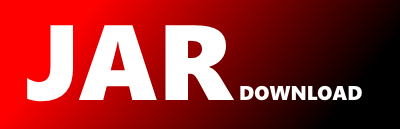
com.pulumi.azure.lb.kotlin.NatPool.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-kotlin Show documentation
Show all versions of pulumi-azure-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azure.lb.kotlin
import com.pulumi.core.Output
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
/**
* Builder for [NatPool].
*/
@PulumiTagMarker
public class NatPoolResourceBuilder internal constructor() {
public var name: String? = null
public var args: NatPoolArgs = NatPoolArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend NatPoolArgsBuilder.() -> Unit) {
val builder = NatPoolArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): NatPool {
val builtJavaResource = com.pulumi.azure.lb.NatPool(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return NatPool(builtJavaResource)
}
}
/**
* Manages a Load Balancer NAT pool.
* > **NOTE:** This resource cannot be used with with virtual machines, instead use the `azure.lb.NatRule` resource.
* > **NOTE** When using this resource, the Load Balancer needs to have a FrontEnd IP Configuration Attached
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as azure from "@pulumi/azure";
* const example = new azure.core.ResourceGroup("example", {
* name: "LoadBalancerRG",
* location: "West Europe",
* });
* const examplePublicIp = new azure.network.PublicIp("example", {
* name: "PublicIPForLB",
* location: example.location,
* resourceGroupName: example.name,
* allocationMethod: "Static",
* });
* const exampleLoadBalancer = new azure.lb.LoadBalancer("example", {
* name: "TestLoadBalancer",
* location: example.location,
* resourceGroupName: example.name,
* frontendIpConfigurations: [{
* name: "PublicIPAddress",
* publicIpAddressId: examplePublicIp.id,
* }],
* });
* const exampleNatPool = new azure.lb.NatPool("example", {
* resourceGroupName: example.name,
* loadbalancerId: exampleLoadBalancer.id,
* name: "SampleApplicationPool",
* protocol: "Tcp",
* frontendPortStart: 80,
* frontendPortEnd: 81,
* backendPort: 8080,
* frontendIpConfigurationName: "PublicIPAddress",
* });
* ```
* ```python
* import pulumi
* import pulumi_azure as azure
* example = azure.core.ResourceGroup("example",
* name="LoadBalancerRG",
* location="West Europe")
* example_public_ip = azure.network.PublicIp("example",
* name="PublicIPForLB",
* location=example.location,
* resource_group_name=example.name,
* allocation_method="Static")
* example_load_balancer = azure.lb.LoadBalancer("example",
* name="TestLoadBalancer",
* location=example.location,
* resource_group_name=example.name,
* frontend_ip_configurations=[azure.lb.LoadBalancerFrontendIpConfigurationArgs(
* name="PublicIPAddress",
* public_ip_address_id=example_public_ip.id,
* )])
* example_nat_pool = azure.lb.NatPool("example",
* resource_group_name=example.name,
* loadbalancer_id=example_load_balancer.id,
* name="SampleApplicationPool",
* protocol="Tcp",
* frontend_port_start=80,
* frontend_port_end=81,
* backend_port=8080,
* frontend_ip_configuration_name="PublicIPAddress")
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Azure = Pulumi.Azure;
* return await Deployment.RunAsync(() =>
* {
* var example = new Azure.Core.ResourceGroup("example", new()
* {
* Name = "LoadBalancerRG",
* Location = "West Europe",
* });
* var examplePublicIp = new Azure.Network.PublicIp("example", new()
* {
* Name = "PublicIPForLB",
* Location = example.Location,
* ResourceGroupName = example.Name,
* AllocationMethod = "Static",
* });
* var exampleLoadBalancer = new Azure.Lb.LoadBalancer("example", new()
* {
* Name = "TestLoadBalancer",
* Location = example.Location,
* ResourceGroupName = example.Name,
* FrontendIpConfigurations = new[]
* {
* new Azure.Lb.Inputs.LoadBalancerFrontendIpConfigurationArgs
* {
* Name = "PublicIPAddress",
* PublicIpAddressId = examplePublicIp.Id,
* },
* },
* });
* var exampleNatPool = new Azure.Lb.NatPool("example", new()
* {
* ResourceGroupName = example.Name,
* LoadbalancerId = exampleLoadBalancer.Id,
* Name = "SampleApplicationPool",
* Protocol = "Tcp",
* FrontendPortStart = 80,
* FrontendPortEnd = 81,
* BackendPort = 8080,
* FrontendIpConfigurationName = "PublicIPAddress",
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-azure/sdk/v5/go/azure/core"
* "github.com/pulumi/pulumi-azure/sdk/v5/go/azure/lb"
* "github.com/pulumi/pulumi-azure/sdk/v5/go/azure/network"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* example, err := core.NewResourceGroup(ctx, "example", &core.ResourceGroupArgs{
* Name: pulumi.String("LoadBalancerRG"),
* Location: pulumi.String("West Europe"),
* })
* if err != nil {
* return err
* }
* examplePublicIp, err := network.NewPublicIp(ctx, "example", &network.PublicIpArgs{
* Name: pulumi.String("PublicIPForLB"),
* Location: example.Location,
* ResourceGroupName: example.Name,
* AllocationMethod: pulumi.String("Static"),
* })
* if err != nil {
* return err
* }
* exampleLoadBalancer, err := lb.NewLoadBalancer(ctx, "example", &lb.LoadBalancerArgs{
* Name: pulumi.String("TestLoadBalancer"),
* Location: example.Location,
* ResourceGroupName: example.Name,
* FrontendIpConfigurations: lb.LoadBalancerFrontendIpConfigurationArray{
* &lb.LoadBalancerFrontendIpConfigurationArgs{
* Name: pulumi.String("PublicIPAddress"),
* PublicIpAddressId: examplePublicIp.ID(),
* },
* },
* })
* if err != nil {
* return err
* }
* _, err = lb.NewNatPool(ctx, "example", &lb.NatPoolArgs{
* ResourceGroupName: example.Name,
* LoadbalancerId: exampleLoadBalancer.ID(),
* Name: pulumi.String("SampleApplicationPool"),
* Protocol: pulumi.String("Tcp"),
* FrontendPortStart: pulumi.Int(80),
* FrontendPortEnd: pulumi.Int(81),
* BackendPort: pulumi.Int(8080),
* FrontendIpConfigurationName: pulumi.String("PublicIPAddress"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azure.core.ResourceGroup;
* import com.pulumi.azure.core.ResourceGroupArgs;
* import com.pulumi.azure.network.PublicIp;
* import com.pulumi.azure.network.PublicIpArgs;
* import com.pulumi.azure.lb.LoadBalancer;
* import com.pulumi.azure.lb.LoadBalancerArgs;
* import com.pulumi.azure.lb.inputs.LoadBalancerFrontendIpConfigurationArgs;
* import com.pulumi.azure.lb.NatPool;
* import com.pulumi.azure.lb.NatPoolArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var example = new ResourceGroup("example", ResourceGroupArgs.builder()
* .name("LoadBalancerRG")
* .location("West Europe")
* .build());
* var examplePublicIp = new PublicIp("examplePublicIp", PublicIpArgs.builder()
* .name("PublicIPForLB")
* .location(example.location())
* .resourceGroupName(example.name())
* .allocationMethod("Static")
* .build());
* var exampleLoadBalancer = new LoadBalancer("exampleLoadBalancer", LoadBalancerArgs.builder()
* .name("TestLoadBalancer")
* .location(example.location())
* .resourceGroupName(example.name())
* .frontendIpConfigurations(LoadBalancerFrontendIpConfigurationArgs.builder()
* .name("PublicIPAddress")
* .publicIpAddressId(examplePublicIp.id())
* .build())
* .build());
* var exampleNatPool = new NatPool("exampleNatPool", NatPoolArgs.builder()
* .resourceGroupName(example.name())
* .loadbalancerId(exampleLoadBalancer.id())
* .name("SampleApplicationPool")
* .protocol("Tcp")
* .frontendPortStart(80)
* .frontendPortEnd(81)
* .backendPort(8080)
* .frontendIpConfigurationName("PublicIPAddress")
* .build());
* }
* }
* ```
* ```yaml
* resources:
* example:
* type: azure:core:ResourceGroup
* properties:
* name: LoadBalancerRG
* location: West Europe
* examplePublicIp:
* type: azure:network:PublicIp
* name: example
* properties:
* name: PublicIPForLB
* location: ${example.location}
* resourceGroupName: ${example.name}
* allocationMethod: Static
* exampleLoadBalancer:
* type: azure:lb:LoadBalancer
* name: example
* properties:
* name: TestLoadBalancer
* location: ${example.location}
* resourceGroupName: ${example.name}
* frontendIpConfigurations:
* - name: PublicIPAddress
* publicIpAddressId: ${examplePublicIp.id}
* exampleNatPool:
* type: azure:lb:NatPool
* name: example
* properties:
* resourceGroupName: ${example.name}
* loadbalancerId: ${exampleLoadBalancer.id}
* name: SampleApplicationPool
* protocol: Tcp
* frontendPortStart: 80
* frontendPortEnd: 81
* backendPort: 8080
* frontendIpConfigurationName: PublicIPAddress
* ```
*
* ## Import
* Load Balancer NAT Pools can be imported using the `resource id`, e.g.
* ```sh
* $ pulumi import azure:lb/natPool:NatPool example /subscriptions/00000000-0000-0000-0000-000000000000/resourceGroups/group1/providers/Microsoft.Network/loadBalancers/lb1/inboundNatPools/pool1
* ```
*/
public class NatPool internal constructor(
override val javaResource: com.pulumi.azure.lb.NatPool,
) : KotlinCustomResource(javaResource, NatPoolMapper) {
/**
* The port used for the internal endpoint. Possible values range between 1 and 65535, inclusive.
*/
public val backendPort: Output
get() = javaResource.backendPort().applyValue({ args0 -> args0 })
/**
* Are the floating IPs enabled for this Load Balancer Rule? A floating IP is reassigned to a secondary server in case the primary server fails. Required to configure a SQL AlwaysOn Availability Group.
*/
public val floatingIpEnabled: Output?
get() = javaResource.floatingIpEnabled().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
public val frontendIpConfigurationId: Output
get() = javaResource.frontendIpConfigurationId().applyValue({ args0 -> args0 })
/**
* The name of the frontend IP configuration exposing this rule.
*/
public val frontendIpConfigurationName: Output
get() = javaResource.frontendIpConfigurationName().applyValue({ args0 -> args0 })
/**
* The last port number in the range of external ports that will be used to provide Inbound NAT to NICs associated with this Load Balancer. Possible values range between 1 and 65534, inclusive.
*/
public val frontendPortEnd: Output
get() = javaResource.frontendPortEnd().applyValue({ args0 -> args0 })
/**
* The first port number in the range of external ports that will be used to provide Inbound NAT to NICs associated with this Load Balancer. Possible values range between 1 and 65534, inclusive.
*/
public val frontendPortStart: Output
get() = javaResource.frontendPortStart().applyValue({ args0 -> args0 })
/**
* Specifies the idle timeout in minutes for TCP connections. Valid values are between `4` and `30`. Defaults to `4`.
*/
public val idleTimeoutInMinutes: Output?
get() = javaResource.idleTimeoutInMinutes().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The ID of the Load Balancer in which to create the NAT pool. Changing this forces a new resource to be created.
*/
public val loadbalancerId: Output
get() = javaResource.loadbalancerId().applyValue({ args0 -> args0 })
/**
* Specifies the name of the NAT pool. Changing this forces a new resource to be created.
*/
public val name: Output
get() = javaResource.name().applyValue({ args0 -> args0 })
/**
* The transport protocol for the external endpoint. Possible values are `All`, `Tcp` and `Udp`.
*/
public val protocol: Output
get() = javaResource.protocol().applyValue({ args0 -> args0 })
/**
* The name of the resource group in which to create the resource. Changing this forces a new resource to be created.
*/
public val resourceGroupName: Output
get() = javaResource.resourceGroupName().applyValue({ args0 -> args0 })
/**
* Is TCP Reset enabled for this Load Balancer Rule?
*/
public val tcpResetEnabled: Output?
get() = javaResource.tcpResetEnabled().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
}
public object NatPoolMapper : ResourceMapper {
override fun supportsMappingOfType(javaResource: Resource): Boolean =
com.pulumi.azure.lb.NatPool::class == javaResource::class
override fun map(javaResource: Resource): NatPool = NatPool(
javaResource as
com.pulumi.azure.lb.NatPool,
)
}
/**
* @see [NatPool].
* @param name The _unique_ name of the resulting resource.
* @param block Builder for [NatPool].
*/
public suspend fun natPool(name: String, block: suspend NatPoolResourceBuilder.() -> Unit):
NatPool {
val builder = NatPoolResourceBuilder()
builder.name(name)
block(builder)
return builder.build()
}
/**
* @see [NatPool].
* @param name The _unique_ name of the resulting resource.
*/
public fun natPool(name: String): NatPool {
val builder = NatPoolResourceBuilder()
builder.name(name)
return builder.build()
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy