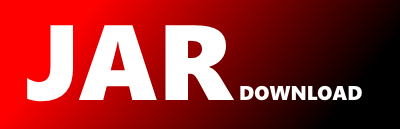
com.pulumi.azure.mssql.kotlin.inputs.ServerAzureadAdministratorArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-kotlin Show documentation
Show all versions of pulumi-azure-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azure.mssql.kotlin.inputs
import com.pulumi.azure.mssql.inputs.ServerAzureadAdministratorArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
*
* @property azureadAuthenticationOnly Specifies whether only AD Users and administrators (e.g. `azuread_administrator[0].login_username`) can be used to login, or also local database users (e.g. `administrator_login`). When `true`, the `administrator_login` and `administrator_login_password` properties can be omitted.
* @property loginUsername The login username of the Azure AD Administrator of this SQL Server.
* @property objectId The object id of the Azure AD Administrator of this SQL Server.
* @property tenantId The tenant id of the Azure AD Administrator of this SQL Server.
*/
public data class ServerAzureadAdministratorArgs(
public val azureadAuthenticationOnly: Output? = null,
public val loginUsername: Output,
public val objectId: Output,
public val tenantId: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azure.mssql.inputs.ServerAzureadAdministratorArgs =
com.pulumi.azure.mssql.inputs.ServerAzureadAdministratorArgs.builder()
.azureadAuthenticationOnly(azureadAuthenticationOnly?.applyValue({ args0 -> args0 }))
.loginUsername(loginUsername.applyValue({ args0 -> args0 }))
.objectId(objectId.applyValue({ args0 -> args0 }))
.tenantId(tenantId?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [ServerAzureadAdministratorArgs].
*/
@PulumiTagMarker
public class ServerAzureadAdministratorArgsBuilder internal constructor() {
private var azureadAuthenticationOnly: Output? = null
private var loginUsername: Output? = null
private var objectId: Output? = null
private var tenantId: Output? = null
/**
* @param value Specifies whether only AD Users and administrators (e.g. `azuread_administrator[0].login_username`) can be used to login, or also local database users (e.g. `administrator_login`). When `true`, the `administrator_login` and `administrator_login_password` properties can be omitted.
*/
@JvmName("grrhanvuhghcrbav")
public suspend fun azureadAuthenticationOnly(`value`: Output) {
this.azureadAuthenticationOnly = value
}
/**
* @param value The login username of the Azure AD Administrator of this SQL Server.
*/
@JvmName("euwgoatbuhxpqlnk")
public suspend fun loginUsername(`value`: Output) {
this.loginUsername = value
}
/**
* @param value The object id of the Azure AD Administrator of this SQL Server.
*/
@JvmName("xulxyhwmsuhosddh")
public suspend fun objectId(`value`: Output) {
this.objectId = value
}
/**
* @param value The tenant id of the Azure AD Administrator of this SQL Server.
*/
@JvmName("hqstuxvsoileyeup")
public suspend fun tenantId(`value`: Output) {
this.tenantId = value
}
/**
* @param value Specifies whether only AD Users and administrators (e.g. `azuread_administrator[0].login_username`) can be used to login, or also local database users (e.g. `administrator_login`). When `true`, the `administrator_login` and `administrator_login_password` properties can be omitted.
*/
@JvmName("nrfejsclkfmmmaau")
public suspend fun azureadAuthenticationOnly(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.azureadAuthenticationOnly = mapped
}
/**
* @param value The login username of the Azure AD Administrator of this SQL Server.
*/
@JvmName("lsdaffkcartltipa")
public suspend fun loginUsername(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.loginUsername = mapped
}
/**
* @param value The object id of the Azure AD Administrator of this SQL Server.
*/
@JvmName("ebefiidnxdjcekjc")
public suspend fun objectId(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.objectId = mapped
}
/**
* @param value The tenant id of the Azure AD Administrator of this SQL Server.
*/
@JvmName("krfavhgolhvxomwp")
public suspend fun tenantId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.tenantId = mapped
}
internal fun build(): ServerAzureadAdministratorArgs = ServerAzureadAdministratorArgs(
azureadAuthenticationOnly = azureadAuthenticationOnly,
loginUsername = loginUsername ?: throw PulumiNullFieldException("loginUsername"),
objectId = objectId ?: throw PulumiNullFieldException("objectId"),
tenantId = tenantId,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy