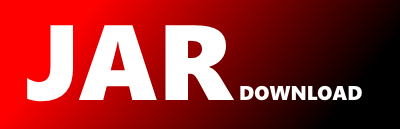
com.pulumi.azure.datafactory.kotlin.inputs.LinkedServiceAzureDatabricksNewClusterConfigArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-kotlin Show documentation
Show all versions of pulumi-azure-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azure.datafactory.kotlin.inputs
import com.pulumi.azure.datafactory.inputs.LinkedServiceAzureDatabricksNewClusterConfigArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Int
import kotlin.Pair
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
import kotlin.collections.Map
import kotlin.jvm.JvmName
/**
*
* @property clusterVersion Spark version of a the cluster.
* @property customTags Tags for the cluster resource.
* @property driverNodeType Driver node type for the cluster.
* @property initScripts User defined initialization scripts for the cluster.
* @property logDestination Location to deliver Spark driver, worker, and event logs.
* @property maxNumberOfWorkers Specifies the maximum number of worker nodes. It should be between 1 and 25000.
* @property minNumberOfWorkers Specifies the minimum number of worker nodes. It should be between 1 and 25000. It defaults to `1`.
* @property nodeType Node type for the new cluster.
* @property sparkConfig User-specified Spark configuration variables key-value pairs.
* @property sparkEnvironmentVariables User-specified Spark environment variables key-value pairs.
*/
public data class LinkedServiceAzureDatabricksNewClusterConfigArgs(
public val clusterVersion: Output,
public val customTags: Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy