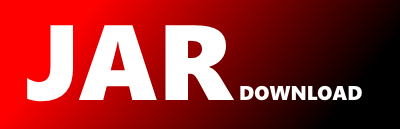
com.pulumi.azure.management.kotlin.GroupPolicyExemptionArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-kotlin Show documentation
Show all versions of pulumi-azure-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azure.management.kotlin
import com.pulumi.azure.management.GroupPolicyExemptionArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
* Manages a Management Group Policy Exemption.
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as azure from "@pulumi/azure";
* const exampleGroup = new azure.management.Group("example", {displayName: "Example MgmtGroup"});
* const example = azure.policy.getPolicySetDefinition({
* displayName: "Audit machines with insecure password security settings",
* });
* const exampleGroupPolicyAssignment = new azure.management.GroupPolicyAssignment("example", {
* name: "assignment1",
* managementGroupId: exampleGroup.id,
* policyDefinitionId: example.then(example => example.id),
* location: "westus",
* identity: {
* type: "SystemAssigned",
* },
* });
* const exampleGroupPolicyExemption = new azure.management.GroupPolicyExemption("example", {
* name: "exemption1",
* managementGroupId: exampleGroup.id,
* policyAssignmentId: exampleGroupPolicyAssignment.id,
* exemptionCategory: "Mitigated",
* });
* ```
* ```python
* import pulumi
* import pulumi_azure as azure
* example_group = azure.management.Group("example", display_name="Example MgmtGroup")
* example = azure.policy.get_policy_set_definition(display_name="Audit machines with insecure password security settings")
* example_group_policy_assignment = azure.management.GroupPolicyAssignment("example",
* name="assignment1",
* management_group_id=example_group.id,
* policy_definition_id=example.id,
* location="westus",
* identity=azure.management.GroupPolicyAssignmentIdentityArgs(
* type="SystemAssigned",
* ))
* example_group_policy_exemption = azure.management.GroupPolicyExemption("example",
* name="exemption1",
* management_group_id=example_group.id,
* policy_assignment_id=example_group_policy_assignment.id,
* exemption_category="Mitigated")
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Azure = Pulumi.Azure;
* return await Deployment.RunAsync(() =>
* {
* var exampleGroup = new Azure.Management.Group("example", new()
* {
* DisplayName = "Example MgmtGroup",
* });
* var example = Azure.Policy.GetPolicySetDefinition.Invoke(new()
* {
* DisplayName = "Audit machines with insecure password security settings",
* });
* var exampleGroupPolicyAssignment = new Azure.Management.GroupPolicyAssignment("example", new()
* {
* Name = "assignment1",
* ManagementGroupId = exampleGroup.Id,
* PolicyDefinitionId = example.Apply(getPolicySetDefinitionResult => getPolicySetDefinitionResult.Id),
* Location = "westus",
* Identity = new Azure.Management.Inputs.GroupPolicyAssignmentIdentityArgs
* {
* Type = "SystemAssigned",
* },
* });
* var exampleGroupPolicyExemption = new Azure.Management.GroupPolicyExemption("example", new()
* {
* Name = "exemption1",
* ManagementGroupId = exampleGroup.Id,
* PolicyAssignmentId = exampleGroupPolicyAssignment.Id,
* ExemptionCategory = "Mitigated",
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-azure/sdk/v5/go/azure/management"
* "github.com/pulumi/pulumi-azure/sdk/v5/go/azure/policy"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* exampleGroup, err := management.NewGroup(ctx, "example", &management.GroupArgs{
* DisplayName: pulumi.String("Example MgmtGroup"),
* })
* if err != nil {
* return err
* }
* example, err := policy.LookupPolicySetDefinition(ctx, &policy.LookupPolicySetDefinitionArgs{
* DisplayName: pulumi.StringRef("Audit machines with insecure password security settings"),
* }, nil)
* if err != nil {
* return err
* }
* exampleGroupPolicyAssignment, err := management.NewGroupPolicyAssignment(ctx, "example", &management.GroupPolicyAssignmentArgs{
* Name: pulumi.String("assignment1"),
* ManagementGroupId: exampleGroup.ID(),
* PolicyDefinitionId: pulumi.String(example.Id),
* Location: pulumi.String("westus"),
* Identity: &management.GroupPolicyAssignmentIdentityArgs{
* Type: pulumi.String("SystemAssigned"),
* },
* })
* if err != nil {
* return err
* }
* _, err = management.NewGroupPolicyExemption(ctx, "example", &management.GroupPolicyExemptionArgs{
* Name: pulumi.String("exemption1"),
* ManagementGroupId: exampleGroup.ID(),
* PolicyAssignmentId: exampleGroupPolicyAssignment.ID(),
* ExemptionCategory: pulumi.String("Mitigated"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azure.management.Group;
* import com.pulumi.azure.management.GroupArgs;
* import com.pulumi.azure.policy.PolicyFunctions;
* import com.pulumi.azure.policy.inputs.GetPolicySetDefinitionArgs;
* import com.pulumi.azure.management.GroupPolicyAssignment;
* import com.pulumi.azure.management.GroupPolicyAssignmentArgs;
* import com.pulumi.azure.management.inputs.GroupPolicyAssignmentIdentityArgs;
* import com.pulumi.azure.management.GroupPolicyExemption;
* import com.pulumi.azure.management.GroupPolicyExemptionArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var exampleGroup = new Group("exampleGroup", GroupArgs.builder()
* .displayName("Example MgmtGroup")
* .build());
* final var example = PolicyFunctions.getPolicySetDefinition(GetPolicySetDefinitionArgs.builder()
* .displayName("Audit machines with insecure password security settings")
* .build());
* var exampleGroupPolicyAssignment = new GroupPolicyAssignment("exampleGroupPolicyAssignment", GroupPolicyAssignmentArgs.builder()
* .name("assignment1")
* .managementGroupId(exampleGroup.id())
* .policyDefinitionId(example.applyValue(getPolicySetDefinitionResult -> getPolicySetDefinitionResult.id()))
* .location("westus")
* .identity(GroupPolicyAssignmentIdentityArgs.builder()
* .type("SystemAssigned")
* .build())
* .build());
* var exampleGroupPolicyExemption = new GroupPolicyExemption("exampleGroupPolicyExemption", GroupPolicyExemptionArgs.builder()
* .name("exemption1")
* .managementGroupId(exampleGroup.id())
* .policyAssignmentId(exampleGroupPolicyAssignment.id())
* .exemptionCategory("Mitigated")
* .build());
* }
* }
* ```
* ```yaml
* resources:
* exampleGroup:
* type: azure:management:Group
* name: example
* properties:
* displayName: Example MgmtGroup
* exampleGroupPolicyAssignment:
* type: azure:management:GroupPolicyAssignment
* name: example
* properties:
* name: assignment1
* managementGroupId: ${exampleGroup.id}
* policyDefinitionId: ${example.id}
* location: westus
* identity:
* type: SystemAssigned
* exampleGroupPolicyExemption:
* type: azure:management:GroupPolicyExemption
* name: example
* properties:
* name: exemption1
* managementGroupId: ${exampleGroup.id}
* policyAssignmentId: ${exampleGroupPolicyAssignment.id}
* exemptionCategory: Mitigated
* variables:
* example:
* fn::invoke:
* Function: azure:policy:getPolicySetDefinition
* Arguments:
* displayName: Audit machines with insecure password security settings
* ```
*
* ## Import
* Policy Exemptions can be imported using the `resource id`, e.g.
* ```sh
* $ pulumi import azure:management/groupPolicyExemption:GroupPolicyExemption exemption1 /providers/Microsoft.Management/managementGroups/group1/providers/Microsoft.Authorization/policyExemptions/exemption1
* ```
* @property description A description to use for this Policy Exemption.
* @property displayName A friendly display name to use for this Policy Exemption.
* @property exemptionCategory The category of this policy exemption. Possible values are `Waiver` and `Mitigated`.
* @property expiresOn The expiration date and time in UTC ISO 8601 format of this policy exemption.
* @property managementGroupId The Management Group ID where the Policy Exemption should be applied. Changing this forces a new resource to be created.
* @property metadata The metadata for this policy exemption. This is a JSON string representing additional metadata that should be stored with the policy exemption.
* @property name The name of the Policy Exemption. Changing this forces a new resource to be created.
* @property policyAssignmentId The ID of the Policy Assignment to be exempted at the specified Scope.
* @property policyDefinitionReferenceIds The policy definition reference ID list when the associated policy assignment is an assignment of a policy set definition.
*/
public data class GroupPolicyExemptionArgs(
public val description: Output? = null,
public val displayName: Output? = null,
public val exemptionCategory: Output? = null,
public val expiresOn: Output? = null,
public val managementGroupId: Output? = null,
public val metadata: Output? = null,
public val name: Output? = null,
public val policyAssignmentId: Output? = null,
public val policyDefinitionReferenceIds: Output>? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azure.management.GroupPolicyExemptionArgs =
com.pulumi.azure.management.GroupPolicyExemptionArgs.builder()
.description(description?.applyValue({ args0 -> args0 }))
.displayName(displayName?.applyValue({ args0 -> args0 }))
.exemptionCategory(exemptionCategory?.applyValue({ args0 -> args0 }))
.expiresOn(expiresOn?.applyValue({ args0 -> args0 }))
.managementGroupId(managementGroupId?.applyValue({ args0 -> args0 }))
.metadata(metadata?.applyValue({ args0 -> args0 }))
.name(name?.applyValue({ args0 -> args0 }))
.policyAssignmentId(policyAssignmentId?.applyValue({ args0 -> args0 }))
.policyDefinitionReferenceIds(
policyDefinitionReferenceIds?.applyValue({ args0 ->
args0.map({ args0 -> args0 })
}),
).build()
}
/**
* Builder for [GroupPolicyExemptionArgs].
*/
@PulumiTagMarker
public class GroupPolicyExemptionArgsBuilder internal constructor() {
private var description: Output? = null
private var displayName: Output? = null
private var exemptionCategory: Output? = null
private var expiresOn: Output? = null
private var managementGroupId: Output? = null
private var metadata: Output? = null
private var name: Output? = null
private var policyAssignmentId: Output? = null
private var policyDefinitionReferenceIds: Output>? = null
/**
* @param value A description to use for this Policy Exemption.
*/
@JvmName("pxgfqfaahetbevcv")
public suspend fun description(`value`: Output) {
this.description = value
}
/**
* @param value A friendly display name to use for this Policy Exemption.
*/
@JvmName("uqhrxipnusrsoqee")
public suspend fun displayName(`value`: Output) {
this.displayName = value
}
/**
* @param value The category of this policy exemption. Possible values are `Waiver` and `Mitigated`.
*/
@JvmName("knfpbuionrvjfdvc")
public suspend fun exemptionCategory(`value`: Output) {
this.exemptionCategory = value
}
/**
* @param value The expiration date and time in UTC ISO 8601 format of this policy exemption.
*/
@JvmName("btqiitothkmowwst")
public suspend fun expiresOn(`value`: Output) {
this.expiresOn = value
}
/**
* @param value The Management Group ID where the Policy Exemption should be applied. Changing this forces a new resource to be created.
*/
@JvmName("unaknofckcxjqeml")
public suspend fun managementGroupId(`value`: Output) {
this.managementGroupId = value
}
/**
* @param value The metadata for this policy exemption. This is a JSON string representing additional metadata that should be stored with the policy exemption.
*/
@JvmName("kogtlefvvslairmb")
public suspend fun metadata(`value`: Output) {
this.metadata = value
}
/**
* @param value The name of the Policy Exemption. Changing this forces a new resource to be created.
*/
@JvmName("eqmkrgmwkfghdnck")
public suspend fun name(`value`: Output) {
this.name = value
}
/**
* @param value The ID of the Policy Assignment to be exempted at the specified Scope.
*/
@JvmName("yraqfqnsericqfgv")
public suspend fun policyAssignmentId(`value`: Output) {
this.policyAssignmentId = value
}
/**
* @param value The policy definition reference ID list when the associated policy assignment is an assignment of a policy set definition.
*/
@JvmName("ofqhwemsulsfarjx")
public suspend fun policyDefinitionReferenceIds(`value`: Output>) {
this.policyDefinitionReferenceIds = value
}
@JvmName("tfydykrkewjniboi")
public suspend fun policyDefinitionReferenceIds(vararg values: Output) {
this.policyDefinitionReferenceIds = Output.all(values.asList())
}
/**
* @param values The policy definition reference ID list when the associated policy assignment is an assignment of a policy set definition.
*/
@JvmName("lthpotvieofianul")
public suspend fun policyDefinitionReferenceIds(values: List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy