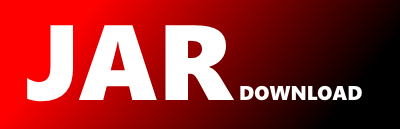
com.pulumi.azure.monitoring.kotlin.inputs.AlertProcessingRuleSuppressionConditionArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-kotlin Show documentation
Show all versions of pulumi-azure-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azure.monitoring.kotlin.inputs
import com.pulumi.azure.monitoring.inputs.AlertProcessingRuleSuppressionConditionArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
*
* @property alertContext A `alert_context` block as defined above.
* @property alertRuleId A `alert_rule_id` block as defined above.
* @property alertRuleName A `alert_rule_name` block as defined above.
* @property description A `description` block as defined below.
* @property monitorCondition A `monitor_condition` block as defined below.
* @property monitorService A `monitor_service` block as defined below.
* @property severity A `severity` block as defined below.
* @property signalType A `signal_type` block as defined below.
* @property targetResource A `target_resource` block as defined below.
* @property targetResourceGroup A `target_resource_group` block as defined below.
* @property targetResourceType A `target_resource_type` block as defined below.
*/
public data class AlertProcessingRuleSuppressionConditionArgs(
public val alertContext: Output? = null,
public val alertRuleId: Output? = null,
public val alertRuleName: Output? =
null,
public val description: Output? = null,
public val monitorCondition: Output? =
null,
public val monitorService: Output? =
null,
public val severity: Output? = null,
public val signalType: Output? = null,
public val targetResource: Output? =
null,
public val targetResourceGroup:
Output? = null,
public val targetResourceType:
Output? = null,
) :
ConvertibleToJava {
override fun toJava():
com.pulumi.azure.monitoring.inputs.AlertProcessingRuleSuppressionConditionArgs =
com.pulumi.azure.monitoring.inputs.AlertProcessingRuleSuppressionConditionArgs.builder()
.alertContext(alertContext?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.alertRuleId(alertRuleId?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.alertRuleName(alertRuleName?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.description(description?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.monitorCondition(monitorCondition?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.monitorService(monitorService?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.severity(severity?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.signalType(signalType?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.targetResource(targetResource?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.targetResourceGroup(
targetResourceGroup?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.targetResourceType(
targetResourceType?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
).build()
}
/**
* Builder for [AlertProcessingRuleSuppressionConditionArgs].
*/
@PulumiTagMarker
public class AlertProcessingRuleSuppressionConditionArgsBuilder internal constructor() {
private var alertContext: Output? = null
private var alertRuleId: Output? = null
private var alertRuleName: Output? =
null
private var description: Output? = null
private var monitorCondition: Output? =
null
private var monitorService: Output? =
null
private var severity: Output? = null
private var signalType: Output? = null
private var targetResource: Output? =
null
private var targetResourceGroup:
Output? = null
private var targetResourceType:
Output? = null
/**
* @param value A `alert_context` block as defined above.
*/
@JvmName("axxkeullxycbbyvx")
public suspend
fun alertContext(`value`: Output) {
this.alertContext = value
}
/**
* @param value A `alert_rule_id` block as defined above.
*/
@JvmName("ngoxcmwpnfvvumvt")
public suspend
fun alertRuleId(`value`: Output) {
this.alertRuleId = value
}
/**
* @param value A `alert_rule_name` block as defined above.
*/
@JvmName("rlyhufgednbnmtcp")
public suspend
fun alertRuleName(`value`: Output) {
this.alertRuleName = value
}
/**
* @param value A `description` block as defined below.
*/
@JvmName("cedrffpbkrbhvdwc")
public suspend
fun description(`value`: Output) {
this.description = value
}
/**
* @param value A `monitor_condition` block as defined below.
*/
@JvmName("pqerpvmunfwlango")
public suspend
fun monitorCondition(`value`: Output) {
this.monitorCondition = value
}
/**
* @param value A `monitor_service` block as defined below.
*/
@JvmName("rugqaelrqoctisqj")
public suspend
fun monitorService(`value`: Output) {
this.monitorService = value
}
/**
* @param value A `severity` block as defined below.
*/
@JvmName("vshjukoexojnqoib")
public suspend
fun severity(`value`: Output) {
this.severity = value
}
/**
* @param value A `signal_type` block as defined below.
*/
@JvmName("frhqtkjfntwaxeft")
public suspend
fun signalType(`value`: Output) {
this.signalType = value
}
/**
* @param value A `target_resource` block as defined below.
*/
@JvmName("bwyvfssrkbmbglxi")
public suspend
fun targetResource(`value`: Output) {
this.targetResource = value
}
/**
* @param value A `target_resource_group` block as defined below.
*/
@JvmName("fuepmfeniryvdbfv")
public suspend
fun targetResourceGroup(`value`: Output) {
this.targetResourceGroup = value
}
/**
* @param value A `target_resource_type` block as defined below.
*/
@JvmName("soecejqusuwxeidp")
public suspend
fun targetResourceType(`value`: Output) {
this.targetResourceType = value
}
/**
* @param value A `alert_context` block as defined above.
*/
@JvmName("wpbkiqtrmusjbili")
public suspend
fun alertContext(`value`: AlertProcessingRuleSuppressionConditionAlertContextArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.alertContext = mapped
}
/**
* @param argument A `alert_context` block as defined above.
*/
@JvmName("sakfefbjciwqjdvw")
public suspend
fun alertContext(argument: suspend AlertProcessingRuleSuppressionConditionAlertContextArgsBuilder.() -> Unit) {
val toBeMapped = AlertProcessingRuleSuppressionConditionAlertContextArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.alertContext = mapped
}
/**
* @param value A `alert_rule_id` block as defined above.
*/
@JvmName("yopcdpprclvwpwgl")
public suspend fun alertRuleId(`value`: AlertProcessingRuleSuppressionConditionAlertRuleIdArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.alertRuleId = mapped
}
/**
* @param argument A `alert_rule_id` block as defined above.
*/
@JvmName("tegsqwtlxjlcuofa")
public suspend
fun alertRuleId(argument: suspend AlertProcessingRuleSuppressionConditionAlertRuleIdArgsBuilder.() -> Unit) {
val toBeMapped = AlertProcessingRuleSuppressionConditionAlertRuleIdArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.alertRuleId = mapped
}
/**
* @param value A `alert_rule_name` block as defined above.
*/
@JvmName("wingcvwkrnnaccch")
public suspend
fun alertRuleName(`value`: AlertProcessingRuleSuppressionConditionAlertRuleNameArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.alertRuleName = mapped
}
/**
* @param argument A `alert_rule_name` block as defined above.
*/
@JvmName("ruwnrkqqklkmbwvu")
public suspend
fun alertRuleName(argument: suspend AlertProcessingRuleSuppressionConditionAlertRuleNameArgsBuilder.() -> Unit) {
val toBeMapped = AlertProcessingRuleSuppressionConditionAlertRuleNameArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.alertRuleName = mapped
}
/**
* @param value A `description` block as defined below.
*/
@JvmName("ijvbrcqydbiaqyio")
public suspend fun description(`value`: AlertProcessingRuleSuppressionConditionDescriptionArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.description = mapped
}
/**
* @param argument A `description` block as defined below.
*/
@JvmName("irtxjbxkytybgawj")
public suspend
fun description(argument: suspend AlertProcessingRuleSuppressionConditionDescriptionArgsBuilder.() -> Unit) {
val toBeMapped = AlertProcessingRuleSuppressionConditionDescriptionArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.description = mapped
}
/**
* @param value A `monitor_condition` block as defined below.
*/
@JvmName("xiejesysmufkplcn")
public suspend
fun monitorCondition(`value`: AlertProcessingRuleSuppressionConditionMonitorConditionArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.monitorCondition = mapped
}
/**
* @param argument A `monitor_condition` block as defined below.
*/
@JvmName("uyjinjvvqpulahff")
public suspend
fun monitorCondition(argument: suspend AlertProcessingRuleSuppressionConditionMonitorConditionArgsBuilder.() -> Unit) {
val toBeMapped =
AlertProcessingRuleSuppressionConditionMonitorConditionArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.monitorCondition = mapped
}
/**
* @param value A `monitor_service` block as defined below.
*/
@JvmName("arcbtkyouiixoofw")
public suspend
fun monitorService(`value`: AlertProcessingRuleSuppressionConditionMonitorServiceArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.monitorService = mapped
}
/**
* @param argument A `monitor_service` block as defined below.
*/
@JvmName("wngxobywmjkaoehj")
public suspend
fun monitorService(argument: suspend AlertProcessingRuleSuppressionConditionMonitorServiceArgsBuilder.() -> Unit) {
val toBeMapped =
AlertProcessingRuleSuppressionConditionMonitorServiceArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.monitorService = mapped
}
/**
* @param value A `severity` block as defined below.
*/
@JvmName("ssbkdeihrnysojgh")
public suspend fun severity(`value`: AlertProcessingRuleSuppressionConditionSeverityArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.severity = mapped
}
/**
* @param argument A `severity` block as defined below.
*/
@JvmName("xyrooiemuskrnoos")
public suspend
fun severity(argument: suspend AlertProcessingRuleSuppressionConditionSeverityArgsBuilder.() -> Unit) {
val toBeMapped = AlertProcessingRuleSuppressionConditionSeverityArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.severity = mapped
}
/**
* @param value A `signal_type` block as defined below.
*/
@JvmName("xbujpcqdnjdmjras")
public suspend fun signalType(`value`: AlertProcessingRuleSuppressionConditionSignalTypeArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.signalType = mapped
}
/**
* @param argument A `signal_type` block as defined below.
*/
@JvmName("dfjsgdvoxhgtupsd")
public suspend
fun signalType(argument: suspend AlertProcessingRuleSuppressionConditionSignalTypeArgsBuilder.() -> Unit) {
val toBeMapped = AlertProcessingRuleSuppressionConditionSignalTypeArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.signalType = mapped
}
/**
* @param value A `target_resource` block as defined below.
*/
@JvmName("hlietjuewagrwxbs")
public suspend
fun targetResource(`value`: AlertProcessingRuleSuppressionConditionTargetResourceArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.targetResource = mapped
}
/**
* @param argument A `target_resource` block as defined below.
*/
@JvmName("rpeohioskdolnwug")
public suspend
fun targetResource(argument: suspend AlertProcessingRuleSuppressionConditionTargetResourceArgsBuilder.() -> Unit) {
val toBeMapped =
AlertProcessingRuleSuppressionConditionTargetResourceArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.targetResource = mapped
}
/**
* @param value A `target_resource_group` block as defined below.
*/
@JvmName("hrmotnbfofjayxnk")
public suspend
fun targetResourceGroup(`value`: AlertProcessingRuleSuppressionConditionTargetResourceGroupArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.targetResourceGroup = mapped
}
/**
* @param argument A `target_resource_group` block as defined below.
*/
@JvmName("kdqnmqebjtnjyqeu")
public suspend
fun targetResourceGroup(argument: suspend AlertProcessingRuleSuppressionConditionTargetResourceGroupArgsBuilder.() -> Unit) {
val toBeMapped =
AlertProcessingRuleSuppressionConditionTargetResourceGroupArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.targetResourceGroup = mapped
}
/**
* @param value A `target_resource_type` block as defined below.
*/
@JvmName("tcsdgxitevorcomr")
public suspend
fun targetResourceType(`value`: AlertProcessingRuleSuppressionConditionTargetResourceTypeArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.targetResourceType = mapped
}
/**
* @param argument A `target_resource_type` block as defined below.
*/
@JvmName("utvpqaygcfcldojj")
public suspend
fun targetResourceType(argument: suspend AlertProcessingRuleSuppressionConditionTargetResourceTypeArgsBuilder.() -> Unit) {
val toBeMapped =
AlertProcessingRuleSuppressionConditionTargetResourceTypeArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.targetResourceType = mapped
}
internal fun build(): AlertProcessingRuleSuppressionConditionArgs =
AlertProcessingRuleSuppressionConditionArgs(
alertContext = alertContext,
alertRuleId = alertRuleId,
alertRuleName = alertRuleName,
description = description,
monitorCondition = monitorCondition,
monitorService = monitorService,
severity = severity,
signalType = signalType,
targetResource = targetResource,
targetResourceGroup = targetResourceGroup,
targetResourceType = targetResourceType,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy