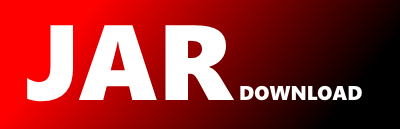
com.pulumi.azure.eventhub.kotlin.SubscriptionArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-kotlin Show documentation
Show all versions of pulumi-azure-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azure.eventhub.kotlin
import com.pulumi.azure.eventhub.SubscriptionArgs.builder
import com.pulumi.azure.eventhub.kotlin.inputs.SubscriptionClientScopedSubscriptionArgs
import com.pulumi.azure.eventhub.kotlin.inputs.SubscriptionClientScopedSubscriptionArgsBuilder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Boolean
import kotlin.Deprecated
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
* Manages a ServiceBus Subscription.
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as azure from "@pulumi/azure";
* const example = new azure.core.ResourceGroup("example", {
* name: "tfex-servicebus-subscription",
* location: "West Europe",
* });
* const exampleNamespace = new azure.servicebus.Namespace("example", {
* name: "tfex-servicebus-namespace",
* location: example.location,
* resourceGroupName: example.name,
* sku: "Standard",
* tags: {
* source: "example",
* },
* });
* const exampleTopic = new azure.servicebus.Topic("example", {
* name: "tfex_servicebus_topic",
* namespaceId: exampleNamespace.id,
* enablePartitioning: true,
* });
* const exampleSubscription = new azure.servicebus.Subscription("example", {
* name: "tfex_servicebus_subscription",
* topicId: exampleTopic.id,
* maxDeliveryCount: 1,
* });
* ```
* ```python
* import pulumi
* import pulumi_azure as azure
* example = azure.core.ResourceGroup("example",
* name="tfex-servicebus-subscription",
* location="West Europe")
* example_namespace = azure.servicebus.Namespace("example",
* name="tfex-servicebus-namespace",
* location=example.location,
* resource_group_name=example.name,
* sku="Standard",
* tags={
* "source": "example",
* })
* example_topic = azure.servicebus.Topic("example",
* name="tfex_servicebus_topic",
* namespace_id=example_namespace.id,
* enable_partitioning=True)
* example_subscription = azure.servicebus.Subscription("example",
* name="tfex_servicebus_subscription",
* topic_id=example_topic.id,
* max_delivery_count=1)
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Azure = Pulumi.Azure;
* return await Deployment.RunAsync(() =>
* {
* var example = new Azure.Core.ResourceGroup("example", new()
* {
* Name = "tfex-servicebus-subscription",
* Location = "West Europe",
* });
* var exampleNamespace = new Azure.ServiceBus.Namespace("example", new()
* {
* Name = "tfex-servicebus-namespace",
* Location = example.Location,
* ResourceGroupName = example.Name,
* Sku = "Standard",
* Tags =
* {
* { "source", "example" },
* },
* });
* var exampleTopic = new Azure.ServiceBus.Topic("example", new()
* {
* Name = "tfex_servicebus_topic",
* NamespaceId = exampleNamespace.Id,
* EnablePartitioning = true,
* });
* var exampleSubscription = new Azure.ServiceBus.Subscription("example", new()
* {
* Name = "tfex_servicebus_subscription",
* TopicId = exampleTopic.Id,
* MaxDeliveryCount = 1,
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-azure/sdk/v5/go/azure/core"
* "github.com/pulumi/pulumi-azure/sdk/v5/go/azure/servicebus"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* example, err := core.NewResourceGroup(ctx, "example", &core.ResourceGroupArgs{
* Name: pulumi.String("tfex-servicebus-subscription"),
* Location: pulumi.String("West Europe"),
* })
* if err != nil {
* return err
* }
* exampleNamespace, err := servicebus.NewNamespace(ctx, "example", &servicebus.NamespaceArgs{
* Name: pulumi.String("tfex-servicebus-namespace"),
* Location: example.Location,
* ResourceGroupName: example.Name,
* Sku: pulumi.String("Standard"),
* Tags: pulumi.StringMap{
* "source": pulumi.String("example"),
* },
* })
* if err != nil {
* return err
* }
* exampleTopic, err := servicebus.NewTopic(ctx, "example", &servicebus.TopicArgs{
* Name: pulumi.String("tfex_servicebus_topic"),
* NamespaceId: exampleNamespace.ID(),
* EnablePartitioning: pulumi.Bool(true),
* })
* if err != nil {
* return err
* }
* _, err = servicebus.NewSubscription(ctx, "example", &servicebus.SubscriptionArgs{
* Name: pulumi.String("tfex_servicebus_subscription"),
* TopicId: exampleTopic.ID(),
* MaxDeliveryCount: pulumi.Int(1),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azure.core.ResourceGroup;
* import com.pulumi.azure.core.ResourceGroupArgs;
* import com.pulumi.azure.servicebus.Namespace;
* import com.pulumi.azure.servicebus.NamespaceArgs;
* import com.pulumi.azure.servicebus.Topic;
* import com.pulumi.azure.servicebus.TopicArgs;
* import com.pulumi.azure.servicebus.Subscription;
* import com.pulumi.azure.servicebus.SubscriptionArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var example = new ResourceGroup("example", ResourceGroupArgs.builder()
* .name("tfex-servicebus-subscription")
* .location("West Europe")
* .build());
* var exampleNamespace = new Namespace("exampleNamespace", NamespaceArgs.builder()
* .name("tfex-servicebus-namespace")
* .location(example.location())
* .resourceGroupName(example.name())
* .sku("Standard")
* .tags(Map.of("source", "example"))
* .build());
* var exampleTopic = new Topic("exampleTopic", TopicArgs.builder()
* .name("tfex_servicebus_topic")
* .namespaceId(exampleNamespace.id())
* .enablePartitioning(true)
* .build());
* var exampleSubscription = new Subscription("exampleSubscription", SubscriptionArgs.builder()
* .name("tfex_servicebus_subscription")
* .topicId(exampleTopic.id())
* .maxDeliveryCount(1)
* .build());
* }
* }
* ```
* ```yaml
* resources:
* example:
* type: azure:core:ResourceGroup
* properties:
* name: tfex-servicebus-subscription
* location: West Europe
* exampleNamespace:
* type: azure:servicebus:Namespace
* name: example
* properties:
* name: tfex-servicebus-namespace
* location: ${example.location}
* resourceGroupName: ${example.name}
* sku: Standard
* tags:
* source: example
* exampleTopic:
* type: azure:servicebus:Topic
* name: example
* properties:
* name: tfex_servicebus_topic
* namespaceId: ${exampleNamespace.id}
* enablePartitioning: true
* exampleSubscription:
* type: azure:servicebus:Subscription
* name: example
* properties:
* name: tfex_servicebus_subscription
* topicId: ${exampleTopic.id}
* maxDeliveryCount: 1
* ```
*
* ## Import
* Service Bus Subscriptions can be imported using the `resource id`, e.g.
* ```sh
* $ pulumi import azure:eventhub/subscription:Subscription example /subscriptions/00000000-0000-0000-0000-000000000000/resourceGroups/mygroup1/providers/Microsoft.ServiceBus/namespaces/sbns1/topics/sntopic1/subscriptions/sbsub1
* ```
* @property autoDeleteOnIdle The idle interval after which the topic is automatically deleted as an [ISO 8601 duration](https://en.wikipedia.org/wiki/ISO_8601#Durations). The minimum duration is `5` minutes or `PT5M`.
* @property clientScopedSubscription A `client_scoped_subscription` block as defined below.
* @property clientScopedSubscriptionEnabled whether the subscription is scoped to a client id. Defaults to `false`.
* > **NOTE:** Client Scoped Subscription can only be used for JMS subscription (Java Message Service).
* @property deadLetteringOnFilterEvaluationError Boolean flag which controls whether the Subscription has dead letter support on filter evaluation exceptions. Defaults to `true`.
* @property deadLetteringOnMessageExpiration Boolean flag which controls whether the Subscription has dead letter support when a message expires.
* @property defaultMessageTtl The Default message timespan to live as an [ISO 8601 duration](https://en.wikipedia.org/wiki/ISO_8601#Durations). This is the duration after which the message expires, starting from when the message is sent to Service Bus. This is the default value used when TimeToLive is not set on a message itself.
* @property enableBatchedOperations Boolean flag which controls whether the Subscription supports batched operations.
* @property forwardDeadLetteredMessagesTo The name of a Queue or Topic to automatically forward Dead Letter messages to.
* @property forwardTo The name of a Queue or Topic to automatically forward messages to.
* @property lockDuration The lock duration for the subscription as an [ISO 8601 duration](https://en.wikipedia.org/wiki/ISO_8601#Durations). The default value is `1` minute or `P0DT0H1M0S` . The maximum value is `5` minutes or `P0DT0H5M0S` .
* @property maxDeliveryCount The maximum number of deliveries.
* @property name Specifies the name of the ServiceBus Subscription resource. Changing this forces a new resource to be created.
* @property requiresSession Boolean flag which controls whether this Subscription supports the concept of a session. Changing this forces a new resource to be created.
* @property status The status of the Subscription. Possible values are `Active`,`ReceiveDisabled`, or `Disabled`. Defaults to `Active`.
* @property topicId The ID of the ServiceBus Topic to create this Subscription in. Changing this forces a new resource to be created.
*/
public data class SubscriptionArgs @Deprecated(
message = """
azure.eventhub.Subscription has been deprecated in favor of azure.servicebus.Subscription
""",
) constructor(
public val autoDeleteOnIdle: Output? = null,
public val clientScopedSubscription: Output? = null,
public val clientScopedSubscriptionEnabled: Output? = null,
public val deadLetteringOnFilterEvaluationError: Output? = null,
public val deadLetteringOnMessageExpiration: Output? = null,
public val defaultMessageTtl: Output? = null,
public val enableBatchedOperations: Output? = null,
public val forwardDeadLetteredMessagesTo: Output? = null,
public val forwardTo: Output? = null,
public val lockDuration: Output? = null,
public val maxDeliveryCount: Output? = null,
public val name: Output? = null,
public val requiresSession: Output? = null,
public val status: Output? = null,
public val topicId: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azure.eventhub.SubscriptionArgs =
com.pulumi.azure.eventhub.SubscriptionArgs.builder()
.autoDeleteOnIdle(autoDeleteOnIdle?.applyValue({ args0 -> args0 }))
.clientScopedSubscription(
clientScopedSubscription?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.clientScopedSubscriptionEnabled(clientScopedSubscriptionEnabled?.applyValue({ args0 -> args0 }))
.deadLetteringOnFilterEvaluationError(
deadLetteringOnFilterEvaluationError?.applyValue({ args0 ->
args0
}),
)
.deadLetteringOnMessageExpiration(deadLetteringOnMessageExpiration?.applyValue({ args0 -> args0 }))
.defaultMessageTtl(defaultMessageTtl?.applyValue({ args0 -> args0 }))
.enableBatchedOperations(enableBatchedOperations?.applyValue({ args0 -> args0 }))
.forwardDeadLetteredMessagesTo(forwardDeadLetteredMessagesTo?.applyValue({ args0 -> args0 }))
.forwardTo(forwardTo?.applyValue({ args0 -> args0 }))
.lockDuration(lockDuration?.applyValue({ args0 -> args0 }))
.maxDeliveryCount(maxDeliveryCount?.applyValue({ args0 -> args0 }))
.name(name?.applyValue({ args0 -> args0 }))
.requiresSession(requiresSession?.applyValue({ args0 -> args0 }))
.status(status?.applyValue({ args0 -> args0 }))
.topicId(topicId?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [SubscriptionArgs].
*/
@PulumiTagMarker
@Deprecated(
message = """
azure.eventhub.Subscription has been deprecated in favor of azure.servicebus.Subscription
""",
)
public class SubscriptionArgsBuilder internal constructor() {
private var autoDeleteOnIdle: Output? = null
private var clientScopedSubscription: Output? = null
private var clientScopedSubscriptionEnabled: Output? = null
private var deadLetteringOnFilterEvaluationError: Output? = null
private var deadLetteringOnMessageExpiration: Output? = null
private var defaultMessageTtl: Output? = null
private var enableBatchedOperations: Output? = null
private var forwardDeadLetteredMessagesTo: Output? = null
private var forwardTo: Output? = null
private var lockDuration: Output? = null
private var maxDeliveryCount: Output? = null
private var name: Output? = null
private var requiresSession: Output? = null
private var status: Output? = null
private var topicId: Output? = null
/**
* @param value The idle interval after which the topic is automatically deleted as an [ISO 8601 duration](https://en.wikipedia.org/wiki/ISO_8601#Durations). The minimum duration is `5` minutes or `PT5M`.
*/
@JvmName("ysiypewulrlvdcox")
public suspend fun autoDeleteOnIdle(`value`: Output) {
this.autoDeleteOnIdle = value
}
/**
* @param value A `client_scoped_subscription` block as defined below.
*/
@JvmName("fxepnqjnowblaadr")
public suspend
fun clientScopedSubscription(`value`: Output) {
this.clientScopedSubscription = value
}
/**
* @param value whether the subscription is scoped to a client id. Defaults to `false`.
* > **NOTE:** Client Scoped Subscription can only be used for JMS subscription (Java Message Service).
*/
@JvmName("nncanygmuuurglfo")
public suspend fun clientScopedSubscriptionEnabled(`value`: Output) {
this.clientScopedSubscriptionEnabled = value
}
/**
* @param value Boolean flag which controls whether the Subscription has dead letter support on filter evaluation exceptions. Defaults to `true`.
*/
@JvmName("wnkxakbcgfatslhs")
public suspend fun deadLetteringOnFilterEvaluationError(`value`: Output) {
this.deadLetteringOnFilterEvaluationError = value
}
/**
* @param value Boolean flag which controls whether the Subscription has dead letter support when a message expires.
*/
@JvmName("lmfdnjsqssataivk")
public suspend fun deadLetteringOnMessageExpiration(`value`: Output) {
this.deadLetteringOnMessageExpiration = value
}
/**
* @param value The Default message timespan to live as an [ISO 8601 duration](https://en.wikipedia.org/wiki/ISO_8601#Durations). This is the duration after which the message expires, starting from when the message is sent to Service Bus. This is the default value used when TimeToLive is not set on a message itself.
*/
@JvmName("pwaehnpjkdjspvtj")
public suspend fun defaultMessageTtl(`value`: Output) {
this.defaultMessageTtl = value
}
/**
* @param value Boolean flag which controls whether the Subscription supports batched operations.
*/
@JvmName("nfooaydpwvfprpfk")
public suspend fun enableBatchedOperations(`value`: Output) {
this.enableBatchedOperations = value
}
/**
* @param value The name of a Queue or Topic to automatically forward Dead Letter messages to.
*/
@JvmName("ckfvkqaswhrtnyxc")
public suspend fun forwardDeadLetteredMessagesTo(`value`: Output) {
this.forwardDeadLetteredMessagesTo = value
}
/**
* @param value The name of a Queue or Topic to automatically forward messages to.
*/
@JvmName("uwbmyhxhyakhqmkx")
public suspend fun forwardTo(`value`: Output) {
this.forwardTo = value
}
/**
* @param value The lock duration for the subscription as an [ISO 8601 duration](https://en.wikipedia.org/wiki/ISO_8601#Durations). The default value is `1` minute or `P0DT0H1M0S` . The maximum value is `5` minutes or `P0DT0H5M0S` .
*/
@JvmName("tljrjomsywibxnhf")
public suspend fun lockDuration(`value`: Output) {
this.lockDuration = value
}
/**
* @param value The maximum number of deliveries.
*/
@JvmName("lfcjrnjgfaspvgkf")
public suspend fun maxDeliveryCount(`value`: Output) {
this.maxDeliveryCount = value
}
/**
* @param value Specifies the name of the ServiceBus Subscription resource. Changing this forces a new resource to be created.
*/
@JvmName("lxjwjgejmmevldgb")
public suspend fun name(`value`: Output) {
this.name = value
}
/**
* @param value Boolean flag which controls whether this Subscription supports the concept of a session. Changing this forces a new resource to be created.
*/
@JvmName("txptkuxoectnigbo")
public suspend fun requiresSession(`value`: Output) {
this.requiresSession = value
}
/**
* @param value The status of the Subscription. Possible values are `Active`,`ReceiveDisabled`, or `Disabled`. Defaults to `Active`.
*/
@JvmName("hyohwprdftjwyqjq")
public suspend fun status(`value`: Output) {
this.status = value
}
/**
* @param value The ID of the ServiceBus Topic to create this Subscription in. Changing this forces a new resource to be created.
*/
@JvmName("jccgucbygjcunsdo")
public suspend fun topicId(`value`: Output) {
this.topicId = value
}
/**
* @param value The idle interval after which the topic is automatically deleted as an [ISO 8601 duration](https://en.wikipedia.org/wiki/ISO_8601#Durations). The minimum duration is `5` minutes or `PT5M`.
*/
@JvmName("guawwcaftrfauujc")
public suspend fun autoDeleteOnIdle(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.autoDeleteOnIdle = mapped
}
/**
* @param value A `client_scoped_subscription` block as defined below.
*/
@JvmName("gcabudhoqmoajtxm")
public suspend fun clientScopedSubscription(`value`: SubscriptionClientScopedSubscriptionArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.clientScopedSubscription = mapped
}
/**
* @param argument A `client_scoped_subscription` block as defined below.
*/
@JvmName("qveovrfbjvdnkhpm")
public suspend
fun clientScopedSubscription(argument: suspend SubscriptionClientScopedSubscriptionArgsBuilder.() -> Unit) {
val toBeMapped = SubscriptionClientScopedSubscriptionArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.clientScopedSubscription = mapped
}
/**
* @param value whether the subscription is scoped to a client id. Defaults to `false`.
* > **NOTE:** Client Scoped Subscription can only be used for JMS subscription (Java Message Service).
*/
@JvmName("lfquosyisjwtlnfi")
public suspend fun clientScopedSubscriptionEnabled(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.clientScopedSubscriptionEnabled = mapped
}
/**
* @param value Boolean flag which controls whether the Subscription has dead letter support on filter evaluation exceptions. Defaults to `true`.
*/
@JvmName("abdjkmkfcqbjnkjc")
public suspend fun deadLetteringOnFilterEvaluationError(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.deadLetteringOnFilterEvaluationError = mapped
}
/**
* @param value Boolean flag which controls whether the Subscription has dead letter support when a message expires.
*/
@JvmName("joyvnrocceqiicvt")
public suspend fun deadLetteringOnMessageExpiration(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.deadLetteringOnMessageExpiration = mapped
}
/**
* @param value The Default message timespan to live as an [ISO 8601 duration](https://en.wikipedia.org/wiki/ISO_8601#Durations). This is the duration after which the message expires, starting from when the message is sent to Service Bus. This is the default value used when TimeToLive is not set on a message itself.
*/
@JvmName("qpbuhblgesqusqyd")
public suspend fun defaultMessageTtl(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.defaultMessageTtl = mapped
}
/**
* @param value Boolean flag which controls whether the Subscription supports batched operations.
*/
@JvmName("kmasaaopbklsrsuw")
public suspend fun enableBatchedOperations(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.enableBatchedOperations = mapped
}
/**
* @param value The name of a Queue or Topic to automatically forward Dead Letter messages to.
*/
@JvmName("onfoethbqepibxjw")
public suspend fun forwardDeadLetteredMessagesTo(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.forwardDeadLetteredMessagesTo = mapped
}
/**
* @param value The name of a Queue or Topic to automatically forward messages to.
*/
@JvmName("npufafqlcoimqavo")
public suspend fun forwardTo(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.forwardTo = mapped
}
/**
* @param value The lock duration for the subscription as an [ISO 8601 duration](https://en.wikipedia.org/wiki/ISO_8601#Durations). The default value is `1` minute or `P0DT0H1M0S` . The maximum value is `5` minutes or `P0DT0H5M0S` .
*/
@JvmName("ismvxaychwrnartq")
public suspend fun lockDuration(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.lockDuration = mapped
}
/**
* @param value The maximum number of deliveries.
*/
@JvmName("nltwgvdxfdxdolyh")
public suspend fun maxDeliveryCount(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.maxDeliveryCount = mapped
}
/**
* @param value Specifies the name of the ServiceBus Subscription resource. Changing this forces a new resource to be created.
*/
@JvmName("xetukxbqkbhdjric")
public suspend fun name(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.name = mapped
}
/**
* @param value Boolean flag which controls whether this Subscription supports the concept of a session. Changing this forces a new resource to be created.
*/
@JvmName("poeenioshcanknwx")
public suspend fun requiresSession(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.requiresSession = mapped
}
/**
* @param value The status of the Subscription. Possible values are `Active`,`ReceiveDisabled`, or `Disabled`. Defaults to `Active`.
*/
@JvmName("mbfldmxtijfkpghy")
public suspend fun status(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.status = mapped
}
/**
* @param value The ID of the ServiceBus Topic to create this Subscription in. Changing this forces a new resource to be created.
*/
@JvmName("ihrrsvqxvmocsfuj")
public suspend fun topicId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.topicId = mapped
}
internal fun build(): SubscriptionArgs = SubscriptionArgs(
autoDeleteOnIdle = autoDeleteOnIdle,
clientScopedSubscription = clientScopedSubscription,
clientScopedSubscriptionEnabled = clientScopedSubscriptionEnabled,
deadLetteringOnFilterEvaluationError = deadLetteringOnFilterEvaluationError,
deadLetteringOnMessageExpiration = deadLetteringOnMessageExpiration,
defaultMessageTtl = defaultMessageTtl,
enableBatchedOperations = enableBatchedOperations,
forwardDeadLetteredMessagesTo = forwardDeadLetteredMessagesTo,
forwardTo = forwardTo,
lockDuration = lockDuration,
maxDeliveryCount = maxDeliveryCount,
name = name,
requiresSession = requiresSession,
status = status,
topicId = topicId,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy