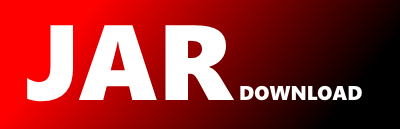
com.pulumi.azure.monitoring.kotlin.DataCollectionRuleArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-kotlin Show documentation
Show all versions of pulumi-azure-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azure.monitoring.kotlin
import com.pulumi.azure.monitoring.DataCollectionRuleArgs.builder
import com.pulumi.azure.monitoring.kotlin.inputs.DataCollectionRuleDataFlowArgs
import com.pulumi.azure.monitoring.kotlin.inputs.DataCollectionRuleDataFlowArgsBuilder
import com.pulumi.azure.monitoring.kotlin.inputs.DataCollectionRuleDataSourcesArgs
import com.pulumi.azure.monitoring.kotlin.inputs.DataCollectionRuleDataSourcesArgsBuilder
import com.pulumi.azure.monitoring.kotlin.inputs.DataCollectionRuleDestinationsArgs
import com.pulumi.azure.monitoring.kotlin.inputs.DataCollectionRuleDestinationsArgsBuilder
import com.pulumi.azure.monitoring.kotlin.inputs.DataCollectionRuleIdentityArgs
import com.pulumi.azure.monitoring.kotlin.inputs.DataCollectionRuleIdentityArgsBuilder
import com.pulumi.azure.monitoring.kotlin.inputs.DataCollectionRuleStreamDeclarationArgs
import com.pulumi.azure.monitoring.kotlin.inputs.DataCollectionRuleStreamDeclarationArgsBuilder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Pair
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.collections.Map
import kotlin.jvm.JvmName
/**
* Manages a Data Collection Rule.
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as azure from "@pulumi/azure";
* const example = new azure.core.ResourceGroup("example", {
* name: "example-resources",
* location: "West Europe",
* });
* const exampleUserAssignedIdentity = new azure.authorization.UserAssignedIdentity("example", {
* name: "example-uai",
* resourceGroupName: example.name,
* location: example.location,
* });
* const exampleAnalyticsWorkspace = new azure.operationalinsights.AnalyticsWorkspace("example", {
* name: "example-workspace",
* resourceGroupName: example.name,
* location: example.location,
* });
* const exampleAnalyticsSolution = new azure.operationalinsights.AnalyticsSolution("example", {
* solutionName: "WindowsEventForwarding",
* location: example.location,
* resourceGroupName: example.name,
* workspaceResourceId: exampleAnalyticsWorkspace.id,
* workspaceName: exampleAnalyticsWorkspace.name,
* plan: {
* publisher: "Microsoft",
* product: "OMSGallery/WindowsEventForwarding",
* },
* });
* const exampleEventHubNamespace = new azure.eventhub.EventHubNamespace("example", {
* name: "exeventns",
* location: example.location,
* resourceGroupName: example.name,
* sku: "Standard",
* capacity: 1,
* });
* const exampleEventHub = new azure.eventhub.EventHub("example", {
* name: "exevent2",
* namespaceName: exampleEventHubNamespace.name,
* resourceGroupName: example.name,
* partitionCount: 2,
* messageRetention: 1,
* });
* const exampleAccount = new azure.storage.Account("example", {
* name: "examstorage",
* resourceGroupName: example.name,
* location: example.location,
* accountTier: "Standard",
* accountReplicationType: "LRS",
* });
* const exampleContainer = new azure.storage.Container("example", {
* name: "examplecontainer",
* storageAccountName: exampleAccount.name,
* containerAccessType: "private",
* });
* const exampleDataCollectionEndpoint = new azure.monitoring.DataCollectionEndpoint("example", {
* name: "example-dcre",
* resourceGroupName: example.name,
* location: example.location,
* });
* const exampleDataCollectionRule = new azure.monitoring.DataCollectionRule("example", {
* name: "example-rule",
* resourceGroupName: example.name,
* location: example.location,
* dataCollectionEndpointId: exampleDataCollectionEndpoint.id,
* destinations: {
* logAnalytics: [{
* workspaceResourceId: exampleAnalyticsWorkspace.id,
* name: "example-destination-log",
* }],
* eventHub: {
* eventHubId: exampleEventHub.id,
* name: "example-destination-eventhub",
* },
* storageBlobs: [{
* storageAccountId: exampleAccount.id,
* containerName: exampleContainer.name,
* name: "example-destination-storage",
* }],
* azureMonitorMetrics: {
* name: "example-destination-metrics",
* },
* },
* dataFlows: [
* {
* streams: ["Microsoft-InsightsMetrics"],
* destinations: ["example-destination-metrics"],
* },
* {
* streams: [
* "Microsoft-InsightsMetrics",
* "Microsoft-Syslog",
* "Microsoft-Perf",
* ],
* destinations: ["example-destination-log"],
* },
* {
* streams: ["Custom-MyTableRawData"],
* destinations: ["example-destination-log"],
* outputStream: "Microsoft-Syslog",
* transformKql: "source | project TimeGenerated = Time, Computer, Message = AdditionalContext",
* },
* ],
* dataSources: {
* syslogs: [{
* facilityNames: ["*"],
* logLevels: ["*"],
* name: "example-datasource-syslog",
* streams: ["Microsoft-Syslog"],
* }],
* iisLogs: [{
* streams: ["Microsoft-W3CIISLog"],
* name: "example-datasource-iis",
* logDirectories: ["C:\\Logs\\W3SVC1"],
* }],
* logFiles: [{
* name: "example-datasource-logfile",
* format: "text",
* streams: ["Custom-MyTableRawData"],
* filePatterns: ["C:\\JavaLogs\\*.log"],
* settings: {
* text: {
* recordStartTimestampFormat: "ISO 8601",
* },
* },
* }],
* performanceCounters: [{
* streams: [
* "Microsoft-Perf",
* "Microsoft-InsightsMetrics",
* ],
* samplingFrequencyInSeconds: 60,
* counterSpecifiers: ["Processor(*)\\% Processor Time"],
* name: "example-datasource-perfcounter",
* }],
* windowsEventLogs: [{
* streams: ["Microsoft-WindowsEvent"],
* xPathQueries: ["*![System/Level=1]"],
* name: "example-datasource-wineventlog",
* }],
* extensions: [{
* streams: ["Microsoft-WindowsEvent"],
* inputDataSources: ["example-datasource-wineventlog"],
* extensionName: "example-extension-name",
* extensionJson: JSON.stringify({
* a: 1,
* b: "hello",
* }),
* name: "example-datasource-extension",
* }],
* },
* streamDeclarations: [{
* streamName: "Custom-MyTableRawData",
* columns: [
* {
* name: "Time",
* type: "datetime",
* },
* {
* name: "Computer",
* type: "string",
* },
* {
* name: "AdditionalContext",
* type: "string",
* },
* ],
* }],
* identity: {
* type: "UserAssigned",
* identityIds: [exampleUserAssignedIdentity.id],
* },
* description: "data collection rule example",
* tags: {
* foo: "bar",
* },
* });
* ```
* ```python
* import pulumi
* import json
* import pulumi_azure as azure
* example = azure.core.ResourceGroup("example",
* name="example-resources",
* location="West Europe")
* example_user_assigned_identity = azure.authorization.UserAssignedIdentity("example",
* name="example-uai",
* resource_group_name=example.name,
* location=example.location)
* example_analytics_workspace = azure.operationalinsights.AnalyticsWorkspace("example",
* name="example-workspace",
* resource_group_name=example.name,
* location=example.location)
* example_analytics_solution = azure.operationalinsights.AnalyticsSolution("example",
* solution_name="WindowsEventForwarding",
* location=example.location,
* resource_group_name=example.name,
* workspace_resource_id=example_analytics_workspace.id,
* workspace_name=example_analytics_workspace.name,
* plan=azure.operationalinsights.AnalyticsSolutionPlanArgs(
* publisher="Microsoft",
* product="OMSGallery/WindowsEventForwarding",
* ))
* example_event_hub_namespace = azure.eventhub.EventHubNamespace("example",
* name="exeventns",
* location=example.location,
* resource_group_name=example.name,
* sku="Standard",
* capacity=1)
* example_event_hub = azure.eventhub.EventHub("example",
* name="exevent2",
* namespace_name=example_event_hub_namespace.name,
* resource_group_name=example.name,
* partition_count=2,
* message_retention=1)
* example_account = azure.storage.Account("example",
* name="examstorage",
* resource_group_name=example.name,
* location=example.location,
* account_tier="Standard",
* account_replication_type="LRS")
* example_container = azure.storage.Container("example",
* name="examplecontainer",
* storage_account_name=example_account.name,
* container_access_type="private")
* example_data_collection_endpoint = azure.monitoring.DataCollectionEndpoint("example",
* name="example-dcre",
* resource_group_name=example.name,
* location=example.location)
* example_data_collection_rule = azure.monitoring.DataCollectionRule("example",
* name="example-rule",
* resource_group_name=example.name,
* location=example.location,
* data_collection_endpoint_id=example_data_collection_endpoint.id,
* destinations=azure.monitoring.DataCollectionRuleDestinationsArgs(
* log_analytics=[azure.monitoring.DataCollectionRuleDestinationsLogAnalyticArgs(
* workspace_resource_id=example_analytics_workspace.id,
* name="example-destination-log",
* )],
* event_hub=azure.monitoring.DataCollectionRuleDestinationsEventHubArgs(
* event_hub_id=example_event_hub.id,
* name="example-destination-eventhub",
* ),
* storage_blobs=[azure.monitoring.DataCollectionRuleDestinationsStorageBlobArgs(
* storage_account_id=example_account.id,
* container_name=example_container.name,
* name="example-destination-storage",
* )],
* azure_monitor_metrics=azure.monitoring.DataCollectionRuleDestinationsAzureMonitorMetricsArgs(
* name="example-destination-metrics",
* ),
* ),
* data_flows=[
* azure.monitoring.DataCollectionRuleDataFlowArgs(
* streams=["Microsoft-InsightsMetrics"],
* destinations=["example-destination-metrics"],
* ),
* azure.monitoring.DataCollectionRuleDataFlowArgs(
* streams=[
* "Microsoft-InsightsMetrics",
* "Microsoft-Syslog",
* "Microsoft-Perf",
* ],
* destinations=["example-destination-log"],
* ),
* azure.monitoring.DataCollectionRuleDataFlowArgs(
* streams=["Custom-MyTableRawData"],
* destinations=["example-destination-log"],
* output_stream="Microsoft-Syslog",
* transform_kql="source | project TimeGenerated = Time, Computer, Message = AdditionalContext",
* ),
* ],
* data_sources=azure.monitoring.DataCollectionRuleDataSourcesArgs(
* syslogs=[azure.monitoring.DataCollectionRuleDataSourcesSyslogArgs(
* facility_names=["*"],
* log_levels=["*"],
* name="example-datasource-syslog",
* streams=["Microsoft-Syslog"],
* )],
* iis_logs=[azure.monitoring.DataCollectionRuleDataSourcesIisLogArgs(
* streams=["Microsoft-W3CIISLog"],
* name="example-datasource-iis",
* log_directories=["C:\\Logs\\W3SVC1"],
* )],
* log_files=[azure.monitoring.DataCollectionRuleDataSourcesLogFileArgs(
* name="example-datasource-logfile",
* format="text",
* streams=["Custom-MyTableRawData"],
* file_patterns=["C:\\JavaLogs\\*.log"],
* settings=azure.monitoring.DataCollectionRuleDataSourcesLogFileSettingsArgs(
* text=azure.monitoring.DataCollectionRuleDataSourcesLogFileSettingsTextArgs(
* record_start_timestamp_format="ISO 8601",
* ),
* ),
* )],
* performance_counters=[azure.monitoring.DataCollectionRuleDataSourcesPerformanceCounterArgs(
* streams=[
* "Microsoft-Perf",
* "Microsoft-InsightsMetrics",
* ],
* sampling_frequency_in_seconds=60,
* counter_specifiers=["Processor(*)\\% Processor Time"],
* name="example-datasource-perfcounter",
* )],
* windows_event_logs=[azure.monitoring.DataCollectionRuleDataSourcesWindowsEventLogArgs(
* streams=["Microsoft-WindowsEvent"],
* x_path_queries=["*![System/Level=1]"],
* name="example-datasource-wineventlog",
* )],
* extensions=[azure.monitoring.DataCollectionRuleDataSourcesExtensionArgs(
* streams=["Microsoft-WindowsEvent"],
* input_data_sources=["example-datasource-wineventlog"],
* extension_name="example-extension-name",
* extension_json=json.dumps({
* "a": 1,
* "b": "hello",
* }),
* name="example-datasource-extension",
* )],
* ),
* stream_declarations=[azure.monitoring.DataCollectionRuleStreamDeclarationArgs(
* stream_name="Custom-MyTableRawData",
* columns=[
* azure.monitoring.DataCollectionRuleStreamDeclarationColumnArgs(
* name="Time",
* type="datetime",
* ),
* azure.monitoring.DataCollectionRuleStreamDeclarationColumnArgs(
* name="Computer",
* type="string",
* ),
* azure.monitoring.DataCollectionRuleStreamDeclarationColumnArgs(
* name="AdditionalContext",
* type="string",
* ),
* ],
* )],
* identity=azure.monitoring.DataCollectionRuleIdentityArgs(
* type="UserAssigned",
* identity_ids=[example_user_assigned_identity.id],
* ),
* description="data collection rule example",
* tags={
* "foo": "bar",
* })
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using System.Text.Json;
* using Pulumi;
* using Azure = Pulumi.Azure;
* return await Deployment.RunAsync(() =>
* {
* var example = new Azure.Core.ResourceGroup("example", new()
* {
* Name = "example-resources",
* Location = "West Europe",
* });
* var exampleUserAssignedIdentity = new Azure.Authorization.UserAssignedIdentity("example", new()
* {
* Name = "example-uai",
* ResourceGroupName = example.Name,
* Location = example.Location,
* });
* var exampleAnalyticsWorkspace = new Azure.OperationalInsights.AnalyticsWorkspace("example", new()
* {
* Name = "example-workspace",
* ResourceGroupName = example.Name,
* Location = example.Location,
* });
* var exampleAnalyticsSolution = new Azure.OperationalInsights.AnalyticsSolution("example", new()
* {
* SolutionName = "WindowsEventForwarding",
* Location = example.Location,
* ResourceGroupName = example.Name,
* WorkspaceResourceId = exampleAnalyticsWorkspace.Id,
* WorkspaceName = exampleAnalyticsWorkspace.Name,
* Plan = new Azure.OperationalInsights.Inputs.AnalyticsSolutionPlanArgs
* {
* Publisher = "Microsoft",
* Product = "OMSGallery/WindowsEventForwarding",
* },
* });
* var exampleEventHubNamespace = new Azure.EventHub.EventHubNamespace("example", new()
* {
* Name = "exeventns",
* Location = example.Location,
* ResourceGroupName = example.Name,
* Sku = "Standard",
* Capacity = 1,
* });
* var exampleEventHub = new Azure.EventHub.EventHub("example", new()
* {
* Name = "exevent2",
* NamespaceName = exampleEventHubNamespace.Name,
* ResourceGroupName = example.Name,
* PartitionCount = 2,
* MessageRetention = 1,
* });
* var exampleAccount = new Azure.Storage.Account("example", new()
* {
* Name = "examstorage",
* ResourceGroupName = example.Name,
* Location = example.Location,
* AccountTier = "Standard",
* AccountReplicationType = "LRS",
* });
* var exampleContainer = new Azure.Storage.Container("example", new()
* {
* Name = "examplecontainer",
* StorageAccountName = exampleAccount.Name,
* ContainerAccessType = "private",
* });
* var exampleDataCollectionEndpoint = new Azure.Monitoring.DataCollectionEndpoint("example", new()
* {
* Name = "example-dcre",
* ResourceGroupName = example.Name,
* Location = example.Location,
* });
* var exampleDataCollectionRule = new Azure.Monitoring.DataCollectionRule("example", new()
* {
* Name = "example-rule",
* ResourceGroupName = example.Name,
* Location = example.Location,
* DataCollectionEndpointId = exampleDataCollectionEndpoint.Id,
* Destinations = new Azure.Monitoring.Inputs.DataCollectionRuleDestinationsArgs
* {
* LogAnalytics = new[]
* {
* new Azure.Monitoring.Inputs.DataCollectionRuleDestinationsLogAnalyticArgs
* {
* WorkspaceResourceId = exampleAnalyticsWorkspace.Id,
* Name = "example-destination-log",
* },
* },
* EventHub = new Azure.Monitoring.Inputs.DataCollectionRuleDestinationsEventHubArgs
* {
* EventHubId = exampleEventHub.Id,
* Name = "example-destination-eventhub",
* },
* StorageBlobs = new[]
* {
* new Azure.Monitoring.Inputs.DataCollectionRuleDestinationsStorageBlobArgs
* {
* StorageAccountId = exampleAccount.Id,
* ContainerName = exampleContainer.Name,
* Name = "example-destination-storage",
* },
* },
* AzureMonitorMetrics = new Azure.Monitoring.Inputs.DataCollectionRuleDestinationsAzureMonitorMetricsArgs
* {
* Name = "example-destination-metrics",
* },
* },
* DataFlows = new[]
* {
* new Azure.Monitoring.Inputs.DataCollectionRuleDataFlowArgs
* {
* Streams = new[]
* {
* "Microsoft-InsightsMetrics",
* },
* Destinations = new[]
* {
* "example-destination-metrics",
* },
* },
* new Azure.Monitoring.Inputs.DataCollectionRuleDataFlowArgs
* {
* Streams = new[]
* {
* "Microsoft-InsightsMetrics",
* "Microsoft-Syslog",
* "Microsoft-Perf",
* },
* Destinations = new[]
* {
* "example-destination-log",
* },
* },
* new Azure.Monitoring.Inputs.DataCollectionRuleDataFlowArgs
* {
* Streams = new[]
* {
* "Custom-MyTableRawData",
* },
* Destinations = new[]
* {
* "example-destination-log",
* },
* OutputStream = "Microsoft-Syslog",
* TransformKql = "source | project TimeGenerated = Time, Computer, Message = AdditionalContext",
* },
* },
* DataSources = new Azure.Monitoring.Inputs.DataCollectionRuleDataSourcesArgs
* {
* Syslogs = new[]
* {
* new Azure.Monitoring.Inputs.DataCollectionRuleDataSourcesSyslogArgs
* {
* FacilityNames = new[]
* {
* "*",
* },
* LogLevels = new[]
* {
* "*",
* },
* Name = "example-datasource-syslog",
* Streams = new[]
* {
* "Microsoft-Syslog",
* },
* },
* },
* IisLogs = new[]
* {
* new Azure.Monitoring.Inputs.DataCollectionRuleDataSourcesIisLogArgs
* {
* Streams = new[]
* {
* "Microsoft-W3CIISLog",
* },
* Name = "example-datasource-iis",
* LogDirectories = new[]
* {
* "C:\\Logs\\W3SVC1",
* },
* },
* },
* LogFiles = new[]
* {
* new Azure.Monitoring.Inputs.DataCollectionRuleDataSourcesLogFileArgs
* {
* Name = "example-datasource-logfile",
* Format = "text",
* Streams = new[]
* {
* "Custom-MyTableRawData",
* },
* FilePatterns = new[]
* {
* "C:\\JavaLogs\\*.log",
* },
* Settings = new Azure.Monitoring.Inputs.DataCollectionRuleDataSourcesLogFileSettingsArgs
* {
* Text = new Azure.Monitoring.Inputs.DataCollectionRuleDataSourcesLogFileSettingsTextArgs
* {
* RecordStartTimestampFormat = "ISO 8601",
* },
* },
* },
* },
* PerformanceCounters = new[]
* {
* new Azure.Monitoring.Inputs.DataCollectionRuleDataSourcesPerformanceCounterArgs
* {
* Streams = new[]
* {
* "Microsoft-Perf",
* "Microsoft-InsightsMetrics",
* },
* SamplingFrequencyInSeconds = 60,
* CounterSpecifiers = new[]
* {
* "Processor(*)\\% Processor Time",
* },
* Name = "example-datasource-perfcounter",
* },
* },
* WindowsEventLogs = new[]
* {
* new Azure.Monitoring.Inputs.DataCollectionRuleDataSourcesWindowsEventLogArgs
* {
* Streams = new[]
* {
* "Microsoft-WindowsEvent",
* },
* XPathQueries = new[]
* {
* "*![System/Level=1]",
* },
* Name = "example-datasource-wineventlog",
* },
* },
* Extensions = new[]
* {
* new Azure.Monitoring.Inputs.DataCollectionRuleDataSourcesExtensionArgs
* {
* Streams = new[]
* {
* "Microsoft-WindowsEvent",
* },
* InputDataSources = new[]
* {
* "example-datasource-wineventlog",
* },
* ExtensionName = "example-extension-name",
* ExtensionJson = JsonSerializer.Serialize(new Dictionary
* {
* ["a"] = 1,
* ["b"] = "hello",
* }),
* Name = "example-datasource-extension",
* },
* },
* },
* StreamDeclarations = new[]
* {
* new Azure.Monitoring.Inputs.DataCollectionRuleStreamDeclarationArgs
* {
* StreamName = "Custom-MyTableRawData",
* Columns = new[]
* {
* new Azure.Monitoring.Inputs.DataCollectionRuleStreamDeclarationColumnArgs
* {
* Name = "Time",
* Type = "datetime",
* },
* new Azure.Monitoring.Inputs.DataCollectionRuleStreamDeclarationColumnArgs
* {
* Name = "Computer",
* Type = "string",
* },
* new Azure.Monitoring.Inputs.DataCollectionRuleStreamDeclarationColumnArgs
* {
* Name = "AdditionalContext",
* Type = "string",
* },
* },
* },
* },
* Identity = new Azure.Monitoring.Inputs.DataCollectionRuleIdentityArgs
* {
* Type = "UserAssigned",
* IdentityIds = new[]
* {
* exampleUserAssignedIdentity.Id,
* },
* },
* Description = "data collection rule example",
* Tags =
* {
* { "foo", "bar" },
* },
* });
* });
* ```
* ```go
* package main
* import (
* "encoding/json"
* "github.com/pulumi/pulumi-azure/sdk/v5/go/azure/authorization"
* "github.com/pulumi/pulumi-azure/sdk/v5/go/azure/core"
* "github.com/pulumi/pulumi-azure/sdk/v5/go/azure/eventhub"
* "github.com/pulumi/pulumi-azure/sdk/v5/go/azure/monitoring"
* "github.com/pulumi/pulumi-azure/sdk/v5/go/azure/operationalinsights"
* "github.com/pulumi/pulumi-azure/sdk/v5/go/azure/storage"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* example, err := core.NewResourceGroup(ctx, "example", &core.ResourceGroupArgs{
* Name: pulumi.String("example-resources"),
* Location: pulumi.String("West Europe"),
* })
* if err != nil {
* return err
* }
* exampleUserAssignedIdentity, err := authorization.NewUserAssignedIdentity(ctx, "example", &authorization.UserAssignedIdentityArgs{
* Name: pulumi.String("example-uai"),
* ResourceGroupName: example.Name,
* Location: example.Location,
* })
* if err != nil {
* return err
* }
* exampleAnalyticsWorkspace, err := operationalinsights.NewAnalyticsWorkspace(ctx, "example", &operationalinsights.AnalyticsWorkspaceArgs{
* Name: pulumi.String("example-workspace"),
* ResourceGroupName: example.Name,
* Location: example.Location,
* })
* if err != nil {
* return err
* }
* _, err = operationalinsights.NewAnalyticsSolution(ctx, "example", &operationalinsights.AnalyticsSolutionArgs{
* SolutionName: pulumi.String("WindowsEventForwarding"),
* Location: example.Location,
* ResourceGroupName: example.Name,
* WorkspaceResourceId: exampleAnalyticsWorkspace.ID(),
* WorkspaceName: exampleAnalyticsWorkspace.Name,
* Plan: &operationalinsights.AnalyticsSolutionPlanArgs{
* Publisher: pulumi.String("Microsoft"),
* Product: pulumi.String("OMSGallery/WindowsEventForwarding"),
* },
* })
* if err != nil {
* return err
* }
* exampleEventHubNamespace, err := eventhub.NewEventHubNamespace(ctx, "example", &eventhub.EventHubNamespaceArgs{
* Name: pulumi.String("exeventns"),
* Location: example.Location,
* ResourceGroupName: example.Name,
* Sku: pulumi.String("Standard"),
* Capacity: pulumi.Int(1),
* })
* if err != nil {
* return err
* }
* exampleEventHub, err := eventhub.NewEventHub(ctx, "example", &eventhub.EventHubArgs{
* Name: pulumi.String("exevent2"),
* NamespaceName: exampleEventHubNamespace.Name,
* ResourceGroupName: example.Name,
* PartitionCount: pulumi.Int(2),
* MessageRetention: pulumi.Int(1),
* })
* if err != nil {
* return err
* }
* exampleAccount, err := storage.NewAccount(ctx, "example", &storage.AccountArgs{
* Name: pulumi.String("examstorage"),
* ResourceGroupName: example.Name,
* Location: example.Location,
* AccountTier: pulumi.String("Standard"),
* AccountReplicationType: pulumi.String("LRS"),
* })
* if err != nil {
* return err
* }
* exampleContainer, err := storage.NewContainer(ctx, "example", &storage.ContainerArgs{
* Name: pulumi.String("examplecontainer"),
* StorageAccountName: exampleAccount.Name,
* ContainerAccessType: pulumi.String("private"),
* })
* if err != nil {
* return err
* }
* exampleDataCollectionEndpoint, err := monitoring.NewDataCollectionEndpoint(ctx, "example", &monitoring.DataCollectionEndpointArgs{
* Name: pulumi.String("example-dcre"),
* ResourceGroupName: example.Name,
* Location: example.Location,
* })
* if err != nil {
* return err
* }
* tmpJSON0, err := json.Marshal(map[string]interface{}{
* "a": 1,
* "b": "hello",
* })
* if err != nil {
* return err
* }
* json0 := string(tmpJSON0)
* _, err = monitoring.NewDataCollectionRule(ctx, "example", &monitoring.DataCollectionRuleArgs{
* Name: pulumi.String("example-rule"),
* ResourceGroupName: example.Name,
* Location: example.Location,
* DataCollectionEndpointId: exampleDataCollectionEndpoint.ID(),
* Destinations: &monitoring.DataCollectionRuleDestinationsArgs{
* LogAnalytics: monitoring.DataCollectionRuleDestinationsLogAnalyticArray{
* &monitoring.DataCollectionRuleDestinationsLogAnalyticArgs{
* WorkspaceResourceId: exampleAnalyticsWorkspace.ID(),
* Name: pulumi.String("example-destination-log"),
* },
* },
* EventHub: &monitoring.DataCollectionRuleDestinationsEventHubArgs{
* EventHubId: exampleEventHub.ID(),
* Name: pulumi.String("example-destination-eventhub"),
* },
* StorageBlobs: monitoring.DataCollectionRuleDestinationsStorageBlobArray{
* &monitoring.DataCollectionRuleDestinationsStorageBlobArgs{
* StorageAccountId: exampleAccount.ID(),
* ContainerName: exampleContainer.Name,
* Name: pulumi.String("example-destination-storage"),
* },
* },
* AzureMonitorMetrics: &monitoring.DataCollectionRuleDestinationsAzureMonitorMetricsArgs{
* Name: pulumi.String("example-destination-metrics"),
* },
* },
* DataFlows: monitoring.DataCollectionRuleDataFlowArray{
* &monitoring.DataCollectionRuleDataFlowArgs{
* Streams: pulumi.StringArray{
* pulumi.String("Microsoft-InsightsMetrics"),
* },
* Destinations: pulumi.StringArray{
* pulumi.String("example-destination-metrics"),
* },
* },
* &monitoring.DataCollectionRuleDataFlowArgs{
* Streams: pulumi.StringArray{
* pulumi.String("Microsoft-InsightsMetrics"),
* pulumi.String("Microsoft-Syslog"),
* pulumi.String("Microsoft-Perf"),
* },
* Destinations: pulumi.StringArray{
* pulumi.String("example-destination-log"),
* },
* },
* &monitoring.DataCollectionRuleDataFlowArgs{
* Streams: pulumi.StringArray{
* pulumi.String("Custom-MyTableRawData"),
* },
* Destinations: pulumi.StringArray{
* pulumi.String("example-destination-log"),
* },
* OutputStream: pulumi.String("Microsoft-Syslog"),
* TransformKql: pulumi.String("source | project TimeGenerated = Time, Computer, Message = AdditionalContext"),
* },
* },
* DataSources: &monitoring.DataCollectionRuleDataSourcesArgs{
* Syslogs: monitoring.DataCollectionRuleDataSourcesSyslogArray{
* &monitoring.DataCollectionRuleDataSourcesSyslogArgs{
* FacilityNames: pulumi.StringArray{
* pulumi.String("*"),
* },
* LogLevels: pulumi.StringArray{
* pulumi.String("*"),
* },
* Name: pulumi.String("example-datasource-syslog"),
* Streams: pulumi.StringArray{
* pulumi.String("Microsoft-Syslog"),
* },
* },
* },
* IisLogs: monitoring.DataCollectionRuleDataSourcesIisLogArray{
* &monitoring.DataCollectionRuleDataSourcesIisLogArgs{
* Streams: pulumi.StringArray{
* pulumi.String("Microsoft-W3CIISLog"),
* },
* Name: pulumi.String("example-datasource-iis"),
* LogDirectories: pulumi.StringArray{
* pulumi.String("C:\\Logs\\W3SVC1"),
* },
* },
* },
* LogFiles: monitoring.DataCollectionRuleDataSourcesLogFileArray{
* &monitoring.DataCollectionRuleDataSourcesLogFileArgs{
* Name: pulumi.String("example-datasource-logfile"),
* Format: pulumi.String("text"),
* Streams: pulumi.StringArray{
* pulumi.String("Custom-MyTableRawData"),
* },
* FilePatterns: pulumi.StringArray{
* pulumi.String("C:\\JavaLogs\\*.log"),
* },
* Settings: &monitoring.DataCollectionRuleDataSourcesLogFileSettingsArgs{
* Text: &monitoring.DataCollectionRuleDataSourcesLogFileSettingsTextArgs{
* RecordStartTimestampFormat: pulumi.String("ISO 8601"),
* },
* },
* },
* },
* PerformanceCounters: monitoring.DataCollectionRuleDataSourcesPerformanceCounterArray{
* &monitoring.DataCollectionRuleDataSourcesPerformanceCounterArgs{
* Streams: pulumi.StringArray{
* pulumi.String("Microsoft-Perf"),
* pulumi.String("Microsoft-InsightsMetrics"),
* },
* SamplingFrequencyInSeconds: pulumi.Int(60),
* CounterSpecifiers: pulumi.StringArray{
* pulumi.String("Processor(*)\\% Processor Time"),
* },
* Name: pulumi.String("example-datasource-perfcounter"),
* },
* },
* WindowsEventLogs: monitoring.DataCollectionRuleDataSourcesWindowsEventLogArray{
* &monitoring.DataCollectionRuleDataSourcesWindowsEventLogArgs{
* Streams: pulumi.StringArray{
* pulumi.String("Microsoft-WindowsEvent"),
* },
* XPathQueries: pulumi.StringArray{
* pulumi.String("*![System/Level=1]"),
* },
* Name: pulumi.String("example-datasource-wineventlog"),
* },
* },
* Extensions: monitoring.DataCollectionRuleDataSourcesExtensionArray{
* &monitoring.DataCollectionRuleDataSourcesExtensionArgs{
* Streams: pulumi.StringArray{
* pulumi.String("Microsoft-WindowsEvent"),
* },
* InputDataSources: pulumi.StringArray{
* pulumi.String("example-datasource-wineventlog"),
* },
* ExtensionName: pulumi.String("example-extension-name"),
* ExtensionJson: pulumi.String(json0),
* Name: pulumi.String("example-datasource-extension"),
* },
* },
* },
* StreamDeclarations: monitoring.DataCollectionRuleStreamDeclarationArray{
* &monitoring.DataCollectionRuleStreamDeclarationArgs{
* StreamName: pulumi.String("Custom-MyTableRawData"),
* Columns: monitoring.DataCollectionRuleStreamDeclarationColumnArray{
* &monitoring.DataCollectionRuleStreamDeclarationColumnArgs{
* Name: pulumi.String("Time"),
* Type: pulumi.String("datetime"),
* },
* &monitoring.DataCollectionRuleStreamDeclarationColumnArgs{
* Name: pulumi.String("Computer"),
* Type: pulumi.String("string"),
* },
* &monitoring.DataCollectionRuleStreamDeclarationColumnArgs{
* Name: pulumi.String("AdditionalContext"),
* Type: pulumi.String("string"),
* },
* },
* },
* },
* Identity: &monitoring.DataCollectionRuleIdentityArgs{
* Type: pulumi.String("UserAssigned"),
* IdentityIds: pulumi.StringArray{
* exampleUserAssignedIdentity.ID(),
* },
* },
* Description: pulumi.String("data collection rule example"),
* Tags: pulumi.StringMap{
* "foo": pulumi.String("bar"),
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azure.core.ResourceGroup;
* import com.pulumi.azure.core.ResourceGroupArgs;
* import com.pulumi.azure.authorization.UserAssignedIdentity;
* import com.pulumi.azure.authorization.UserAssignedIdentityArgs;
* import com.pulumi.azure.operationalinsights.AnalyticsWorkspace;
* import com.pulumi.azure.operationalinsights.AnalyticsWorkspaceArgs;
* import com.pulumi.azure.operationalinsights.AnalyticsSolution;
* import com.pulumi.azure.operationalinsights.AnalyticsSolutionArgs;
* import com.pulumi.azure.operationalinsights.inputs.AnalyticsSolutionPlanArgs;
* import com.pulumi.azure.eventhub.EventHubNamespace;
* import com.pulumi.azure.eventhub.EventHubNamespaceArgs;
* import com.pulumi.azure.eventhub.EventHub;
* import com.pulumi.azure.eventhub.EventHubArgs;
* import com.pulumi.azure.storage.Account;
* import com.pulumi.azure.storage.AccountArgs;
* import com.pulumi.azure.storage.Container;
* import com.pulumi.azure.storage.ContainerArgs;
* import com.pulumi.azure.monitoring.DataCollectionEndpoint;
* import com.pulumi.azure.monitoring.DataCollectionEndpointArgs;
* import com.pulumi.azure.monitoring.DataCollectionRule;
* import com.pulumi.azure.monitoring.DataCollectionRuleArgs;
* import com.pulumi.azure.monitoring.inputs.DataCollectionRuleDestinationsArgs;
* import com.pulumi.azure.monitoring.inputs.DataCollectionRuleDestinationsEventHubArgs;
* import com.pulumi.azure.monitoring.inputs.DataCollectionRuleDestinationsAzureMonitorMetricsArgs;
* import com.pulumi.azure.monitoring.inputs.DataCollectionRuleDataFlowArgs;
* import com.pulumi.azure.monitoring.inputs.DataCollectionRuleDataSourcesArgs;
* import com.pulumi.azure.monitoring.inputs.DataCollectionRuleStreamDeclarationArgs;
* import com.pulumi.azure.monitoring.inputs.DataCollectionRuleIdentityArgs;
* import static com.pulumi.codegen.internal.Serialization.*;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var example = new ResourceGroup("example", ResourceGroupArgs.builder()
* .name("example-resources")
* .location("West Europe")
* .build());
* var exampleUserAssignedIdentity = new UserAssignedIdentity("exampleUserAssignedIdentity", UserAssignedIdentityArgs.builder()
* .name("example-uai")
* .resourceGroupName(example.name())
* .location(example.location())
* .build());
* var exampleAnalyticsWorkspace = new AnalyticsWorkspace("exampleAnalyticsWorkspace", AnalyticsWorkspaceArgs.builder()
* .name("example-workspace")
* .resourceGroupName(example.name())
* .location(example.location())
* .build());
* var exampleAnalyticsSolution = new AnalyticsSolution("exampleAnalyticsSolution", AnalyticsSolutionArgs.builder()
* .solutionName("WindowsEventForwarding")
* .location(example.location())
* .resourceGroupName(example.name())
* .workspaceResourceId(exampleAnalyticsWorkspace.id())
* .workspaceName(exampleAnalyticsWorkspace.name())
* .plan(AnalyticsSolutionPlanArgs.builder()
* .publisher("Microsoft")
* .product("OMSGallery/WindowsEventForwarding")
* .build())
* .build());
* var exampleEventHubNamespace = new EventHubNamespace("exampleEventHubNamespace", EventHubNamespaceArgs.builder()
* .name("exeventns")
* .location(example.location())
* .resourceGroupName(example.name())
* .sku("Standard")
* .capacity(1)
* .build());
* var exampleEventHub = new EventHub("exampleEventHub", EventHubArgs.builder()
* .name("exevent2")
* .namespaceName(exampleEventHubNamespace.name())
* .resourceGroupName(example.name())
* .partitionCount(2)
* .messageRetention(1)
* .build());
* var exampleAccount = new Account("exampleAccount", AccountArgs.builder()
* .name("examstorage")
* .resourceGroupName(example.name())
* .location(example.location())
* .accountTier("Standard")
* .accountReplicationType("LRS")
* .build());
* var exampleContainer = new Container("exampleContainer", ContainerArgs.builder()
* .name("examplecontainer")
* .storageAccountName(exampleAccount.name())
* .containerAccessType("private")
* .build());
* var exampleDataCollectionEndpoint = new DataCollectionEndpoint("exampleDataCollectionEndpoint", DataCollectionEndpointArgs.builder()
* .name("example-dcre")
* .resourceGroupName(example.name())
* .location(example.location())
* .build());
* var exampleDataCollectionRule = new DataCollectionRule("exampleDataCollectionRule", DataCollectionRuleArgs.builder()
* .name("example-rule")
* .resourceGroupName(example.name())
* .location(example.location())
* .dataCollectionEndpointId(exampleDataCollectionEndpoint.id())
* .destinations(DataCollectionRuleDestinationsArgs.builder()
* .logAnalytics(DataCollectionRuleDestinationsLogAnalyticArgs.builder()
* .workspaceResourceId(exampleAnalyticsWorkspace.id())
* .name("example-destination-log")
* .build())
* .eventHub(DataCollectionRuleDestinationsEventHubArgs.builder()
* .eventHubId(exampleEventHub.id())
* .name("example-destination-eventhub")
* .build())
* .storageBlobs(DataCollectionRuleDestinationsStorageBlobArgs.builder()
* .storageAccountId(exampleAccount.id())
* .containerName(exampleContainer.name())
* .name("example-destination-storage")
* .build())
* .azureMonitorMetrics(DataCollectionRuleDestinationsAzureMonitorMetricsArgs.builder()
* .name("example-destination-metrics")
* .build())
* .build())
* .dataFlows(
* DataCollectionRuleDataFlowArgs.builder()
* .streams("Microsoft-InsightsMetrics")
* .destinations("example-destination-metrics")
* .build(),
* DataCollectionRuleDataFlowArgs.builder()
* .streams(
* "Microsoft-InsightsMetrics",
* "Microsoft-Syslog",
* "Microsoft-Perf")
* .destinations("example-destination-log")
* .build(),
* DataCollectionRuleDataFlowArgs.builder()
* .streams("Custom-MyTableRawData")
* .destinations("example-destination-log")
* .outputStream("Microsoft-Syslog")
* .transformKql("source | project TimeGenerated = Time, Computer, Message = AdditionalContext")
* .build())
* .dataSources(DataCollectionRuleDataSourcesArgs.builder()
* .syslogs(DataCollectionRuleDataSourcesSyslogArgs.builder()
* .facilityNames("*")
* .logLevels("*")
* .name("example-datasource-syslog")
* .streams("Microsoft-Syslog")
* .build())
* .iisLogs(DataCollectionRuleDataSourcesIisLogArgs.builder()
* .streams("Microsoft-W3CIISLog")
* .name("example-datasource-iis")
* .logDirectories("C:\\Logs\\W3SVC1")
* .build())
* .logFiles(DataCollectionRuleDataSourcesLogFileArgs.builder()
* .name("example-datasource-logfile")
* .format("text")
* .streams("Custom-MyTableRawData")
* .filePatterns("C:\\JavaLogs\\*.log")
* .settings(DataCollectionRuleDataSourcesLogFileSettingsArgs.builder()
* .text(DataCollectionRuleDataSourcesLogFileSettingsTextArgs.builder()
* .recordStartTimestampFormat("ISO 8601")
* .build())
* .build())
* .build())
* .performanceCounters(DataCollectionRuleDataSourcesPerformanceCounterArgs.builder()
* .streams(
* "Microsoft-Perf",
* "Microsoft-InsightsMetrics")
* .samplingFrequencyInSeconds(60)
* .counterSpecifiers("Processor(*)\\% Processor Time")
* .name("example-datasource-perfcounter")
* .build())
* .windowsEventLogs(DataCollectionRuleDataSourcesWindowsEventLogArgs.builder()
* .streams("Microsoft-WindowsEvent")
* .xPathQueries("*![System/Level=1]")
* .name("example-datasource-wineventlog")
* .build())
* .extensions(DataCollectionRuleDataSourcesExtensionArgs.builder()
* .streams("Microsoft-WindowsEvent")
* .inputDataSources("example-datasource-wineventlog")
* .extensionName("example-extension-name")
* .extensionJson(serializeJson(
* jsonObject(
* jsonProperty("a", 1),
* jsonProperty("b", "hello")
* )))
* .name("example-datasource-extension")
* .build())
* .build())
* .streamDeclarations(DataCollectionRuleStreamDeclarationArgs.builder()
* .streamName("Custom-MyTableRawData")
* .columns(
* DataCollectionRuleStreamDeclarationColumnArgs.builder()
* .name("Time")
* .type("datetime")
* .build(),
* DataCollectionRuleStreamDeclarationColumnArgs.builder()
* .name("Computer")
* .type("string")
* .build(),
* DataCollectionRuleStreamDeclarationColumnArgs.builder()
* .name("AdditionalContext")
* .type("string")
* .build())
* .build())
* .identity(DataCollectionRuleIdentityArgs.builder()
* .type("UserAssigned")
* .identityIds(exampleUserAssignedIdentity.id())
* .build())
* .description("data collection rule example")
* .tags(Map.of("foo", "bar"))
* .build());
* }
* }
* ```
* ```yaml
* resources:
* example:
* type: azure:core:ResourceGroup
* properties:
* name: example-resources
* location: West Europe
* exampleUserAssignedIdentity:
* type: azure:authorization:UserAssignedIdentity
* name: example
* properties:
* name: example-uai
* resourceGroupName: ${example.name}
* location: ${example.location}
* exampleAnalyticsWorkspace:
* type: azure:operationalinsights:AnalyticsWorkspace
* name: example
* properties:
* name: example-workspace
* resourceGroupName: ${example.name}
* location: ${example.location}
* exampleAnalyticsSolution:
* type: azure:operationalinsights:AnalyticsSolution
* name: example
* properties:
* solutionName: WindowsEventForwarding
* location: ${example.location}
* resourceGroupName: ${example.name}
* workspaceResourceId: ${exampleAnalyticsWorkspace.id}
* workspaceName: ${exampleAnalyticsWorkspace.name}
* plan:
* publisher: Microsoft
* product: OMSGallery/WindowsEventForwarding
* exampleEventHubNamespace:
* type: azure:eventhub:EventHubNamespace
* name: example
* properties:
* name: exeventns
* location: ${example.location}
* resourceGroupName: ${example.name}
* sku: Standard
* capacity: 1
* exampleEventHub:
* type: azure:eventhub:EventHub
* name: example
* properties:
* name: exevent2
* namespaceName: ${exampleEventHubNamespace.name}
* resourceGroupName: ${example.name}
* partitionCount: 2
* messageRetention: 1
* exampleAccount:
* type: azure:storage:Account
* name: example
* properties:
* name: examstorage
* resourceGroupName: ${example.name}
* location: ${example.location}
* accountTier: Standard
* accountReplicationType: LRS
* exampleContainer:
* type: azure:storage:Container
* name: example
* properties:
* name: examplecontainer
* storageAccountName: ${exampleAccount.name}
* containerAccessType: private
* exampleDataCollectionEndpoint:
* type: azure:monitoring:DataCollectionEndpoint
* name: example
* properties:
* name: example-dcre
* resourceGroupName: ${example.name}
* location: ${example.location}
* exampleDataCollectionRule:
* type: azure:monitoring:DataCollectionRule
* name: example
* properties:
* name: example-rule
* resourceGroupName: ${example.name}
* location: ${example.location}
* dataCollectionEndpointId: ${exampleDataCollectionEndpoint.id}
* destinations:
* logAnalytics:
* - workspaceResourceId: ${exampleAnalyticsWorkspace.id}
* name: example-destination-log
* eventHub:
* eventHubId: ${exampleEventHub.id}
* name: example-destination-eventhub
* storageBlobs:
* - storageAccountId: ${exampleAccount.id}
* containerName: ${exampleContainer.name}
* name: example-destination-storage
* azureMonitorMetrics:
* name: example-destination-metrics
* dataFlows:
* - streams:
* - Microsoft-InsightsMetrics
* destinations:
* - example-destination-metrics
* - streams:
* - Microsoft-InsightsMetrics
* - Microsoft-Syslog
* - Microsoft-Perf
* destinations:
* - example-destination-log
* - streams:
* - Custom-MyTableRawData
* destinations:
* - example-destination-log
* outputStream: Microsoft-Syslog
* transformKql: source | project TimeGenerated = Time, Computer, Message = AdditionalContext
* dataSources:
* syslogs:
* - facilityNames:
* - '*'
* logLevels:
* - '*'
* name: example-datasource-syslog
* streams:
* - Microsoft-Syslog
* iisLogs:
* - streams:
* - Microsoft-W3CIISLog
* name: example-datasource-iis
* logDirectories:
* - C:\Logs\W3SVC1
* logFiles:
* - name: example-datasource-logfile
* format: text
* streams:
* - Custom-MyTableRawData
* filePatterns:
* - C:\JavaLogs\*.log
* settings:
* text:
* recordStartTimestampFormat: ISO 8601
* performanceCounters:
* - streams:
* - Microsoft-Perf
* - Microsoft-InsightsMetrics
* samplingFrequencyInSeconds: 60
* counterSpecifiers:
* - Processor(*)\% Processor Time
* name: example-datasource-perfcounter
* windowsEventLogs:
* - streams:
* - Microsoft-WindowsEvent
* xPathQueries:
* - '*![System/Level=1]'
* name: example-datasource-wineventlog
* extensions:
* - streams:
* - Microsoft-WindowsEvent
* inputDataSources:
* - example-datasource-wineventlog
* extensionName: example-extension-name
* extensionJson:
* fn::toJSON:
* a: 1
* b: hello
* name: example-datasource-extension
* streamDeclarations:
* - streamName: Custom-MyTableRawData
* columns:
* - name: Time
* type: datetime
* - name: Computer
* type: string
* - name: AdditionalContext
* type: string
* identity:
* type: UserAssigned
* identityIds:
* - ${exampleUserAssignedIdentity.id}
* description: data collection rule example
* tags:
* foo: bar
* ```
*
* ## Import
* Data Collection Rules can be imported using the `resource id`, e.g.
* ```sh
* $ pulumi import azure:monitoring/dataCollectionRule:DataCollectionRule example /subscriptions/12345678-1234-9876-4563-123456789012/resourceGroups/group1/providers/Microsoft.Insights/dataCollectionRules/rule1
* ```
* @property dataCollectionEndpointId The resource ID of the Data Collection Endpoint that this rule can be used with.
* @property dataFlows One or more `data_flow` blocks as defined below.
* @property dataSources A `data_sources` block as defined below. This property is optional and can be omitted if the rule is meant to be used via direct calls to the provisioned endpoint.
* @property description The description of the Data Collection Rule.
* @property destinations A `destinations` block as defined below.
* @property identity An `identity` block as defined below.
* @property kind The kind of the Data Collection Rule. Possible values are `Linux`, `Windows`, `AgentDirectToStore` and `WorkspaceTransforms`. A rule of kind `Linux` does not allow for `windows_event_log` data sources. And a rule of kind `Windows` does not allow for `syslog` data sources. If kind is not specified, all kinds of data sources are allowed.
* > **NOTE** Once `kind` has been set, changing it forces a new Data Collection Rule to be created.
* @property location The Azure Region where the Data Collection Rule should exist. Changing this forces a new Data Collection Rule to be created.
* @property name The name which should be used for this Data Collection Rule. Changing this forces a new Data Collection Rule to be created.
* @property resourceGroupName The name of the Resource Group where the Data Collection Rule should exist. Changing this forces a new Data Collection Rule to be created.
* @property streamDeclarations A `stream_declaration` block as defined below.
* @property tags A mapping of tags which should be assigned to the Data Collection Rule.
*/
public data class DataCollectionRuleArgs(
public val dataCollectionEndpointId: Output? = null,
public val dataFlows: Output>? = null,
public val dataSources: Output? = null,
public val description: Output? = null,
public val destinations: Output? = null,
public val identity: Output? = null,
public val kind: Output? = null,
public val location: Output? = null,
public val name: Output? = null,
public val resourceGroupName: Output? = null,
public val streamDeclarations: Output>? = null,
public val tags: Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy