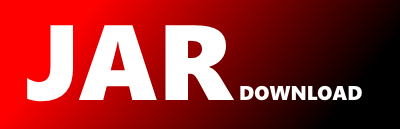
com.pulumi.azure.monitoring.kotlin.inputs.ActionGroupItsmReceiverArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-kotlin Show documentation
Show all versions of pulumi-azure-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azure.monitoring.kotlin.inputs
import com.pulumi.azure.monitoring.inputs.ActionGroupItsmReceiverArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
*
* @property connectionId The unique connection identifier of the ITSM connection.
* @property name The name of the ITSM receiver.
* @property region The region of the workspace.
* > **NOTE** `ticket_configuration` should be JSON blob with `PayloadRevision` and `WorkItemType` keys (e.g., `ticket_configuration="{\"PayloadRevision\":0,\"WorkItemType\":\"Incident\"}"`), and `ticket_configuration="{}"` will return an error, see more at this [REST API issue](https://github.com/Azure/azure-rest-api-specs/issues/20488)
* @property ticketConfiguration A JSON blob for the configurations of the ITSM action. CreateMultipleWorkItems option will be part of this blob as well.
* @property workspaceId The Azure Log Analytics workspace ID where this connection is defined. Format is `|`, for example `00000000-0000-0000-0000-000000000000|00000000-0000-0000-0000-000000000000`.
*/
public data class ActionGroupItsmReceiverArgs(
public val connectionId: Output,
public val name: Output,
public val region: Output,
public val ticketConfiguration: Output,
public val workspaceId: Output,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azure.monitoring.inputs.ActionGroupItsmReceiverArgs =
com.pulumi.azure.monitoring.inputs.ActionGroupItsmReceiverArgs.builder()
.connectionId(connectionId.applyValue({ args0 -> args0 }))
.name(name.applyValue({ args0 -> args0 }))
.region(region.applyValue({ args0 -> args0 }))
.ticketConfiguration(ticketConfiguration.applyValue({ args0 -> args0 }))
.workspaceId(workspaceId.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [ActionGroupItsmReceiverArgs].
*/
@PulumiTagMarker
public class ActionGroupItsmReceiverArgsBuilder internal constructor() {
private var connectionId: Output? = null
private var name: Output? = null
private var region: Output? = null
private var ticketConfiguration: Output? = null
private var workspaceId: Output? = null
/**
* @param value The unique connection identifier of the ITSM connection.
*/
@JvmName("dbclgxrlcvoogvrs")
public suspend fun connectionId(`value`: Output) {
this.connectionId = value
}
/**
* @param value The name of the ITSM receiver.
*/
@JvmName("yhurpwnuqvobrefv")
public suspend fun name(`value`: Output) {
this.name = value
}
/**
* @param value The region of the workspace.
* > **NOTE** `ticket_configuration` should be JSON blob with `PayloadRevision` and `WorkItemType` keys (e.g., `ticket_configuration="{\"PayloadRevision\":0,\"WorkItemType\":\"Incident\"}"`), and `ticket_configuration="{}"` will return an error, see more at this [REST API issue](https://github.com/Azure/azure-rest-api-specs/issues/20488)
*/
@JvmName("scqeccqhvtnacgcu")
public suspend fun region(`value`: Output) {
this.region = value
}
/**
* @param value A JSON blob for the configurations of the ITSM action. CreateMultipleWorkItems option will be part of this blob as well.
*/
@JvmName("lwweehxttijojfff")
public suspend fun ticketConfiguration(`value`: Output) {
this.ticketConfiguration = value
}
/**
* @param value The Azure Log Analytics workspace ID where this connection is defined. Format is `|`, for example `00000000-0000-0000-0000-000000000000|00000000-0000-0000-0000-000000000000`.
*/
@JvmName("qisnvkphdklwrbcp")
public suspend fun workspaceId(`value`: Output) {
this.workspaceId = value
}
/**
* @param value The unique connection identifier of the ITSM connection.
*/
@JvmName("rbluiikuwheknmcm")
public suspend fun connectionId(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.connectionId = mapped
}
/**
* @param value The name of the ITSM receiver.
*/
@JvmName("npehdetpkdnqjxkv")
public suspend fun name(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.name = mapped
}
/**
* @param value The region of the workspace.
* > **NOTE** `ticket_configuration` should be JSON blob with `PayloadRevision` and `WorkItemType` keys (e.g., `ticket_configuration="{\"PayloadRevision\":0,\"WorkItemType\":\"Incident\"}"`), and `ticket_configuration="{}"` will return an error, see more at this [REST API issue](https://github.com/Azure/azure-rest-api-specs/issues/20488)
*/
@JvmName("psjvmimxyonkyffu")
public suspend fun region(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.region = mapped
}
/**
* @param value A JSON blob for the configurations of the ITSM action. CreateMultipleWorkItems option will be part of this blob as well.
*/
@JvmName("poxhrkeevrcfsofu")
public suspend fun ticketConfiguration(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.ticketConfiguration = mapped
}
/**
* @param value The Azure Log Analytics workspace ID where this connection is defined. Format is `|`, for example `00000000-0000-0000-0000-000000000000|00000000-0000-0000-0000-000000000000`.
*/
@JvmName("lctojijmjnofqrlw")
public suspend fun workspaceId(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.workspaceId = mapped
}
internal fun build(): ActionGroupItsmReceiverArgs = ActionGroupItsmReceiverArgs(
connectionId = connectionId ?: throw PulumiNullFieldException("connectionId"),
name = name ?: throw PulumiNullFieldException("name"),
region = region ?: throw PulumiNullFieldException("region"),
ticketConfiguration = ticketConfiguration ?: throw PulumiNullFieldException("ticketConfiguration"),
workspaceId = workspaceId ?: throw PulumiNullFieldException("workspaceId"),
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy