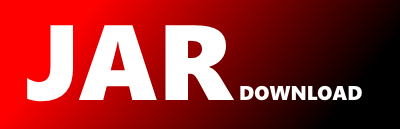
com.pulumi.azure.lab.kotlin.inputs.ServicePlanDefaultAutoShutdownArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-kotlin Show documentation
Show all versions of pulumi-azure-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azure.lab.kotlin.inputs
import com.pulumi.azure.lab.inputs.ServicePlanDefaultAutoShutdownArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
*
* @property disconnectDelay The amount of time a VM will stay running after a user disconnects if this behavior is enabled. This value must be formatted as an ISO 8601 string.
* @property idleDelay The amount of time a VM will idle before it is shutdown if this behavior is enabled. This value must be formatted as an ISO 8601 string.
* @property noConnectDelay The amount of time a VM will stay running before it is shutdown if no connection is made and this behavior is enabled. This value must be formatted as an ISO 8601 string.
* @property shutdownOnIdle Will a VM get shutdown when it has idled for a period of time? Possible values are `LowUsage` and `UserAbsence`.
* > **NOTE:** This property is `None` when it isn't specified. No need to set `idle_delay` when `shutdown_on_idle` isn't specified.
*/
public data class ServicePlanDefaultAutoShutdownArgs(
public val disconnectDelay: Output? = null,
public val idleDelay: Output? = null,
public val noConnectDelay: Output? = null,
public val shutdownOnIdle: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azure.lab.inputs.ServicePlanDefaultAutoShutdownArgs =
com.pulumi.azure.lab.inputs.ServicePlanDefaultAutoShutdownArgs.builder()
.disconnectDelay(disconnectDelay?.applyValue({ args0 -> args0 }))
.idleDelay(idleDelay?.applyValue({ args0 -> args0 }))
.noConnectDelay(noConnectDelay?.applyValue({ args0 -> args0 }))
.shutdownOnIdle(shutdownOnIdle?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [ServicePlanDefaultAutoShutdownArgs].
*/
@PulumiTagMarker
public class ServicePlanDefaultAutoShutdownArgsBuilder internal constructor() {
private var disconnectDelay: Output? = null
private var idleDelay: Output? = null
private var noConnectDelay: Output? = null
private var shutdownOnIdle: Output? = null
/**
* @param value The amount of time a VM will stay running after a user disconnects if this behavior is enabled. This value must be formatted as an ISO 8601 string.
*/
@JvmName("pseejorebxsncggh")
public suspend fun disconnectDelay(`value`: Output) {
this.disconnectDelay = value
}
/**
* @param value The amount of time a VM will idle before it is shutdown if this behavior is enabled. This value must be formatted as an ISO 8601 string.
*/
@JvmName("pnlclsldeqeuined")
public suspend fun idleDelay(`value`: Output) {
this.idleDelay = value
}
/**
* @param value The amount of time a VM will stay running before it is shutdown if no connection is made and this behavior is enabled. This value must be formatted as an ISO 8601 string.
*/
@JvmName("tqsxgevlclnlwiln")
public suspend fun noConnectDelay(`value`: Output) {
this.noConnectDelay = value
}
/**
* @param value Will a VM get shutdown when it has idled for a period of time? Possible values are `LowUsage` and `UserAbsence`.
* > **NOTE:** This property is `None` when it isn't specified. No need to set `idle_delay` when `shutdown_on_idle` isn't specified.
*/
@JvmName("cydudxqlwmyqcsdj")
public suspend fun shutdownOnIdle(`value`: Output) {
this.shutdownOnIdle = value
}
/**
* @param value The amount of time a VM will stay running after a user disconnects if this behavior is enabled. This value must be formatted as an ISO 8601 string.
*/
@JvmName("jendujjuoukskqnf")
public suspend fun disconnectDelay(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.disconnectDelay = mapped
}
/**
* @param value The amount of time a VM will idle before it is shutdown if this behavior is enabled. This value must be formatted as an ISO 8601 string.
*/
@JvmName("uwlkdriicjaqwuxw")
public suspend fun idleDelay(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.idleDelay = mapped
}
/**
* @param value The amount of time a VM will stay running before it is shutdown if no connection is made and this behavior is enabled. This value must be formatted as an ISO 8601 string.
*/
@JvmName("clfopygfcvxfdflv")
public suspend fun noConnectDelay(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.noConnectDelay = mapped
}
/**
* @param value Will a VM get shutdown when it has idled for a period of time? Possible values are `LowUsage` and `UserAbsence`.
* > **NOTE:** This property is `None` when it isn't specified. No need to set `idle_delay` when `shutdown_on_idle` isn't specified.
*/
@JvmName("kinskhlvtcedvvcb")
public suspend fun shutdownOnIdle(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.shutdownOnIdle = mapped
}
internal fun build(): ServicePlanDefaultAutoShutdownArgs = ServicePlanDefaultAutoShutdownArgs(
disconnectDelay = disconnectDelay,
idleDelay = idleDelay,
noConnectDelay = noConnectDelay,
shutdownOnIdle = shutdownOnIdle,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy