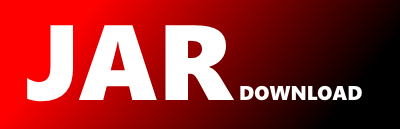
com.pulumi.azure.netapp.kotlin.outputs.AccountActiveDirectory.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-kotlin Show documentation
Show all versions of pulumi-azure-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azure.netapp.kotlin.outputs
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
/**
*
* @property aesEncryptionEnabled If enabled, AES encryption will be enabled for SMB communication. Defaults to `false`.
* @property dnsServers A list of DNS server IP addresses for the Active Directory domain. Only allows `IPv4` address.
* @property domain The name of the Active Directory domain.
* @property kerberosAdName Name of the active directory machine.
* @property kerberosKdcIp kdc server IP addresses for the active directory machine.
* > **IMPORTANT:** If you plan on using **Kerberos** volumes, both `ad_name` and `kdc_ip` are required in order to create the volume.
* @property ldapOverTlsEnabled Specifies whether or not the LDAP traffic needs to be secured via TLS. Defaults to `false`.
* @property ldapSigningEnabled Specifies whether or not the LDAP traffic needs to be signed. Defaults to `false`.
* @property localNfsUsersWithLdapAllowed If enabled, NFS client local users can also (in addition to LDAP users) access the NFS volumes. Defaults to `false`.
* @property organizationalUnit The Organizational Unit (OU) within Active Directory where machines will be created. If blank, defaults to `CN=Computers`.
* @property password The password associated with the `username`.
* @property serverRootCaCertificate When LDAP over SSL/TLS is enabled, the LDAP client is required to have a *base64 encoded Active Directory Certificate Service's self-signed root CA certificate*, this optional parameter is used only for dual protocol with LDAP user-mapping volumes. Required if `ldap_over_tls_enabled` is set to `true`.
* @property siteName The Active Directory site the service will limit Domain Controller discovery to. If blank, defaults to `Default-First-Site-Name`.
* @property smbServerName The NetBIOS name which should be used for the NetApp SMB Server, which will be registered as a computer account in the AD and used to mount volumes.
* @property username The Username of Active Directory Domain Administrator.
*/
public data class AccountActiveDirectory(
public val aesEncryptionEnabled: Boolean? = null,
public val dnsServers: List,
public val domain: String,
public val kerberosAdName: String? = null,
public val kerberosKdcIp: String? = null,
public val ldapOverTlsEnabled: Boolean? = null,
public val ldapSigningEnabled: Boolean? = null,
public val localNfsUsersWithLdapAllowed: Boolean? = null,
public val organizationalUnit: String? = null,
public val password: String,
public val serverRootCaCertificate: String? = null,
public val siteName: String? = null,
public val smbServerName: String,
public val username: String,
) {
public companion object {
public fun toKotlin(javaType: com.pulumi.azure.netapp.outputs.AccountActiveDirectory):
AccountActiveDirectory = AccountActiveDirectory(
aesEncryptionEnabled = javaType.aesEncryptionEnabled().map({ args0 -> args0 }).orElse(null),
dnsServers = javaType.dnsServers().map({ args0 -> args0 }),
domain = javaType.domain(),
kerberosAdName = javaType.kerberosAdName().map({ args0 -> args0 }).orElse(null),
kerberosKdcIp = javaType.kerberosKdcIp().map({ args0 -> args0 }).orElse(null),
ldapOverTlsEnabled = javaType.ldapOverTlsEnabled().map({ args0 -> args0 }).orElse(null),
ldapSigningEnabled = javaType.ldapSigningEnabled().map({ args0 -> args0 }).orElse(null),
localNfsUsersWithLdapAllowed = javaType.localNfsUsersWithLdapAllowed().map({ args0 ->
args0
}).orElse(null),
organizationalUnit = javaType.organizationalUnit().map({ args0 -> args0 }).orElse(null),
password = javaType.password(),
serverRootCaCertificate = javaType.serverRootCaCertificate().map({ args0 -> args0 }).orElse(null),
siteName = javaType.siteName().map({ args0 -> args0 }).orElse(null),
smbServerName = javaType.smbServerName(),
username = javaType.username(),
)
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy