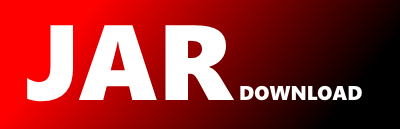
com.pulumi.azure.mssql.kotlin.inputs.ManagedDatabaseLongTermRetentionPolicyArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-kotlin Show documentation
Show all versions of pulumi-azure-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azure.mssql.kotlin.inputs
import com.pulumi.azure.mssql.inputs.ManagedDatabaseLongTermRetentionPolicyArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
*
* @property immutableBackupsEnabled Specifies if the backups are immutable. Defaults to `false`.
* @property monthlyRetention The monthly retention policy for an LTR backup in an ISO 8601 format. Valid value is between 1 to 120 months. e.g. `P1Y`, `P1M`, `P4W` or `P30D`.
* @property weekOfYear The week of year to take the yearly backup. Value has to be between `1` and `52`.
* @property weeklyRetention The weekly retention policy for an LTR backup in an ISO 8601 format. Valid value is between 1 to 520 weeks. e.g. `P1Y`, `P1M`, `P1W` or `P7D`.
* @property yearlyRetention The yearly retention policy for an LTR backup in an ISO 8601 format. Valid value is between 1 to 10 years. e.g. `P1Y`, `P12M`, `P52W` or `P365D`.
*/
public data class ManagedDatabaseLongTermRetentionPolicyArgs(
public val immutableBackupsEnabled: Output? = null,
public val monthlyRetention: Output? = null,
public val weekOfYear: Output? = null,
public val weeklyRetention: Output? = null,
public val yearlyRetention: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azure.mssql.inputs.ManagedDatabaseLongTermRetentionPolicyArgs =
com.pulumi.azure.mssql.inputs.ManagedDatabaseLongTermRetentionPolicyArgs.builder()
.immutableBackupsEnabled(immutableBackupsEnabled?.applyValue({ args0 -> args0 }))
.monthlyRetention(monthlyRetention?.applyValue({ args0 -> args0 }))
.weekOfYear(weekOfYear?.applyValue({ args0 -> args0 }))
.weeklyRetention(weeklyRetention?.applyValue({ args0 -> args0 }))
.yearlyRetention(yearlyRetention?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [ManagedDatabaseLongTermRetentionPolicyArgs].
*/
@PulumiTagMarker
public class ManagedDatabaseLongTermRetentionPolicyArgsBuilder internal constructor() {
private var immutableBackupsEnabled: Output? = null
private var monthlyRetention: Output? = null
private var weekOfYear: Output? = null
private var weeklyRetention: Output? = null
private var yearlyRetention: Output? = null
/**
* @param value Specifies if the backups are immutable. Defaults to `false`.
*/
@JvmName("crisbgqidliuxkmu")
public suspend fun immutableBackupsEnabled(`value`: Output) {
this.immutableBackupsEnabled = value
}
/**
* @param value The monthly retention policy for an LTR backup in an ISO 8601 format. Valid value is between 1 to 120 months. e.g. `P1Y`, `P1M`, `P4W` or `P30D`.
*/
@JvmName("tqwtbmslnxarijqw")
public suspend fun monthlyRetention(`value`: Output) {
this.monthlyRetention = value
}
/**
* @param value The week of year to take the yearly backup. Value has to be between `1` and `52`.
*/
@JvmName("oiqinswmmsmjivnk")
public suspend fun weekOfYear(`value`: Output) {
this.weekOfYear = value
}
/**
* @param value The weekly retention policy for an LTR backup in an ISO 8601 format. Valid value is between 1 to 520 weeks. e.g. `P1Y`, `P1M`, `P1W` or `P7D`.
*/
@JvmName("sbtaiucccxcdeaoe")
public suspend fun weeklyRetention(`value`: Output) {
this.weeklyRetention = value
}
/**
* @param value The yearly retention policy for an LTR backup in an ISO 8601 format. Valid value is between 1 to 10 years. e.g. `P1Y`, `P12M`, `P52W` or `P365D`.
*/
@JvmName("tapvlcuflbmjdfnp")
public suspend fun yearlyRetention(`value`: Output) {
this.yearlyRetention = value
}
/**
* @param value Specifies if the backups are immutable. Defaults to `false`.
*/
@JvmName("xqwfnhcsbnohesmr")
public suspend fun immutableBackupsEnabled(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.immutableBackupsEnabled = mapped
}
/**
* @param value The monthly retention policy for an LTR backup in an ISO 8601 format. Valid value is between 1 to 120 months. e.g. `P1Y`, `P1M`, `P4W` or `P30D`.
*/
@JvmName("cwbltfkemalsiatf")
public suspend fun monthlyRetention(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.monthlyRetention = mapped
}
/**
* @param value The week of year to take the yearly backup. Value has to be between `1` and `52`.
*/
@JvmName("ooerdrrxjfjqxgqu")
public suspend fun weekOfYear(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.weekOfYear = mapped
}
/**
* @param value The weekly retention policy for an LTR backup in an ISO 8601 format. Valid value is between 1 to 520 weeks. e.g. `P1Y`, `P1M`, `P1W` or `P7D`.
*/
@JvmName("aqyfehfulwuygfkj")
public suspend fun weeklyRetention(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.weeklyRetention = mapped
}
/**
* @param value The yearly retention policy for an LTR backup in an ISO 8601 format. Valid value is between 1 to 10 years. e.g. `P1Y`, `P12M`, `P52W` or `P365D`.
*/
@JvmName("gaerndbponbcillk")
public suspend fun yearlyRetention(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.yearlyRetention = mapped
}
internal fun build(): ManagedDatabaseLongTermRetentionPolicyArgs =
ManagedDatabaseLongTermRetentionPolicyArgs(
immutableBackupsEnabled = immutableBackupsEnabled,
monthlyRetention = monthlyRetention,
weekOfYear = weekOfYear,
weeklyRetention = weeklyRetention,
yearlyRetention = yearlyRetention,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy