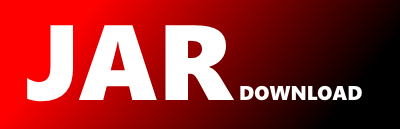
com.pulumi.azure.maintenance.kotlin.inputs.ConfigurationInstallPatchesArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-kotlin Show documentation
Show all versions of pulumi-azure-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azure.maintenance.kotlin.inputs
import com.pulumi.azure.maintenance.inputs.ConfigurationInstallPatchesArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
*
* @property linuxes A `linux` block as defined above. This property only applies when `scope` is set to `InGuestPatch`
* @property reboot Possible reboot preference as defined by the user based on which it would be decided to reboot the machine or not after the patch operation is completed. Possible values are `Always`, `IfRequired` and `Never`. This property only applies when `scope` is set to `InGuestPatch`.
* @property windows A `windows` block as defined above. This property only applies when `scope` is set to `InGuestPatch`
*/
public data class ConfigurationInstallPatchesArgs(
public val linuxes: Output>? = null,
public val reboot: Output? = null,
public val windows: Output>? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azure.maintenance.inputs.ConfigurationInstallPatchesArgs =
com.pulumi.azure.maintenance.inputs.ConfigurationInstallPatchesArgs.builder()
.linuxes(
linuxes?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
)
.reboot(reboot?.applyValue({ args0 -> args0 }))
.windows(
windows?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
).build()
}
/**
* Builder for [ConfigurationInstallPatchesArgs].
*/
@PulumiTagMarker
public class ConfigurationInstallPatchesArgsBuilder internal constructor() {
private var linuxes: Output>? = null
private var reboot: Output? = null
private var windows: Output>? = null
/**
* @param value A `linux` block as defined above. This property only applies when `scope` is set to `InGuestPatch`
*/
@JvmName("poaeigeesbnbtvhm")
public suspend fun linuxes(`value`: Output>) {
this.linuxes = value
}
@JvmName("rmsqdkpkwaitmxab")
public suspend fun linuxes(vararg values: Output) {
this.linuxes = Output.all(values.asList())
}
/**
* @param values A `linux` block as defined above. This property only applies when `scope` is set to `InGuestPatch`
*/
@JvmName("mtwqyuhymteiyadl")
public suspend fun linuxes(values: List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy