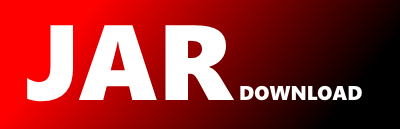
com.pulumi.azure.domainservices.kotlin.inputs.ServiceSecureLdapArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-kotlin Show documentation
Show all versions of pulumi-azure-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azure.domainservices.kotlin.inputs
import com.pulumi.azure.domainservices.inputs.ServiceSecureLdapArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
*
* @property certificateExpiry The expiry time of the certificate.
* @property certificateThumbprint The thumbprint of the certificate.
* @property enabled Whether to enable secure LDAP for the managed domain. For more information, please see [official documentation on enabling LDAPS](https://docs.microsoft.com/azure/active-directory-domain-services/tutorial-configure-ldaps), paying particular attention to the section on network security to avoid unnecessarily exposing your service to Internet-borne bruteforce attacks.
* @property externalAccessEnabled Whether to enable external access to LDAPS over the Internet. Defaults to `false`.
* @property pfxCertificate The certificate/private key to use for LDAPS, as a base64-encoded TripleDES-SHA1 encrypted PKCS#12 bundle (PFX file).
* @property pfxCertificatePassword The password to use for decrypting the PKCS#12 bundle (PFX file).
* @property publicCertificate The public certificate.
*/
public data class ServiceSecureLdapArgs(
public val certificateExpiry: Output? = null,
public val certificateThumbprint: Output? = null,
public val enabled: Output,
public val externalAccessEnabled: Output? = null,
public val pfxCertificate: Output,
public val pfxCertificatePassword: Output,
public val publicCertificate: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azure.domainservices.inputs.ServiceSecureLdapArgs =
com.pulumi.azure.domainservices.inputs.ServiceSecureLdapArgs.builder()
.certificateExpiry(certificateExpiry?.applyValue({ args0 -> args0 }))
.certificateThumbprint(certificateThumbprint?.applyValue({ args0 -> args0 }))
.enabled(enabled.applyValue({ args0 -> args0 }))
.externalAccessEnabled(externalAccessEnabled?.applyValue({ args0 -> args0 }))
.pfxCertificate(pfxCertificate.applyValue({ args0 -> args0 }))
.pfxCertificatePassword(pfxCertificatePassword.applyValue({ args0 -> args0 }))
.publicCertificate(publicCertificate?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [ServiceSecureLdapArgs].
*/
@PulumiTagMarker
public class ServiceSecureLdapArgsBuilder internal constructor() {
private var certificateExpiry: Output? = null
private var certificateThumbprint: Output? = null
private var enabled: Output? = null
private var externalAccessEnabled: Output? = null
private var pfxCertificate: Output? = null
private var pfxCertificatePassword: Output? = null
private var publicCertificate: Output? = null
/**
* @param value The expiry time of the certificate.
*/
@JvmName("qgxvdcruymucohis")
public suspend fun certificateExpiry(`value`: Output) {
this.certificateExpiry = value
}
/**
* @param value The thumbprint of the certificate.
*/
@JvmName("wycsqccnpcfnoayx")
public suspend fun certificateThumbprint(`value`: Output) {
this.certificateThumbprint = value
}
/**
* @param value Whether to enable secure LDAP for the managed domain. For more information, please see [official documentation on enabling LDAPS](https://docs.microsoft.com/azure/active-directory-domain-services/tutorial-configure-ldaps), paying particular attention to the section on network security to avoid unnecessarily exposing your service to Internet-borne bruteforce attacks.
*/
@JvmName("kihoubojkuvrlvfg")
public suspend fun enabled(`value`: Output) {
this.enabled = value
}
/**
* @param value Whether to enable external access to LDAPS over the Internet. Defaults to `false`.
*/
@JvmName("lrcogrtbsbrhcbtb")
public suspend fun externalAccessEnabled(`value`: Output) {
this.externalAccessEnabled = value
}
/**
* @param value The certificate/private key to use for LDAPS, as a base64-encoded TripleDES-SHA1 encrypted PKCS#12 bundle (PFX file).
*/
@JvmName("naymprglrsttxqdi")
public suspend fun pfxCertificate(`value`: Output) {
this.pfxCertificate = value
}
/**
* @param value The password to use for decrypting the PKCS#12 bundle (PFX file).
*/
@JvmName("lultmamleejbexxu")
public suspend fun pfxCertificatePassword(`value`: Output) {
this.pfxCertificatePassword = value
}
/**
* @param value The public certificate.
*/
@JvmName("gqvgstqouvpdkkbn")
public suspend fun publicCertificate(`value`: Output) {
this.publicCertificate = value
}
/**
* @param value The expiry time of the certificate.
*/
@JvmName("ipnpqxesbyqfykci")
public suspend fun certificateExpiry(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.certificateExpiry = mapped
}
/**
* @param value The thumbprint of the certificate.
*/
@JvmName("yboakajwdpexbubm")
public suspend fun certificateThumbprint(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.certificateThumbprint = mapped
}
/**
* @param value Whether to enable secure LDAP for the managed domain. For more information, please see [official documentation on enabling LDAPS](https://docs.microsoft.com/azure/active-directory-domain-services/tutorial-configure-ldaps), paying particular attention to the section on network security to avoid unnecessarily exposing your service to Internet-borne bruteforce attacks.
*/
@JvmName("vxdvljkacvlnhmsc")
public suspend fun enabled(`value`: Boolean) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.enabled = mapped
}
/**
* @param value Whether to enable external access to LDAPS over the Internet. Defaults to `false`.
*/
@JvmName("tpyarunwgrijlppb")
public suspend fun externalAccessEnabled(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.externalAccessEnabled = mapped
}
/**
* @param value The certificate/private key to use for LDAPS, as a base64-encoded TripleDES-SHA1 encrypted PKCS#12 bundle (PFX file).
*/
@JvmName("micytwpdioykoxdp")
public suspend fun pfxCertificate(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.pfxCertificate = mapped
}
/**
* @param value The password to use for decrypting the PKCS#12 bundle (PFX file).
*/
@JvmName("nhrowdsdcunowjgh")
public suspend fun pfxCertificatePassword(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.pfxCertificatePassword = mapped
}
/**
* @param value The public certificate.
*/
@JvmName("yrsvaqwdlkrcgoin")
public suspend fun publicCertificate(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.publicCertificate = mapped
}
internal fun build(): ServiceSecureLdapArgs = ServiceSecureLdapArgs(
certificateExpiry = certificateExpiry,
certificateThumbprint = certificateThumbprint,
enabled = enabled ?: throw PulumiNullFieldException("enabled"),
externalAccessEnabled = externalAccessEnabled,
pfxCertificate = pfxCertificate ?: throw PulumiNullFieldException("pfxCertificate"),
pfxCertificatePassword = pfxCertificatePassword ?: throw
PulumiNullFieldException("pfxCertificatePassword"),
publicCertificate = publicCertificate,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy