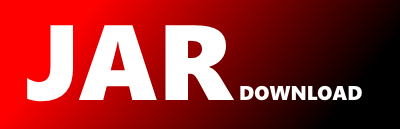
com.pulumi.azure.kotlin.inputs.ProviderFeaturesKeyVaultArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-kotlin Show documentation
Show all versions of pulumi-azure-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azure.kotlin.inputs
import com.pulumi.azure.inputs.ProviderFeaturesKeyVaultArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Boolean
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
*
* @property purgeSoftDeleteOnDestroy When enabled soft-deleted `azure.keyvault.KeyVault` resources will be permanently deleted (e.g purged), when destroyed
* @property purgeSoftDeletedCertificatesOnDestroy When enabled soft-deleted `azure.keyvault.Certificate` resources will be permanently deleted (e.g purged), when destroyed
* @property purgeSoftDeletedHardwareSecurityModuleKeysOnDestroy When enabled soft-deleted `azure.keyvault.ManagedHardwareSecurityModuleKey` resources will be permanently deleted (e.g purged), when destroyed
* @property purgeSoftDeletedHardwareSecurityModulesOnDestroy When enabled soft-deleted `azure.keyvault.ManagedHardwareSecurityModule` resources will be permanently deleted (e.g purged), when destroyed
* @property purgeSoftDeletedKeysOnDestroy When enabled soft-deleted `azure.keyvault.Key` resources will be permanently deleted (e.g purged), when destroyed
* @property purgeSoftDeletedSecretsOnDestroy When enabled soft-deleted `azure.keyvault.Secret` resources will be permanently deleted (e.g purged), when destroyed
* @property recoverSoftDeletedCertificates When enabled soft-deleted `azure.keyvault.Certificate` resources will be restored, instead of creating new ones
* @property recoverSoftDeletedHardwareSecurityModuleKeys When enabled soft-deleted `azure.keyvault.ManagedHardwareSecurityModuleKey` resources will be restored, instead of creating new ones
* @property recoverSoftDeletedKeyVaults When enabled soft-deleted `azure.keyvault.KeyVault` resources will be restored, instead of creating new ones
* @property recoverSoftDeletedKeys When enabled soft-deleted `azure.keyvault.Key` resources will be restored, instead of creating new ones
* @property recoverSoftDeletedSecrets When enabled soft-deleted `azure.keyvault.Secret` resources will be restored, instead of creating new ones
*/
public data class ProviderFeaturesKeyVaultArgs(
public val purgeSoftDeleteOnDestroy: Output? = null,
public val purgeSoftDeletedCertificatesOnDestroy: Output? = null,
public val purgeSoftDeletedHardwareSecurityModuleKeysOnDestroy: Output? = null,
public val purgeSoftDeletedHardwareSecurityModulesOnDestroy: Output? = null,
public val purgeSoftDeletedKeysOnDestroy: Output? = null,
public val purgeSoftDeletedSecretsOnDestroy: Output? = null,
public val recoverSoftDeletedCertificates: Output? = null,
public val recoverSoftDeletedHardwareSecurityModuleKeys: Output? = null,
public val recoverSoftDeletedKeyVaults: Output? = null,
public val recoverSoftDeletedKeys: Output? = null,
public val recoverSoftDeletedSecrets: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azure.inputs.ProviderFeaturesKeyVaultArgs =
com.pulumi.azure.inputs.ProviderFeaturesKeyVaultArgs.builder()
.purgeSoftDeleteOnDestroy(purgeSoftDeleteOnDestroy?.applyValue({ args0 -> args0 }))
.purgeSoftDeletedCertificatesOnDestroy(
purgeSoftDeletedCertificatesOnDestroy?.applyValue({ args0 ->
args0
}),
)
.purgeSoftDeletedHardwareSecurityModuleKeysOnDestroy(
purgeSoftDeletedHardwareSecurityModuleKeysOnDestroy?.applyValue({ args0 ->
args0
}),
)
.purgeSoftDeletedHardwareSecurityModulesOnDestroy(
purgeSoftDeletedHardwareSecurityModulesOnDestroy?.applyValue({ args0 ->
args0
}),
)
.purgeSoftDeletedKeysOnDestroy(purgeSoftDeletedKeysOnDestroy?.applyValue({ args0 -> args0 }))
.purgeSoftDeletedSecretsOnDestroy(purgeSoftDeletedSecretsOnDestroy?.applyValue({ args0 -> args0 }))
.recoverSoftDeletedCertificates(recoverSoftDeletedCertificates?.applyValue({ args0 -> args0 }))
.recoverSoftDeletedHardwareSecurityModuleKeys(
recoverSoftDeletedHardwareSecurityModuleKeys?.applyValue({ args0 ->
args0
}),
)
.recoverSoftDeletedKeyVaults(recoverSoftDeletedKeyVaults?.applyValue({ args0 -> args0 }))
.recoverSoftDeletedKeys(recoverSoftDeletedKeys?.applyValue({ args0 -> args0 }))
.recoverSoftDeletedSecrets(recoverSoftDeletedSecrets?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [ProviderFeaturesKeyVaultArgs].
*/
@PulumiTagMarker
public class ProviderFeaturesKeyVaultArgsBuilder internal constructor() {
private var purgeSoftDeleteOnDestroy: Output? = null
private var purgeSoftDeletedCertificatesOnDestroy: Output? = null
private var purgeSoftDeletedHardwareSecurityModuleKeysOnDestroy: Output? = null
private var purgeSoftDeletedHardwareSecurityModulesOnDestroy: Output? = null
private var purgeSoftDeletedKeysOnDestroy: Output? = null
private var purgeSoftDeletedSecretsOnDestroy: Output? = null
private var recoverSoftDeletedCertificates: Output? = null
private var recoverSoftDeletedHardwareSecurityModuleKeys: Output? = null
private var recoverSoftDeletedKeyVaults: Output? = null
private var recoverSoftDeletedKeys: Output? = null
private var recoverSoftDeletedSecrets: Output? = null
/**
* @param value When enabled soft-deleted `azure.keyvault.KeyVault` resources will be permanently deleted (e.g purged), when destroyed
*/
@JvmName("ibuqnxrcgabjbixo")
public suspend fun purgeSoftDeleteOnDestroy(`value`: Output) {
this.purgeSoftDeleteOnDestroy = value
}
/**
* @param value When enabled soft-deleted `azure.keyvault.Certificate` resources will be permanently deleted (e.g purged), when destroyed
*/
@JvmName("tktbemhkdulhpqmq")
public suspend fun purgeSoftDeletedCertificatesOnDestroy(`value`: Output) {
this.purgeSoftDeletedCertificatesOnDestroy = value
}
/**
* @param value When enabled soft-deleted `azure.keyvault.ManagedHardwareSecurityModuleKey` resources will be permanently deleted (e.g purged), when destroyed
*/
@JvmName("loerxtjpetdpwuba")
public suspend fun purgeSoftDeletedHardwareSecurityModuleKeysOnDestroy(`value`: Output) {
this.purgeSoftDeletedHardwareSecurityModuleKeysOnDestroy = value
}
/**
* @param value When enabled soft-deleted `azure.keyvault.ManagedHardwareSecurityModule` resources will be permanently deleted (e.g purged), when destroyed
*/
@JvmName("sgdknmkohmqkqgmx")
public suspend fun purgeSoftDeletedHardwareSecurityModulesOnDestroy(`value`: Output) {
this.purgeSoftDeletedHardwareSecurityModulesOnDestroy = value
}
/**
* @param value When enabled soft-deleted `azure.keyvault.Key` resources will be permanently deleted (e.g purged), when destroyed
*/
@JvmName("richufjejqgjnmja")
public suspend fun purgeSoftDeletedKeysOnDestroy(`value`: Output) {
this.purgeSoftDeletedKeysOnDestroy = value
}
/**
* @param value When enabled soft-deleted `azure.keyvault.Secret` resources will be permanently deleted (e.g purged), when destroyed
*/
@JvmName("dtkljeokarjgnymv")
public suspend fun purgeSoftDeletedSecretsOnDestroy(`value`: Output) {
this.purgeSoftDeletedSecretsOnDestroy = value
}
/**
* @param value When enabled soft-deleted `azure.keyvault.Certificate` resources will be restored, instead of creating new ones
*/
@JvmName("qtrqqgqijwccvssk")
public suspend fun recoverSoftDeletedCertificates(`value`: Output) {
this.recoverSoftDeletedCertificates = value
}
/**
* @param value When enabled soft-deleted `azure.keyvault.ManagedHardwareSecurityModuleKey` resources will be restored, instead of creating new ones
*/
@JvmName("hhmrvhgmvossewud")
public suspend fun recoverSoftDeletedHardwareSecurityModuleKeys(`value`: Output) {
this.recoverSoftDeletedHardwareSecurityModuleKeys = value
}
/**
* @param value When enabled soft-deleted `azure.keyvault.KeyVault` resources will be restored, instead of creating new ones
*/
@JvmName("ufefvjsbgumjlepd")
public suspend fun recoverSoftDeletedKeyVaults(`value`: Output) {
this.recoverSoftDeletedKeyVaults = value
}
/**
* @param value When enabled soft-deleted `azure.keyvault.Key` resources will be restored, instead of creating new ones
*/
@JvmName("ugojmykjjsmrsmuy")
public suspend fun recoverSoftDeletedKeys(`value`: Output) {
this.recoverSoftDeletedKeys = value
}
/**
* @param value When enabled soft-deleted `azure.keyvault.Secret` resources will be restored, instead of creating new ones
*/
@JvmName("eonyudnumlykwypr")
public suspend fun recoverSoftDeletedSecrets(`value`: Output) {
this.recoverSoftDeletedSecrets = value
}
/**
* @param value When enabled soft-deleted `azure.keyvault.KeyVault` resources will be permanently deleted (e.g purged), when destroyed
*/
@JvmName("iwjrjchwevoojnbf")
public suspend fun purgeSoftDeleteOnDestroy(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.purgeSoftDeleteOnDestroy = mapped
}
/**
* @param value When enabled soft-deleted `azure.keyvault.Certificate` resources will be permanently deleted (e.g purged), when destroyed
*/
@JvmName("pxyllqveyjivkdny")
public suspend fun purgeSoftDeletedCertificatesOnDestroy(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.purgeSoftDeletedCertificatesOnDestroy = mapped
}
/**
* @param value When enabled soft-deleted `azure.keyvault.ManagedHardwareSecurityModuleKey` resources will be permanently deleted (e.g purged), when destroyed
*/
@JvmName("cbhwtqddsakhulxd")
public suspend fun purgeSoftDeletedHardwareSecurityModuleKeysOnDestroy(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.purgeSoftDeletedHardwareSecurityModuleKeysOnDestroy = mapped
}
/**
* @param value When enabled soft-deleted `azure.keyvault.ManagedHardwareSecurityModule` resources will be permanently deleted (e.g purged), when destroyed
*/
@JvmName("uyshkhmwirlsypwh")
public suspend fun purgeSoftDeletedHardwareSecurityModulesOnDestroy(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.purgeSoftDeletedHardwareSecurityModulesOnDestroy = mapped
}
/**
* @param value When enabled soft-deleted `azure.keyvault.Key` resources will be permanently deleted (e.g purged), when destroyed
*/
@JvmName("bmjmdinlmturodfx")
public suspend fun purgeSoftDeletedKeysOnDestroy(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.purgeSoftDeletedKeysOnDestroy = mapped
}
/**
* @param value When enabled soft-deleted `azure.keyvault.Secret` resources will be permanently deleted (e.g purged), when destroyed
*/
@JvmName("qjgoqqfbrmlavvdq")
public suspend fun purgeSoftDeletedSecretsOnDestroy(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.purgeSoftDeletedSecretsOnDestroy = mapped
}
/**
* @param value When enabled soft-deleted `azure.keyvault.Certificate` resources will be restored, instead of creating new ones
*/
@JvmName("vhbntwqpyvwemrsj")
public suspend fun recoverSoftDeletedCertificates(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.recoverSoftDeletedCertificates = mapped
}
/**
* @param value When enabled soft-deleted `azure.keyvault.ManagedHardwareSecurityModuleKey` resources will be restored, instead of creating new ones
*/
@JvmName("wxgbousvuebytgcu")
public suspend fun recoverSoftDeletedHardwareSecurityModuleKeys(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.recoverSoftDeletedHardwareSecurityModuleKeys = mapped
}
/**
* @param value When enabled soft-deleted `azure.keyvault.KeyVault` resources will be restored, instead of creating new ones
*/
@JvmName("fruthkkeejyxewds")
public suspend fun recoverSoftDeletedKeyVaults(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.recoverSoftDeletedKeyVaults = mapped
}
/**
* @param value When enabled soft-deleted `azure.keyvault.Key` resources will be restored, instead of creating new ones
*/
@JvmName("lugdamrymvvpdfhu")
public suspend fun recoverSoftDeletedKeys(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.recoverSoftDeletedKeys = mapped
}
/**
* @param value When enabled soft-deleted `azure.keyvault.Secret` resources will be restored, instead of creating new ones
*/
@JvmName("qjqbgkqivhswcnak")
public suspend fun recoverSoftDeletedSecrets(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.recoverSoftDeletedSecrets = mapped
}
internal fun build(): ProviderFeaturesKeyVaultArgs = ProviderFeaturesKeyVaultArgs(
purgeSoftDeleteOnDestroy = purgeSoftDeleteOnDestroy,
purgeSoftDeletedCertificatesOnDestroy = purgeSoftDeletedCertificatesOnDestroy,
purgeSoftDeletedHardwareSecurityModuleKeysOnDestroy = purgeSoftDeletedHardwareSecurityModuleKeysOnDestroy,
purgeSoftDeletedHardwareSecurityModulesOnDestroy = purgeSoftDeletedHardwareSecurityModulesOnDestroy,
purgeSoftDeletedKeysOnDestroy = purgeSoftDeletedKeysOnDestroy,
purgeSoftDeletedSecretsOnDestroy = purgeSoftDeletedSecretsOnDestroy,
recoverSoftDeletedCertificates = recoverSoftDeletedCertificates,
recoverSoftDeletedHardwareSecurityModuleKeys = recoverSoftDeletedHardwareSecurityModuleKeys,
recoverSoftDeletedKeyVaults = recoverSoftDeletedKeyVaults,
recoverSoftDeletedKeys = recoverSoftDeletedKeys,
recoverSoftDeletedSecrets = recoverSoftDeletedSecrets,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy