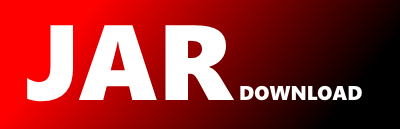
com.pulumi.azure.monitoring.kotlin.inputs.ActionRuleSuppressionConditionArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-kotlin Show documentation
Show all versions of pulumi-azure-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azure.monitoring.kotlin.inputs
import com.pulumi.azure.monitoring.inputs.ActionRuleSuppressionConditionArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
*
* @property alertContext A `alert_context` block as defined below.
* @property alertRuleId A `alert_rule_id` block as defined below.
* @property description A `description` block as defined below.
* @property monitor A `monitor` block as defined below.
* @property monitorService A `monitor_service` block as defined below.
* @property severity A `severity` block as defined below.
* @property targetResourceType A `target_resource_type` block as defined below.
*/
public data class ActionRuleSuppressionConditionArgs(
public val alertContext: Output? = null,
public val alertRuleId: Output? = null,
public val description: Output? = null,
public val monitor: Output? = null,
public val monitorService: Output? = null,
public val severity: Output? = null,
public val targetResourceType: Output? =
null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azure.monitoring.inputs.ActionRuleSuppressionConditionArgs =
com.pulumi.azure.monitoring.inputs.ActionRuleSuppressionConditionArgs.builder()
.alertContext(alertContext?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.alertRuleId(alertRuleId?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.description(description?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.monitor(monitor?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.monitorService(monitorService?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.severity(severity?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.targetResourceType(
targetResourceType?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
).build()
}
/**
* Builder for [ActionRuleSuppressionConditionArgs].
*/
@PulumiTagMarker
public class ActionRuleSuppressionConditionArgsBuilder internal constructor() {
private var alertContext: Output? = null
private var alertRuleId: Output? = null
private var description: Output? = null
private var monitor: Output? = null
private var monitorService: Output? = null
private var severity: Output? = null
private var targetResourceType: Output? =
null
/**
* @param value A `alert_context` block as defined below.
*/
@JvmName("buovqxmmtbdktghg")
public suspend fun alertContext(`value`: Output) {
this.alertContext = value
}
/**
* @param value A `alert_rule_id` block as defined below.
*/
@JvmName("ruhttbhyifsebjsp")
public suspend fun alertRuleId(`value`: Output) {
this.alertRuleId = value
}
/**
* @param value A `description` block as defined below.
*/
@JvmName("vpimvalchoetamqh")
public suspend fun description(`value`: Output) {
this.description = value
}
/**
* @param value A `monitor` block as defined below.
*/
@JvmName("daubicmegjjhalgu")
public suspend fun monitor(`value`: Output) {
this.monitor = value
}
/**
* @param value A `monitor_service` block as defined below.
*/
@JvmName("mrlkjmxpikovhmcv")
public suspend
fun monitorService(`value`: Output) {
this.monitorService = value
}
/**
* @param value A `severity` block as defined below.
*/
@JvmName("hhdqitdngruoihbr")
public suspend fun severity(`value`: Output) {
this.severity = value
}
/**
* @param value A `target_resource_type` block as defined below.
*/
@JvmName("joartbjpwiqeuoyh")
public suspend
fun targetResourceType(`value`: Output) {
this.targetResourceType = value
}
/**
* @param value A `alert_context` block as defined below.
*/
@JvmName("nnuuogdkcjfpqyqq")
public suspend fun alertContext(`value`: ActionRuleSuppressionConditionAlertContextArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.alertContext = mapped
}
/**
* @param argument A `alert_context` block as defined below.
*/
@JvmName("ifobdnawhovbfyjh")
public suspend
fun alertContext(argument: suspend ActionRuleSuppressionConditionAlertContextArgsBuilder.() -> Unit) {
val toBeMapped = ActionRuleSuppressionConditionAlertContextArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.alertContext = mapped
}
/**
* @param value A `alert_rule_id` block as defined below.
*/
@JvmName("brwfyrnwyybmvpoq")
public suspend fun alertRuleId(`value`: ActionRuleSuppressionConditionAlertRuleIdArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.alertRuleId = mapped
}
/**
* @param argument A `alert_rule_id` block as defined below.
*/
@JvmName("fsgpyuauxckjakyq")
public suspend
fun alertRuleId(argument: suspend ActionRuleSuppressionConditionAlertRuleIdArgsBuilder.() -> Unit) {
val toBeMapped = ActionRuleSuppressionConditionAlertRuleIdArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.alertRuleId = mapped
}
/**
* @param value A `description` block as defined below.
*/
@JvmName("asqaxjadciidjksa")
public suspend fun description(`value`: ActionRuleSuppressionConditionDescriptionArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.description = mapped
}
/**
* @param argument A `description` block as defined below.
*/
@JvmName("lstgnhbrcidnmjtl")
public suspend
fun description(argument: suspend ActionRuleSuppressionConditionDescriptionArgsBuilder.() -> Unit) {
val toBeMapped = ActionRuleSuppressionConditionDescriptionArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.description = mapped
}
/**
* @param value A `monitor` block as defined below.
*/
@JvmName("ucydpjfmnlqnffqf")
public suspend fun monitor(`value`: ActionRuleSuppressionConditionMonitorArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.monitor = mapped
}
/**
* @param argument A `monitor` block as defined below.
*/
@JvmName("vdywbcvvgjhydgyq")
public suspend
fun monitor(argument: suspend ActionRuleSuppressionConditionMonitorArgsBuilder.() -> Unit) {
val toBeMapped = ActionRuleSuppressionConditionMonitorArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.monitor = mapped
}
/**
* @param value A `monitor_service` block as defined below.
*/
@JvmName("obtwtmjwqtlhlyip")
public suspend fun monitorService(`value`: ActionRuleSuppressionConditionMonitorServiceArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.monitorService = mapped
}
/**
* @param argument A `monitor_service` block as defined below.
*/
@JvmName("ujfyqekwoqdfmpyi")
public suspend
fun monitorService(argument: suspend ActionRuleSuppressionConditionMonitorServiceArgsBuilder.() -> Unit) {
val toBeMapped = ActionRuleSuppressionConditionMonitorServiceArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.monitorService = mapped
}
/**
* @param value A `severity` block as defined below.
*/
@JvmName("dqgdvgwygnfpqowa")
public suspend fun severity(`value`: ActionRuleSuppressionConditionSeverityArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.severity = mapped
}
/**
* @param argument A `severity` block as defined below.
*/
@JvmName("nosrtihjhhgwbnrl")
public suspend
fun severity(argument: suspend ActionRuleSuppressionConditionSeverityArgsBuilder.() -> Unit) {
val toBeMapped = ActionRuleSuppressionConditionSeverityArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.severity = mapped
}
/**
* @param value A `target_resource_type` block as defined below.
*/
@JvmName("fuenclhpwlswtmhi")
public suspend
fun targetResourceType(`value`: ActionRuleSuppressionConditionTargetResourceTypeArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.targetResourceType = mapped
}
/**
* @param argument A `target_resource_type` block as defined below.
*/
@JvmName("yorndbeuiqrvvpbb")
public suspend
fun targetResourceType(argument: suspend ActionRuleSuppressionConditionTargetResourceTypeArgsBuilder.() -> Unit) {
val toBeMapped = ActionRuleSuppressionConditionTargetResourceTypeArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.targetResourceType = mapped
}
internal fun build(): ActionRuleSuppressionConditionArgs = ActionRuleSuppressionConditionArgs(
alertContext = alertContext,
alertRuleId = alertRuleId,
description = description,
monitor = monitor,
monitorService = monitorService,
severity = severity,
targetResourceType = targetResourceType,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy