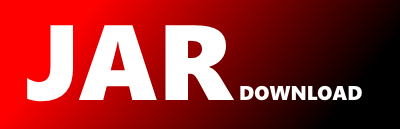
com.pulumi.azure.iot.kotlin.inputs.SecuritySolutionRecommendationsEnabledArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-kotlin Show documentation
Show all versions of pulumi-azure-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azure.iot.kotlin.inputs
import com.pulumi.azure.iot.inputs.SecuritySolutionRecommendationsEnabledArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Boolean
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
*
* @property acrAuthentication Is Principal Authentication enabled for the ACR repository? Defaults to `true`.
* @property agentSendUnutilizedMsg Is Agent send underutilized messages enabled? Defaults to `true`.
* @property baseline Is Security related system configuration issues identified? Defaults to `true`.
* @property edgeHubMemOptimize Is IoT Edge Hub memory optimized? Defaults to `true`.
* @property edgeLoggingOption Is logging configured for IoT Edge module? Defaults to `true`.
* @property inconsistentModuleSettings Is inconsistent module settings enabled for SecurityGroup? Defaults to `true`.
* @property installAgent is Azure IoT Security agent installed? Defaults to `true`.
* @property ipFilterDenyAll Is Default IP filter policy denied? Defaults to `true`.
* @property ipFilterPermissiveRule Is IP filter rule source allowable IP range too large? Defaults to `true`.
* @property openPorts Is any ports open on the device? Defaults to `true`.
* @property permissiveFirewallPolicy Does firewall policy exist which allow necessary communication to/from the device? Defaults to `true`.
* @property permissiveInputFirewallRules Is only necessary addresses or ports are permitted in? Defaults to `true`.
* @property permissiveOutputFirewallRules Is only necessary addresses or ports are permitted out? Defaults to `true`.
* @property privilegedDockerOptions Is high level permissions are needed for the module? Defaults to `true`.
* @property sharedCredentials Is any credentials shared among devices? Defaults to `true`.
* @property vulnerableTlsCipherSuite Does TLS cipher suite need to be updated? Defaults to `true`.
*/
public data class SecuritySolutionRecommendationsEnabledArgs(
public val acrAuthentication: Output? = null,
public val agentSendUnutilizedMsg: Output? = null,
public val baseline: Output? = null,
public val edgeHubMemOptimize: Output? = null,
public val edgeLoggingOption: Output? = null,
public val inconsistentModuleSettings: Output? = null,
public val installAgent: Output? = null,
public val ipFilterDenyAll: Output? = null,
public val ipFilterPermissiveRule: Output? = null,
public val openPorts: Output? = null,
public val permissiveFirewallPolicy: Output? = null,
public val permissiveInputFirewallRules: Output? = null,
public val permissiveOutputFirewallRules: Output? = null,
public val privilegedDockerOptions: Output? = null,
public val sharedCredentials: Output? = null,
public val vulnerableTlsCipherSuite: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azure.iot.inputs.SecuritySolutionRecommendationsEnabledArgs =
com.pulumi.azure.iot.inputs.SecuritySolutionRecommendationsEnabledArgs.builder()
.acrAuthentication(acrAuthentication?.applyValue({ args0 -> args0 }))
.agentSendUnutilizedMsg(agentSendUnutilizedMsg?.applyValue({ args0 -> args0 }))
.baseline(baseline?.applyValue({ args0 -> args0 }))
.edgeHubMemOptimize(edgeHubMemOptimize?.applyValue({ args0 -> args0 }))
.edgeLoggingOption(edgeLoggingOption?.applyValue({ args0 -> args0 }))
.inconsistentModuleSettings(inconsistentModuleSettings?.applyValue({ args0 -> args0 }))
.installAgent(installAgent?.applyValue({ args0 -> args0 }))
.ipFilterDenyAll(ipFilterDenyAll?.applyValue({ args0 -> args0 }))
.ipFilterPermissiveRule(ipFilterPermissiveRule?.applyValue({ args0 -> args0 }))
.openPorts(openPorts?.applyValue({ args0 -> args0 }))
.permissiveFirewallPolicy(permissiveFirewallPolicy?.applyValue({ args0 -> args0 }))
.permissiveInputFirewallRules(permissiveInputFirewallRules?.applyValue({ args0 -> args0 }))
.permissiveOutputFirewallRules(permissiveOutputFirewallRules?.applyValue({ args0 -> args0 }))
.privilegedDockerOptions(privilegedDockerOptions?.applyValue({ args0 -> args0 }))
.sharedCredentials(sharedCredentials?.applyValue({ args0 -> args0 }))
.vulnerableTlsCipherSuite(vulnerableTlsCipherSuite?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [SecuritySolutionRecommendationsEnabledArgs].
*/
@PulumiTagMarker
public class SecuritySolutionRecommendationsEnabledArgsBuilder internal constructor() {
private var acrAuthentication: Output? = null
private var agentSendUnutilizedMsg: Output? = null
private var baseline: Output? = null
private var edgeHubMemOptimize: Output? = null
private var edgeLoggingOption: Output? = null
private var inconsistentModuleSettings: Output? = null
private var installAgent: Output? = null
private var ipFilterDenyAll: Output? = null
private var ipFilterPermissiveRule: Output? = null
private var openPorts: Output? = null
private var permissiveFirewallPolicy: Output? = null
private var permissiveInputFirewallRules: Output? = null
private var permissiveOutputFirewallRules: Output? = null
private var privilegedDockerOptions: Output? = null
private var sharedCredentials: Output? = null
private var vulnerableTlsCipherSuite: Output? = null
/**
* @param value Is Principal Authentication enabled for the ACR repository? Defaults to `true`.
*/
@JvmName("ixanrwesbylknbom")
public suspend fun acrAuthentication(`value`: Output) {
this.acrAuthentication = value
}
/**
* @param value Is Agent send underutilized messages enabled? Defaults to `true`.
*/
@JvmName("ucmehbqabatvyhjt")
public suspend fun agentSendUnutilizedMsg(`value`: Output) {
this.agentSendUnutilizedMsg = value
}
/**
* @param value Is Security related system configuration issues identified? Defaults to `true`.
*/
@JvmName("ovgxrhutqyfwnjou")
public suspend fun baseline(`value`: Output) {
this.baseline = value
}
/**
* @param value Is IoT Edge Hub memory optimized? Defaults to `true`.
*/
@JvmName("nbenhedxmjgpgqiq")
public suspend fun edgeHubMemOptimize(`value`: Output) {
this.edgeHubMemOptimize = value
}
/**
* @param value Is logging configured for IoT Edge module? Defaults to `true`.
*/
@JvmName("chbjmekednvvndxo")
public suspend fun edgeLoggingOption(`value`: Output) {
this.edgeLoggingOption = value
}
/**
* @param value Is inconsistent module settings enabled for SecurityGroup? Defaults to `true`.
*/
@JvmName("laskwwoiumttujuh")
public suspend fun inconsistentModuleSettings(`value`: Output) {
this.inconsistentModuleSettings = value
}
/**
* @param value is Azure IoT Security agent installed? Defaults to `true`.
*/
@JvmName("ysaukdcvyrnfaqde")
public suspend fun installAgent(`value`: Output) {
this.installAgent = value
}
/**
* @param value Is Default IP filter policy denied? Defaults to `true`.
*/
@JvmName("udgsojwpholmvkax")
public suspend fun ipFilterDenyAll(`value`: Output) {
this.ipFilterDenyAll = value
}
/**
* @param value Is IP filter rule source allowable IP range too large? Defaults to `true`.
*/
@JvmName("aqgrlxayvutwrvmu")
public suspend fun ipFilterPermissiveRule(`value`: Output) {
this.ipFilterPermissiveRule = value
}
/**
* @param value Is any ports open on the device? Defaults to `true`.
*/
@JvmName("xrmakpffejwjhhox")
public suspend fun openPorts(`value`: Output) {
this.openPorts = value
}
/**
* @param value Does firewall policy exist which allow necessary communication to/from the device? Defaults to `true`.
*/
@JvmName("boqofjginomgukcw")
public suspend fun permissiveFirewallPolicy(`value`: Output) {
this.permissiveFirewallPolicy = value
}
/**
* @param value Is only necessary addresses or ports are permitted in? Defaults to `true`.
*/
@JvmName("cqmuihlhurleqmwg")
public suspend fun permissiveInputFirewallRules(`value`: Output) {
this.permissiveInputFirewallRules = value
}
/**
* @param value Is only necessary addresses or ports are permitted out? Defaults to `true`.
*/
@JvmName("xjqloojnnjicdfjf")
public suspend fun permissiveOutputFirewallRules(`value`: Output) {
this.permissiveOutputFirewallRules = value
}
/**
* @param value Is high level permissions are needed for the module? Defaults to `true`.
*/
@JvmName("ufbxefntiftaloor")
public suspend fun privilegedDockerOptions(`value`: Output) {
this.privilegedDockerOptions = value
}
/**
* @param value Is any credentials shared among devices? Defaults to `true`.
*/
@JvmName("mlcwvcdcxfyjdqoa")
public suspend fun sharedCredentials(`value`: Output) {
this.sharedCredentials = value
}
/**
* @param value Does TLS cipher suite need to be updated? Defaults to `true`.
*/
@JvmName("uyekyejpgwrpfnim")
public suspend fun vulnerableTlsCipherSuite(`value`: Output) {
this.vulnerableTlsCipherSuite = value
}
/**
* @param value Is Principal Authentication enabled for the ACR repository? Defaults to `true`.
*/
@JvmName("alrpaudlkuecuwbu")
public suspend fun acrAuthentication(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.acrAuthentication = mapped
}
/**
* @param value Is Agent send underutilized messages enabled? Defaults to `true`.
*/
@JvmName("gayhtirwgsomsqjq")
public suspend fun agentSendUnutilizedMsg(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.agentSendUnutilizedMsg = mapped
}
/**
* @param value Is Security related system configuration issues identified? Defaults to `true`.
*/
@JvmName("nxjvppcdunbciljg")
public suspend fun baseline(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.baseline = mapped
}
/**
* @param value Is IoT Edge Hub memory optimized? Defaults to `true`.
*/
@JvmName("korukdevrlbineec")
public suspend fun edgeHubMemOptimize(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.edgeHubMemOptimize = mapped
}
/**
* @param value Is logging configured for IoT Edge module? Defaults to `true`.
*/
@JvmName("iugkdtruqcggylvp")
public suspend fun edgeLoggingOption(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.edgeLoggingOption = mapped
}
/**
* @param value Is inconsistent module settings enabled for SecurityGroup? Defaults to `true`.
*/
@JvmName("napxviucvuqgpwro")
public suspend fun inconsistentModuleSettings(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.inconsistentModuleSettings = mapped
}
/**
* @param value is Azure IoT Security agent installed? Defaults to `true`.
*/
@JvmName("ldkjyngrcejfguvy")
public suspend fun installAgent(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.installAgent = mapped
}
/**
* @param value Is Default IP filter policy denied? Defaults to `true`.
*/
@JvmName("irixvwuoihvnglhn")
public suspend fun ipFilterDenyAll(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.ipFilterDenyAll = mapped
}
/**
* @param value Is IP filter rule source allowable IP range too large? Defaults to `true`.
*/
@JvmName("gumdtxaebkpjaxof")
public suspend fun ipFilterPermissiveRule(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.ipFilterPermissiveRule = mapped
}
/**
* @param value Is any ports open on the device? Defaults to `true`.
*/
@JvmName("jmvpfprpbtajfdjy")
public suspend fun openPorts(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.openPorts = mapped
}
/**
* @param value Does firewall policy exist which allow necessary communication to/from the device? Defaults to `true`.
*/
@JvmName("dqcatbjdbxaaflsu")
public suspend fun permissiveFirewallPolicy(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.permissiveFirewallPolicy = mapped
}
/**
* @param value Is only necessary addresses or ports are permitted in? Defaults to `true`.
*/
@JvmName("bptvqusjqsgfrkuk")
public suspend fun permissiveInputFirewallRules(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.permissiveInputFirewallRules = mapped
}
/**
* @param value Is only necessary addresses or ports are permitted out? Defaults to `true`.
*/
@JvmName("wcbblqaqmsindxbu")
public suspend fun permissiveOutputFirewallRules(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.permissiveOutputFirewallRules = mapped
}
/**
* @param value Is high level permissions are needed for the module? Defaults to `true`.
*/
@JvmName("orfgdnygoclvwhwk")
public suspend fun privilegedDockerOptions(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.privilegedDockerOptions = mapped
}
/**
* @param value Is any credentials shared among devices? Defaults to `true`.
*/
@JvmName("kiwukvylkovqsofh")
public suspend fun sharedCredentials(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.sharedCredentials = mapped
}
/**
* @param value Does TLS cipher suite need to be updated? Defaults to `true`.
*/
@JvmName("wnjcaqdtqnfbxfat")
public suspend fun vulnerableTlsCipherSuite(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.vulnerableTlsCipherSuite = mapped
}
internal fun build(): SecuritySolutionRecommendationsEnabledArgs =
SecuritySolutionRecommendationsEnabledArgs(
acrAuthentication = acrAuthentication,
agentSendUnutilizedMsg = agentSendUnutilizedMsg,
baseline = baseline,
edgeHubMemOptimize = edgeHubMemOptimize,
edgeLoggingOption = edgeLoggingOption,
inconsistentModuleSettings = inconsistentModuleSettings,
installAgent = installAgent,
ipFilterDenyAll = ipFilterDenyAll,
ipFilterPermissiveRule = ipFilterPermissiveRule,
openPorts = openPorts,
permissiveFirewallPolicy = permissiveFirewallPolicy,
permissiveInputFirewallRules = permissiveInputFirewallRules,
permissiveOutputFirewallRules = permissiveOutputFirewallRules,
privilegedDockerOptions = privilegedDockerOptions,
sharedCredentials = sharedCredentials,
vulnerableTlsCipherSuite = vulnerableTlsCipherSuite,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy