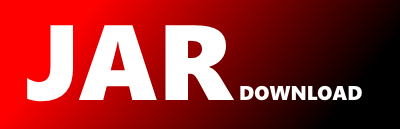
com.pulumi.azure.media.kotlin.LiveEvent.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-kotlin Show documentation
Show all versions of pulumi-azure-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azure.media.kotlin
import com.pulumi.azure.media.kotlin.outputs.LiveEventCrossSiteAccessPolicy
import com.pulumi.azure.media.kotlin.outputs.LiveEventEncoding
import com.pulumi.azure.media.kotlin.outputs.LiveEventInput
import com.pulumi.azure.media.kotlin.outputs.LiveEventPreview
import com.pulumi.core.Output
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.collections.Map
import com.pulumi.azure.media.kotlin.outputs.LiveEventCrossSiteAccessPolicy.Companion.toKotlin as liveEventCrossSiteAccessPolicyToKotlin
import com.pulumi.azure.media.kotlin.outputs.LiveEventEncoding.Companion.toKotlin as liveEventEncodingToKotlin
import com.pulumi.azure.media.kotlin.outputs.LiveEventInput.Companion.toKotlin as liveEventInputToKotlin
import com.pulumi.azure.media.kotlin.outputs.LiveEventPreview.Companion.toKotlin as liveEventPreviewToKotlin
/**
* Builder for [LiveEvent].
*/
@PulumiTagMarker
public class LiveEventResourceBuilder internal constructor() {
public var name: String? = null
public var args: LiveEventArgs = LiveEventArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend LiveEventArgsBuilder.() -> Unit) {
val builder = LiveEventArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): LiveEvent {
val builtJavaResource = com.pulumi.azure.media.LiveEvent(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return LiveEvent(builtJavaResource)
}
}
/**
* Manages a Live Event.
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as azure from "@pulumi/azure";
* const example = new azure.core.ResourceGroup("example", {
* name: "media-resources",
* location: "West Europe",
* });
* const exampleAccount = new azure.storage.Account("example", {
* name: "examplestoracc",
* resourceGroupName: example.name,
* location: example.location,
* accountTier: "Standard",
* accountReplicationType: "GRS",
* });
* const exampleServiceAccount = new azure.media.ServiceAccount("example", {
* name: "examplemediaacc",
* location: example.location,
* resourceGroupName: example.name,
* storageAccounts: [{
* id: exampleAccount.id,
* isPrimary: true,
* }],
* });
* const exampleLiveEvent = new azure.media.LiveEvent("example", {
* name: "example",
* resourceGroupName: example.name,
* location: example.location,
* mediaServicesAccountName: exampleServiceAccount.name,
* description: "My Event Description",
* input: {
* streamingProtocol: "RTMP",
* ipAccessControlAllows: [{
* name: "AllowAll",
* address: "0.0.0.0",
* subnetPrefixLength: 0,
* }],
* },
* encoding: {
* type: "Standard",
* presetName: "Default720p",
* stretchMode: "AutoFit",
* keyFrameInterval: "PT2S",
* },
* preview: {
* ipAccessControlAllows: [{
* name: "AllowAll",
* address: "0.0.0.0",
* subnetPrefixLength: 0,
* }],
* },
* streamOptions: ["LowLatency"],
* useStaticHostname: true,
* hostnamePrefix: "special-event",
* transcriptionLanguages: ["en-US"],
* });
* ```
* ```python
* import pulumi
* import pulumi_azure as azure
* example = azure.core.ResourceGroup("example",
* name="media-resources",
* location="West Europe")
* example_account = azure.storage.Account("example",
* name="examplestoracc",
* resource_group_name=example.name,
* location=example.location,
* account_tier="Standard",
* account_replication_type="GRS")
* example_service_account = azure.media.ServiceAccount("example",
* name="examplemediaacc",
* location=example.location,
* resource_group_name=example.name,
* storage_accounts=[azure.media.ServiceAccountStorageAccountArgs(
* id=example_account.id,
* is_primary=True,
* )])
* example_live_event = azure.media.LiveEvent("example",
* name="example",
* resource_group_name=example.name,
* location=example.location,
* media_services_account_name=example_service_account.name,
* description="My Event Description",
* input=azure.media.LiveEventInputArgs(
* streaming_protocol="RTMP",
* ip_access_control_allows=[azure.media.LiveEventInputIpAccessControlAllowArgs(
* name="AllowAll",
* address="0.0.0.0",
* subnet_prefix_length=0,
* )],
* ),
* encoding=azure.media.LiveEventEncodingArgs(
* type="Standard",
* preset_name="Default720p",
* stretch_mode="AutoFit",
* key_frame_interval="PT2S",
* ),
* preview=azure.media.LiveEventPreviewArgs(
* ip_access_control_allows=[azure.media.LiveEventPreviewIpAccessControlAllowArgs(
* name="AllowAll",
* address="0.0.0.0",
* subnet_prefix_length=0,
* )],
* ),
* stream_options=["LowLatency"],
* use_static_hostname=True,
* hostname_prefix="special-event",
* transcription_languages=["en-US"])
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Azure = Pulumi.Azure;
* return await Deployment.RunAsync(() =>
* {
* var example = new Azure.Core.ResourceGroup("example", new()
* {
* Name = "media-resources",
* Location = "West Europe",
* });
* var exampleAccount = new Azure.Storage.Account("example", new()
* {
* Name = "examplestoracc",
* ResourceGroupName = example.Name,
* Location = example.Location,
* AccountTier = "Standard",
* AccountReplicationType = "GRS",
* });
* var exampleServiceAccount = new Azure.Media.ServiceAccount("example", new()
* {
* Name = "examplemediaacc",
* Location = example.Location,
* ResourceGroupName = example.Name,
* StorageAccounts = new[]
* {
* new Azure.Media.Inputs.ServiceAccountStorageAccountArgs
* {
* Id = exampleAccount.Id,
* IsPrimary = true,
* },
* },
* });
* var exampleLiveEvent = new Azure.Media.LiveEvent("example", new()
* {
* Name = "example",
* ResourceGroupName = example.Name,
* Location = example.Location,
* MediaServicesAccountName = exampleServiceAccount.Name,
* Description = "My Event Description",
* Input = new Azure.Media.Inputs.LiveEventInputArgs
* {
* StreamingProtocol = "RTMP",
* IpAccessControlAllows = new[]
* {
* new Azure.Media.Inputs.LiveEventInputIpAccessControlAllowArgs
* {
* Name = "AllowAll",
* Address = "0.0.0.0",
* SubnetPrefixLength = 0,
* },
* },
* },
* Encoding = new Azure.Media.Inputs.LiveEventEncodingArgs
* {
* Type = "Standard",
* PresetName = "Default720p",
* StretchMode = "AutoFit",
* KeyFrameInterval = "PT2S",
* },
* Preview = new Azure.Media.Inputs.LiveEventPreviewArgs
* {
* IpAccessControlAllows = new[]
* {
* new Azure.Media.Inputs.LiveEventPreviewIpAccessControlAllowArgs
* {
* Name = "AllowAll",
* Address = "0.0.0.0",
* SubnetPrefixLength = 0,
* },
* },
* },
* StreamOptions = new[]
* {
* "LowLatency",
* },
* UseStaticHostname = true,
* HostnamePrefix = "special-event",
* TranscriptionLanguages = new[]
* {
* "en-US",
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-azure/sdk/v5/go/azure/core"
* "github.com/pulumi/pulumi-azure/sdk/v5/go/azure/media"
* "github.com/pulumi/pulumi-azure/sdk/v5/go/azure/storage"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* example, err := core.NewResourceGroup(ctx, "example", &core.ResourceGroupArgs{
* Name: pulumi.String("media-resources"),
* Location: pulumi.String("West Europe"),
* })
* if err != nil {
* return err
* }
* exampleAccount, err := storage.NewAccount(ctx, "example", &storage.AccountArgs{
* Name: pulumi.String("examplestoracc"),
* ResourceGroupName: example.Name,
* Location: example.Location,
* AccountTier: pulumi.String("Standard"),
* AccountReplicationType: pulumi.String("GRS"),
* })
* if err != nil {
* return err
* }
* exampleServiceAccount, err := media.NewServiceAccount(ctx, "example", &media.ServiceAccountArgs{
* Name: pulumi.String("examplemediaacc"),
* Location: example.Location,
* ResourceGroupName: example.Name,
* StorageAccounts: media.ServiceAccountStorageAccountArray{
* &media.ServiceAccountStorageAccountArgs{
* Id: exampleAccount.ID(),
* IsPrimary: pulumi.Bool(true),
* },
* },
* })
* if err != nil {
* return err
* }
* _, err = media.NewLiveEvent(ctx, "example", &media.LiveEventArgs{
* Name: pulumi.String("example"),
* ResourceGroupName: example.Name,
* Location: example.Location,
* MediaServicesAccountName: exampleServiceAccount.Name,
* Description: pulumi.String("My Event Description"),
* Input: &media.LiveEventInputTypeArgs{
* StreamingProtocol: pulumi.String("RTMP"),
* IpAccessControlAllows: media.LiveEventInputIpAccessControlAllowArray{
* &media.LiveEventInputIpAccessControlAllowArgs{
* Name: pulumi.String("AllowAll"),
* Address: pulumi.String("0.0.0.0"),
* SubnetPrefixLength: pulumi.Int(0),
* },
* },
* },
* Encoding: &media.LiveEventEncodingArgs{
* Type: pulumi.String("Standard"),
* PresetName: pulumi.String("Default720p"),
* StretchMode: pulumi.String("AutoFit"),
* KeyFrameInterval: pulumi.String("PT2S"),
* },
* Preview: &media.LiveEventPreviewArgs{
* IpAccessControlAllows: media.LiveEventPreviewIpAccessControlAllowArray{
* &media.LiveEventPreviewIpAccessControlAllowArgs{
* Name: pulumi.String("AllowAll"),
* Address: pulumi.String("0.0.0.0"),
* SubnetPrefixLength: pulumi.Int(0),
* },
* },
* },
* StreamOptions: pulumi.StringArray{
* pulumi.String("LowLatency"),
* },
* UseStaticHostname: pulumi.Bool(true),
* HostnamePrefix: pulumi.String("special-event"),
* TranscriptionLanguages: pulumi.StringArray{
* pulumi.String("en-US"),
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azure.core.ResourceGroup;
* import com.pulumi.azure.core.ResourceGroupArgs;
* import com.pulumi.azure.storage.Account;
* import com.pulumi.azure.storage.AccountArgs;
* import com.pulumi.azure.media.ServiceAccount;
* import com.pulumi.azure.media.ServiceAccountArgs;
* import com.pulumi.azure.media.inputs.ServiceAccountStorageAccountArgs;
* import com.pulumi.azure.media.LiveEvent;
* import com.pulumi.azure.media.LiveEventArgs;
* import com.pulumi.azure.media.inputs.LiveEventInputArgs;
* import com.pulumi.azure.media.inputs.LiveEventEncodingArgs;
* import com.pulumi.azure.media.inputs.LiveEventPreviewArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var example = new ResourceGroup("example", ResourceGroupArgs.builder()
* .name("media-resources")
* .location("West Europe")
* .build());
* var exampleAccount = new Account("exampleAccount", AccountArgs.builder()
* .name("examplestoracc")
* .resourceGroupName(example.name())
* .location(example.location())
* .accountTier("Standard")
* .accountReplicationType("GRS")
* .build());
* var exampleServiceAccount = new ServiceAccount("exampleServiceAccount", ServiceAccountArgs.builder()
* .name("examplemediaacc")
* .location(example.location())
* .resourceGroupName(example.name())
* .storageAccounts(ServiceAccountStorageAccountArgs.builder()
* .id(exampleAccount.id())
* .isPrimary(true)
* .build())
* .build());
* var exampleLiveEvent = new LiveEvent("exampleLiveEvent", LiveEventArgs.builder()
* .name("example")
* .resourceGroupName(example.name())
* .location(example.location())
* .mediaServicesAccountName(exampleServiceAccount.name())
* .description("My Event Description")
* .input(LiveEventInputArgs.builder()
* .streamingProtocol("RTMP")
* .ipAccessControlAllows(LiveEventInputIpAccessControlAllowArgs.builder()
* .name("AllowAll")
* .address("0.0.0.0")
* .subnetPrefixLength(0)
* .build())
* .build())
* .encoding(LiveEventEncodingArgs.builder()
* .type("Standard")
* .presetName("Default720p")
* .stretchMode("AutoFit")
* .keyFrameInterval("PT2S")
* .build())
* .preview(LiveEventPreviewArgs.builder()
* .ipAccessControlAllows(LiveEventPreviewIpAccessControlAllowArgs.builder()
* .name("AllowAll")
* .address("0.0.0.0")
* .subnetPrefixLength(0)
* .build())
* .build())
* .streamOptions("LowLatency")
* .useStaticHostname(true)
* .hostnamePrefix("special-event")
* .transcriptionLanguages("en-US")
* .build());
* }
* }
* ```
* ```yaml
* resources:
* example:
* type: azure:core:ResourceGroup
* properties:
* name: media-resources
* location: West Europe
* exampleAccount:
* type: azure:storage:Account
* name: example
* properties:
* name: examplestoracc
* resourceGroupName: ${example.name}
* location: ${example.location}
* accountTier: Standard
* accountReplicationType: GRS
* exampleServiceAccount:
* type: azure:media:ServiceAccount
* name: example
* properties:
* name: examplemediaacc
* location: ${example.location}
* resourceGroupName: ${example.name}
* storageAccounts:
* - id: ${exampleAccount.id}
* isPrimary: true
* exampleLiveEvent:
* type: azure:media:LiveEvent
* name: example
* properties:
* name: example
* resourceGroupName: ${example.name}
* location: ${example.location}
* mediaServicesAccountName: ${exampleServiceAccount.name}
* description: My Event Description
* input:
* streamingProtocol: RTMP
* ipAccessControlAllows:
* - name: AllowAll
* address: 0.0.0.0
* subnetPrefixLength: 0
* encoding:
* type: Standard
* presetName: Default720p
* stretchMode: AutoFit
* keyFrameInterval: PT2S
* preview:
* ipAccessControlAllows:
* - name: AllowAll
* address: 0.0.0.0
* subnetPrefixLength: 0
* streamOptions:
* - LowLatency
* useStaticHostname: true
* hostnamePrefix: special-event
* transcriptionLanguages:
* - en-US
* ```
*
* ## Import
* Live Events can be imported using the `resource id`, e.g.
* ```sh
* $ pulumi import azure:media/liveEvent:LiveEvent example /subscriptions/00000000-0000-0000-0000-000000000000/resourceGroups/resGroup1/providers/Microsoft.Media/mediaServices/account1/liveEvents/event1
* ```
*/
public class LiveEvent internal constructor(
override val javaResource: com.pulumi.azure.media.LiveEvent,
) : KotlinCustomResource(javaResource, LiveEventMapper) {
/**
* The flag indicates if the resource should be automatically started on creation. Changing this forces a new resource to be created.
*/
public val autoStartEnabled: Output?
get() = javaResource.autoStartEnabled().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* A `cross_site_access_policy` block as defined below.
*/
public val crossSiteAccessPolicy: Output?
get() = javaResource.crossSiteAccessPolicy().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> liveEventCrossSiteAccessPolicyToKotlin(args0) })
}).orElse(null)
})
/**
* A description for the live event.
*/
public val description: Output?
get() = javaResource.description().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* A `encoding` block as defined below.
*/
public val encoding: Output?
get() = javaResource.encoding().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
liveEventEncodingToKotlin(args0)
})
}).orElse(null)
})
/**
* When `use_static_hostname` is set to true, the `hostname_prefix` specifies the first part of the hostname assigned to the live event preview and ingest endpoints. The final hostname would be a combination of this prefix, the media service account name and a short code for the Azure Media Services data center.
*/
public val hostnamePrefix: Output?
get() = javaResource.hostnamePrefix().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* A `input` block as defined below.
*/
public val input: Output
get() = javaResource.input().applyValue({ args0 ->
args0.let({ args0 ->
liveEventInputToKotlin(args0)
})
})
/**
* The Azure Region where the Live Event should exist. Changing this forces a new Live Event to be created.
*/
public val location: Output
get() = javaResource.location().applyValue({ args0 -> args0 })
/**
* The Media Services account name. Changing this forces a new Live Event to be created.
*/
public val mediaServicesAccountName: Output
get() = javaResource.mediaServicesAccountName().applyValue({ args0 -> args0 })
/**
* The name which should be used for this Live Event. Changing this forces a new Live Event to be created.
*/
public val name: Output
get() = javaResource.name().applyValue({ args0 -> args0 })
/**
* A `preview` block as defined below.
*/
public val preview: Output
get() = javaResource.preview().applyValue({ args0 ->
args0.let({ args0 ->
liveEventPreviewToKotlin(args0)
})
})
/**
* The name of the Resource Group where the Live Event should exist. Changing this forces a new Live Event to be created.
*/
public val resourceGroupName: Output
get() = javaResource.resourceGroupName().applyValue({ args0 -> args0 })
/**
* A list of options to use for the LiveEvent. Possible values are `Default`, `LowLatency`, `LowLatencyV2`. Please see more at this [document](https://learn.microsoft.com/en-us/azure/media-services/latest/live-event-latency-reference#lowlatency-and-lowlatencyv2-options). Changing this forces a new resource to be created.
*/
public val streamOptions: Output>?
get() = javaResource.streamOptions().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 -> args0 })
}).orElse(null)
})
/**
* A mapping of tags which should be assigned to the Live Event.
*/
public val tags: Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy