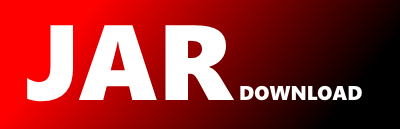
com.pulumi.azure.lb.kotlin.LbFunctions.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-kotlin Show documentation
Show all versions of pulumi-azure-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azure.lb.kotlin
import com.pulumi.azure.lb.LbFunctions.getBackendAddressPoolPlain
import com.pulumi.azure.lb.LbFunctions.getLBOutboundRulePlain
import com.pulumi.azure.lb.LbFunctions.getLBPlain
import com.pulumi.azure.lb.LbFunctions.getLBRulePlain
import com.pulumi.azure.lb.kotlin.inputs.GetBackendAddressPoolPlainArgs
import com.pulumi.azure.lb.kotlin.inputs.GetBackendAddressPoolPlainArgsBuilder
import com.pulumi.azure.lb.kotlin.inputs.GetLBOutboundRulePlainArgs
import com.pulumi.azure.lb.kotlin.inputs.GetLBOutboundRulePlainArgsBuilder
import com.pulumi.azure.lb.kotlin.inputs.GetLBPlainArgs
import com.pulumi.azure.lb.kotlin.inputs.GetLBPlainArgsBuilder
import com.pulumi.azure.lb.kotlin.inputs.GetLBRulePlainArgs
import com.pulumi.azure.lb.kotlin.inputs.GetLBRulePlainArgsBuilder
import com.pulumi.azure.lb.kotlin.outputs.GetBackendAddressPoolResult
import com.pulumi.azure.lb.kotlin.outputs.GetLBOutboundRuleResult
import com.pulumi.azure.lb.kotlin.outputs.GetLBResult
import com.pulumi.azure.lb.kotlin.outputs.GetLBRuleResult
import kotlinx.coroutines.future.await
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import com.pulumi.azure.lb.kotlin.outputs.GetBackendAddressPoolResult.Companion.toKotlin as getBackendAddressPoolResultToKotlin
import com.pulumi.azure.lb.kotlin.outputs.GetLBOutboundRuleResult.Companion.toKotlin as getLBOutboundRuleResultToKotlin
import com.pulumi.azure.lb.kotlin.outputs.GetLBResult.Companion.toKotlin as getLBResultToKotlin
import com.pulumi.azure.lb.kotlin.outputs.GetLBRuleResult.Companion.toKotlin as getLBRuleResultToKotlin
public object LbFunctions {
/**
* Use this data source to access information about an existing Load Balancer's Backend Address Pool.
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as azure from "@pulumi/azure";
* const example = azure.lb.getLB({
* name: "example-lb",
* resourceGroupName: "example-resources",
* });
* const exampleGetBackendAddressPool = example.then(example => azure.lb.getBackendAddressPool({
* name: "first",
* loadbalancerId: example.id,
* }));
* export const backendAddressPoolId = exampleGetBackendAddressPool.then(exampleGetBackendAddressPool => exampleGetBackendAddressPool.id);
* export const backendIpConfigurationIds = beap.backendIpConfigurations.map(__item => __item.id);
* ```
* ```python
* import pulumi
* import pulumi_azure as azure
* example = azure.lb.get_lb(name="example-lb",
* resource_group_name="example-resources")
* example_get_backend_address_pool = azure.lb.get_backend_address_pool(name="first",
* loadbalancer_id=example.id)
* pulumi.export("backendAddressPoolId", example_get_backend_address_pool.id)
* pulumi.export("backendIpConfigurationIds", [__item["id"] for __item in beap["backendIpConfigurations"]])
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Azure = Pulumi.Azure;
* return await Deployment.RunAsync(() =>
* {
* var example = Azure.Lb.GetLB.Invoke(new()
* {
* Name = "example-lb",
* ResourceGroupName = "example-resources",
* });
* var exampleGetBackendAddressPool = Azure.Lb.GetBackendAddressPool.Invoke(new()
* {
* Name = "first",
* LoadbalancerId = example.Apply(getLBResult => getLBResult.Id),
* });
* return new Dictionary
* {
* ["backendAddressPoolId"] = exampleGetBackendAddressPool.Apply(getBackendAddressPoolResult => getBackendAddressPoolResult.Id),
* ["backendIpConfigurationIds"] = beap.BackendIpConfigurations.Select(__item => __item.Id).ToList(),
* };
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-azure/sdk/v5/go/azure/lb"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* example, err := lb.GetLB(ctx, &lb.GetLBArgs{
* Name: "example-lb",
* ResourceGroupName: "example-resources",
* }, nil)
* if err != nil {
* return err
* }
* exampleGetBackendAddressPool, err := lb.LookupBackendAddressPool(ctx, &lb.LookupBackendAddressPoolArgs{
* Name: "first",
* LoadbalancerId: example.Id,
* }, nil)
* if err != nil {
* return err
* }
* ctx.Export("backendAddressPoolId", exampleGetBackendAddressPool.Id)
* var splat0 []interface{}
* for _, val0 := range beap.BackendIpConfigurations {
* splat0 = append(splat0, val0.Id)
* }
* ctx.Export("backendIpConfigurationIds", splat0)
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azure.lb.LbFunctions;
* import com.pulumi.azure.lb.inputs.GetLBArgs;
* import com.pulumi.azure.lb.inputs.GetBackendAddressPoolArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* final var example = LbFunctions.getLB(GetLBArgs.builder()
* .name("example-lb")
* .resourceGroupName("example-resources")
* .build());
* final var exampleGetBackendAddressPool = LbFunctions.getBackendAddressPool(GetBackendAddressPoolArgs.builder()
* .name("first")
* .loadbalancerId(example.applyValue(getLBResult -> getLBResult.id()))
* .build());
* ctx.export("backendAddressPoolId", exampleGetBackendAddressPool.applyValue(getBackendAddressPoolResult -> getBackendAddressPoolResult.id()));
* ctx.export("backendIpConfigurationIds", beap.backendIpConfigurations().stream().map(element -> element.id()).collect(toList()));
* }
* }
* ```
*
* @param argument A collection of arguments for invoking getBackendAddressPool.
* @return A collection of values returned by getBackendAddressPool.
*/
public suspend fun getBackendAddressPool(argument: GetBackendAddressPoolPlainArgs):
GetBackendAddressPoolResult =
getBackendAddressPoolResultToKotlin(getBackendAddressPoolPlain(argument.toJava()).await())
/**
* @see [getBackendAddressPool].
* @param loadbalancerId The ID of the Load Balancer in which the Backend Address Pool exists.
* @param name Specifies the name of the Backend Address Pool.
* @return A collection of values returned by getBackendAddressPool.
*/
public suspend fun getBackendAddressPool(loadbalancerId: String, name: String):
GetBackendAddressPoolResult {
val argument = GetBackendAddressPoolPlainArgs(
loadbalancerId = loadbalancerId,
name = name,
)
return getBackendAddressPoolResultToKotlin(getBackendAddressPoolPlain(argument.toJava()).await())
}
/**
* @see [getBackendAddressPool].
* @param argument Builder for [com.pulumi.azure.lb.kotlin.inputs.GetBackendAddressPoolPlainArgs].
* @return A collection of values returned by getBackendAddressPool.
*/
public suspend
fun getBackendAddressPool(argument: suspend GetBackendAddressPoolPlainArgsBuilder.() -> Unit):
GetBackendAddressPoolResult {
val builder = GetBackendAddressPoolPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getBackendAddressPoolResultToKotlin(getBackendAddressPoolPlain(builtArgument.toJava()).await())
}
/**
* Use this data source to access information about an existing Load Balancer
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as azure from "@pulumi/azure";
* const example = azure.lb.getLB({
* name: "example-lb",
* resourceGroupName: "example-resources",
* });
* export const loadbalancerId = example.then(example => example.id);
* ```
* ```python
* import pulumi
* import pulumi_azure as azure
* example = azure.lb.get_lb(name="example-lb",
* resource_group_name="example-resources")
* pulumi.export("loadbalancerId", example.id)
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Azure = Pulumi.Azure;
* return await Deployment.RunAsync(() =>
* {
* var example = Azure.Lb.GetLB.Invoke(new()
* {
* Name = "example-lb",
* ResourceGroupName = "example-resources",
* });
* return new Dictionary
* {
* ["loadbalancerId"] = example.Apply(getLBResult => getLBResult.Id),
* };
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-azure/sdk/v5/go/azure/lb"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* example, err := lb.GetLB(ctx, &lb.GetLBArgs{
* Name: "example-lb",
* ResourceGroupName: "example-resources",
* }, nil)
* if err != nil {
* return err
* }
* ctx.Export("loadbalancerId", example.Id)
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azure.lb.LbFunctions;
* import com.pulumi.azure.lb.inputs.GetLBArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* final var example = LbFunctions.getLB(GetLBArgs.builder()
* .name("example-lb")
* .resourceGroupName("example-resources")
* .build());
* ctx.export("loadbalancerId", example.applyValue(getLBResult -> getLBResult.id()));
* }
* }
* ```
* ```yaml
* variables:
* example:
* fn::invoke:
* Function: azure:lb:getLB
* Arguments:
* name: example-lb
* resourceGroupName: example-resources
* outputs:
* loadbalancerId: ${example.id}
* ```
*
* @param argument A collection of arguments for invoking getLB.
* @return A collection of values returned by getLB.
*/
public suspend fun getLB(argument: GetLBPlainArgs): GetLBResult =
getLBResultToKotlin(getLBPlain(argument.toJava()).await())
/**
* @see [getLB].
* @param name Specifies the name of the Load Balancer.
* @param resourceGroupName The name of the Resource Group in which the Load Balancer exists.
* @return A collection of values returned by getLB.
*/
public suspend fun getLB(name: String, resourceGroupName: String): GetLBResult {
val argument = GetLBPlainArgs(
name = name,
resourceGroupName = resourceGroupName,
)
return getLBResultToKotlin(getLBPlain(argument.toJava()).await())
}
/**
* @see [getLB].
* @param argument Builder for [com.pulumi.azure.lb.kotlin.inputs.GetLBPlainArgs].
* @return A collection of values returned by getLB.
*/
public suspend fun getLB(argument: suspend GetLBPlainArgsBuilder.() -> Unit): GetLBResult {
val builder = GetLBPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getLBResultToKotlin(getLBPlain(builtArgument.toJava()).await())
}
/**
* Use this data source to access information about an existing Load Balancer Outbound Rule.
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as azure from "@pulumi/azure";
* const example = azure.lb.getLBOutboundRule({
* name: "existing_lb_outbound_rule",
* loadbalancerId: "existing_load_balancer_id",
* });
* export const id = example.then(example => example.id);
* ```
* ```python
* import pulumi
* import pulumi_azure as azure
* example = azure.lb.get_lb_outbound_rule(name="existing_lb_outbound_rule",
* loadbalancer_id="existing_load_balancer_id")
* pulumi.export("id", example.id)
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Azure = Pulumi.Azure;
* return await Deployment.RunAsync(() =>
* {
* var example = Azure.Lb.GetLBOutboundRule.Invoke(new()
* {
* Name = "existing_lb_outbound_rule",
* LoadbalancerId = "existing_load_balancer_id",
* });
* return new Dictionary
* {
* ["id"] = example.Apply(getLBOutboundRuleResult => getLBOutboundRuleResult.Id),
* };
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-azure/sdk/v5/go/azure/lb"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* example, err := lb.GetLBOutboundRule(ctx, &lb.GetLBOutboundRuleArgs{
* Name: "existing_lb_outbound_rule",
* LoadbalancerId: "existing_load_balancer_id",
* }, nil)
* if err != nil {
* return err
* }
* ctx.Export("id", example.Id)
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azure.lb.LbFunctions;
* import com.pulumi.azure.lb.inputs.GetLBOutboundRuleArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* final var example = LbFunctions.getLBOutboundRule(GetLBOutboundRuleArgs.builder()
* .name("existing_lb_outbound_rule")
* .loadbalancerId("existing_load_balancer_id")
* .build());
* ctx.export("id", example.applyValue(getLBOutboundRuleResult -> getLBOutboundRuleResult.id()));
* }
* }
* ```
* ```yaml
* variables:
* example:
* fn::invoke:
* Function: azure:lb:getLBOutboundRule
* Arguments:
* name: existing_lb_outbound_rule
* loadbalancerId: existing_load_balancer_id
* outputs:
* id: ${example.id}
* ```
*
* @param argument A collection of arguments for invoking getLBOutboundRule.
* @return A collection of values returned by getLBOutboundRule.
*/
public suspend fun getLBOutboundRule(argument: GetLBOutboundRulePlainArgs):
GetLBOutboundRuleResult =
getLBOutboundRuleResultToKotlin(getLBOutboundRulePlain(argument.toJava()).await())
/**
* @see [getLBOutboundRule].
* @param loadbalancerId The ID of the Load Balancer in which the Outbound Rule exists.
* @param name The name of this Load Balancer Outbound Rule.
* @return A collection of values returned by getLBOutboundRule.
*/
public suspend fun getLBOutboundRule(loadbalancerId: String, name: String):
GetLBOutboundRuleResult {
val argument = GetLBOutboundRulePlainArgs(
loadbalancerId = loadbalancerId,
name = name,
)
return getLBOutboundRuleResultToKotlin(getLBOutboundRulePlain(argument.toJava()).await())
}
/**
* @see [getLBOutboundRule].
* @param argument Builder for [com.pulumi.azure.lb.kotlin.inputs.GetLBOutboundRulePlainArgs].
* @return A collection of values returned by getLBOutboundRule.
*/
public suspend
fun getLBOutboundRule(argument: suspend GetLBOutboundRulePlainArgsBuilder.() -> Unit):
GetLBOutboundRuleResult {
val builder = GetLBOutboundRulePlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getLBOutboundRuleResultToKotlin(getLBOutboundRulePlain(builtArgument.toJava()).await())
}
/**
* Use this data source to access information about an existing Load Balancer Rule.
* ## Example Usage
*
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azure.lb.LbFunctions;
* import com.pulumi.azure.lb.inputs.GetLBArgs;
* import com.pulumi.azure.lb.inputs.GetLBRuleArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* final var example = LbFunctions.getLB(GetLBArgs.builder()
* .name("example-lb")
* .resourceGroupName("example-resources")
* .build());
* final var exampleGetLBRule = LbFunctions.getLBRule(GetLBRuleArgs.builder()
* .name("first")
* .resourceGroupName("example-resources")
* .loadbalancerId(example.applyValue(getLBResult -> getLBResult.id()))
* .build());
* ctx.export("lbRuleId", exampleGetLBRule.applyValue(getLBRuleResult -> getLBRuleResult.id()));
* }
* }
* ```
* ```yaml
* variables:
* example:
* fn::invoke:
* Function: azure:lb:getLB
* Arguments:
* name: example-lb
* resourceGroupName: example-resources
* exampleGetLBRule:
* fn::invoke:
* Function: azure:lb:getLBRule
* Arguments:
* name: first
* resourceGroupName: example-resources
* loadbalancerId: ${example.id}
* outputs:
* lbRuleId: ${exampleGetLBRule.id}
* ```
*
* @param argument A collection of arguments for invoking getLBRule.
* @return A collection of values returned by getLBRule.
*/
public suspend fun getLBRule(argument: GetLBRulePlainArgs): GetLBRuleResult =
getLBRuleResultToKotlin(getLBRulePlain(argument.toJava()).await())
/**
* @see [getLBRule].
* @param loadbalancerId The ID of the Load Balancer Rule.
* @param name The name of this Load Balancer Rule.
* @return A collection of values returned by getLBRule.
*/
public suspend fun getLBRule(loadbalancerId: String, name: String): GetLBRuleResult {
val argument = GetLBRulePlainArgs(
loadbalancerId = loadbalancerId,
name = name,
)
return getLBRuleResultToKotlin(getLBRulePlain(argument.toJava()).await())
}
/**
* @see [getLBRule].
* @param argument Builder for [com.pulumi.azure.lb.kotlin.inputs.GetLBRulePlainArgs].
* @return A collection of values returned by getLBRule.
*/
public suspend fun getLBRule(argument: suspend GetLBRulePlainArgsBuilder.() -> Unit):
GetLBRuleResult {
val builder = GetLBRulePlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getLBRuleResultToKotlin(getLBRulePlain(builtArgument.toJava()).await())
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy