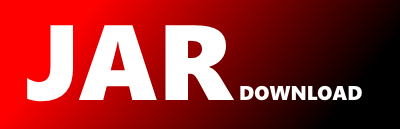
com.pulumi.azure.desktopvirtualization.kotlin.outputs.ScalingPlanSchedule.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-kotlin Show documentation
Show all versions of pulumi-azure-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azure.desktopvirtualization.kotlin.outputs
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
/**
*
* @property daysOfWeeks A list of Days of the Week on which this schedule will be used. Possible values are `Monday`, `Tuesday`, `Wednesday`, `Thursday`, `Friday`, `Saturday`, and `Sunday`
* @property name The name of the schedule.
* @property offPeakLoadBalancingAlgorithm The load Balancing Algorithm to use during Off-Peak Hours. Possible values are `DepthFirst` and `BreadthFirst`.
* @property offPeakStartTime The time at which Off-Peak scaling will begin. This is also the end-time for the Ramp-Down period. The time must be specified in "HH:MM" format.
* @property peakLoadBalancingAlgorithm The load Balancing Algorithm to use during Peak Hours. Possible values are `DepthFirst` and `BreadthFirst`.
* @property peakStartTime The time at which Peak scaling will begin. This is also the end-time for the Ramp-Up period. The time must be specified in "HH:MM" format.
* @property rampDownCapacityThresholdPercent This is the value in percentage of used host pool capacity that will be considered to evaluate whether to turn on/off virtual machines during the ramp-down and off-peak hours. For example, if capacity threshold is specified as 60% and your total host pool capacity is 100 sessions, autoscale will turn on additional session hosts once the host pool exceeds a load of 60 sessions.
* @property rampDownForceLogoffUsers Whether users will be forced to log-off session hosts once the `ramp_down_wait_time_minutes` value has been exceeded during the Ramp-Down period. Possible
* @property rampDownLoadBalancingAlgorithm The load Balancing Algorithm to use during the Ramp-Down period. Possible values are `DepthFirst` and `BreadthFirst`.
* @property rampDownMinimumHostsPercent The minimum percentage of session host virtual machines that you would like to get to for ramp-down and off-peak hours. For example, if Minimum percentage of hosts is specified as 10% and total number of session hosts in your host pool is 10, autoscale will ensure a minimum of 1 session host is available to take user connections.
* @property rampDownNotificationMessage The notification message to send to users during Ramp-Down period when they are required to log-off.
* @property rampDownStartTime The time at which Ramp-Down scaling will begin. This is also the end-time for the Ramp-Up period. The time must be specified in "HH:MM" format.
* @property rampDownStopHostsWhen Controls Session Host shutdown behaviour during Ramp-Down period. Session Hosts can either be shutdown when all sessions on the Session Host have ended, or when there are no Active sessions left on the Session Host. Possible values are `ZeroSessions` and `ZeroActiveSessions`.
* @property rampDownWaitTimeMinutes The number of minutes during Ramp-Down period that autoscale will wait after setting the session host VMs to drain mode, notifying any currently signed in users to save their work before forcing the users to logoff. Once all user sessions on the session host VM have been logged off, Autoscale will shut down the VM.
* @property rampUpCapacityThresholdPercent This is the value of percentage of used host pool capacity that will be considered to evaluate whether to turn on/off virtual machines during the ramp-up and peak hours. For example, if capacity threshold is specified as `60%` and your total host pool capacity is `100` sessions, autoscale will turn on additional session hosts once the host pool exceeds a load of `60` sessions.
* @property rampUpLoadBalancingAlgorithm The load Balancing Algorithm to use during the Ramp-Up period. Possible values are `DepthFirst` and `BreadthFirst`.
* @property rampUpMinimumHostsPercent Specifies the minimum percentage of session host virtual machines to start during ramp-up for peak hours. For example, if Minimum percentage of hosts is specified as `10%` and total number of session hosts in your host pool is `10`, autoscale will ensure a minimum of `1` session host is available to take user connections.
* @property rampUpStartTime The time at which Ramp-Up scaling will begin. This is also the end-time for the Ramp-Up period. The time must be specified in "HH:MM" format.
*/
public data class ScalingPlanSchedule(
public val daysOfWeeks: List,
public val name: String,
public val offPeakLoadBalancingAlgorithm: String,
public val offPeakStartTime: String,
public val peakLoadBalancingAlgorithm: String,
public val peakStartTime: String,
public val rampDownCapacityThresholdPercent: Int,
public val rampDownForceLogoffUsers: Boolean,
public val rampDownLoadBalancingAlgorithm: String,
public val rampDownMinimumHostsPercent: Int,
public val rampDownNotificationMessage: String,
public val rampDownStartTime: String,
public val rampDownStopHostsWhen: String,
public val rampDownWaitTimeMinutes: Int,
public val rampUpCapacityThresholdPercent: Int? = null,
public val rampUpLoadBalancingAlgorithm: String,
public val rampUpMinimumHostsPercent: Int? = null,
public val rampUpStartTime: String,
) {
public companion object {
public
fun toKotlin(javaType: com.pulumi.azure.desktopvirtualization.outputs.ScalingPlanSchedule):
ScalingPlanSchedule = ScalingPlanSchedule(
daysOfWeeks = javaType.daysOfWeeks().map({ args0 -> args0 }),
name = javaType.name(),
offPeakLoadBalancingAlgorithm = javaType.offPeakLoadBalancingAlgorithm(),
offPeakStartTime = javaType.offPeakStartTime(),
peakLoadBalancingAlgorithm = javaType.peakLoadBalancingAlgorithm(),
peakStartTime = javaType.peakStartTime(),
rampDownCapacityThresholdPercent = javaType.rampDownCapacityThresholdPercent(),
rampDownForceLogoffUsers = javaType.rampDownForceLogoffUsers(),
rampDownLoadBalancingAlgorithm = javaType.rampDownLoadBalancingAlgorithm(),
rampDownMinimumHostsPercent = javaType.rampDownMinimumHostsPercent(),
rampDownNotificationMessage = javaType.rampDownNotificationMessage(),
rampDownStartTime = javaType.rampDownStartTime(),
rampDownStopHostsWhen = javaType.rampDownStopHostsWhen(),
rampDownWaitTimeMinutes = javaType.rampDownWaitTimeMinutes(),
rampUpCapacityThresholdPercent = javaType.rampUpCapacityThresholdPercent().map({ args0 ->
args0
}).orElse(null),
rampUpLoadBalancingAlgorithm = javaType.rampUpLoadBalancingAlgorithm(),
rampUpMinimumHostsPercent = javaType.rampUpMinimumHostsPercent().map({ args0 ->
args0
}).orElse(null),
rampUpStartTime = javaType.rampUpStartTime(),
)
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy