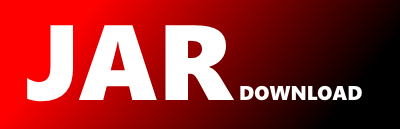
com.pulumi.azure.dataprotection.kotlin.BackupInstancePostgresqlArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-kotlin Show documentation
Show all versions of pulumi-azure-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azure.dataprotection.kotlin
import com.pulumi.azure.dataprotection.BackupInstancePostgresqlArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
* Manages a Backup Instance to back up PostgreSQL.
* > **Note:** Before using this resource, there are some prerequisite permissions for configure backup and restore. See more details from .
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as azure from "@pulumi/azure";
* const current = azure.core.getClientConfig({});
* const example = new azure.core.ResourceGroup("example", {
* name: "example",
* location: "West Europe",
* });
* const exampleServer = new azure.postgresql.Server("example", {
* name: "example",
* location: example.location,
* resourceGroupName: example.name,
* skuName: "B_Gen5_2",
* storageMb: 5120,
* backupRetentionDays: 7,
* geoRedundantBackupEnabled: false,
* autoGrowEnabled: true,
* administratorLogin: "psqladmin",
* administratorLoginPassword: "H@Sh1CoR3!",
* version: "9.5",
* sslEnforcementEnabled: true,
* });
* const exampleFirewallRule = new azure.postgresql.FirewallRule("example", {
* name: "AllowAllWindowsAzureIps",
* resourceGroupName: example.name,
* serverName: exampleServer.name,
* startIpAddress: "0.0.0.0",
* endIpAddress: "0.0.0.0",
* });
* const exampleDatabase = new azure.postgresql.Database("example", {
* name: "example",
* resourceGroupName: example.name,
* serverName: exampleServer.name,
* charset: "UTF8",
* collation: "English_United States.1252",
* });
* const exampleBackupVault = new azure.dataprotection.BackupVault("example", {
* name: "example",
* resourceGroupName: example.name,
* location: example.location,
* datastoreType: "VaultStore",
* redundancy: "LocallyRedundant",
* identity: {
* type: "SystemAssigned",
* },
* });
* const exampleKeyVault = new azure.keyvault.KeyVault("example", {
* name: "example",
* location: example.location,
* resourceGroupName: example.name,
* tenantId: current.then(current => current.tenantId),
* skuName: "premium",
* softDeleteRetentionDays: 7,
* accessPolicies: [
* {
* tenantId: current.then(current => current.tenantId),
* objectId: current.then(current => current.objectId),
* keyPermissions: [
* "Create",
* "Get",
* ],
* secretPermissions: [
* "Set",
* "Get",
* "Delete",
* "Purge",
* "Recover",
* ],
* },
* {
* tenantId: exampleBackupVault.identity.apply(identity => identity?.tenantId),
* objectId: exampleBackupVault.identity.apply(identity => identity?.principalId),
* keyPermissions: [
* "Create",
* "Get",
* ],
* secretPermissions: [
* "Set",
* "Get",
* "Delete",
* "Purge",
* "Recover",
* ],
* },
* ],
* });
* const exampleSecret = new azure.keyvault.Secret("example", {
* name: "example",
* value: pulumi.interpolate`Server=${exampleServer.name}.postgres.database.azure.com;Database=${exampleDatabase.name};Port=5432;User Id=psqladmin@${exampleServer.name};Password=H@Sh1CoR3!;Ssl Mode=Require;`,
* keyVaultId: exampleKeyVault.id,
* });
* const exampleBackupPolicyPostgresql = new azure.dataprotection.BackupPolicyPostgresql("example", {
* name: "example",
* resourceGroupName: example.name,
* vaultName: exampleBackupVault.name,
* backupRepeatingTimeIntervals: ["R/2021-05-23T02:30:00+00:00/P1W"],
* defaultRetentionDuration: "P4M",
* });
* const exampleAssignment = new azure.authorization.Assignment("example", {
* scope: exampleServer.id,
* roleDefinitionName: "Reader",
* principalId: exampleBackupVault.identity.apply(identity => identity?.principalId),
* });
* const exampleBackupInstancePostgresql = new azure.dataprotection.BackupInstancePostgresql("example", {
* name: "example",
* location: example.location,
* vaultId: exampleBackupVault.id,
* databaseId: exampleDatabase.id,
* backupPolicyId: exampleBackupPolicyPostgresql.id,
* databaseCredentialKeyVaultSecretId: exampleSecret.versionlessId,
* });
* ```
* ```python
* import pulumi
* import pulumi_azure as azure
* current = azure.core.get_client_config()
* example = azure.core.ResourceGroup("example",
* name="example",
* location="West Europe")
* example_server = azure.postgresql.Server("example",
* name="example",
* location=example.location,
* resource_group_name=example.name,
* sku_name="B_Gen5_2",
* storage_mb=5120,
* backup_retention_days=7,
* geo_redundant_backup_enabled=False,
* auto_grow_enabled=True,
* administrator_login="psqladmin",
* administrator_login_password="H@Sh1CoR3!",
* version="9.5",
* ssl_enforcement_enabled=True)
* example_firewall_rule = azure.postgresql.FirewallRule("example",
* name="AllowAllWindowsAzureIps",
* resource_group_name=example.name,
* server_name=example_server.name,
* start_ip_address="0.0.0.0",
* end_ip_address="0.0.0.0")
* example_database = azure.postgresql.Database("example",
* name="example",
* resource_group_name=example.name,
* server_name=example_server.name,
* charset="UTF8",
* collation="English_United States.1252")
* example_backup_vault = azure.dataprotection.BackupVault("example",
* name="example",
* resource_group_name=example.name,
* location=example.location,
* datastore_type="VaultStore",
* redundancy="LocallyRedundant",
* identity=azure.dataprotection.BackupVaultIdentityArgs(
* type="SystemAssigned",
* ))
* example_key_vault = azure.keyvault.KeyVault("example",
* name="example",
* location=example.location,
* resource_group_name=example.name,
* tenant_id=current.tenant_id,
* sku_name="premium",
* soft_delete_retention_days=7,
* access_policies=[
* azure.keyvault.KeyVaultAccessPolicyArgs(
* tenant_id=current.tenant_id,
* object_id=current.object_id,
* key_permissions=[
* "Create",
* "Get",
* ],
* secret_permissions=[
* "Set",
* "Get",
* "Delete",
* "Purge",
* "Recover",
* ],
* ),
* azure.keyvault.KeyVaultAccessPolicyArgs(
* tenant_id=example_backup_vault.identity.tenant_id,
* object_id=example_backup_vault.identity.principal_id,
* key_permissions=[
* "Create",
* "Get",
* ],
* secret_permissions=[
* "Set",
* "Get",
* "Delete",
* "Purge",
* "Recover",
* ],
* ),
* ])
* example_secret = azure.keyvault.Secret("example",
* name="example",
* value=pulumi.Output.all(example_server.name, example_database.name, example_server.name).apply(lambda exampleServerName, exampleDatabaseName, exampleServerName1: f"Server={example_server_name}.postgres.database.azure.com;Database={example_database_name};Port=5432;User Id=psqladmin@{example_server_name1};Password=H@Sh1CoR3!;Ssl Mode=Require;"),
* key_vault_id=example_key_vault.id)
* example_backup_policy_postgresql = azure.dataprotection.BackupPolicyPostgresql("example",
* name="example",
* resource_group_name=example.name,
* vault_name=example_backup_vault.name,
* backup_repeating_time_intervals=["R/2021-05-23T02:30:00+00:00/P1W"],
* default_retention_duration="P4M")
* example_assignment = azure.authorization.Assignment("example",
* scope=example_server.id,
* role_definition_name="Reader",
* principal_id=example_backup_vault.identity.principal_id)
* example_backup_instance_postgresql = azure.dataprotection.BackupInstancePostgresql("example",
* name="example",
* location=example.location,
* vault_id=example_backup_vault.id,
* database_id=example_database.id,
* backup_policy_id=example_backup_policy_postgresql.id,
* database_credential_key_vault_secret_id=example_secret.versionless_id)
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Azure = Pulumi.Azure;
* return await Deployment.RunAsync(() =>
* {
* var current = Azure.Core.GetClientConfig.Invoke();
* var example = new Azure.Core.ResourceGroup("example", new()
* {
* Name = "example",
* Location = "West Europe",
* });
* var exampleServer = new Azure.PostgreSql.Server("example", new()
* {
* Name = "example",
* Location = example.Location,
* ResourceGroupName = example.Name,
* SkuName = "B_Gen5_2",
* StorageMb = 5120,
* BackupRetentionDays = 7,
* GeoRedundantBackupEnabled = false,
* AutoGrowEnabled = true,
* AdministratorLogin = "psqladmin",
* AdministratorLoginPassword = "H@Sh1CoR3!",
* Version = "9.5",
* SslEnforcementEnabled = true,
* });
* var exampleFirewallRule = new Azure.PostgreSql.FirewallRule("example", new()
* {
* Name = "AllowAllWindowsAzureIps",
* ResourceGroupName = example.Name,
* ServerName = exampleServer.Name,
* StartIpAddress = "0.0.0.0",
* EndIpAddress = "0.0.0.0",
* });
* var exampleDatabase = new Azure.PostgreSql.Database("example", new()
* {
* Name = "example",
* ResourceGroupName = example.Name,
* ServerName = exampleServer.Name,
* Charset = "UTF8",
* Collation = "English_United States.1252",
* });
* var exampleBackupVault = new Azure.DataProtection.BackupVault("example", new()
* {
* Name = "example",
* ResourceGroupName = example.Name,
* Location = example.Location,
* DatastoreType = "VaultStore",
* Redundancy = "LocallyRedundant",
* Identity = new Azure.DataProtection.Inputs.BackupVaultIdentityArgs
* {
* Type = "SystemAssigned",
* },
* });
* var exampleKeyVault = new Azure.KeyVault.KeyVault("example", new()
* {
* Name = "example",
* Location = example.Location,
* ResourceGroupName = example.Name,
* TenantId = current.Apply(getClientConfigResult => getClientConfigResult.TenantId),
* SkuName = "premium",
* SoftDeleteRetentionDays = 7,
* AccessPolicies = new[]
* {
* new Azure.KeyVault.Inputs.KeyVaultAccessPolicyArgs
* {
* TenantId = current.Apply(getClientConfigResult => getClientConfigResult.TenantId),
* ObjectId = current.Apply(getClientConfigResult => getClientConfigResult.ObjectId),
* KeyPermissions = new[]
* {
* "Create",
* "Get",
* },
* SecretPermissions = new[]
* {
* "Set",
* "Get",
* "Delete",
* "Purge",
* "Recover",
* },
* },
* new Azure.KeyVault.Inputs.KeyVaultAccessPolicyArgs
* {
* TenantId = exampleBackupVault.Identity.Apply(identity => identity?.TenantId),
* ObjectId = exampleBackupVault.Identity.Apply(identity => identity?.PrincipalId),
* KeyPermissions = new[]
* {
* "Create",
* "Get",
* },
* SecretPermissions = new[]
* {
* "Set",
* "Get",
* "Delete",
* "Purge",
* "Recover",
* },
* },
* },
* });
* var exampleSecret = new Azure.KeyVault.Secret("example", new()
* {
* Name = "example",
* Value = Output.Tuple(exampleServer.Name, exampleDatabase.Name, exampleServer.Name).Apply(values =>
* {
* var exampleServerName = values.Item1;
* var exampleDatabaseName = values.Item2;
* var exampleServerName1 = values.Item3;
* return $"Server={exampleServerName}.postgres.database.azure.com;Database={exampleDatabaseName};Port=5432;User Id=psqladmin@{exampleServerName1};Password=H@Sh1CoR3!;Ssl Mode=Require;";
* }),
* KeyVaultId = exampleKeyVault.Id,
* });
* var exampleBackupPolicyPostgresql = new Azure.DataProtection.BackupPolicyPostgresql("example", new()
* {
* Name = "example",
* ResourceGroupName = example.Name,
* VaultName = exampleBackupVault.Name,
* BackupRepeatingTimeIntervals = new[]
* {
* "R/2021-05-23T02:30:00+00:00/P1W",
* },
* DefaultRetentionDuration = "P4M",
* });
* var exampleAssignment = new Azure.Authorization.Assignment("example", new()
* {
* Scope = exampleServer.Id,
* RoleDefinitionName = "Reader",
* PrincipalId = exampleBackupVault.Identity.Apply(identity => identity?.PrincipalId),
* });
* var exampleBackupInstancePostgresql = new Azure.DataProtection.BackupInstancePostgresql("example", new()
* {
* Name = "example",
* Location = example.Location,
* VaultId = exampleBackupVault.Id,
* DatabaseId = exampleDatabase.Id,
* BackupPolicyId = exampleBackupPolicyPostgresql.Id,
* DatabaseCredentialKeyVaultSecretId = exampleSecret.VersionlessId,
* });
* });
* ```
* ```go
* package main
* import (
* "fmt"
* "github.com/pulumi/pulumi-azure/sdk/v5/go/azure/authorization"
* "github.com/pulumi/pulumi-azure/sdk/v5/go/azure/core"
* "github.com/pulumi/pulumi-azure/sdk/v5/go/azure/dataprotection"
* "github.com/pulumi/pulumi-azure/sdk/v5/go/azure/keyvault"
* "github.com/pulumi/pulumi-azure/sdk/v5/go/azure/postgresql"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* current, err := core.GetClientConfig(ctx, nil, nil)
* if err != nil {
* return err
* }
* example, err := core.NewResourceGroup(ctx, "example", &core.ResourceGroupArgs{
* Name: pulumi.String("example"),
* Location: pulumi.String("West Europe"),
* })
* if err != nil {
* return err
* }
* exampleServer, err := postgresql.NewServer(ctx, "example", &postgresql.ServerArgs{
* Name: pulumi.String("example"),
* Location: example.Location,
* ResourceGroupName: example.Name,
* SkuName: pulumi.String("B_Gen5_2"),
* StorageMb: pulumi.Int(5120),
* BackupRetentionDays: pulumi.Int(7),
* GeoRedundantBackupEnabled: pulumi.Bool(false),
* AutoGrowEnabled: pulumi.Bool(true),
* AdministratorLogin: pulumi.String("psqladmin"),
* AdministratorLoginPassword: pulumi.String("H@Sh1CoR3!"),
* Version: pulumi.String("9.5"),
* SslEnforcementEnabled: pulumi.Bool(true),
* })
* if err != nil {
* return err
* }
* _, err = postgresql.NewFirewallRule(ctx, "example", &postgresql.FirewallRuleArgs{
* Name: pulumi.String("AllowAllWindowsAzureIps"),
* ResourceGroupName: example.Name,
* ServerName: exampleServer.Name,
* StartIpAddress: pulumi.String("0.0.0.0"),
* EndIpAddress: pulumi.String("0.0.0.0"),
* })
* if err != nil {
* return err
* }
* exampleDatabase, err := postgresql.NewDatabase(ctx, "example", &postgresql.DatabaseArgs{
* Name: pulumi.String("example"),
* ResourceGroupName: example.Name,
* ServerName: exampleServer.Name,
* Charset: pulumi.String("UTF8"),
* Collation: pulumi.String("English_United States.1252"),
* })
* if err != nil {
* return err
* }
* exampleBackupVault, err := dataprotection.NewBackupVault(ctx, "example", &dataprotection.BackupVaultArgs{
* Name: pulumi.String("example"),
* ResourceGroupName: example.Name,
* Location: example.Location,
* DatastoreType: pulumi.String("VaultStore"),
* Redundancy: pulumi.String("LocallyRedundant"),
* Identity: &dataprotection.BackupVaultIdentityArgs{
* Type: pulumi.String("SystemAssigned"),
* },
* })
* if err != nil {
* return err
* }
* exampleKeyVault, err := keyvault.NewKeyVault(ctx, "example", &keyvault.KeyVaultArgs{
* Name: pulumi.String("example"),
* Location: example.Location,
* ResourceGroupName: example.Name,
* TenantId: pulumi.String(current.TenantId),
* SkuName: pulumi.String("premium"),
* SoftDeleteRetentionDays: pulumi.Int(7),
* AccessPolicies: keyvault.KeyVaultAccessPolicyArray{
* &keyvault.KeyVaultAccessPolicyArgs{
* TenantId: pulumi.String(current.TenantId),
* ObjectId: pulumi.String(current.ObjectId),
* KeyPermissions: pulumi.StringArray{
* pulumi.String("Create"),
* pulumi.String("Get"),
* },
* SecretPermissions: pulumi.StringArray{
* pulumi.String("Set"),
* pulumi.String("Get"),
* pulumi.String("Delete"),
* pulumi.String("Purge"),
* pulumi.String("Recover"),
* },
* },
* &keyvault.KeyVaultAccessPolicyArgs{
* TenantId: exampleBackupVault.Identity.ApplyT(func(identity dataprotection.BackupVaultIdentity) (*string, error) {
* return &identity.TenantId, nil
* }).(pulumi.StringPtrOutput),
* ObjectId: exampleBackupVault.Identity.ApplyT(func(identity dataprotection.BackupVaultIdentity) (*string, error) {
* return &identity.PrincipalId, nil
* }).(pulumi.StringPtrOutput),
* KeyPermissions: pulumi.StringArray{
* pulumi.String("Create"),
* pulumi.String("Get"),
* },
* SecretPermissions: pulumi.StringArray{
* pulumi.String("Set"),
* pulumi.String("Get"),
* pulumi.String("Delete"),
* pulumi.String("Purge"),
* pulumi.String("Recover"),
* },
* },
* },
* })
* if err != nil {
* return err
* }
* exampleSecret, err := keyvault.NewSecret(ctx, "example", &keyvault.SecretArgs{
* Name: pulumi.String("example"),
* Value: pulumi.All(exampleServer.Name, exampleDatabase.Name, exampleServer.Name).ApplyT(func(_args []interface{}) (string, error) {
* exampleServerName := _args[0].(string)
* exampleDatabaseName := _args[1].(string)
* exampleServerName1 := _args[2].(string)
* return fmt.Sprintf("Server=%v.postgres.database.azure.com;Database=%v;Port=5432;User Id=psqladmin@%v;Password=H@Sh1CoR3!;Ssl Mode=Require;", exampleServerName, exampleDatabaseName, exampleServerName1), nil
* }).(pulumi.StringOutput),
* KeyVaultId: exampleKeyVault.ID(),
* })
* if err != nil {
* return err
* }
* exampleBackupPolicyPostgresql, err := dataprotection.NewBackupPolicyPostgresql(ctx, "example", &dataprotection.BackupPolicyPostgresqlArgs{
* Name: pulumi.String("example"),
* ResourceGroupName: example.Name,
* VaultName: exampleBackupVault.Name,
* BackupRepeatingTimeIntervals: pulumi.StringArray{
* pulumi.String("R/2021-05-23T02:30:00+00:00/P1W"),
* },
* DefaultRetentionDuration: pulumi.String("P4M"),
* })
* if err != nil {
* return err
* }
* _, err = authorization.NewAssignment(ctx, "example", &authorization.AssignmentArgs{
* Scope: exampleServer.ID(),
* RoleDefinitionName: pulumi.String("Reader"),
* PrincipalId: exampleBackupVault.Identity.ApplyT(func(identity dataprotection.BackupVaultIdentity) (*string, error) {
* return &identity.PrincipalId, nil
* }).(pulumi.StringPtrOutput),
* })
* if err != nil {
* return err
* }
* _, err = dataprotection.NewBackupInstancePostgresql(ctx, "example", &dataprotection.BackupInstancePostgresqlArgs{
* Name: pulumi.String("example"),
* Location: example.Location,
* VaultId: exampleBackupVault.ID(),
* DatabaseId: exampleDatabase.ID(),
* BackupPolicyId: exampleBackupPolicyPostgresql.ID(),
* DatabaseCredentialKeyVaultSecretId: exampleSecret.VersionlessId,
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azure.core.CoreFunctions;
* import com.pulumi.azure.core.ResourceGroup;
* import com.pulumi.azure.core.ResourceGroupArgs;
* import com.pulumi.azure.postgresql.Server;
* import com.pulumi.azure.postgresql.ServerArgs;
* import com.pulumi.azure.postgresql.FirewallRule;
* import com.pulumi.azure.postgresql.FirewallRuleArgs;
* import com.pulumi.azure.postgresql.Database;
* import com.pulumi.azure.postgresql.DatabaseArgs;
* import com.pulumi.azure.dataprotection.BackupVault;
* import com.pulumi.azure.dataprotection.BackupVaultArgs;
* import com.pulumi.azure.dataprotection.inputs.BackupVaultIdentityArgs;
* import com.pulumi.azure.keyvault.KeyVault;
* import com.pulumi.azure.keyvault.KeyVaultArgs;
* import com.pulumi.azure.keyvault.inputs.KeyVaultAccessPolicyArgs;
* import com.pulumi.azure.keyvault.Secret;
* import com.pulumi.azure.keyvault.SecretArgs;
* import com.pulumi.azure.dataprotection.BackupPolicyPostgresql;
* import com.pulumi.azure.dataprotection.BackupPolicyPostgresqlArgs;
* import com.pulumi.azure.authorization.Assignment;
* import com.pulumi.azure.authorization.AssignmentArgs;
* import com.pulumi.azure.dataprotection.BackupInstancePostgresql;
* import com.pulumi.azure.dataprotection.BackupInstancePostgresqlArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* final var current = CoreFunctions.getClientConfig();
* var example = new ResourceGroup("example", ResourceGroupArgs.builder()
* .name("example")
* .location("West Europe")
* .build());
* var exampleServer = new Server("exampleServer", ServerArgs.builder()
* .name("example")
* .location(example.location())
* .resourceGroupName(example.name())
* .skuName("B_Gen5_2")
* .storageMb(5120)
* .backupRetentionDays(7)
* .geoRedundantBackupEnabled(false)
* .autoGrowEnabled(true)
* .administratorLogin("psqladmin")
* .administratorLoginPassword("H@Sh1CoR3!")
* .version("9.5")
* .sslEnforcementEnabled(true)
* .build());
* var exampleFirewallRule = new FirewallRule("exampleFirewallRule", FirewallRuleArgs.builder()
* .name("AllowAllWindowsAzureIps")
* .resourceGroupName(example.name())
* .serverName(exampleServer.name())
* .startIpAddress("0.0.0.0")
* .endIpAddress("0.0.0.0")
* .build());
* var exampleDatabase = new Database("exampleDatabase", DatabaseArgs.builder()
* .name("example")
* .resourceGroupName(example.name())
* .serverName(exampleServer.name())
* .charset("UTF8")
* .collation("English_United States.1252")
* .build());
* var exampleBackupVault = new BackupVault("exampleBackupVault", BackupVaultArgs.builder()
* .name("example")
* .resourceGroupName(example.name())
* .location(example.location())
* .datastoreType("VaultStore")
* .redundancy("LocallyRedundant")
* .identity(BackupVaultIdentityArgs.builder()
* .type("SystemAssigned")
* .build())
* .build());
* var exampleKeyVault = new KeyVault("exampleKeyVault", KeyVaultArgs.builder()
* .name("example")
* .location(example.location())
* .resourceGroupName(example.name())
* .tenantId(current.applyValue(getClientConfigResult -> getClientConfigResult.tenantId()))
* .skuName("premium")
* .softDeleteRetentionDays(7)
* .accessPolicies(
* KeyVaultAccessPolicyArgs.builder()
* .tenantId(current.applyValue(getClientConfigResult -> getClientConfigResult.tenantId()))
* .objectId(current.applyValue(getClientConfigResult -> getClientConfigResult.objectId()))
* .keyPermissions(
* "Create",
* "Get")
* .secretPermissions(
* "Set",
* "Get",
* "Delete",
* "Purge",
* "Recover")
* .build(),
* KeyVaultAccessPolicyArgs.builder()
* .tenantId(exampleBackupVault.identity().applyValue(identity -> identity.tenantId()))
* .objectId(exampleBackupVault.identity().applyValue(identity -> identity.principalId()))
* .keyPermissions(
* "Create",
* "Get")
* .secretPermissions(
* "Set",
* "Get",
* "Delete",
* "Purge",
* "Recover")
* .build())
* .build());
* var exampleSecret = new Secret("exampleSecret", SecretArgs.builder()
* .name("example")
* .value(Output.tuple(exampleServer.name(), exampleDatabase.name(), exampleServer.name()).applyValue(values -> {
* var exampleServerName = values.t1;
* var exampleDatabaseName = values.t2;
* var exampleServerName1 = values.t3;
* return String.format("Server=%s.postgres.database.azure.com;Database=%s;Port=5432;User Id=psqladmin@%s;Password=H@Sh1CoR3!;Ssl Mode=Require;", exampleServerName,exampleDatabaseName,exampleServerName1);
* }))
* .keyVaultId(exampleKeyVault.id())
* .build());
* var exampleBackupPolicyPostgresql = new BackupPolicyPostgresql("exampleBackupPolicyPostgresql", BackupPolicyPostgresqlArgs.builder()
* .name("example")
* .resourceGroupName(example.name())
* .vaultName(exampleBackupVault.name())
* .backupRepeatingTimeIntervals("R/2021-05-23T02:30:00+00:00/P1W")
* .defaultRetentionDuration("P4M")
* .build());
* var exampleAssignment = new Assignment("exampleAssignment", AssignmentArgs.builder()
* .scope(exampleServer.id())
* .roleDefinitionName("Reader")
* .principalId(exampleBackupVault.identity().applyValue(identity -> identity.principalId()))
* .build());
* var exampleBackupInstancePostgresql = new BackupInstancePostgresql("exampleBackupInstancePostgresql", BackupInstancePostgresqlArgs.builder()
* .name("example")
* .location(example.location())
* .vaultId(exampleBackupVault.id())
* .databaseId(exampleDatabase.id())
* .backupPolicyId(exampleBackupPolicyPostgresql.id())
* .databaseCredentialKeyVaultSecretId(exampleSecret.versionlessId())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* example:
* type: azure:core:ResourceGroup
* properties:
* name: example
* location: West Europe
* exampleServer:
* type: azure:postgresql:Server
* name: example
* properties:
* name: example
* location: ${example.location}
* resourceGroupName: ${example.name}
* skuName: B_Gen5_2
* storageMb: 5120
* backupRetentionDays: 7
* geoRedundantBackupEnabled: false
* autoGrowEnabled: true
* administratorLogin: psqladmin
* administratorLoginPassword: H@Sh1CoR3!
* version: '9.5'
* sslEnforcementEnabled: true
* exampleFirewallRule:
* type: azure:postgresql:FirewallRule
* name: example
* properties:
* name: AllowAllWindowsAzureIps
* resourceGroupName: ${example.name}
* serverName: ${exampleServer.name}
* startIpAddress: 0.0.0.0
* endIpAddress: 0.0.0.0
* exampleDatabase:
* type: azure:postgresql:Database
* name: example
* properties:
* name: example
* resourceGroupName: ${example.name}
* serverName: ${exampleServer.name}
* charset: UTF8
* collation: English_United States.1252
* exampleBackupVault:
* type: azure:dataprotection:BackupVault
* name: example
* properties:
* name: example
* resourceGroupName: ${example.name}
* location: ${example.location}
* datastoreType: VaultStore
* redundancy: LocallyRedundant
* identity:
* type: SystemAssigned
* exampleKeyVault:
* type: azure:keyvault:KeyVault
* name: example
* properties:
* name: example
* location: ${example.location}
* resourceGroupName: ${example.name}
* tenantId: ${current.tenantId}
* skuName: premium
* softDeleteRetentionDays: 7
* accessPolicies:
* - tenantId: ${current.tenantId}
* objectId: ${current.objectId}
* keyPermissions:
* - Create
* - Get
* secretPermissions:
* - Set
* - Get
* - Delete
* - Purge
* - Recover
* - tenantId: ${exampleBackupVault.identity.tenantId}
* objectId: ${exampleBackupVault.identity.principalId}
* keyPermissions:
* - Create
* - Get
* secretPermissions:
* - Set
* - Get
* - Delete
* - Purge
* - Recover
* exampleSecret:
* type: azure:keyvault:Secret
* name: example
* properties:
* name: example
* value: Server=${exampleServer.name}.postgres.database.azure.com;Database=${exampleDatabase.name};Port=5432;User Id=psqladmin@${exampleServer.name};Password=H@Sh1CoR3!;Ssl Mode=Require;
* keyVaultId: ${exampleKeyVault.id}
* exampleBackupPolicyPostgresql:
* type: azure:dataprotection:BackupPolicyPostgresql
* name: example
* properties:
* name: example
* resourceGroupName: ${example.name}
* vaultName: ${exampleBackupVault.name}
* backupRepeatingTimeIntervals:
* - R/2021-05-23T02:30:00+00:00/P1W
* defaultRetentionDuration: P4M
* exampleAssignment:
* type: azure:authorization:Assignment
* name: example
* properties:
* scope: ${exampleServer.id}
* roleDefinitionName: Reader
* principalId: ${exampleBackupVault.identity.principalId}
* exampleBackupInstancePostgresql:
* type: azure:dataprotection:BackupInstancePostgresql
* name: example
* properties:
* name: example
* location: ${example.location}
* vaultId: ${exampleBackupVault.id}
* databaseId: ${exampleDatabase.id}
* backupPolicyId: ${exampleBackupPolicyPostgresql.id}
* databaseCredentialKeyVaultSecretId: ${exampleSecret.versionlessId}
* variables:
* current:
* fn::invoke:
* Function: azure:core:getClientConfig
* Arguments: {}
* ```
*
* ## Import
* Backup Instance PostgreSQL can be imported using the `resource id`, e.g.
* ```sh
* $ pulumi import azure:dataprotection/backupInstancePostgresql:BackupInstancePostgresql example /subscriptions/00000000-0000-0000-0000-000000000000/resourceGroups/group1/providers/Microsoft.DataProtection/backupVaults/vault1/backupInstances/backupInstance1
* ```
* @property backupPolicyId The ID of the Backup Policy.
* @property databaseCredentialKeyVaultSecretId The ID or versionless ID of the key vault secret which stores the connection string of the database.
* @property databaseId The ID of the source database. Changing this forces a new Backup Instance PostgreSQL to be created.
* @property location The location of the source database. Changing this forces a new Backup Instance PostgreSQL to be created.
* @property name The name which should be used for this Backup Instance PostgreSQL. Changing this forces a new Backup Instance PostgreSQL to be created.
* @property vaultId The ID of the Backup Vault within which the PostgreSQL Backup Instance should exist. Changing this forces a new Backup Instance PostgreSQL to be created.
*/
public data class BackupInstancePostgresqlArgs(
public val backupPolicyId: Output? = null,
public val databaseCredentialKeyVaultSecretId: Output? = null,
public val databaseId: Output? = null,
public val location: Output? = null,
public val name: Output? = null,
public val vaultId: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azure.dataprotection.BackupInstancePostgresqlArgs =
com.pulumi.azure.dataprotection.BackupInstancePostgresqlArgs.builder()
.backupPolicyId(backupPolicyId?.applyValue({ args0 -> args0 }))
.databaseCredentialKeyVaultSecretId(
databaseCredentialKeyVaultSecretId?.applyValue({ args0 ->
args0
}),
)
.databaseId(databaseId?.applyValue({ args0 -> args0 }))
.location(location?.applyValue({ args0 -> args0 }))
.name(name?.applyValue({ args0 -> args0 }))
.vaultId(vaultId?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [BackupInstancePostgresqlArgs].
*/
@PulumiTagMarker
public class BackupInstancePostgresqlArgsBuilder internal constructor() {
private var backupPolicyId: Output? = null
private var databaseCredentialKeyVaultSecretId: Output? = null
private var databaseId: Output? = null
private var location: Output? = null
private var name: Output? = null
private var vaultId: Output? = null
/**
* @param value The ID of the Backup Policy.
*/
@JvmName("fpeuyvxbryttgivf")
public suspend fun backupPolicyId(`value`: Output) {
this.backupPolicyId = value
}
/**
* @param value The ID or versionless ID of the key vault secret which stores the connection string of the database.
*/
@JvmName("uaxkewacdernftep")
public suspend fun databaseCredentialKeyVaultSecretId(`value`: Output) {
this.databaseCredentialKeyVaultSecretId = value
}
/**
* @param value The ID of the source database. Changing this forces a new Backup Instance PostgreSQL to be created.
*/
@JvmName("wmaqvyhvlmhwnsge")
public suspend fun databaseId(`value`: Output) {
this.databaseId = value
}
/**
* @param value The location of the source database. Changing this forces a new Backup Instance PostgreSQL to be created.
*/
@JvmName("cudmaexgdmwmrovn")
public suspend fun location(`value`: Output) {
this.location = value
}
/**
* @param value The name which should be used for this Backup Instance PostgreSQL. Changing this forces a new Backup Instance PostgreSQL to be created.
*/
@JvmName("sxoojqyebhxqxrjm")
public suspend fun name(`value`: Output) {
this.name = value
}
/**
* @param value The ID of the Backup Vault within which the PostgreSQL Backup Instance should exist. Changing this forces a new Backup Instance PostgreSQL to be created.
*/
@JvmName("egxadhbqpoipgmvk")
public suspend fun vaultId(`value`: Output) {
this.vaultId = value
}
/**
* @param value The ID of the Backup Policy.
*/
@JvmName("weaohujugpltgmhv")
public suspend fun backupPolicyId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.backupPolicyId = mapped
}
/**
* @param value The ID or versionless ID of the key vault secret which stores the connection string of the database.
*/
@JvmName("jkdarwcxhljqmkqf")
public suspend fun databaseCredentialKeyVaultSecretId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.databaseCredentialKeyVaultSecretId = mapped
}
/**
* @param value The ID of the source database. Changing this forces a new Backup Instance PostgreSQL to be created.
*/
@JvmName("qgxufqrwofvworaj")
public suspend fun databaseId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.databaseId = mapped
}
/**
* @param value The location of the source database. Changing this forces a new Backup Instance PostgreSQL to be created.
*/
@JvmName("suabcqhyvgpexgip")
public suspend fun location(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.location = mapped
}
/**
* @param value The name which should be used for this Backup Instance PostgreSQL. Changing this forces a new Backup Instance PostgreSQL to be created.
*/
@JvmName("ljwsigxceckfroci")
public suspend fun name(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.name = mapped
}
/**
* @param value The ID of the Backup Vault within which the PostgreSQL Backup Instance should exist. Changing this forces a new Backup Instance PostgreSQL to be created.
*/
@JvmName("bilmmefppuggheus")
public suspend fun vaultId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.vaultId = mapped
}
internal fun build(): BackupInstancePostgresqlArgs = BackupInstancePostgresqlArgs(
backupPolicyId = backupPolicyId,
databaseCredentialKeyVaultSecretId = databaseCredentialKeyVaultSecretId,
databaseId = databaseId,
location = location,
name = name,
vaultId = vaultId,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy