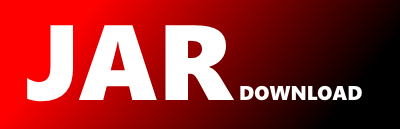
com.pulumi.azure.keyvault.kotlin.ManagedHardwareSecurityModuleKeyArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-kotlin Show documentation
Show all versions of pulumi-azure-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azure.keyvault.kotlin
import com.pulumi.azure.keyvault.ManagedHardwareSecurityModuleKeyArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Int
import kotlin.Pair
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
import kotlin.collections.Map
import kotlin.jvm.JvmName
/**
* Manages a Key Vault Managed Hardware Security Module Key.
* > **Note:** The Azure Provider includes a Feature Toggle which will purge a Key Vault Managed Hardware Security Module Key resource on destroy, rather than the default soft-delete. See `purge_soft_deleted_hardware_security_modules_on_destroy` for more information.
*
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azure.core.CoreFunctions;
* import com.pulumi.azure.keyvault.ManagedHardwareSecurityModule;
* import com.pulumi.azure.keyvault.ManagedHardwareSecurityModuleArgs;
* import com.pulumi.azure.keyvault.ManagedHardwareSecurityModuleRoleAssignment;
* import com.pulumi.azure.keyvault.ManagedHardwareSecurityModuleRoleAssignmentArgs;
* import com.pulumi.azure.keyvault.ManagedHardwareSecurityModuleKey;
* import com.pulumi.azure.keyvault.ManagedHardwareSecurityModuleKeyArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* final var current = CoreFunctions.getClientConfig();
* var example = new ManagedHardwareSecurityModule("example", ManagedHardwareSecurityModuleArgs.builder()
* .name("example")
* .resourceGroupName(exampleAzurermResourceGroup.name())
* .location(exampleAzurermResourceGroup.location())
* .skuName("Standard_B1")
* .tenantId(current.applyValue(getClientConfigResult -> getClientConfigResult.tenantId()))
* .adminObjectIds(current.applyValue(getClientConfigResult -> getClientConfigResult.objectId()))
* .purgeProtectionEnabled(false)
* .activeConfig(%!v(PANIC=Format method: runtime error: invalid memory address or nil pointer dereference))
* .build());
* // this gives your service principal the HSM Crypto User role which lets you create and destroy hsm keys
* var hsm_crypto_user = new ManagedHardwareSecurityModuleRoleAssignment("hsm-crypto-user", ManagedHardwareSecurityModuleRoleAssignmentArgs.builder()
* .vaultBaseUrl(test.hsmUri())
* .name("1e243909-064c-6ac3-84e9-1c8bf8d6ad22")
* .scope("/keys")
* .roleDefinitionId("/Microsoft.KeyVault/providers/Microsoft.Authorization/roleDefinitions/21dbd100-6940-42c2-9190-5d6cb909625b")
* .principalId(current.applyValue(getClientConfigResult -> getClientConfigResult.objectId()))
* .build());
* // this gives your service principal the HSM Crypto Officer role which lets you purge hsm keys
* var hsm_crypto_officer = new ManagedHardwareSecurityModuleRoleAssignment("hsm-crypto-officer", ManagedHardwareSecurityModuleRoleAssignmentArgs.builder()
* .vaultBaseUrl(test.hsmUri())
* .name("1e243909-064c-6ac3-84e9-1c8bf8d6ad23")
* .scope("/keys")
* .roleDefinitionId("/Microsoft.KeyVault/providers/Microsoft.Authorization/roleDefinitions/515eb02d-2335-4d2d-92f2-b1cbdf9c3778")
* .principalId(current.applyValue(getClientConfigResult -> getClientConfigResult.objectId()))
* .build());
* var exampleManagedHardwareSecurityModuleKey = new ManagedHardwareSecurityModuleKey("exampleManagedHardwareSecurityModuleKey", ManagedHardwareSecurityModuleKeyArgs.builder()
* .name("example")
* .managedHsmId(test.id())
* .keyType("EC-HSM")
* .curve("P-521")
* .keyOpts("sign")
* .build());
* }
* }
* ```
* ```yaml
* resources:
* example:
* type: azure:keyvault:ManagedHardwareSecurityModule
* properties:
* name: example
* resourceGroupName: ${exampleAzurermResourceGroup.name}
* location: ${exampleAzurermResourceGroup.location}
* skuName: Standard_B1
* tenantId: ${current.tenantId}
* adminObjectIds:
* - ${current.objectId}
* purgeProtectionEnabled: false
* activeConfig:
* - securityDomainCertificate:
* - ${cert[0].id}
* - ${cert[1].id}
* - ${cert[2].id}
* securityDomainQuorum: 2
* # this gives your service principal the HSM Crypto User role which lets you create and destroy hsm keys
* hsm-crypto-user:
* type: azure:keyvault:ManagedHardwareSecurityModuleRoleAssignment
* properties:
* vaultBaseUrl: ${test.hsmUri}
* name: 1e243909-064c-6ac3-84e9-1c8bf8d6ad22
* scope: /keys
* roleDefinitionId: /Microsoft.KeyVault/providers/Microsoft.Authorization/roleDefinitions/21dbd100-6940-42c2-9190-5d6cb909625b
* principalId: ${current.objectId}
* # this gives your service principal the HSM Crypto Officer role which lets you purge hsm keys
* hsm-crypto-officer:
* type: azure:keyvault:ManagedHardwareSecurityModuleRoleAssignment
* properties:
* vaultBaseUrl: ${test.hsmUri}
* name: 1e243909-064c-6ac3-84e9-1c8bf8d6ad23
* scope: /keys
* roleDefinitionId: /Microsoft.KeyVault/providers/Microsoft.Authorization/roleDefinitions/515eb02d-2335-4d2d-92f2-b1cbdf9c3778
* principalId: ${current.objectId}
* exampleManagedHardwareSecurityModuleKey:
* type: azure:keyvault:ManagedHardwareSecurityModuleKey
* name: example
* properties:
* name: example
* managedHsmId: ${test.id}
* keyType: EC-HSM
* curve: P-521
* keyOpts:
* - sign
* variables:
* current:
* fn::invoke:
* Function: azure:core:getClientConfig
* Arguments: {}
* ```
*
* ## Import
* Key Vault Managed Hardware Security Module Key can be imported using the `resource id`, e.g.
* ```sh
* $ pulumi import azure:keyvault/managedHardwareSecurityModuleKey:ManagedHardwareSecurityModuleKey example https://exampleHSM.managedhsm.azure.net/keys/exampleKey
* ```
* @property curve Specifies the curve to use when creating an `EC-HSM` key. Possible values are `P-256`, `P-256K`, `P-384`, and `P-521`. This field is required if `key_type` is `EC-HSM`. Changing this forces a new resource to be created.
* @property expirationDate Expiration UTC datetime (Y-m-d'T'H:M:S'Z'). When this parameter gets changed on reruns, if newer date is ahead of current date, an update is performed. If the newer date is before the current date, resource will be force created.
* @property keyOpts A list of JSON web key operations. Possible values include: `decrypt`, `encrypt`, `sign`, `unwrapKey`, `verify` and `wrapKey`. Please note these values are case-sensitive.
* @property keySize Specifies the Size of the RSA key to create in bytes. For example, 1024 or 2048. *Note*: This field is required if `key_type` is `RSA-HSM`. Changing this forces a new resource to be created.
* @property keyType Specifies the Key Type to use for this Key Vault Managed Hardware Security Module Key. Possible values are `EC-HSM` and `RSA-HSM`. Changing this forces a new resource to be created.
* @property managedHsmId Specifies the ID of the Key Vault Managed Hardware Security Module that they key will be owned by. Changing this forces a new resource to be created.
* @property name Specifies the name of the Key Vault Managed Hardware Security Module Key. Changing this forces a new resource to be created.
* @property notBeforeDate Key not usable before the provided UTC datetime (Y-m-d'T'H:M:S'Z').
* > **Note:** Once `expiration_date` is set, it's not possible to unset the key even if it is deleted & recreated as underlying Azure API uses the restore of the purged key.
* @property tags A mapping of tags to assign to the resource.
*/
public data class ManagedHardwareSecurityModuleKeyArgs(
public val curve: Output? = null,
public val expirationDate: Output? = null,
public val keyOpts: Output>? = null,
public val keySize: Output? = null,
public val keyType: Output? = null,
public val managedHsmId: Output? = null,
public val name: Output? = null,
public val notBeforeDate: Output? = null,
public val tags: Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy