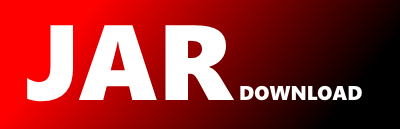
com.pulumi.azure.keyvault.kotlin.inputs.GetEncryptedValuePlainArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-kotlin Show documentation
Show all versions of pulumi-azure-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azure.keyvault.kotlin.inputs
import com.pulumi.azure.keyvault.inputs.GetEncryptedValuePlainArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
* A collection of arguments for invoking getEncryptedValue.
* @property algorithm The Algorithm which should be used to Decrypt/Encrypt this Value. Possible values are `RSA1_5`, `RSA-OAEP` and `RSA-OAEP-256`.
* @property encryptedData The Base64 URL Encoded Encrypted Data which should be decrypted into `plain_text_value`.
* @property keyVaultKeyId The ID of the Key Vault Key which should be used to Decrypt/Encrypt this Value.
* @property plainTextValue The plain-text value which should be Encrypted into `encrypted_data`.
* > **Note:** One of either `encrypted_data` or `plain_text_value` must be specified and is used to populate the encrypted/decrypted value for the other field.
*/
public data class GetEncryptedValuePlainArgs(
public val algorithm: String,
public val encryptedData: String? = null,
public val keyVaultKeyId: String,
public val plainTextValue: String? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azure.keyvault.inputs.GetEncryptedValuePlainArgs =
com.pulumi.azure.keyvault.inputs.GetEncryptedValuePlainArgs.builder()
.algorithm(algorithm.let({ args0 -> args0 }))
.encryptedData(encryptedData?.let({ args0 -> args0 }))
.keyVaultKeyId(keyVaultKeyId.let({ args0 -> args0 }))
.plainTextValue(plainTextValue?.let({ args0 -> args0 })).build()
}
/**
* Builder for [GetEncryptedValuePlainArgs].
*/
@PulumiTagMarker
public class GetEncryptedValuePlainArgsBuilder internal constructor() {
private var algorithm: String? = null
private var encryptedData: String? = null
private var keyVaultKeyId: String? = null
private var plainTextValue: String? = null
/**
* @param value The Algorithm which should be used to Decrypt/Encrypt this Value. Possible values are `RSA1_5`, `RSA-OAEP` and `RSA-OAEP-256`.
*/
@JvmName("gdqjwvfnfxfbwmvk")
public suspend fun algorithm(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> args0 })
this.algorithm = mapped
}
/**
* @param value The Base64 URL Encoded Encrypted Data which should be decrypted into `plain_text_value`.
*/
@JvmName("ygjayjdctukbxjoq")
public suspend fun encryptedData(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> args0 })
this.encryptedData = mapped
}
/**
* @param value The ID of the Key Vault Key which should be used to Decrypt/Encrypt this Value.
*/
@JvmName("hcrwugtcixknafnd")
public suspend fun keyVaultKeyId(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> args0 })
this.keyVaultKeyId = mapped
}
/**
* @param value The plain-text value which should be Encrypted into `encrypted_data`.
* > **Note:** One of either `encrypted_data` or `plain_text_value` must be specified and is used to populate the encrypted/decrypted value for the other field.
*/
@JvmName("pclnagsyyoesbsea")
public suspend fun plainTextValue(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> args0 })
this.plainTextValue = mapped
}
internal fun build(): GetEncryptedValuePlainArgs = GetEncryptedValuePlainArgs(
algorithm = algorithm ?: throw PulumiNullFieldException("algorithm"),
encryptedData = encryptedData,
keyVaultKeyId = keyVaultKeyId ?: throw PulumiNullFieldException("keyVaultKeyId"),
plainTextValue = plainTextValue,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy