) {
this.nodeSize = value
}
/**
* @param value Number of nodes for the Azure-SSIS Integration Runtime. Max is `10`. Defaults to `1`.
*/
@JvmName("agggbvxcnnfenlap")
public suspend fun numberOfNodes(`value`: Output) {
this.numberOfNodes = value
}
/**
* @param value One or more `package_store` block as defined below.
*/
@JvmName("sgxwqoyvhrpnppgr")
public suspend fun packageStores(`value`: Output>) {
this.packageStores = value
}
@JvmName("buiqjseasdcfrajn")
public suspend fun packageStores(vararg values: Output) {
this.packageStores = Output.all(values.asList())
}
/**
* @param values One or more `package_store` block as defined below.
*/
@JvmName("exauevgmxmbwibxg")
public suspend fun packageStores(values: List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy