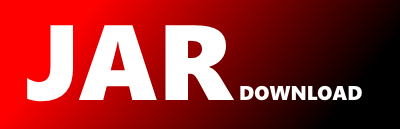
com.pulumi.azure.mssql.kotlin.inputs.VirtualMachineGroupWsfcDomainProfileArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-kotlin Show documentation
Show all versions of pulumi-azure-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azure.mssql.kotlin.inputs
import com.pulumi.azure.mssql.inputs.VirtualMachineGroupWsfcDomainProfileArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
*
* @property clusterBootstrapAccountName The account name used for creating cluster. Changing this forces a new resource to be created.
* @property clusterOperatorAccountName The account name used for operating cluster. Changing this forces a new resource to be created.
* @property clusterSubnetType The subnet type of the SQL Virtual Machine cluster. Possible values are `MultiSubnet` and `SingleSubnet`. Changing this forces a new resource to be created.
* @property fqdn The fully qualified name of the domain. Changing this forces a new resource to be created.
* @property organizationalUnitPath The organizational Unit path in which the nodes and cluster will be present. Changing this forces a new resource to be created.
* @property sqlServiceAccountName The account name under which SQL service will run on all participating SQL virtual machines in the cluster. Changing this forces a new resource to be created.
* @property storageAccountPrimaryKey The primary key of the Storage Account.
* @property storageAccountUrl The SAS URL to the Storage Container of the witness storage account. Changing this forces a new resource to be created.
*/
public data class VirtualMachineGroupWsfcDomainProfileArgs(
public val clusterBootstrapAccountName: Output? = null,
public val clusterOperatorAccountName: Output? = null,
public val clusterSubnetType: Output,
public val fqdn: Output,
public val organizationalUnitPath: Output? = null,
public val sqlServiceAccountName: Output? = null,
public val storageAccountPrimaryKey: Output? = null,
public val storageAccountUrl: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azure.mssql.inputs.VirtualMachineGroupWsfcDomainProfileArgs =
com.pulumi.azure.mssql.inputs.VirtualMachineGroupWsfcDomainProfileArgs.builder()
.clusterBootstrapAccountName(clusterBootstrapAccountName?.applyValue({ args0 -> args0 }))
.clusterOperatorAccountName(clusterOperatorAccountName?.applyValue({ args0 -> args0 }))
.clusterSubnetType(clusterSubnetType.applyValue({ args0 -> args0 }))
.fqdn(fqdn.applyValue({ args0 -> args0 }))
.organizationalUnitPath(organizationalUnitPath?.applyValue({ args0 -> args0 }))
.sqlServiceAccountName(sqlServiceAccountName?.applyValue({ args0 -> args0 }))
.storageAccountPrimaryKey(storageAccountPrimaryKey?.applyValue({ args0 -> args0 }))
.storageAccountUrl(storageAccountUrl?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [VirtualMachineGroupWsfcDomainProfileArgs].
*/
@PulumiTagMarker
public class VirtualMachineGroupWsfcDomainProfileArgsBuilder internal constructor() {
private var clusterBootstrapAccountName: Output? = null
private var clusterOperatorAccountName: Output? = null
private var clusterSubnetType: Output? = null
private var fqdn: Output? = null
private var organizationalUnitPath: Output? = null
private var sqlServiceAccountName: Output? = null
private var storageAccountPrimaryKey: Output? = null
private var storageAccountUrl: Output? = null
/**
* @param value The account name used for creating cluster. Changing this forces a new resource to be created.
*/
@JvmName("ittlppahjtaboykc")
public suspend fun clusterBootstrapAccountName(`value`: Output) {
this.clusterBootstrapAccountName = value
}
/**
* @param value The account name used for operating cluster. Changing this forces a new resource to be created.
*/
@JvmName("kiefieaknwbaqliu")
public suspend fun clusterOperatorAccountName(`value`: Output) {
this.clusterOperatorAccountName = value
}
/**
* @param value The subnet type of the SQL Virtual Machine cluster. Possible values are `MultiSubnet` and `SingleSubnet`. Changing this forces a new resource to be created.
*/
@JvmName("atuwatqiwvwgbnjg")
public suspend fun clusterSubnetType(`value`: Output) {
this.clusterSubnetType = value
}
/**
* @param value The fully qualified name of the domain. Changing this forces a new resource to be created.
*/
@JvmName("jfrchyahfupltnip")
public suspend fun fqdn(`value`: Output) {
this.fqdn = value
}
/**
* @param value The organizational Unit path in which the nodes and cluster will be present. Changing this forces a new resource to be created.
*/
@JvmName("ijjedawxoejmdtmv")
public suspend fun organizationalUnitPath(`value`: Output) {
this.organizationalUnitPath = value
}
/**
* @param value The account name under which SQL service will run on all participating SQL virtual machines in the cluster. Changing this forces a new resource to be created.
*/
@JvmName("lywpskrnekmrqjdo")
public suspend fun sqlServiceAccountName(`value`: Output) {
this.sqlServiceAccountName = value
}
/**
* @param value The primary key of the Storage Account.
*/
@JvmName("jppjmjgreldiriuw")
public suspend fun storageAccountPrimaryKey(`value`: Output) {
this.storageAccountPrimaryKey = value
}
/**
* @param value The SAS URL to the Storage Container of the witness storage account. Changing this forces a new resource to be created.
*/
@JvmName("ffcofnmrjphugtfi")
public suspend fun storageAccountUrl(`value`: Output) {
this.storageAccountUrl = value
}
/**
* @param value The account name used for creating cluster. Changing this forces a new resource to be created.
*/
@JvmName("ofayfgyihwvhoncy")
public suspend fun clusterBootstrapAccountName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.clusterBootstrapAccountName = mapped
}
/**
* @param value The account name used for operating cluster. Changing this forces a new resource to be created.
*/
@JvmName("qlcekrtxgkapilgn")
public suspend fun clusterOperatorAccountName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.clusterOperatorAccountName = mapped
}
/**
* @param value The subnet type of the SQL Virtual Machine cluster. Possible values are `MultiSubnet` and `SingleSubnet`. Changing this forces a new resource to be created.
*/
@JvmName("fnbveoyynahpxgyg")
public suspend fun clusterSubnetType(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.clusterSubnetType = mapped
}
/**
* @param value The fully qualified name of the domain. Changing this forces a new resource to be created.
*/
@JvmName("kptwlwgcftwaorxu")
public suspend fun fqdn(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.fqdn = mapped
}
/**
* @param value The organizational Unit path in which the nodes and cluster will be present. Changing this forces a new resource to be created.
*/
@JvmName("yjsmxqndfnjbesso")
public suspend fun organizationalUnitPath(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.organizationalUnitPath = mapped
}
/**
* @param value The account name under which SQL service will run on all participating SQL virtual machines in the cluster. Changing this forces a new resource to be created.
*/
@JvmName("hntdtuvhhyygthda")
public suspend fun sqlServiceAccountName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.sqlServiceAccountName = mapped
}
/**
* @param value The primary key of the Storage Account.
*/
@JvmName("mgtaokojnmgdicid")
public suspend fun storageAccountPrimaryKey(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.storageAccountPrimaryKey = mapped
}
/**
* @param value The SAS URL to the Storage Container of the witness storage account. Changing this forces a new resource to be created.
*/
@JvmName("smpeidihbhorolif")
public suspend fun storageAccountUrl(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.storageAccountUrl = mapped
}
internal fun build(): VirtualMachineGroupWsfcDomainProfileArgs =
VirtualMachineGroupWsfcDomainProfileArgs(
clusterBootstrapAccountName = clusterBootstrapAccountName,
clusterOperatorAccountName = clusterOperatorAccountName,
clusterSubnetType = clusterSubnetType ?: throw PulumiNullFieldException("clusterSubnetType"),
fqdn = fqdn ?: throw PulumiNullFieldException("fqdn"),
organizationalUnitPath = organizationalUnitPath,
sqlServiceAccountName = sqlServiceAccountName,
storageAccountPrimaryKey = storageAccountPrimaryKey,
storageAccountUrl = storageAccountUrl,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy