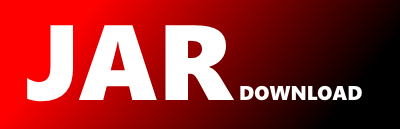
com.pulumi.azure.netapp.kotlin.inputs.VolumeExportPolicyRuleArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-kotlin Show documentation
Show all versions of pulumi-azure-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azure.netapp.kotlin.inputs
import com.pulumi.azure.netapp.inputs.VolumeExportPolicyRuleArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
*
* @property allowedClients A list of allowed clients IPv4 addresses.
* @property kerberos5ReadOnlyEnabled Is Kerberos 5 read-only access permitted to this volume?
* @property kerberos5ReadWriteEnabled Is Kerberos 5 read/write permitted to this volume?
* @property kerberos5iReadOnlyEnabled Is Kerberos 5i read-only permitted to this volume?
* @property kerberos5iReadWriteEnabled Is Kerberos 5i read/write permitted to this volume?
* @property kerberos5pReadOnlyEnabled Is Kerberos 5p read-only permitted to this volume?
* @property kerberos5pReadWriteEnabled Is Kerberos 5p read/write permitted to this volume?
* @property protocolsEnabled A list of allowed protocols. Valid values include `CIFS`, `NFSv3`, or `NFSv4.1`. Only one value is supported at this time. This replaces the previous arguments: `cifs_enabled`, `nfsv3_enabled` and `nfsv4_enabled`.
* @property rootAccessEnabled Is root access permitted to this volume?
* @property ruleIndex The index number of the rule.
* @property unixReadOnly Is the file system on unix read only?
* @property unixReadWrite Is the file system on unix read and write?
*/
public data class VolumeExportPolicyRuleArgs(
public val allowedClients: Output>,
public val kerberos5ReadOnlyEnabled: Output? = null,
public val kerberos5ReadWriteEnabled: Output? = null,
public val kerberos5iReadOnlyEnabled: Output? = null,
public val kerberos5iReadWriteEnabled: Output? = null,
public val kerberos5pReadOnlyEnabled: Output? = null,
public val kerberos5pReadWriteEnabled: Output? = null,
public val protocolsEnabled: Output? = null,
public val rootAccessEnabled: Output? = null,
public val ruleIndex: Output,
public val unixReadOnly: Output? = null,
public val unixReadWrite: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azure.netapp.inputs.VolumeExportPolicyRuleArgs =
com.pulumi.azure.netapp.inputs.VolumeExportPolicyRuleArgs.builder()
.allowedClients(allowedClients.applyValue({ args0 -> args0.map({ args0 -> args0 }) }))
.kerberos5ReadOnlyEnabled(kerberos5ReadOnlyEnabled?.applyValue({ args0 -> args0 }))
.kerberos5ReadWriteEnabled(kerberos5ReadWriteEnabled?.applyValue({ args0 -> args0 }))
.kerberos5iReadOnlyEnabled(kerberos5iReadOnlyEnabled?.applyValue({ args0 -> args0 }))
.kerberos5iReadWriteEnabled(kerberos5iReadWriteEnabled?.applyValue({ args0 -> args0 }))
.kerberos5pReadOnlyEnabled(kerberos5pReadOnlyEnabled?.applyValue({ args0 -> args0 }))
.kerberos5pReadWriteEnabled(kerberos5pReadWriteEnabled?.applyValue({ args0 -> args0 }))
.protocolsEnabled(protocolsEnabled?.applyValue({ args0 -> args0 }))
.rootAccessEnabled(rootAccessEnabled?.applyValue({ args0 -> args0 }))
.ruleIndex(ruleIndex.applyValue({ args0 -> args0 }))
.unixReadOnly(unixReadOnly?.applyValue({ args0 -> args0 }))
.unixReadWrite(unixReadWrite?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [VolumeExportPolicyRuleArgs].
*/
@PulumiTagMarker
public class VolumeExportPolicyRuleArgsBuilder internal constructor() {
private var allowedClients: Output>? = null
private var kerberos5ReadOnlyEnabled: Output? = null
private var kerberos5ReadWriteEnabled: Output? = null
private var kerberos5iReadOnlyEnabled: Output? = null
private var kerberos5iReadWriteEnabled: Output? = null
private var kerberos5pReadOnlyEnabled: Output? = null
private var kerberos5pReadWriteEnabled: Output? = null
private var protocolsEnabled: Output? = null
private var rootAccessEnabled: Output? = null
private var ruleIndex: Output? = null
private var unixReadOnly: Output? = null
private var unixReadWrite: Output? = null
/**
* @param value A list of allowed clients IPv4 addresses.
*/
@JvmName("ttkojefugwjhphww")
public suspend fun allowedClients(`value`: Output>) {
this.allowedClients = value
}
@JvmName("vjktqmffoikekksm")
public suspend fun allowedClients(vararg values: Output) {
this.allowedClients = Output.all(values.asList())
}
/**
* @param values A list of allowed clients IPv4 addresses.
*/
@JvmName("yntaqapecwyjqofn")
public suspend fun allowedClients(values: List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy