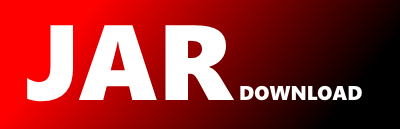
com.pulumi.azure.management.kotlin.GroupPolicyRemediationArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-kotlin Show documentation
Show all versions of pulumi-azure-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azure.management.kotlin
import com.pulumi.azure.management.GroupPolicyRemediationArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Deprecated
import kotlin.Double
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
* Manages an Azure Management Group Policy Remediation.
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as azure from "@pulumi/azure";
* const exampleGroup = new azure.management.Group("example", {displayName: "Example Management Group"});
* const example = azure.policy.getPolicyDefintion({
* displayName: "Allowed locations",
* });
* const exampleGroupPolicyAssignment = new azure.management.GroupPolicyAssignment("example", {
* name: "exampleAssignment",
* managementGroupId: exampleGroup.id,
* policyDefinitionId: example.then(example => example.id),
* parameters: JSON.stringify({
* listOfAllowedLocations: {
* value: ["East US"],
* },
* }),
* });
* const exampleGroupPolicyRemediation = new azure.management.GroupPolicyRemediation("example", {
* name: "example",
* managementGroupId: exampleGroup.id,
* policyAssignmentId: exampleGroupPolicyAssignment.id,
* });
* ```
* ```python
* import pulumi
* import json
* import pulumi_azure as azure
* example_group = azure.management.Group("example", display_name="Example Management Group")
* example = azure.policy.get_policy_defintion(display_name="Allowed locations")
* example_group_policy_assignment = azure.management.GroupPolicyAssignment("example",
* name="exampleAssignment",
* management_group_id=example_group.id,
* policy_definition_id=example.id,
* parameters=json.dumps({
* "listOfAllowedLocations": {
* "value": ["East US"],
* },
* }))
* example_group_policy_remediation = azure.management.GroupPolicyRemediation("example",
* name="example",
* management_group_id=example_group.id,
* policy_assignment_id=example_group_policy_assignment.id)
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using System.Text.Json;
* using Pulumi;
* using Azure = Pulumi.Azure;
* return await Deployment.RunAsync(() =>
* {
* var exampleGroup = new Azure.Management.Group("example", new()
* {
* DisplayName = "Example Management Group",
* });
* var example = Azure.Policy.GetPolicyDefintion.Invoke(new()
* {
* DisplayName = "Allowed locations",
* });
* var exampleGroupPolicyAssignment = new Azure.Management.GroupPolicyAssignment("example", new()
* {
* Name = "exampleAssignment",
* ManagementGroupId = exampleGroup.Id,
* PolicyDefinitionId = example.Apply(getPolicyDefintionResult => getPolicyDefintionResult.Id),
* Parameters = JsonSerializer.Serialize(new Dictionary
* {
* ["listOfAllowedLocations"] = new Dictionary
* {
* ["value"] = new[]
* {
* "East US",
* },
* },
* }),
* });
* var exampleGroupPolicyRemediation = new Azure.Management.GroupPolicyRemediation("example", new()
* {
* Name = "example",
* ManagementGroupId = exampleGroup.Id,
* PolicyAssignmentId = exampleGroupPolicyAssignment.Id,
* });
* });
* ```
* ```go
* package main
* import (
* "encoding/json"
* "github.com/pulumi/pulumi-azure/sdk/v5/go/azure/management"
* "github.com/pulumi/pulumi-azure/sdk/v5/go/azure/policy"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* exampleGroup, err := management.NewGroup(ctx, "example", &management.GroupArgs{
* DisplayName: pulumi.String("Example Management Group"),
* })
* if err != nil {
* return err
* }
* example, err := policy.GetPolicyDefintion(ctx, &policy.GetPolicyDefintionArgs{
* DisplayName: pulumi.StringRef("Allowed locations"),
* }, nil)
* if err != nil {
* return err
* }
* tmpJSON0, err := json.Marshal(map[string]interface{}{
* "listOfAllowedLocations": map[string]interface{}{
* "value": []string{
* "East US",
* },
* },
* })
* if err != nil {
* return err
* }
* json0 := string(tmpJSON0)
* exampleGroupPolicyAssignment, err := management.NewGroupPolicyAssignment(ctx, "example", &management.GroupPolicyAssignmentArgs{
* Name: pulumi.String("exampleAssignment"),
* ManagementGroupId: exampleGroup.ID(),
* PolicyDefinitionId: pulumi.String(example.Id),
* Parameters: pulumi.String(json0),
* })
* if err != nil {
* return err
* }
* _, err = management.NewGroupPolicyRemediation(ctx, "example", &management.GroupPolicyRemediationArgs{
* Name: pulumi.String("example"),
* ManagementGroupId: exampleGroup.ID(),
* PolicyAssignmentId: exampleGroupPolicyAssignment.ID(),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azure.management.Group;
* import com.pulumi.azure.management.GroupArgs;
* import com.pulumi.azure.policy.PolicyFunctions;
* import com.pulumi.azure.policy.inputs.GetPolicyDefintionArgs;
* import com.pulumi.azure.management.GroupPolicyAssignment;
* import com.pulumi.azure.management.GroupPolicyAssignmentArgs;
* import com.pulumi.azure.management.GroupPolicyRemediation;
* import com.pulumi.azure.management.GroupPolicyRemediationArgs;
* import static com.pulumi.codegen.internal.Serialization.*;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var exampleGroup = new Group("exampleGroup", GroupArgs.builder()
* .displayName("Example Management Group")
* .build());
* final var example = PolicyFunctions.getPolicyDefintion(GetPolicyDefintionArgs.builder()
* .displayName("Allowed locations")
* .build());
* var exampleGroupPolicyAssignment = new GroupPolicyAssignment("exampleGroupPolicyAssignment", GroupPolicyAssignmentArgs.builder()
* .name("exampleAssignment")
* .managementGroupId(exampleGroup.id())
* .policyDefinitionId(example.applyValue(getPolicyDefintionResult -> getPolicyDefintionResult.id()))
* .parameters(serializeJson(
* jsonObject(
* jsonProperty("listOfAllowedLocations", jsonObject(
* jsonProperty("value", jsonArray("East US"))
* ))
* )))
* .build());
* var exampleGroupPolicyRemediation = new GroupPolicyRemediation("exampleGroupPolicyRemediation", GroupPolicyRemediationArgs.builder()
* .name("example")
* .managementGroupId(exampleGroup.id())
* .policyAssignmentId(exampleGroupPolicyAssignment.id())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* exampleGroup:
* type: azure:management:Group
* name: example
* properties:
* displayName: Example Management Group
* exampleGroupPolicyAssignment:
* type: azure:management:GroupPolicyAssignment
* name: example
* properties:
* name: exampleAssignment
* managementGroupId: ${exampleGroup.id}
* policyDefinitionId: ${example.id}
* parameters:
* fn::toJSON:
* listOfAllowedLocations:
* value:
* - East US
* exampleGroupPolicyRemediation:
* type: azure:management:GroupPolicyRemediation
* name: example
* properties:
* name: example
* managementGroupId: ${exampleGroup.id}
* policyAssignmentId: ${exampleGroupPolicyAssignment.id}
* variables:
* example:
* fn::invoke:
* Function: azure:policy:getPolicyDefintion
* Arguments:
* displayName: Allowed locations
* ```
*
* ## Import
* Policy Remediations can be imported using the `resource id`, e.g.
* ```sh
* $ pulumi import azure:management/groupPolicyRemediation:GroupPolicyRemediation example /providers/Microsoft.Management/managementGroups/my-mgmt-group-id/providers/Microsoft.PolicyInsights/remediations/remediation1
* ```
* @property failurePercentage A number between 0.0 to 1.0 representing the percentage failure threshold. The remediation will fail if the percentage of failed remediation operations (i.e. failed deployments) exceeds this threshold.
* @property locationFilters A list of the resource locations that will be remediated.
* @property managementGroupId The Management Group ID at which the Policy Remediation should be applied. Changing this forces a new resource to be created.
* @property name The name of the Policy Remediation. Changing this forces a new resource to be created.
* @property parallelDeployments Determines how many resources to remediate at any given time. Can be used to increase or reduce the pace of the remediation. If not provided, the default parallel deployments value is used.
* @property policyAssignmentId The ID of the Policy Assignment that should be remediated.
* @property policyDefinitionId The unique ID for the policy definition within the policy set definition that should be remediated. Required when the policy assignment being remediated assigns a policy set definition.
* > **Note:** This property has been deprecated and will be removed in version 4.0 of the provider in favour of `policy_definition_reference_id`.
* @property policyDefinitionReferenceId The unique ID for the policy definition reference within the policy set definition that should be remediated. Required when the policy assignment being remediated assigns a policy set definition.
* @property resourceCount Determines the max number of resources that can be remediated by the remediation job. If not provided, the default resource count is used.
* @property resourceDiscoveryMode The way that resources to remediate are discovered. Possible values are `ExistingNonCompliant`, `ReEvaluateCompliance`. Defaults to `ExistingNonCompliant`.
* > **Note:** This property has been deprecated and will be removed in version 4.0 of the provider as evaluating compliance before remediation is only supported at subscription scope and below.
*/
public data class GroupPolicyRemediationArgs(
public val failurePercentage: Output? = null,
public val locationFilters: Output>? = null,
public val managementGroupId: Output? = null,
public val name: Output? = null,
public val parallelDeployments: Output? = null,
public val policyAssignmentId: Output? = null,
@Deprecated(
message = """
`policy_definition_id` will be removed in version 4.0 of the AzureRM Provider in favour of
`policy_definition_reference_id`.
""",
)
public val policyDefinitionId: Output? = null,
public val policyDefinitionReferenceId: Output? = null,
public val resourceCount: Output? = null,
@Deprecated(
message = """
`resource_discovery_mode` will be removed in version 4.0 of the AzureRM Provider as evaluating
compliance before remediation is only supported at subscription scope and below.
""",
)
public val resourceDiscoveryMode: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azure.management.GroupPolicyRemediationArgs =
com.pulumi.azure.management.GroupPolicyRemediationArgs.builder()
.failurePercentage(failurePercentage?.applyValue({ args0 -> args0 }))
.locationFilters(locationFilters?.applyValue({ args0 -> args0.map({ args0 -> args0 }) }))
.managementGroupId(managementGroupId?.applyValue({ args0 -> args0 }))
.name(name?.applyValue({ args0 -> args0 }))
.parallelDeployments(parallelDeployments?.applyValue({ args0 -> args0 }))
.policyAssignmentId(policyAssignmentId?.applyValue({ args0 -> args0 }))
.policyDefinitionId(policyDefinitionId?.applyValue({ args0 -> args0 }))
.policyDefinitionReferenceId(policyDefinitionReferenceId?.applyValue({ args0 -> args0 }))
.resourceCount(resourceCount?.applyValue({ args0 -> args0 }))
.resourceDiscoveryMode(resourceDiscoveryMode?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [GroupPolicyRemediationArgs].
*/
@PulumiTagMarker
public class GroupPolicyRemediationArgsBuilder internal constructor() {
private var failurePercentage: Output? = null
private var locationFilters: Output>? = null
private var managementGroupId: Output? = null
private var name: Output? = null
private var parallelDeployments: Output? = null
private var policyAssignmentId: Output? = null
private var policyDefinitionId: Output? = null
private var policyDefinitionReferenceId: Output? = null
private var resourceCount: Output? = null
private var resourceDiscoveryMode: Output? = null
/**
* @param value A number between 0.0 to 1.0 representing the percentage failure threshold. The remediation will fail if the percentage of failed remediation operations (i.e. failed deployments) exceeds this threshold.
*/
@JvmName("ixyggqjbybnsorgr")
public suspend fun failurePercentage(`value`: Output) {
this.failurePercentage = value
}
/**
* @param value A list of the resource locations that will be remediated.
*/
@JvmName("ssqxqlpqmxdqpnys")
public suspend fun locationFilters(`value`: Output>) {
this.locationFilters = value
}
@JvmName("eppogkjgxnbefmcq")
public suspend fun locationFilters(vararg values: Output) {
this.locationFilters = Output.all(values.asList())
}
/**
* @param values A list of the resource locations that will be remediated.
*/
@JvmName("daoahxewkcwqvqjp")
public suspend fun locationFilters(values: List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy