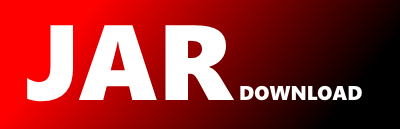
com.pulumi.azure.keyvault.kotlin.inputs.CertifiateCertificatePolicyArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-kotlin Show documentation
Show all versions of pulumi-azure-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azure.keyvault.kotlin.inputs
import com.pulumi.azure.keyvault.inputs.CertifiateCertificatePolicyArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
*
* @property issuerParameters A `issuer_parameters` block as defined below.
* @property keyProperties A `key_properties` block as defined below.
* @property lifetimeActions A `lifetime_action` block as defined below.
* @property secretProperties A `secret_properties` block as defined below.
* @property x509CertificateProperties A `x509_certificate_properties` block as defined below. Required when `certificate` block is not specified.
*/
public data class CertifiateCertificatePolicyArgs(
public val issuerParameters: Output,
public val keyProperties: Output,
public val lifetimeActions: Output>? = null,
public val secretProperties: Output,
public val x509CertificateProperties:
Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azure.keyvault.inputs.CertifiateCertificatePolicyArgs =
com.pulumi.azure.keyvault.inputs.CertifiateCertificatePolicyArgs.builder()
.issuerParameters(issuerParameters.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.keyProperties(keyProperties.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.lifetimeActions(
lifetimeActions?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
)
.secretProperties(secretProperties.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.x509CertificateProperties(
x509CertificateProperties?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
).build()
}
/**
* Builder for [CertifiateCertificatePolicyArgs].
*/
@PulumiTagMarker
public class CertifiateCertificatePolicyArgsBuilder internal constructor() {
private var issuerParameters: Output? = null
private var keyProperties: Output? = null
private var lifetimeActions: Output>? = null
private var secretProperties: Output? = null
private var x509CertificateProperties:
Output? = null
/**
* @param value A `issuer_parameters` block as defined below.
*/
@JvmName("wbitmpjxqwlrxmmt")
public suspend
fun issuerParameters(`value`: Output) {
this.issuerParameters = value
}
/**
* @param value A `key_properties` block as defined below.
*/
@JvmName("jhfoaoabvqxgkxpp")
public suspend fun keyProperties(`value`: Output) {
this.keyProperties = value
}
/**
* @param value A `lifetime_action` block as defined below.
*/
@JvmName("hmiclwvabhvphgqg")
public suspend
fun lifetimeActions(`value`: Output>) {
this.lifetimeActions = value
}
@JvmName("wslgwhojqgcoinhd")
public suspend fun lifetimeActions(
vararg
values: Output,
) {
this.lifetimeActions = Output.all(values.asList())
}
/**
* @param values A `lifetime_action` block as defined below.
*/
@JvmName("vwaxfrxhydrrevnq")
public suspend
fun lifetimeActions(values: List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy