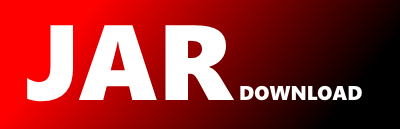
com.pulumi.azure.machinelearning.kotlin.DatastoreFileshareArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-kotlin Show documentation
Show all versions of pulumi-azure-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azure.machinelearning.kotlin
import com.pulumi.azure.machinelearning.DatastoreFileshareArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Pair
import kotlin.String
import kotlin.Suppress
import kotlin.collections.Map
import kotlin.jvm.JvmName
/**
* Manages a Machine Learning File Share DataStore.
* ## Example Usage
* ### With Azure File Share
*
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azure.core.CoreFunctions;
* import com.pulumi.azure.core.ResourceGroup;
* import com.pulumi.azure.core.ResourceGroupArgs;
* import com.pulumi.azure.appinsights.Insights;
* import com.pulumi.azure.appinsights.InsightsArgs;
* import com.pulumi.azure.keyvault.KeyVault;
* import com.pulumi.azure.keyvault.KeyVaultArgs;
* import com.pulumi.azure.storage.Account;
* import com.pulumi.azure.storage.AccountArgs;
* import com.pulumi.azure.machinelearning.Workspace;
* import com.pulumi.azure.machinelearning.WorkspaceArgs;
* import com.pulumi.azure.machinelearning.inputs.WorkspaceIdentityArgs;
* import com.pulumi.azure.storage.Share;
* import com.pulumi.azure.storage.ShareArgs;
* import com.pulumi.azure.machinelearning.DatastoreFileshare;
* import com.pulumi.azure.machinelearning.DatastoreFileshareArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* final var current = CoreFunctions.getClientConfig();
* var example = new ResourceGroup("example", ResourceGroupArgs.builder()
* .name("example-resources")
* .location("West Europe")
* .build());
* var exampleInsights = new Insights("exampleInsights", InsightsArgs.builder()
* .name("workspace-example-ai")
* .location(example.location())
* .resourceGroupName(example.name())
* .applicationType("web")
* .build());
* var exampleKeyVault = new KeyVault("exampleKeyVault", KeyVaultArgs.builder()
* .name("workspaceexamplekeyvault")
* .location(example.location())
* .resourceGroupName(example.name())
* .tenantId(current.applyValue(getClientConfigResult -> getClientConfigResult.tenantId()))
* .skuName("premium")
* .build());
* var exampleAccount = new Account("exampleAccount", AccountArgs.builder()
* .name("workspacestorageaccount")
* .location(example.location())
* .resourceGroupName(example.name())
* .accountTier("Standard")
* .accountReplicationType("GRS")
* .build());
* var exampleWorkspace = new Workspace("exampleWorkspace", WorkspaceArgs.builder()
* .name("example-workspace")
* .location(example.location())
* .resourceGroupName(example.name())
* .applicationInsightsId(exampleInsights.id())
* .keyVaultId(exampleKeyVault.id())
* .storageAccountId(exampleAccount.id())
* .identity(WorkspaceIdentityArgs.builder()
* .type("SystemAssigned")
* .build())
* .build());
* var exampleShare = new Share("exampleShare", ShareArgs.builder()
* .name("example")
* .storageAccountName(exampleAccount.name())
* .quota(1)
* .build());
* var exampleDatastoreFileshare = new DatastoreFileshare("exampleDatastoreFileshare", DatastoreFileshareArgs.builder()
* .name("example-datastore")
* .workspaceId(exampleWorkspace.id())
* .storageFilesahareId(test.resourceManagerId())
* .accountKey(exampleAccount.primaryAccessKey())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* example:
* type: azure:core:ResourceGroup
* properties:
* name: example-resources
* location: West Europe
* exampleInsights:
* type: azure:appinsights:Insights
* name: example
* properties:
* name: workspace-example-ai
* location: ${example.location}
* resourceGroupName: ${example.name}
* applicationType: web
* exampleKeyVault:
* type: azure:keyvault:KeyVault
* name: example
* properties:
* name: workspaceexamplekeyvault
* location: ${example.location}
* resourceGroupName: ${example.name}
* tenantId: ${current.tenantId}
* skuName: premium
* exampleAccount:
* type: azure:storage:Account
* name: example
* properties:
* name: workspacestorageaccount
* location: ${example.location}
* resourceGroupName: ${example.name}
* accountTier: Standard
* accountReplicationType: GRS
* exampleWorkspace:
* type: azure:machinelearning:Workspace
* name: example
* properties:
* name: example-workspace
* location: ${example.location}
* resourceGroupName: ${example.name}
* applicationInsightsId: ${exampleInsights.id}
* keyVaultId: ${exampleKeyVault.id}
* storageAccountId: ${exampleAccount.id}
* identity:
* type: SystemAssigned
* exampleShare:
* type: azure:storage:Share
* name: example
* properties:
* name: example
* storageAccountName: ${exampleAccount.name}
* quota: 1
* exampleDatastoreFileshare:
* type: azure:machinelearning:DatastoreFileshare
* name: example
* properties:
* name: example-datastore
* workspaceId: ${exampleWorkspace.id}
* storageFilesahareId: ${test.resourceManagerId}
* accountKey: ${exampleAccount.primaryAccessKey}
* variables:
* current:
* fn::invoke:
* Function: azure:core:getClientConfig
* Arguments: {}
* ```
*
* ## Import
* Machine Learning DataStores can be imported using the `resource id`, e.g.
* ```sh
* $ pulumi import azure:machinelearning/datastoreFileshare:DatastoreFileshare example /subscriptions/00000000-0000-0000-0000-000000000000/resourceGroups/group1/providers/Microsoft.MachineLearningServices/workspaces/mlw1/dataStores/datastore1
* ```
* @property accountKey The access key of the Storage Account. Conflicts with `shared_access_signature`.
* @property description Text used to describe the asset. Changing this forces a new Machine Learning DataStore to be created.
* @property name The name of the Machine Learning DataStore. Changing this forces a new Machine Learning DataStore to be created.
* @property serviceDataIdentity Specifies which identity to use when retrieving data from the specified source. Defaults to `None`. Possible values are `None`, `WorkspaceSystemAssignedIdentity` and `WorkspaceUserAssignedIdentity`.
* @property sharedAccessSignature The Shared Access Signature of the Storage Account. Conflicts with `account_key`.
* @property storageFileshareId The ID of the Storage Account File Share. Changing this forces a new Machine Learning DataStore to be created.
* @property tags A mapping of tags which should be assigned to the Machine Learning DataStore. Changing this forces a new Machine Learning DataStore to be created.
* @property workspaceId The ID of the Machine Learning Workspace. Changing this forces a new Machine Learning DataStore to be created.
*/
public data class DatastoreFileshareArgs(
public val accountKey: Output? = null,
public val description: Output? = null,
public val name: Output? = null,
public val serviceDataIdentity: Output? = null,
public val sharedAccessSignature: Output? = null,
public val storageFileshareId: Output? = null,
public val tags: Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy