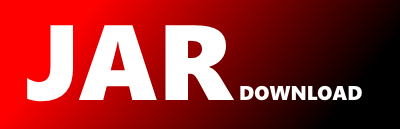
com.pulumi.azure.kusto.kotlin.CosmosdbDataConnectionArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-kotlin Show documentation
Show all versions of pulumi-azure-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azure.kusto.kotlin
import com.pulumi.azure.kusto.CosmosdbDataConnectionArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
* Manages a Kusto / Cosmos Database Data Connection.
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as azure from "@pulumi/azure";
* const current = azure.core.getClientConfig({});
* const exampleResourceGroup = new azure.core.ResourceGroup("example", {
* name: "exampleRG",
* location: "West Europe",
* });
* const builtin = azure.authorization.getRoleDefinition({
* roleDefinitionId: "fbdf93bf-df7d-467e-a4d2-9458aa1360c8",
* });
* const exampleCluster = new azure.kusto.Cluster("example", {
* name: "examplekc",
* location: exampleResourceGroup.location,
* resourceGroupName: exampleResourceGroup.name,
* sku: {
* name: "Dev(No SLA)_Standard_D11_v2",
* capacity: 1,
* },
* identity: {
* type: "SystemAssigned",
* },
* });
* const exampleAssignment = new azure.authorization.Assignment("example", {
* scope: exampleResourceGroup.id,
* roleDefinitionName: builtin.then(builtin => builtin.name),
* principalId: exampleCluster.identity.apply(identity => identity?.principalId),
* });
* const exampleAccount = new azure.cosmosdb.Account("example", {
* name: "example-ca",
* location: exampleResourceGroup.location,
* resourceGroupName: exampleResourceGroup.name,
* offerType: "Standard",
* kind: "GlobalDocumentDB",
* consistencyPolicy: {
* consistencyLevel: "Session",
* maxIntervalInSeconds: 5,
* maxStalenessPrefix: 100,
* },
* geoLocations: [{
* location: exampleResourceGroup.location,
* failoverPriority: 0,
* }],
* });
* const exampleSqlDatabase = new azure.cosmosdb.SqlDatabase("example", {
* name: "examplecosmosdbsqldb",
* resourceGroupName: exampleAccount.resourceGroupName,
* accountName: exampleAccount.name,
* });
* const exampleSqlContainer = new azure.cosmosdb.SqlContainer("example", {
* name: "examplecosmosdbsqlcon",
* resourceGroupName: exampleAccount.resourceGroupName,
* accountName: exampleAccount.name,
* databaseName: exampleSqlDatabase.name,
* partitionKeyPath: "/part",
* throughput: 400,
* });
* const example = azure.cosmosdb.getSqlRoleDefinitionOutput({
* roleDefinitionId: "00000000-0000-0000-0000-000000000001",
* resourceGroupName: exampleResourceGroup.name,
* accountName: exampleAccount.name,
* });
* const exampleSqlRoleAssignment = new azure.cosmosdb.SqlRoleAssignment("example", {
* resourceGroupName: exampleResourceGroup.name,
* accountName: exampleAccount.name,
* roleDefinitionId: example.apply(example => example.id),
* principalId: exampleCluster.identity.apply(identity => identity?.principalId),
* scope: exampleAccount.id,
* });
* const exampleDatabase = new azure.kusto.Database("example", {
* name: "examplekd",
* resourceGroupName: exampleResourceGroup.name,
* location: exampleResourceGroup.location,
* clusterName: exampleCluster.name,
* });
* const exampleScript = new azure.kusto.Script("example", {
* name: "create-table-script",
* databaseId: exampleDatabase.id,
* scriptContent: `.create table TestTable(Id:string, Name:string, _ts:long, _timestamp:datetime)
* .create table TestTable ingestion json mapping "TestMapping"
* '['
* ' {"column":"Id","path":".id"},'
* ' {"column":"Name","path":".name"},'
* ' {"column":"_ts","path":"._ts"},'
* ' {"column":"_timestamp","path":"._ts", "transform":"DateTimeFromUnixSeconds"}'
* ']'
* .alter table TestTable policy ingestionbatching "{'MaximumBatchingTimeSpan': '0:0:10', 'MaximumNumberOfItems': 10000}"
* `,
* });
* const exampleCosmosdbDataConnection = new azure.kusto.CosmosdbDataConnection("example", {
* name: "examplekcdcd",
* location: exampleResourceGroup.location,
* cosmosdbContainerId: exampleSqlContainer.id,
* kustoDatabaseId: exampleDatabase.id,
* managedIdentityId: exampleCluster.id,
* tableName: "TestTable",
* mappingRuleName: "TestMapping",
* retrievalStartDate: "2023-06-26T12:00:00.6554616Z",
* });
* ```
* ```python
* import pulumi
* import pulumi_azure as azure
* current = azure.core.get_client_config()
* example_resource_group = azure.core.ResourceGroup("example",
* name="exampleRG",
* location="West Europe")
* builtin = azure.authorization.get_role_definition(role_definition_id="fbdf93bf-df7d-467e-a4d2-9458aa1360c8")
* example_cluster = azure.kusto.Cluster("example",
* name="examplekc",
* location=example_resource_group.location,
* resource_group_name=example_resource_group.name,
* sku=azure.kusto.ClusterSkuArgs(
* name="Dev(No SLA)_Standard_D11_v2",
* capacity=1,
* ),
* identity=azure.kusto.ClusterIdentityArgs(
* type="SystemAssigned",
* ))
* example_assignment = azure.authorization.Assignment("example",
* scope=example_resource_group.id,
* role_definition_name=builtin.name,
* principal_id=example_cluster.identity.principal_id)
* example_account = azure.cosmosdb.Account("example",
* name="example-ca",
* location=example_resource_group.location,
* resource_group_name=example_resource_group.name,
* offer_type="Standard",
* kind="GlobalDocumentDB",
* consistency_policy=azure.cosmosdb.AccountConsistencyPolicyArgs(
* consistency_level="Session",
* max_interval_in_seconds=5,
* max_staleness_prefix=100,
* ),
* geo_locations=[azure.cosmosdb.AccountGeoLocationArgs(
* location=example_resource_group.location,
* failover_priority=0,
* )])
* example_sql_database = azure.cosmosdb.SqlDatabase("example",
* name="examplecosmosdbsqldb",
* resource_group_name=example_account.resource_group_name,
* account_name=example_account.name)
* example_sql_container = azure.cosmosdb.SqlContainer("example",
* name="examplecosmosdbsqlcon",
* resource_group_name=example_account.resource_group_name,
* account_name=example_account.name,
* database_name=example_sql_database.name,
* partition_key_path="/part",
* throughput=400)
* example = azure.cosmosdb.get_sql_role_definition_output(role_definition_id="00000000-0000-0000-0000-000000000001",
* resource_group_name=example_resource_group.name,
* account_name=example_account.name)
* example_sql_role_assignment = azure.cosmosdb.SqlRoleAssignment("example",
* resource_group_name=example_resource_group.name,
* account_name=example_account.name,
* role_definition_id=example.id,
* principal_id=example_cluster.identity.principal_id,
* scope=example_account.id)
* example_database = azure.kusto.Database("example",
* name="examplekd",
* resource_group_name=example_resource_group.name,
* location=example_resource_group.location,
* cluster_name=example_cluster.name)
* example_script = azure.kusto.Script("example",
* name="create-table-script",
* database_id=example_database.id,
* script_content=""".create table TestTable(Id:string, Name:string, _ts:long, _timestamp:datetime)
* .create table TestTable ingestion json mapping "TestMapping"
* '['
* ' {"column":"Id","path":"$.id"},'
* ' {"column":"Name","path":"$.name"},'
* ' {"column":"_ts","path":"$._ts"},'
* ' {"column":"_timestamp","path":"$._ts", "transform":"DateTimeFromUnixSeconds"}'
* ']'
* .alter table TestTable policy ingestionbatching "{'MaximumBatchingTimeSpan': '0:0:10', 'MaximumNumberOfItems': 10000}"
* """)
* example_cosmosdb_data_connection = azure.kusto.CosmosdbDataConnection("example",
* name="examplekcdcd",
* location=example_resource_group.location,
* cosmosdb_container_id=example_sql_container.id,
* kusto_database_id=example_database.id,
* managed_identity_id=example_cluster.id,
* table_name="TestTable",
* mapping_rule_name="TestMapping",
* retrieval_start_date="2023-06-26T12:00:00.6554616Z")
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Azure = Pulumi.Azure;
* return await Deployment.RunAsync(() =>
* {
* var current = Azure.Core.GetClientConfig.Invoke();
* var exampleResourceGroup = new Azure.Core.ResourceGroup("example", new()
* {
* Name = "exampleRG",
* Location = "West Europe",
* });
* var builtin = Azure.Authorization.GetRoleDefinition.Invoke(new()
* {
* RoleDefinitionId = "fbdf93bf-df7d-467e-a4d2-9458aa1360c8",
* });
* var exampleCluster = new Azure.Kusto.Cluster("example", new()
* {
* Name = "examplekc",
* Location = exampleResourceGroup.Location,
* ResourceGroupName = exampleResourceGroup.Name,
* Sku = new Azure.Kusto.Inputs.ClusterSkuArgs
* {
* Name = "Dev(No SLA)_Standard_D11_v2",
* Capacity = 1,
* },
* Identity = new Azure.Kusto.Inputs.ClusterIdentityArgs
* {
* Type = "SystemAssigned",
* },
* });
* var exampleAssignment = new Azure.Authorization.Assignment("example", new()
* {
* Scope = exampleResourceGroup.Id,
* RoleDefinitionName = builtin.Apply(getRoleDefinitionResult => getRoleDefinitionResult.Name),
* PrincipalId = exampleCluster.Identity.Apply(identity => identity?.PrincipalId),
* });
* var exampleAccount = new Azure.CosmosDB.Account("example", new()
* {
* Name = "example-ca",
* Location = exampleResourceGroup.Location,
* ResourceGroupName = exampleResourceGroup.Name,
* OfferType = "Standard",
* Kind = "GlobalDocumentDB",
* ConsistencyPolicy = new Azure.CosmosDB.Inputs.AccountConsistencyPolicyArgs
* {
* ConsistencyLevel = "Session",
* MaxIntervalInSeconds = 5,
* MaxStalenessPrefix = 100,
* },
* GeoLocations = new[]
* {
* new Azure.CosmosDB.Inputs.AccountGeoLocationArgs
* {
* Location = exampleResourceGroup.Location,
* FailoverPriority = 0,
* },
* },
* });
* var exampleSqlDatabase = new Azure.CosmosDB.SqlDatabase("example", new()
* {
* Name = "examplecosmosdbsqldb",
* ResourceGroupName = exampleAccount.ResourceGroupName,
* AccountName = exampleAccount.Name,
* });
* var exampleSqlContainer = new Azure.CosmosDB.SqlContainer("example", new()
* {
* Name = "examplecosmosdbsqlcon",
* ResourceGroupName = exampleAccount.ResourceGroupName,
* AccountName = exampleAccount.Name,
* DatabaseName = exampleSqlDatabase.Name,
* PartitionKeyPath = "/part",
* Throughput = 400,
* });
* var example = Azure.CosmosDB.GetSqlRoleDefinition.Invoke(new()
* {
* RoleDefinitionId = "00000000-0000-0000-0000-000000000001",
* ResourceGroupName = exampleResourceGroup.Name,
* AccountName = exampleAccount.Name,
* });
* var exampleSqlRoleAssignment = new Azure.CosmosDB.SqlRoleAssignment("example", new()
* {
* ResourceGroupName = exampleResourceGroup.Name,
* AccountName = exampleAccount.Name,
* RoleDefinitionId = example.Apply(getSqlRoleDefinitionResult => getSqlRoleDefinitionResult.Id),
* PrincipalId = exampleCluster.Identity.Apply(identity => identity?.PrincipalId),
* Scope = exampleAccount.Id,
* });
* var exampleDatabase = new Azure.Kusto.Database("example", new()
* {
* Name = "examplekd",
* ResourceGroupName = exampleResourceGroup.Name,
* Location = exampleResourceGroup.Location,
* ClusterName = exampleCluster.Name,
* });
* var exampleScript = new Azure.Kusto.Script("example", new()
* {
* Name = "create-table-script",
* DatabaseId = exampleDatabase.Id,
* ScriptContent = @".create table TestTable(Id:string, Name:string, _ts:long, _timestamp:datetime)
* .create table TestTable ingestion json mapping ""TestMapping""
* '['
* ' {""column"":""Id"",""path"":""$.id""},'
* ' {""column"":""Name"",""path"":""$.name""},'
* ' {""column"":""_ts"",""path"":""$._ts""},'
* ' {""column"":""_timestamp"",""path"":""$._ts"", ""transform"":""DateTimeFromUnixSeconds""}'
* ']'
* .alter table TestTable policy ingestionbatching ""{'MaximumBatchingTimeSpan': '0:0:10', 'MaximumNumberOfItems': 10000}""
* ",
* });
* var exampleCosmosdbDataConnection = new Azure.Kusto.CosmosdbDataConnection("example", new()
* {
* Name = "examplekcdcd",
* Location = exampleResourceGroup.Location,
* CosmosdbContainerId = exampleSqlContainer.Id,
* KustoDatabaseId = exampleDatabase.Id,
* ManagedIdentityId = exampleCluster.Id,
* TableName = "TestTable",
* MappingRuleName = "TestMapping",
* RetrievalStartDate = "2023-06-26T12:00:00.6554616Z",
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-azure/sdk/v5/go/azure/authorization"
* "github.com/pulumi/pulumi-azure/sdk/v5/go/azure/core"
* "github.com/pulumi/pulumi-azure/sdk/v5/go/azure/cosmosdb"
* "github.com/pulumi/pulumi-azure/sdk/v5/go/azure/kusto"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := core.GetClientConfig(ctx, nil, nil)
* if err != nil {
* return err
* }
* exampleResourceGroup, err := core.NewResourceGroup(ctx, "example", &core.ResourceGroupArgs{
* Name: pulumi.String("exampleRG"),
* Location: pulumi.String("West Europe"),
* })
* if err != nil {
* return err
* }
* builtin, err := authorization.LookupRoleDefinition(ctx, &authorization.LookupRoleDefinitionArgs{
* RoleDefinitionId: pulumi.StringRef("fbdf93bf-df7d-467e-a4d2-9458aa1360c8"),
* }, nil)
* if err != nil {
* return err
* }
* exampleCluster, err := kusto.NewCluster(ctx, "example", &kusto.ClusterArgs{
* Name: pulumi.String("examplekc"),
* Location: exampleResourceGroup.Location,
* ResourceGroupName: exampleResourceGroup.Name,
* Sku: &kusto.ClusterSkuArgs{
* Name: pulumi.String("Dev(No SLA)_Standard_D11_v2"),
* Capacity: pulumi.Int(1),
* },
* Identity: &kusto.ClusterIdentityArgs{
* Type: pulumi.String("SystemAssigned"),
* },
* })
* if err != nil {
* return err
* }
* _, err = authorization.NewAssignment(ctx, "example", &authorization.AssignmentArgs{
* Scope: exampleResourceGroup.ID(),
* RoleDefinitionName: pulumi.String(builtin.Name),
* PrincipalId: exampleCluster.Identity.ApplyT(func(identity kusto.ClusterIdentity) (*string, error) {
* return &identity.PrincipalId, nil
* }).(pulumi.StringPtrOutput),
* })
* if err != nil {
* return err
* }
* exampleAccount, err := cosmosdb.NewAccount(ctx, "example", &cosmosdb.AccountArgs{
* Name: pulumi.String("example-ca"),
* Location: exampleResourceGroup.Location,
* ResourceGroupName: exampleResourceGroup.Name,
* OfferType: pulumi.String("Standard"),
* Kind: pulumi.String("GlobalDocumentDB"),
* ConsistencyPolicy: &cosmosdb.AccountConsistencyPolicyArgs{
* ConsistencyLevel: pulumi.String("Session"),
* MaxIntervalInSeconds: pulumi.Int(5),
* MaxStalenessPrefix: pulumi.Int(100),
* },
* GeoLocations: cosmosdb.AccountGeoLocationArray{
* &cosmosdb.AccountGeoLocationArgs{
* Location: exampleResourceGroup.Location,
* FailoverPriority: pulumi.Int(0),
* },
* },
* })
* if err != nil {
* return err
* }
* exampleSqlDatabase, err := cosmosdb.NewSqlDatabase(ctx, "example", &cosmosdb.SqlDatabaseArgs{
* Name: pulumi.String("examplecosmosdbsqldb"),
* ResourceGroupName: exampleAccount.ResourceGroupName,
* AccountName: exampleAccount.Name,
* })
* if err != nil {
* return err
* }
* exampleSqlContainer, err := cosmosdb.NewSqlContainer(ctx, "example", &cosmosdb.SqlContainerArgs{
* Name: pulumi.String("examplecosmosdbsqlcon"),
* ResourceGroupName: exampleAccount.ResourceGroupName,
* AccountName: exampleAccount.Name,
* DatabaseName: exampleSqlDatabase.Name,
* PartitionKeyPath: pulumi.String("/part"),
* Throughput: pulumi.Int(400),
* })
* if err != nil {
* return err
* }
* example := cosmosdb.LookupSqlRoleDefinitionOutput(ctx, cosmosdb.GetSqlRoleDefinitionOutputArgs{
* RoleDefinitionId: pulumi.String("00000000-0000-0000-0000-000000000001"),
* ResourceGroupName: exampleResourceGroup.Name,
* AccountName: exampleAccount.Name,
* }, nil)
* _, err = cosmosdb.NewSqlRoleAssignment(ctx, "example", &cosmosdb.SqlRoleAssignmentArgs{
* ResourceGroupName: exampleResourceGroup.Name,
* AccountName: exampleAccount.Name,
* RoleDefinitionId: example.ApplyT(func(example cosmosdb.GetSqlRoleDefinitionResult) (*string, error) {
* return &example.Id, nil
* }).(pulumi.StringPtrOutput),
* PrincipalId: exampleCluster.Identity.ApplyT(func(identity kusto.ClusterIdentity) (*string, error) {
* return &identity.PrincipalId, nil
* }).(pulumi.StringPtrOutput),
* Scope: exampleAccount.ID(),
* })
* if err != nil {
* return err
* }
* exampleDatabase, err := kusto.NewDatabase(ctx, "example", &kusto.DatabaseArgs{
* Name: pulumi.String("examplekd"),
* ResourceGroupName: exampleResourceGroup.Name,
* Location: exampleResourceGroup.Location,
* ClusterName: exampleCluster.Name,
* })
* if err != nil {
* return err
* }
* _, err = kusto.NewScript(ctx, "example", &kusto.ScriptArgs{
* Name: pulumi.String("create-table-script"),
* DatabaseId: exampleDatabase.ID(),
* ScriptContent: pulumi.String(`.create table TestTable(Id:string, Name:string, _ts:long, _timestamp:datetime)
* .create table TestTable ingestion json mapping "TestMapping"
* '['
* ' {"column":"Id","path":"$.id"},'
* ' {"column":"Name","path":"$.name"},'
* ' {"column":"_ts","path":"$._ts"},'
* ' {"column":"_timestamp","path":"$._ts", "transform":"DateTimeFromUnixSeconds"}'
* ']'
* .alter table TestTable policy ingestionbatching "{'MaximumBatchingTimeSpan': '0:0:10', 'MaximumNumberOfItems': 10000}"
* `),
* })
* if err != nil {
* return err
* }
* _, err = kusto.NewCosmosdbDataConnection(ctx, "example", &kusto.CosmosdbDataConnectionArgs{
* Name: pulumi.String("examplekcdcd"),
* Location: exampleResourceGroup.Location,
* CosmosdbContainerId: exampleSqlContainer.ID(),
* KustoDatabaseId: exampleDatabase.ID(),
* ManagedIdentityId: exampleCluster.ID(),
* TableName: pulumi.String("TestTable"),
* MappingRuleName: pulumi.String("TestMapping"),
* RetrievalStartDate: pulumi.String("2023-06-26T12:00:00.6554616Z"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azure.core.CoreFunctions;
* import com.pulumi.azure.core.ResourceGroup;
* import com.pulumi.azure.core.ResourceGroupArgs;
* import com.pulumi.azure.authorization.AuthorizationFunctions;
* import com.pulumi.azure.authorization.inputs.GetRoleDefinitionArgs;
* import com.pulumi.azure.kusto.Cluster;
* import com.pulumi.azure.kusto.ClusterArgs;
* import com.pulumi.azure.kusto.inputs.ClusterSkuArgs;
* import com.pulumi.azure.kusto.inputs.ClusterIdentityArgs;
* import com.pulumi.azure.authorization.Assignment;
* import com.pulumi.azure.authorization.AssignmentArgs;
* import com.pulumi.azure.cosmosdb.Account;
* import com.pulumi.azure.cosmosdb.AccountArgs;
* import com.pulumi.azure.cosmosdb.inputs.AccountConsistencyPolicyArgs;
* import com.pulumi.azure.cosmosdb.inputs.AccountGeoLocationArgs;
* import com.pulumi.azure.cosmosdb.SqlDatabase;
* import com.pulumi.azure.cosmosdb.SqlDatabaseArgs;
* import com.pulumi.azure.cosmosdb.SqlContainer;
* import com.pulumi.azure.cosmosdb.SqlContainerArgs;
* import com.pulumi.azure.cosmosdb.CosmosdbFunctions;
* import com.pulumi.azure.cosmosdb.inputs.GetSqlRoleDefinitionArgs;
* import com.pulumi.azure.cosmosdb.SqlRoleAssignment;
* import com.pulumi.azure.cosmosdb.SqlRoleAssignmentArgs;
* import com.pulumi.azure.kusto.Database;
* import com.pulumi.azure.kusto.DatabaseArgs;
* import com.pulumi.azure.kusto.Script;
* import com.pulumi.azure.kusto.ScriptArgs;
* import com.pulumi.azure.kusto.CosmosdbDataConnection;
* import com.pulumi.azure.kusto.CosmosdbDataConnectionArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* final var current = CoreFunctions.getClientConfig();
* var exampleResourceGroup = new ResourceGroup("exampleResourceGroup", ResourceGroupArgs.builder()
* .name("exampleRG")
* .location("West Europe")
* .build());
* final var builtin = AuthorizationFunctions.getRoleDefinition(GetRoleDefinitionArgs.builder()
* .roleDefinitionId("fbdf93bf-df7d-467e-a4d2-9458aa1360c8")
* .build());
* var exampleCluster = new Cluster("exampleCluster", ClusterArgs.builder()
* .name("examplekc")
* .location(exampleResourceGroup.location())
* .resourceGroupName(exampleResourceGroup.name())
* .sku(ClusterSkuArgs.builder()
* .name("Dev(No SLA)_Standard_D11_v2")
* .capacity(1)
* .build())
* .identity(ClusterIdentityArgs.builder()
* .type("SystemAssigned")
* .build())
* .build());
* var exampleAssignment = new Assignment("exampleAssignment", AssignmentArgs.builder()
* .scope(exampleResourceGroup.id())
* .roleDefinitionName(builtin.applyValue(getRoleDefinitionResult -> getRoleDefinitionResult.name()))
* .principalId(exampleCluster.identity().applyValue(identity -> identity.principalId()))
* .build());
* var exampleAccount = new Account("exampleAccount", AccountArgs.builder()
* .name("example-ca")
* .location(exampleResourceGroup.location())
* .resourceGroupName(exampleResourceGroup.name())
* .offerType("Standard")
* .kind("GlobalDocumentDB")
* .consistencyPolicy(AccountConsistencyPolicyArgs.builder()
* .consistencyLevel("Session")
* .maxIntervalInSeconds(5)
* .maxStalenessPrefix(100)
* .build())
* .geoLocations(AccountGeoLocationArgs.builder()
* .location(exampleResourceGroup.location())
* .failoverPriority(0)
* .build())
* .build());
* var exampleSqlDatabase = new SqlDatabase("exampleSqlDatabase", SqlDatabaseArgs.builder()
* .name("examplecosmosdbsqldb")
* .resourceGroupName(exampleAccount.resourceGroupName())
* .accountName(exampleAccount.name())
* .build());
* var exampleSqlContainer = new SqlContainer("exampleSqlContainer", SqlContainerArgs.builder()
* .name("examplecosmosdbsqlcon")
* .resourceGroupName(exampleAccount.resourceGroupName())
* .accountName(exampleAccount.name())
* .databaseName(exampleSqlDatabase.name())
* .partitionKeyPath("/part")
* .throughput(400)
* .build());
* final var example = CosmosdbFunctions.getSqlRoleDefinition(GetSqlRoleDefinitionArgs.builder()
* .roleDefinitionId("00000000-0000-0000-0000-000000000001")
* .resourceGroupName(exampleResourceGroup.name())
* .accountName(exampleAccount.name())
* .build());
* var exampleSqlRoleAssignment = new SqlRoleAssignment("exampleSqlRoleAssignment", SqlRoleAssignmentArgs.builder()
* .resourceGroupName(exampleResourceGroup.name())
* .accountName(exampleAccount.name())
* .roleDefinitionId(example.applyValue(getSqlRoleDefinitionResult -> getSqlRoleDefinitionResult).applyValue(example -> example.applyValue(getSqlRoleDefinitionResult -> getSqlRoleDefinitionResult.id())))
* .principalId(exampleCluster.identity().applyValue(identity -> identity.principalId()))
* .scope(exampleAccount.id())
* .build());
* var exampleDatabase = new Database("exampleDatabase", DatabaseArgs.builder()
* .name("examplekd")
* .resourceGroupName(exampleResourceGroup.name())
* .location(exampleResourceGroup.location())
* .clusterName(exampleCluster.name())
* .build());
* var exampleScript = new Script("exampleScript", ScriptArgs.builder()
* .name("create-table-script")
* .databaseId(exampleDatabase.id())
* .scriptContent("""
* .create table TestTable(Id:string, Name:string, _ts:long, _timestamp:datetime)
* .create table TestTable ingestion json mapping "TestMapping"
* '['
* ' {"column":"Id","path":"$.id"},'
* ' {"column":"Name","path":"$.name"},'
* ' {"column":"_ts","path":"$._ts"},'
* ' {"column":"_timestamp","path":"$._ts", "transform":"DateTimeFromUnixSeconds"}'
* ']'
* .alter table TestTable policy ingestionbatching "{'MaximumBatchingTimeSpan': '0:0:10', 'MaximumNumberOfItems': 10000}"
* """)
* .build());
* var exampleCosmosdbDataConnection = new CosmosdbDataConnection("exampleCosmosdbDataConnection", CosmosdbDataConnectionArgs.builder()
* .name("examplekcdcd")
* .location(exampleResourceGroup.location())
* .cosmosdbContainerId(exampleSqlContainer.id())
* .kustoDatabaseId(exampleDatabase.id())
* .managedIdentityId(exampleCluster.id())
* .tableName("TestTable")
* .mappingRuleName("TestMapping")
* .retrievalStartDate("2023-06-26T12:00:00.6554616Z")
* .build());
* }
* }
* ```
* ```yaml
* resources:
* exampleResourceGroup:
* type: azure:core:ResourceGroup
* name: example
* properties:
* name: exampleRG
* location: West Europe
* exampleAssignment:
* type: azure:authorization:Assignment
* name: example
* properties:
* scope: ${exampleResourceGroup.id}
* roleDefinitionName: ${builtin.name}
* principalId: ${exampleCluster.identity.principalId}
* exampleAccount:
* type: azure:cosmosdb:Account
* name: example
* properties:
* name: example-ca
* location: ${exampleResourceGroup.location}
* resourceGroupName: ${exampleResourceGroup.name}
* offerType: Standard
* kind: GlobalDocumentDB
* consistencyPolicy:
* consistencyLevel: Session
* maxIntervalInSeconds: 5
* maxStalenessPrefix: 100
* geoLocations:
* - location: ${exampleResourceGroup.location}
* failoverPriority: 0
* exampleSqlDatabase:
* type: azure:cosmosdb:SqlDatabase
* name: example
* properties:
* name: examplecosmosdbsqldb
* resourceGroupName: ${exampleAccount.resourceGroupName}
* accountName: ${exampleAccount.name}
* exampleSqlContainer:
* type: azure:cosmosdb:SqlContainer
* name: example
* properties:
* name: examplecosmosdbsqlcon
* resourceGroupName: ${exampleAccount.resourceGroupName}
* accountName: ${exampleAccount.name}
* databaseName: ${exampleSqlDatabase.name}
* partitionKeyPath: /part
* throughput: 400
* exampleSqlRoleAssignment:
* type: azure:cosmosdb:SqlRoleAssignment
* name: example
* properties:
* resourceGroupName: ${exampleResourceGroup.name}
* accountName: ${exampleAccount.name}
* roleDefinitionId: ${example.id}
* principalId: ${exampleCluster.identity.principalId}
* scope: ${exampleAccount.id}
* exampleCluster:
* type: azure:kusto:Cluster
* name: example
* properties:
* name: examplekc
* location: ${exampleResourceGroup.location}
* resourceGroupName: ${exampleResourceGroup.name}
* sku:
* name: Dev(No SLA)_Standard_D11_v2
* capacity: 1
* identity:
* type: SystemAssigned
* exampleDatabase:
* type: azure:kusto:Database
* name: example
* properties:
* name: examplekd
* resourceGroupName: ${exampleResourceGroup.name}
* location: ${exampleResourceGroup.location}
* clusterName: ${exampleCluster.name}
* exampleScript:
* type: azure:kusto:Script
* name: example
* properties:
* name: create-table-script
* databaseId: ${exampleDatabase.id}
* scriptContent: |
* .create table TestTable(Id:string, Name:string, _ts:long, _timestamp:datetime)
* .create table TestTable ingestion json mapping "TestMapping"
* '['
* ' {"column":"Id","path":"$.id"},'
* ' {"column":"Name","path":"$.name"},'
* ' {"column":"_ts","path":"$._ts"},'
* ' {"column":"_timestamp","path":"$._ts", "transform":"DateTimeFromUnixSeconds"}'
* ']'
* .alter table TestTable policy ingestionbatching "{'MaximumBatchingTimeSpan': '0:0:10', 'MaximumNumberOfItems': 10000}"
* exampleCosmosdbDataConnection:
* type: azure:kusto:CosmosdbDataConnection
* name: example
* properties:
* name: examplekcdcd
* location: ${exampleResourceGroup.location}
* cosmosdbContainerId: ${exampleSqlContainer.id}
* kustoDatabaseId: ${exampleDatabase.id}
* managedIdentityId: ${exampleCluster.id}
* tableName: TestTable
* mappingRuleName: TestMapping
* retrievalStartDate: 2023-06-26T12:00:00.6554616Z
* variables:
* current:
* fn::invoke:
* Function: azure:core:getClientConfig
* Arguments: {}
* builtin:
* fn::invoke:
* Function: azure:authorization:getRoleDefinition
* Arguments:
* roleDefinitionId: fbdf93bf-df7d-467e-a4d2-9458aa1360c8
* example:
* fn::invoke:
* Function: azure:cosmosdb:getSqlRoleDefinition
* Arguments:
* roleDefinitionId: 00000000-0000-0000-0000-000000000001
* resourceGroupName: ${exampleResourceGroup.name}
* accountName: ${exampleAccount.name}
* ```
*
* ## Import
* Kusto / Cosmos Database Data Connection can be imported using the `resource id`, e.g.
* ```sh
* $ pulumi import azure:kusto/cosmosdbDataConnection:CosmosdbDataConnection example /subscriptions/00000000-0000-0000-0000-000000000000/resourceGroups/group1/providers/Microsoft.Kusto/clusters/cluster1/databases/database1/dataConnections/dataConnection1
* ```
* @property cosmosdbContainerId The name of an existing container in the Cosmos DB database. Changing this forces a new Kusto Cosmos DB Connection to be created.
* @property kustoDatabaseId The name of the database in the Kusto cluster. Changing this forces a new Kusto Cosmos DB Connection to be created.
* @property location The Azure Region where the Data Explorer should exist. Changing this forces a new Kusto Cosmos DB Connection to be created.
* @property managedIdentityId The resource ID of a managed system or user-assigned identity. The identity is used to authenticate with Cosmos DB. Changing this forces a new Kusto Cosmos DB Connection to be created.
* @property mappingRuleName The name of an existing mapping rule to use when ingesting the retrieved data. Changing this forces a new Kusto Cosmos DB Connection to be created.
* @property name The name of the data connection. Changing this forces a new Kusto Cosmos DB Connection to be created.
* @property retrievalStartDate If defined, the data connection retrieves Cosmos DB documents created or updated after the specified retrieval start date. Changing this forces a new Kusto Cosmos DB Connection to be created.
* @property tableName The case-sensitive name of the existing target table in your cluster. Retrieved data is ingested into this table. Changing this forces a new Kusto Cosmos DB Connection to be created.
*/
public data class CosmosdbDataConnectionArgs(
public val cosmosdbContainerId: Output? = null,
public val kustoDatabaseId: Output? = null,
public val location: Output? = null,
public val managedIdentityId: Output? = null,
public val mappingRuleName: Output? = null,
public val name: Output? = null,
public val retrievalStartDate: Output? = null,
public val tableName: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azure.kusto.CosmosdbDataConnectionArgs =
com.pulumi.azure.kusto.CosmosdbDataConnectionArgs.builder()
.cosmosdbContainerId(cosmosdbContainerId?.applyValue({ args0 -> args0 }))
.kustoDatabaseId(kustoDatabaseId?.applyValue({ args0 -> args0 }))
.location(location?.applyValue({ args0 -> args0 }))
.managedIdentityId(managedIdentityId?.applyValue({ args0 -> args0 }))
.mappingRuleName(mappingRuleName?.applyValue({ args0 -> args0 }))
.name(name?.applyValue({ args0 -> args0 }))
.retrievalStartDate(retrievalStartDate?.applyValue({ args0 -> args0 }))
.tableName(tableName?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [CosmosdbDataConnectionArgs].
*/
@PulumiTagMarker
public class CosmosdbDataConnectionArgsBuilder internal constructor() {
private var cosmosdbContainerId: Output? = null
private var kustoDatabaseId: Output? = null
private var location: Output? = null
private var managedIdentityId: Output? = null
private var mappingRuleName: Output? = null
private var name: Output? = null
private var retrievalStartDate: Output? = null
private var tableName: Output? = null
/**
* @param value The name of an existing container in the Cosmos DB database. Changing this forces a new Kusto Cosmos DB Connection to be created.
*/
@JvmName("tmrqckqggfsqxijn")
public suspend fun cosmosdbContainerId(`value`: Output) {
this.cosmosdbContainerId = value
}
/**
* @param value The name of the database in the Kusto cluster. Changing this forces a new Kusto Cosmos DB Connection to be created.
*/
@JvmName("ghrtrpougbfmcmuf")
public suspend fun kustoDatabaseId(`value`: Output) {
this.kustoDatabaseId = value
}
/**
* @param value The Azure Region where the Data Explorer should exist. Changing this forces a new Kusto Cosmos DB Connection to be created.
*/
@JvmName("jyrtfuauurjcobgj")
public suspend fun location(`value`: Output) {
this.location = value
}
/**
* @param value The resource ID of a managed system or user-assigned identity. The identity is used to authenticate with Cosmos DB. Changing this forces a new Kusto Cosmos DB Connection to be created.
*/
@JvmName("hsikolgmnvwvlund")
public suspend fun managedIdentityId(`value`: Output) {
this.managedIdentityId = value
}
/**
* @param value The name of an existing mapping rule to use when ingesting the retrieved data. Changing this forces a new Kusto Cosmos DB Connection to be created.
*/
@JvmName("fqwsfojgfmfprgfy")
public suspend fun mappingRuleName(`value`: Output) {
this.mappingRuleName = value
}
/**
* @param value The name of the data connection. Changing this forces a new Kusto Cosmos DB Connection to be created.
*/
@JvmName("uqrglicmsxxqpahb")
public suspend fun name(`value`: Output) {
this.name = value
}
/**
* @param value If defined, the data connection retrieves Cosmos DB documents created or updated after the specified retrieval start date. Changing this forces a new Kusto Cosmos DB Connection to be created.
*/
@JvmName("yytpqtkiruolacns")
public suspend fun retrievalStartDate(`value`: Output) {
this.retrievalStartDate = value
}
/**
* @param value The case-sensitive name of the existing target table in your cluster. Retrieved data is ingested into this table. Changing this forces a new Kusto Cosmos DB Connection to be created.
*/
@JvmName("roteaeftiagitqql")
public suspend fun tableName(`value`: Output) {
this.tableName = value
}
/**
* @param value The name of an existing container in the Cosmos DB database. Changing this forces a new Kusto Cosmos DB Connection to be created.
*/
@JvmName("mynlcrlrasghhjjr")
public suspend fun cosmosdbContainerId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.cosmosdbContainerId = mapped
}
/**
* @param value The name of the database in the Kusto cluster. Changing this forces a new Kusto Cosmos DB Connection to be created.
*/
@JvmName("jkalpkeiquodxhsa")
public suspend fun kustoDatabaseId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.kustoDatabaseId = mapped
}
/**
* @param value The Azure Region where the Data Explorer should exist. Changing this forces a new Kusto Cosmos DB Connection to be created.
*/
@JvmName("rrapyujhxirovgqh")
public suspend fun location(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.location = mapped
}
/**
* @param value The resource ID of a managed system or user-assigned identity. The identity is used to authenticate with Cosmos DB. Changing this forces a new Kusto Cosmos DB Connection to be created.
*/
@JvmName("cfdkgetxmwynlqfs")
public suspend fun managedIdentityId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.managedIdentityId = mapped
}
/**
* @param value The name of an existing mapping rule to use when ingesting the retrieved data. Changing this forces a new Kusto Cosmos DB Connection to be created.
*/
@JvmName("lmnhisvmwhugkltc")
public suspend fun mappingRuleName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.mappingRuleName = mapped
}
/**
* @param value The name of the data connection. Changing this forces a new Kusto Cosmos DB Connection to be created.
*/
@JvmName("klwlywepspyajucr")
public suspend fun name(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.name = mapped
}
/**
* @param value If defined, the data connection retrieves Cosmos DB documents created or updated after the specified retrieval start date. Changing this forces a new Kusto Cosmos DB Connection to be created.
*/
@JvmName("irfrilmsjfbxsram")
public suspend fun retrievalStartDate(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.retrievalStartDate = mapped
}
/**
* @param value The case-sensitive name of the existing target table in your cluster. Retrieved data is ingested into this table. Changing this forces a new Kusto Cosmos DB Connection to be created.
*/
@JvmName("gswvyupqoyipbask")
public suspend fun tableName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.tableName = mapped
}
internal fun build(): CosmosdbDataConnectionArgs = CosmosdbDataConnectionArgs(
cosmosdbContainerId = cosmosdbContainerId,
kustoDatabaseId = kustoDatabaseId,
location = location,
managedIdentityId = managedIdentityId,
mappingRuleName = mappingRuleName,
name = name,
retrievalStartDate = retrievalStartDate,
tableName = tableName,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy