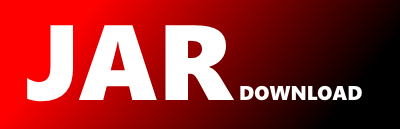
com.pulumi.azure.eventgrid.kotlin.inputs.SystemTopicEventSubscriptionWebhookEndpointArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-kotlin Show documentation
Show all versions of pulumi-azure-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azure.eventgrid.kotlin.inputs
import com.pulumi.azure.eventgrid.inputs.SystemTopicEventSubscriptionWebhookEndpointArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
*
* @property activeDirectoryAppIdOrUri The Azure Active Directory Application ID or URI to get the access token that will be included as the bearer token in delivery requests.
* @property activeDirectoryTenantId The Azure Active Directory Tenant ID to get the access token that will be included as the bearer token in delivery requests.
* @property baseUrl The base url of the webhook where the Event Subscription will receive events.
* @property maxEventsPerBatch Maximum number of events per batch.
* @property preferredBatchSizeInKilobytes Preferred batch size in Kilobytes.
* @property url Specifies the url of the webhook where the Event Subscription will receive events.
*/
public data class SystemTopicEventSubscriptionWebhookEndpointArgs(
public val activeDirectoryAppIdOrUri: Output? = null,
public val activeDirectoryTenantId: Output? = null,
public val baseUrl: Output? = null,
public val maxEventsPerBatch: Output? = null,
public val preferredBatchSizeInKilobytes: Output? = null,
public val url: Output,
) :
ConvertibleToJava {
override fun toJava():
com.pulumi.azure.eventgrid.inputs.SystemTopicEventSubscriptionWebhookEndpointArgs =
com.pulumi.azure.eventgrid.inputs.SystemTopicEventSubscriptionWebhookEndpointArgs.builder()
.activeDirectoryAppIdOrUri(activeDirectoryAppIdOrUri?.applyValue({ args0 -> args0 }))
.activeDirectoryTenantId(activeDirectoryTenantId?.applyValue({ args0 -> args0 }))
.baseUrl(baseUrl?.applyValue({ args0 -> args0 }))
.maxEventsPerBatch(maxEventsPerBatch?.applyValue({ args0 -> args0 }))
.preferredBatchSizeInKilobytes(preferredBatchSizeInKilobytes?.applyValue({ args0 -> args0 }))
.url(url.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [SystemTopicEventSubscriptionWebhookEndpointArgs].
*/
@PulumiTagMarker
public class SystemTopicEventSubscriptionWebhookEndpointArgsBuilder internal constructor() {
private var activeDirectoryAppIdOrUri: Output? = null
private var activeDirectoryTenantId: Output? = null
private var baseUrl: Output? = null
private var maxEventsPerBatch: Output? = null
private var preferredBatchSizeInKilobytes: Output? = null
private var url: Output? = null
/**
* @param value The Azure Active Directory Application ID or URI to get the access token that will be included as the bearer token in delivery requests.
*/
@JvmName("incjnmtlyfromeqd")
public suspend fun activeDirectoryAppIdOrUri(`value`: Output) {
this.activeDirectoryAppIdOrUri = value
}
/**
* @param value The Azure Active Directory Tenant ID to get the access token that will be included as the bearer token in delivery requests.
*/
@JvmName("cpunlwqhstoeaflv")
public suspend fun activeDirectoryTenantId(`value`: Output) {
this.activeDirectoryTenantId = value
}
/**
* @param value The base url of the webhook where the Event Subscription will receive events.
*/
@JvmName("fwxixbpjiploydxa")
public suspend fun baseUrl(`value`: Output) {
this.baseUrl = value
}
/**
* @param value Maximum number of events per batch.
*/
@JvmName("euetoirqbkplqxff")
public suspend fun maxEventsPerBatch(`value`: Output) {
this.maxEventsPerBatch = value
}
/**
* @param value Preferred batch size in Kilobytes.
*/
@JvmName("jkmdrrdsawmwuuel")
public suspend fun preferredBatchSizeInKilobytes(`value`: Output) {
this.preferredBatchSizeInKilobytes = value
}
/**
* @param value Specifies the url of the webhook where the Event Subscription will receive events.
*/
@JvmName("gllyqjwwlepygwhl")
public suspend fun url(`value`: Output) {
this.url = value
}
/**
* @param value The Azure Active Directory Application ID or URI to get the access token that will be included as the bearer token in delivery requests.
*/
@JvmName("jvyemagwdukmvwkl")
public suspend fun activeDirectoryAppIdOrUri(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.activeDirectoryAppIdOrUri = mapped
}
/**
* @param value The Azure Active Directory Tenant ID to get the access token that will be included as the bearer token in delivery requests.
*/
@JvmName("wayricffqbphnfdh")
public suspend fun activeDirectoryTenantId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.activeDirectoryTenantId = mapped
}
/**
* @param value The base url of the webhook where the Event Subscription will receive events.
*/
@JvmName("rsbjrsfviukkueln")
public suspend fun baseUrl(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.baseUrl = mapped
}
/**
* @param value Maximum number of events per batch.
*/
@JvmName("skcjuigcqgprwats")
public suspend fun maxEventsPerBatch(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.maxEventsPerBatch = mapped
}
/**
* @param value Preferred batch size in Kilobytes.
*/
@JvmName("udtrulnclpuykhce")
public suspend fun preferredBatchSizeInKilobytes(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.preferredBatchSizeInKilobytes = mapped
}
/**
* @param value Specifies the url of the webhook where the Event Subscription will receive events.
*/
@JvmName("cstpfatydvbahxvi")
public suspend fun url(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.url = mapped
}
internal fun build(): SystemTopicEventSubscriptionWebhookEndpointArgs =
SystemTopicEventSubscriptionWebhookEndpointArgs(
activeDirectoryAppIdOrUri = activeDirectoryAppIdOrUri,
activeDirectoryTenantId = activeDirectoryTenantId,
baseUrl = baseUrl,
maxEventsPerBatch = maxEventsPerBatch,
preferredBatchSizeInKilobytes = preferredBatchSizeInKilobytes,
url = url ?: throw PulumiNullFieldException("url"),
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy