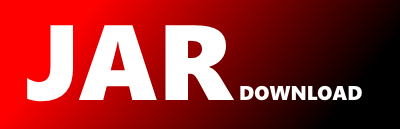
com.pulumi.azure.kusto.kotlin.ClusterManagedPrivateEndpointArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-kotlin Show documentation
Show all versions of pulumi-azure-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azure.kusto.kotlin
import com.pulumi.azure.kusto.ClusterManagedPrivateEndpointArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
* Manages a Managed Private Endpoint for a Kusto Cluster.
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as azure from "@pulumi/azure";
* const current = azure.core.getClientConfig({});
* const example = new azure.core.ResourceGroup("example", {
* name: "example-resources",
* location: "West Europe",
* });
* const exampleCluster = new azure.kusto.Cluster("example", {
* name: "examplekc",
* location: example.location,
* resourceGroupName: example.name,
* sku: {
* name: "Dev(No SLA)_Standard_D11_v2",
* capacity: 1,
* },
* });
* const exampleAccount = new azure.storage.Account("example", {
* name: "examplesa",
* resourceGroupName: example.name,
* location: example.location,
* accountTier: "Standard",
* accountReplicationType: "LRS",
* });
* const exampleClusterManagedPrivateEndpoint = new azure.kusto.ClusterManagedPrivateEndpoint("example", {
* name: "examplempe",
* resourceGroupName: example.name,
* clusterName: exampleCluster.name,
* privateLinkResourceId: exampleAccount.id,
* privateLinkResourceRegion: exampleAccount.location,
* groupId: "blob",
* requestMessage: "Please Approve",
* });
* ```
* ```python
* import pulumi
* import pulumi_azure as azure
* current = azure.core.get_client_config()
* example = azure.core.ResourceGroup("example",
* name="example-resources",
* location="West Europe")
* example_cluster = azure.kusto.Cluster("example",
* name="examplekc",
* location=example.location,
* resource_group_name=example.name,
* sku=azure.kusto.ClusterSkuArgs(
* name="Dev(No SLA)_Standard_D11_v2",
* capacity=1,
* ))
* example_account = azure.storage.Account("example",
* name="examplesa",
* resource_group_name=example.name,
* location=example.location,
* account_tier="Standard",
* account_replication_type="LRS")
* example_cluster_managed_private_endpoint = azure.kusto.ClusterManagedPrivateEndpoint("example",
* name="examplempe",
* resource_group_name=example.name,
* cluster_name=example_cluster.name,
* private_link_resource_id=example_account.id,
* private_link_resource_region=example_account.location,
* group_id="blob",
* request_message="Please Approve")
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Azure = Pulumi.Azure;
* return await Deployment.RunAsync(() =>
* {
* var current = Azure.Core.GetClientConfig.Invoke();
* var example = new Azure.Core.ResourceGroup("example", new()
* {
* Name = "example-resources",
* Location = "West Europe",
* });
* var exampleCluster = new Azure.Kusto.Cluster("example", new()
* {
* Name = "examplekc",
* Location = example.Location,
* ResourceGroupName = example.Name,
* Sku = new Azure.Kusto.Inputs.ClusterSkuArgs
* {
* Name = "Dev(No SLA)_Standard_D11_v2",
* Capacity = 1,
* },
* });
* var exampleAccount = new Azure.Storage.Account("example", new()
* {
* Name = "examplesa",
* ResourceGroupName = example.Name,
* Location = example.Location,
* AccountTier = "Standard",
* AccountReplicationType = "LRS",
* });
* var exampleClusterManagedPrivateEndpoint = new Azure.Kusto.ClusterManagedPrivateEndpoint("example", new()
* {
* Name = "examplempe",
* ResourceGroupName = example.Name,
* ClusterName = exampleCluster.Name,
* PrivateLinkResourceId = exampleAccount.Id,
* PrivateLinkResourceRegion = exampleAccount.Location,
* GroupId = "blob",
* RequestMessage = "Please Approve",
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-azure/sdk/v5/go/azure/core"
* "github.com/pulumi/pulumi-azure/sdk/v5/go/azure/kusto"
* "github.com/pulumi/pulumi-azure/sdk/v5/go/azure/storage"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := core.GetClientConfig(ctx, nil, nil)
* if err != nil {
* return err
* }
* example, err := core.NewResourceGroup(ctx, "example", &core.ResourceGroupArgs{
* Name: pulumi.String("example-resources"),
* Location: pulumi.String("West Europe"),
* })
* if err != nil {
* return err
* }
* exampleCluster, err := kusto.NewCluster(ctx, "example", &kusto.ClusterArgs{
* Name: pulumi.String("examplekc"),
* Location: example.Location,
* ResourceGroupName: example.Name,
* Sku: &kusto.ClusterSkuArgs{
* Name: pulumi.String("Dev(No SLA)_Standard_D11_v2"),
* Capacity: pulumi.Int(1),
* },
* })
* if err != nil {
* return err
* }
* exampleAccount, err := storage.NewAccount(ctx, "example", &storage.AccountArgs{
* Name: pulumi.String("examplesa"),
* ResourceGroupName: example.Name,
* Location: example.Location,
* AccountTier: pulumi.String("Standard"),
* AccountReplicationType: pulumi.String("LRS"),
* })
* if err != nil {
* return err
* }
* _, err = kusto.NewClusterManagedPrivateEndpoint(ctx, "example", &kusto.ClusterManagedPrivateEndpointArgs{
* Name: pulumi.String("examplempe"),
* ResourceGroupName: example.Name,
* ClusterName: exampleCluster.Name,
* PrivateLinkResourceId: exampleAccount.ID(),
* PrivateLinkResourceRegion: exampleAccount.Location,
* GroupId: pulumi.String("blob"),
* RequestMessage: pulumi.String("Please Approve"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azure.core.CoreFunctions;
* import com.pulumi.azure.core.ResourceGroup;
* import com.pulumi.azure.core.ResourceGroupArgs;
* import com.pulumi.azure.kusto.Cluster;
* import com.pulumi.azure.kusto.ClusterArgs;
* import com.pulumi.azure.kusto.inputs.ClusterSkuArgs;
* import com.pulumi.azure.storage.Account;
* import com.pulumi.azure.storage.AccountArgs;
* import com.pulumi.azure.kusto.ClusterManagedPrivateEndpoint;
* import com.pulumi.azure.kusto.ClusterManagedPrivateEndpointArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* final var current = CoreFunctions.getClientConfig();
* var example = new ResourceGroup("example", ResourceGroupArgs.builder()
* .name("example-resources")
* .location("West Europe")
* .build());
* var exampleCluster = new Cluster("exampleCluster", ClusterArgs.builder()
* .name("examplekc")
* .location(example.location())
* .resourceGroupName(example.name())
* .sku(ClusterSkuArgs.builder()
* .name("Dev(No SLA)_Standard_D11_v2")
* .capacity(1)
* .build())
* .build());
* var exampleAccount = new Account("exampleAccount", AccountArgs.builder()
* .name("examplesa")
* .resourceGroupName(example.name())
* .location(example.location())
* .accountTier("Standard")
* .accountReplicationType("LRS")
* .build());
* var exampleClusterManagedPrivateEndpoint = new ClusterManagedPrivateEndpoint("exampleClusterManagedPrivateEndpoint", ClusterManagedPrivateEndpointArgs.builder()
* .name("examplempe")
* .resourceGroupName(example.name())
* .clusterName(exampleCluster.name())
* .privateLinkResourceId(exampleAccount.id())
* .privateLinkResourceRegion(exampleAccount.location())
* .groupId("blob")
* .requestMessage("Please Approve")
* .build());
* }
* }
* ```
* ```yaml
* resources:
* example:
* type: azure:core:ResourceGroup
* properties:
* name: example-resources
* location: West Europe
* exampleCluster:
* type: azure:kusto:Cluster
* name: example
* properties:
* name: examplekc
* location: ${example.location}
* resourceGroupName: ${example.name}
* sku:
* name: Dev(No SLA)_Standard_D11_v2
* capacity: 1
* exampleAccount:
* type: azure:storage:Account
* name: example
* properties:
* name: examplesa
* resourceGroupName: ${example.name}
* location: ${example.location}
* accountTier: Standard
* accountReplicationType: LRS
* exampleClusterManagedPrivateEndpoint:
* type: azure:kusto:ClusterManagedPrivateEndpoint
* name: example
* properties:
* name: examplempe
* resourceGroupName: ${example.name}
* clusterName: ${exampleCluster.name}
* privateLinkResourceId: ${exampleAccount.id}
* privateLinkResourceRegion: ${exampleAccount.location}
* groupId: blob
* requestMessage: Please Approve
* variables:
* current:
* fn::invoke:
* Function: azure:core:getClientConfig
* Arguments: {}
* ```
*
* ## Import
* Managed Private Endpoint for a Kusto Cluster can be imported using the `resource id`, e.g.
* ```sh
* $ pulumi import azure:kusto/clusterManagedPrivateEndpoint:ClusterManagedPrivateEndpoint example /subscriptions/00000000-0000-0000-0000-000000000000/resourceGroups/group1/providers/Microsoft.Kusto/clusters/cluster1/managedPrivateEndpoints/managedPrivateEndpoint1
* ```
* @property clusterName The name of the Kusto Cluster. Changing this forces a new resource to be created.
* @property groupId The group id in which the managed private endpoint is created. Changing this forces a new resource to be created.
* @property name The name of the Managed Private Endpoints to create. Changing this forces a new resource to be created.
* @property privateLinkResourceId The ARM resource ID of the resource for which the managed private endpoint is created. Changing this forces a new resource to be created.
* @property privateLinkResourceRegion The region of the resource to which the managed private endpoint is created. Changing this forces a new resource to be created.
* @property requestMessage The user request message.
* @property resourceGroupName Specifies the Resource Group where the Kusto Cluster should exist. Changing this forces a new resource to be created.
*/
public data class ClusterManagedPrivateEndpointArgs(
public val clusterName: Output? = null,
public val groupId: Output? = null,
public val name: Output? = null,
public val privateLinkResourceId: Output? = null,
public val privateLinkResourceRegion: Output? = null,
public val requestMessage: Output? = null,
public val resourceGroupName: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azure.kusto.ClusterManagedPrivateEndpointArgs =
com.pulumi.azure.kusto.ClusterManagedPrivateEndpointArgs.builder()
.clusterName(clusterName?.applyValue({ args0 -> args0 }))
.groupId(groupId?.applyValue({ args0 -> args0 }))
.name(name?.applyValue({ args0 -> args0 }))
.privateLinkResourceId(privateLinkResourceId?.applyValue({ args0 -> args0 }))
.privateLinkResourceRegion(privateLinkResourceRegion?.applyValue({ args0 -> args0 }))
.requestMessage(requestMessage?.applyValue({ args0 -> args0 }))
.resourceGroupName(resourceGroupName?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [ClusterManagedPrivateEndpointArgs].
*/
@PulumiTagMarker
public class ClusterManagedPrivateEndpointArgsBuilder internal constructor() {
private var clusterName: Output? = null
private var groupId: Output? = null
private var name: Output? = null
private var privateLinkResourceId: Output? = null
private var privateLinkResourceRegion: Output? = null
private var requestMessage: Output? = null
private var resourceGroupName: Output? = null
/**
* @param value The name of the Kusto Cluster. Changing this forces a new resource to be created.
*/
@JvmName("xxlcajrvqadmwfvp")
public suspend fun clusterName(`value`: Output) {
this.clusterName = value
}
/**
* @param value The group id in which the managed private endpoint is created. Changing this forces a new resource to be created.
*/
@JvmName("jhxaspaioyxdirgq")
public suspend fun groupId(`value`: Output) {
this.groupId = value
}
/**
* @param value The name of the Managed Private Endpoints to create. Changing this forces a new resource to be created.
*/
@JvmName("neqcbfuttxgrxwmc")
public suspend fun name(`value`: Output) {
this.name = value
}
/**
* @param value The ARM resource ID of the resource for which the managed private endpoint is created. Changing this forces a new resource to be created.
*/
@JvmName("yeofabrvkcxluwqh")
public suspend fun privateLinkResourceId(`value`: Output) {
this.privateLinkResourceId = value
}
/**
* @param value The region of the resource to which the managed private endpoint is created. Changing this forces a new resource to be created.
*/
@JvmName("jodnyjfucvnylutg")
public suspend fun privateLinkResourceRegion(`value`: Output) {
this.privateLinkResourceRegion = value
}
/**
* @param value The user request message.
*/
@JvmName("nrmlnpiqrfkkgyxo")
public suspend fun requestMessage(`value`: Output) {
this.requestMessage = value
}
/**
* @param value Specifies the Resource Group where the Kusto Cluster should exist. Changing this forces a new resource to be created.
*/
@JvmName("lirjhssncqugudeq")
public suspend fun resourceGroupName(`value`: Output) {
this.resourceGroupName = value
}
/**
* @param value The name of the Kusto Cluster. Changing this forces a new resource to be created.
*/
@JvmName("hdescfgqqduqwynj")
public suspend fun clusterName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.clusterName = mapped
}
/**
* @param value The group id in which the managed private endpoint is created. Changing this forces a new resource to be created.
*/
@JvmName("aamoriktpiseydmo")
public suspend fun groupId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.groupId = mapped
}
/**
* @param value The name of the Managed Private Endpoints to create. Changing this forces a new resource to be created.
*/
@JvmName("hlkimrmenenjyiae")
public suspend fun name(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.name = mapped
}
/**
* @param value The ARM resource ID of the resource for which the managed private endpoint is created. Changing this forces a new resource to be created.
*/
@JvmName("gsuxjoqpevjviydl")
public suspend fun privateLinkResourceId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.privateLinkResourceId = mapped
}
/**
* @param value The region of the resource to which the managed private endpoint is created. Changing this forces a new resource to be created.
*/
@JvmName("jgadfbgknbiakgkc")
public suspend fun privateLinkResourceRegion(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.privateLinkResourceRegion = mapped
}
/**
* @param value The user request message.
*/
@JvmName("fdebmstfvdvhqnly")
public suspend fun requestMessage(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.requestMessage = mapped
}
/**
* @param value Specifies the Resource Group where the Kusto Cluster should exist. Changing this forces a new resource to be created.
*/
@JvmName("qdjckithjuupougi")
public suspend fun resourceGroupName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.resourceGroupName = mapped
}
internal fun build(): ClusterManagedPrivateEndpointArgs = ClusterManagedPrivateEndpointArgs(
clusterName = clusterName,
groupId = groupId,
name = name,
privateLinkResourceId = privateLinkResourceId,
privateLinkResourceRegion = privateLinkResourceRegion,
requestMessage = requestMessage,
resourceGroupName = resourceGroupName,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy