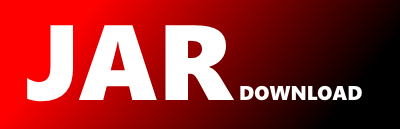
com.pulumi.azure.network.kotlin.ExpressRouteConnectionArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-kotlin Show documentation
Show all versions of pulumi-azure-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azure.network.kotlin
import com.pulumi.azure.network.ExpressRouteConnectionArgs.builder
import com.pulumi.azure.network.kotlin.inputs.ExpressRouteConnectionRoutingArgs
import com.pulumi.azure.network.kotlin.inputs.ExpressRouteConnectionRoutingArgsBuilder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
* Manages an Express Route Connection.
* > **NOTE:** The provider status of the Express Route Circuit must be set as provisioned while creating the Express Route Connection. See more details [here](https://docs.microsoft.com/azure/expressroute/expressroute-howto-circuit-portal-resource-manager#send-the-service-key-to-your-connectivity-provider-for-provisioning).
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as azure from "@pulumi/azure";
* const example = new azure.core.ResourceGroup("example", {
* name: "example-resources",
* location: "West Europe",
* });
* const exampleVirtualWan = new azure.network.VirtualWan("example", {
* name: "example-vwan",
* resourceGroupName: example.name,
* location: example.location,
* });
* const exampleVirtualHub = new azure.network.VirtualHub("example", {
* name: "example-vhub",
* resourceGroupName: example.name,
* location: example.location,
* virtualWanId: exampleVirtualWan.id,
* addressPrefix: "10.0.1.0/24",
* });
* const exampleExpressRouteGateway = new azure.network.ExpressRouteGateway("example", {
* name: "example-expressroutegateway",
* resourceGroupName: example.name,
* location: example.location,
* virtualHubId: exampleVirtualHub.id,
* scaleUnits: 1,
* });
* const exampleExpressRoutePort = new azure.network.ExpressRoutePort("example", {
* name: "example-erp",
* resourceGroupName: example.name,
* location: example.location,
* peeringLocation: "Equinix-Seattle-SE2",
* bandwidthInGbps: 10,
* encapsulation: "Dot1Q",
* });
* const exampleExpressRouteCircuit = new azure.network.ExpressRouteCircuit("example", {
* name: "example-erc",
* location: example.location,
* resourceGroupName: example.name,
* expressRoutePortId: exampleExpressRoutePort.id,
* bandwidthInGbps: 5,
* sku: {
* tier: "Standard",
* family: "MeteredData",
* },
* });
* const exampleExpressRouteCircuitPeering = new azure.network.ExpressRouteCircuitPeering("example", {
* peeringType: "AzurePrivatePeering",
* expressRouteCircuitName: exampleExpressRouteCircuit.name,
* resourceGroupName: example.name,
* sharedKey: "ItsASecret",
* peerAsn: 100,
* primaryPeerAddressPrefix: "192.168.1.0/30",
* secondaryPeerAddressPrefix: "192.168.2.0/30",
* vlanId: 100,
* });
* const exampleExpressRouteConnection = new azure.network.ExpressRouteConnection("example", {
* name: "example-expressrouteconn",
* expressRouteGatewayId: exampleExpressRouteGateway.id,
* expressRouteCircuitPeeringId: exampleExpressRouteCircuitPeering.id,
* });
* ```
* ```python
* import pulumi
* import pulumi_azure as azure
* example = azure.core.ResourceGroup("example",
* name="example-resources",
* location="West Europe")
* example_virtual_wan = azure.network.VirtualWan("example",
* name="example-vwan",
* resource_group_name=example.name,
* location=example.location)
* example_virtual_hub = azure.network.VirtualHub("example",
* name="example-vhub",
* resource_group_name=example.name,
* location=example.location,
* virtual_wan_id=example_virtual_wan.id,
* address_prefix="10.0.1.0/24")
* example_express_route_gateway = azure.network.ExpressRouteGateway("example",
* name="example-expressroutegateway",
* resource_group_name=example.name,
* location=example.location,
* virtual_hub_id=example_virtual_hub.id,
* scale_units=1)
* example_express_route_port = azure.network.ExpressRoutePort("example",
* name="example-erp",
* resource_group_name=example.name,
* location=example.location,
* peering_location="Equinix-Seattle-SE2",
* bandwidth_in_gbps=10,
* encapsulation="Dot1Q")
* example_express_route_circuit = azure.network.ExpressRouteCircuit("example",
* name="example-erc",
* location=example.location,
* resource_group_name=example.name,
* express_route_port_id=example_express_route_port.id,
* bandwidth_in_gbps=5,
* sku=azure.network.ExpressRouteCircuitSkuArgs(
* tier="Standard",
* family="MeteredData",
* ))
* example_express_route_circuit_peering = azure.network.ExpressRouteCircuitPeering("example",
* peering_type="AzurePrivatePeering",
* express_route_circuit_name=example_express_route_circuit.name,
* resource_group_name=example.name,
* shared_key="ItsASecret",
* peer_asn=100,
* primary_peer_address_prefix="192.168.1.0/30",
* secondary_peer_address_prefix="192.168.2.0/30",
* vlan_id=100)
* example_express_route_connection = azure.network.ExpressRouteConnection("example",
* name="example-expressrouteconn",
* express_route_gateway_id=example_express_route_gateway.id,
* express_route_circuit_peering_id=example_express_route_circuit_peering.id)
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Azure = Pulumi.Azure;
* return await Deployment.RunAsync(() =>
* {
* var example = new Azure.Core.ResourceGroup("example", new()
* {
* Name = "example-resources",
* Location = "West Europe",
* });
* var exampleVirtualWan = new Azure.Network.VirtualWan("example", new()
* {
* Name = "example-vwan",
* ResourceGroupName = example.Name,
* Location = example.Location,
* });
* var exampleVirtualHub = new Azure.Network.VirtualHub("example", new()
* {
* Name = "example-vhub",
* ResourceGroupName = example.Name,
* Location = example.Location,
* VirtualWanId = exampleVirtualWan.Id,
* AddressPrefix = "10.0.1.0/24",
* });
* var exampleExpressRouteGateway = new Azure.Network.ExpressRouteGateway("example", new()
* {
* Name = "example-expressroutegateway",
* ResourceGroupName = example.Name,
* Location = example.Location,
* VirtualHubId = exampleVirtualHub.Id,
* ScaleUnits = 1,
* });
* var exampleExpressRoutePort = new Azure.Network.ExpressRoutePort("example", new()
* {
* Name = "example-erp",
* ResourceGroupName = example.Name,
* Location = example.Location,
* PeeringLocation = "Equinix-Seattle-SE2",
* BandwidthInGbps = 10,
* Encapsulation = "Dot1Q",
* });
* var exampleExpressRouteCircuit = new Azure.Network.ExpressRouteCircuit("example", new()
* {
* Name = "example-erc",
* Location = example.Location,
* ResourceGroupName = example.Name,
* ExpressRoutePortId = exampleExpressRoutePort.Id,
* BandwidthInGbps = 5,
* Sku = new Azure.Network.Inputs.ExpressRouteCircuitSkuArgs
* {
* Tier = "Standard",
* Family = "MeteredData",
* },
* });
* var exampleExpressRouteCircuitPeering = new Azure.Network.ExpressRouteCircuitPeering("example", new()
* {
* PeeringType = "AzurePrivatePeering",
* ExpressRouteCircuitName = exampleExpressRouteCircuit.Name,
* ResourceGroupName = example.Name,
* SharedKey = "ItsASecret",
* PeerAsn = 100,
* PrimaryPeerAddressPrefix = "192.168.1.0/30",
* SecondaryPeerAddressPrefix = "192.168.2.0/30",
* VlanId = 100,
* });
* var exampleExpressRouteConnection = new Azure.Network.ExpressRouteConnection("example", new()
* {
* Name = "example-expressrouteconn",
* ExpressRouteGatewayId = exampleExpressRouteGateway.Id,
* ExpressRouteCircuitPeeringId = exampleExpressRouteCircuitPeering.Id,
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-azure/sdk/v5/go/azure/core"
* "github.com/pulumi/pulumi-azure/sdk/v5/go/azure/network"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* example, err := core.NewResourceGroup(ctx, "example", &core.ResourceGroupArgs{
* Name: pulumi.String("example-resources"),
* Location: pulumi.String("West Europe"),
* })
* if err != nil {
* return err
* }
* exampleVirtualWan, err := network.NewVirtualWan(ctx, "example", &network.VirtualWanArgs{
* Name: pulumi.String("example-vwan"),
* ResourceGroupName: example.Name,
* Location: example.Location,
* })
* if err != nil {
* return err
* }
* exampleVirtualHub, err := network.NewVirtualHub(ctx, "example", &network.VirtualHubArgs{
* Name: pulumi.String("example-vhub"),
* ResourceGroupName: example.Name,
* Location: example.Location,
* VirtualWanId: exampleVirtualWan.ID(),
* AddressPrefix: pulumi.String("10.0.1.0/24"),
* })
* if err != nil {
* return err
* }
* exampleExpressRouteGateway, err := network.NewExpressRouteGateway(ctx, "example", &network.ExpressRouteGatewayArgs{
* Name: pulumi.String("example-expressroutegateway"),
* ResourceGroupName: example.Name,
* Location: example.Location,
* VirtualHubId: exampleVirtualHub.ID(),
* ScaleUnits: pulumi.Int(1),
* })
* if err != nil {
* return err
* }
* exampleExpressRoutePort, err := network.NewExpressRoutePort(ctx, "example", &network.ExpressRoutePortArgs{
* Name: pulumi.String("example-erp"),
* ResourceGroupName: example.Name,
* Location: example.Location,
* PeeringLocation: pulumi.String("Equinix-Seattle-SE2"),
* BandwidthInGbps: pulumi.Int(10),
* Encapsulation: pulumi.String("Dot1Q"),
* })
* if err != nil {
* return err
* }
* exampleExpressRouteCircuit, err := network.NewExpressRouteCircuit(ctx, "example", &network.ExpressRouteCircuitArgs{
* Name: pulumi.String("example-erc"),
* Location: example.Location,
* ResourceGroupName: example.Name,
* ExpressRoutePortId: exampleExpressRoutePort.ID(),
* BandwidthInGbps: pulumi.Float64(5),
* Sku: &network.ExpressRouteCircuitSkuArgs{
* Tier: pulumi.String("Standard"),
* Family: pulumi.String("MeteredData"),
* },
* })
* if err != nil {
* return err
* }
* exampleExpressRouteCircuitPeering, err := network.NewExpressRouteCircuitPeering(ctx, "example", &network.ExpressRouteCircuitPeeringArgs{
* PeeringType: pulumi.String("AzurePrivatePeering"),
* ExpressRouteCircuitName: exampleExpressRouteCircuit.Name,
* ResourceGroupName: example.Name,
* SharedKey: pulumi.String("ItsASecret"),
* PeerAsn: pulumi.Int(100),
* PrimaryPeerAddressPrefix: pulumi.String("192.168.1.0/30"),
* SecondaryPeerAddressPrefix: pulumi.String("192.168.2.0/30"),
* VlanId: pulumi.Int(100),
* })
* if err != nil {
* return err
* }
* _, err = network.NewExpressRouteConnection(ctx, "example", &network.ExpressRouteConnectionArgs{
* Name: pulumi.String("example-expressrouteconn"),
* ExpressRouteGatewayId: exampleExpressRouteGateway.ID(),
* ExpressRouteCircuitPeeringId: exampleExpressRouteCircuitPeering.ID(),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azure.core.ResourceGroup;
* import com.pulumi.azure.core.ResourceGroupArgs;
* import com.pulumi.azure.network.VirtualWan;
* import com.pulumi.azure.network.VirtualWanArgs;
* import com.pulumi.azure.network.VirtualHub;
* import com.pulumi.azure.network.VirtualHubArgs;
* import com.pulumi.azure.network.ExpressRouteGateway;
* import com.pulumi.azure.network.ExpressRouteGatewayArgs;
* import com.pulumi.azure.network.ExpressRoutePort;
* import com.pulumi.azure.network.ExpressRoutePortArgs;
* import com.pulumi.azure.network.ExpressRouteCircuit;
* import com.pulumi.azure.network.ExpressRouteCircuitArgs;
* import com.pulumi.azure.network.inputs.ExpressRouteCircuitSkuArgs;
* import com.pulumi.azure.network.ExpressRouteCircuitPeering;
* import com.pulumi.azure.network.ExpressRouteCircuitPeeringArgs;
* import com.pulumi.azure.network.ExpressRouteConnection;
* import com.pulumi.azure.network.ExpressRouteConnectionArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var example = new ResourceGroup("example", ResourceGroupArgs.builder()
* .name("example-resources")
* .location("West Europe")
* .build());
* var exampleVirtualWan = new VirtualWan("exampleVirtualWan", VirtualWanArgs.builder()
* .name("example-vwan")
* .resourceGroupName(example.name())
* .location(example.location())
* .build());
* var exampleVirtualHub = new VirtualHub("exampleVirtualHub", VirtualHubArgs.builder()
* .name("example-vhub")
* .resourceGroupName(example.name())
* .location(example.location())
* .virtualWanId(exampleVirtualWan.id())
* .addressPrefix("10.0.1.0/24")
* .build());
* var exampleExpressRouteGateway = new ExpressRouteGateway("exampleExpressRouteGateway", ExpressRouteGatewayArgs.builder()
* .name("example-expressroutegateway")
* .resourceGroupName(example.name())
* .location(example.location())
* .virtualHubId(exampleVirtualHub.id())
* .scaleUnits(1)
* .build());
* var exampleExpressRoutePort = new ExpressRoutePort("exampleExpressRoutePort", ExpressRoutePortArgs.builder()
* .name("example-erp")
* .resourceGroupName(example.name())
* .location(example.location())
* .peeringLocation("Equinix-Seattle-SE2")
* .bandwidthInGbps(10)
* .encapsulation("Dot1Q")
* .build());
* var exampleExpressRouteCircuit = new ExpressRouteCircuit("exampleExpressRouteCircuit", ExpressRouteCircuitArgs.builder()
* .name("example-erc")
* .location(example.location())
* .resourceGroupName(example.name())
* .expressRoutePortId(exampleExpressRoutePort.id())
* .bandwidthInGbps(5)
* .sku(ExpressRouteCircuitSkuArgs.builder()
* .tier("Standard")
* .family("MeteredData")
* .build())
* .build());
* var exampleExpressRouteCircuitPeering = new ExpressRouteCircuitPeering("exampleExpressRouteCircuitPeering", ExpressRouteCircuitPeeringArgs.builder()
* .peeringType("AzurePrivatePeering")
* .expressRouteCircuitName(exampleExpressRouteCircuit.name())
* .resourceGroupName(example.name())
* .sharedKey("ItsASecret")
* .peerAsn(100)
* .primaryPeerAddressPrefix("192.168.1.0/30")
* .secondaryPeerAddressPrefix("192.168.2.0/30")
* .vlanId(100)
* .build());
* var exampleExpressRouteConnection = new ExpressRouteConnection("exampleExpressRouteConnection", ExpressRouteConnectionArgs.builder()
* .name("example-expressrouteconn")
* .expressRouteGatewayId(exampleExpressRouteGateway.id())
* .expressRouteCircuitPeeringId(exampleExpressRouteCircuitPeering.id())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* example:
* type: azure:core:ResourceGroup
* properties:
* name: example-resources
* location: West Europe
* exampleVirtualWan:
* type: azure:network:VirtualWan
* name: example
* properties:
* name: example-vwan
* resourceGroupName: ${example.name}
* location: ${example.location}
* exampleVirtualHub:
* type: azure:network:VirtualHub
* name: example
* properties:
* name: example-vhub
* resourceGroupName: ${example.name}
* location: ${example.location}
* virtualWanId: ${exampleVirtualWan.id}
* addressPrefix: 10.0.1.0/24
* exampleExpressRouteGateway:
* type: azure:network:ExpressRouteGateway
* name: example
* properties:
* name: example-expressroutegateway
* resourceGroupName: ${example.name}
* location: ${example.location}
* virtualHubId: ${exampleVirtualHub.id}
* scaleUnits: 1
* exampleExpressRoutePort:
* type: azure:network:ExpressRoutePort
* name: example
* properties:
* name: example-erp
* resourceGroupName: ${example.name}
* location: ${example.location}
* peeringLocation: Equinix-Seattle-SE2
* bandwidthInGbps: 10
* encapsulation: Dot1Q
* exampleExpressRouteCircuit:
* type: azure:network:ExpressRouteCircuit
* name: example
* properties:
* name: example-erc
* location: ${example.location}
* resourceGroupName: ${example.name}
* expressRoutePortId: ${exampleExpressRoutePort.id}
* bandwidthInGbps: 5
* sku:
* tier: Standard
* family: MeteredData
* exampleExpressRouteCircuitPeering:
* type: azure:network:ExpressRouteCircuitPeering
* name: example
* properties:
* peeringType: AzurePrivatePeering
* expressRouteCircuitName: ${exampleExpressRouteCircuit.name}
* resourceGroupName: ${example.name}
* sharedKey: ItsASecret
* peerAsn: 100
* primaryPeerAddressPrefix: 192.168.1.0/30
* secondaryPeerAddressPrefix: 192.168.2.0/30
* vlanId: 100
* exampleExpressRouteConnection:
* type: azure:network:ExpressRouteConnection
* name: example
* properties:
* name: example-expressrouteconn
* expressRouteGatewayId: ${exampleExpressRouteGateway.id}
* expressRouteCircuitPeeringId: ${exampleExpressRouteCircuitPeering.id}
* ```
*
* ## Import
* Express Route Connections can be imported using the `resource id`, e.g.
* ```sh
* $ pulumi import azure:network/expressRouteConnection:ExpressRouteConnection example /subscriptions/00000000-0000-0000-0000-000000000000/resourceGroups/group1/providers/Microsoft.Network/expressRouteGateways/expressRouteGateway1/expressRouteConnections/connection1
* ```
* @property authorizationKey The authorization key to establish the Express Route Connection.
* @property enableInternetSecurity Is Internet security enabled for this Express Route Connection?
* @property expressRouteCircuitPeeringId The ID of the Express Route Circuit Peering that this Express Route Connection connects with. Changing this forces a new resource to be created.
* @property expressRouteGatewayBypassEnabled Specified whether Fast Path is enabled for Virtual Wan Firewall Hub. Defaults to `false`.
* @property expressRouteGatewayId The ID of the Express Route Gateway that this Express Route Connection connects with. Changing this forces a new resource to be created.
* @property name The name which should be used for this Express Route Connection. Changing this forces a new resource to be created.
* @property privateLinkFastPathEnabled Bypass the Express Route gateway when accessing private-links. When enabled `express_route_gateway_bypass_enabled` must be set to `true`. Defaults to `false`.
* @property routing A `routing` block as defined below.
* @property routingWeight The routing weight associated to the Express Route Connection. Possible value is between `0` and `32000`. Defaults to `0`.
*/
public data class ExpressRouteConnectionArgs(
public val authorizationKey: Output? = null,
public val enableInternetSecurity: Output? = null,
public val expressRouteCircuitPeeringId: Output? = null,
public val expressRouteGatewayBypassEnabled: Output? = null,
public val expressRouteGatewayId: Output? = null,
public val name: Output? = null,
public val privateLinkFastPathEnabled: Output? = null,
public val routing: Output? = null,
public val routingWeight: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azure.network.ExpressRouteConnectionArgs =
com.pulumi.azure.network.ExpressRouteConnectionArgs.builder()
.authorizationKey(authorizationKey?.applyValue({ args0 -> args0 }))
.enableInternetSecurity(enableInternetSecurity?.applyValue({ args0 -> args0 }))
.expressRouteCircuitPeeringId(expressRouteCircuitPeeringId?.applyValue({ args0 -> args0 }))
.expressRouteGatewayBypassEnabled(expressRouteGatewayBypassEnabled?.applyValue({ args0 -> args0 }))
.expressRouteGatewayId(expressRouteGatewayId?.applyValue({ args0 -> args0 }))
.name(name?.applyValue({ args0 -> args0 }))
.privateLinkFastPathEnabled(privateLinkFastPathEnabled?.applyValue({ args0 -> args0 }))
.routing(routing?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.routingWeight(routingWeight?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [ExpressRouteConnectionArgs].
*/
@PulumiTagMarker
public class ExpressRouteConnectionArgsBuilder internal constructor() {
private var authorizationKey: Output? = null
private var enableInternetSecurity: Output? = null
private var expressRouteCircuitPeeringId: Output? = null
private var expressRouteGatewayBypassEnabled: Output? = null
private var expressRouteGatewayId: Output? = null
private var name: Output? = null
private var privateLinkFastPathEnabled: Output? = null
private var routing: Output? = null
private var routingWeight: Output? = null
/**
* @param value The authorization key to establish the Express Route Connection.
*/
@JvmName("oqpvlwadesookcwg")
public suspend fun authorizationKey(`value`: Output) {
this.authorizationKey = value
}
/**
* @param value Is Internet security enabled for this Express Route Connection?
*/
@JvmName("ndkfderiwetrptoj")
public suspend fun enableInternetSecurity(`value`: Output) {
this.enableInternetSecurity = value
}
/**
* @param value The ID of the Express Route Circuit Peering that this Express Route Connection connects with. Changing this forces a new resource to be created.
*/
@JvmName("lptlmpbiulrulkwv")
public suspend fun expressRouteCircuitPeeringId(`value`: Output) {
this.expressRouteCircuitPeeringId = value
}
/**
* @param value Specified whether Fast Path is enabled for Virtual Wan Firewall Hub. Defaults to `false`.
*/
@JvmName("takoxwnbvuimvfyt")
public suspend fun expressRouteGatewayBypassEnabled(`value`: Output) {
this.expressRouteGatewayBypassEnabled = value
}
/**
* @param value The ID of the Express Route Gateway that this Express Route Connection connects with. Changing this forces a new resource to be created.
*/
@JvmName("qemudgefxssyeloa")
public suspend fun expressRouteGatewayId(`value`: Output) {
this.expressRouteGatewayId = value
}
/**
* @param value The name which should be used for this Express Route Connection. Changing this forces a new resource to be created.
*/
@JvmName("nkwcyvxkgurgtdtr")
public suspend fun name(`value`: Output) {
this.name = value
}
/**
* @param value Bypass the Express Route gateway when accessing private-links. When enabled `express_route_gateway_bypass_enabled` must be set to `true`. Defaults to `false`.
*/
@JvmName("elcegvapnisjgklv")
public suspend fun privateLinkFastPathEnabled(`value`: Output) {
this.privateLinkFastPathEnabled = value
}
/**
* @param value A `routing` block as defined below.
*/
@JvmName("mcrtwbeupkpadqva")
public suspend fun routing(`value`: Output) {
this.routing = value
}
/**
* @param value The routing weight associated to the Express Route Connection. Possible value is between `0` and `32000`. Defaults to `0`.
*/
@JvmName("lyuxqkbwxtxlvkto")
public suspend fun routingWeight(`value`: Output) {
this.routingWeight = value
}
/**
* @param value The authorization key to establish the Express Route Connection.
*/
@JvmName("mtfuayqewoyfdevv")
public suspend fun authorizationKey(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.authorizationKey = mapped
}
/**
* @param value Is Internet security enabled for this Express Route Connection?
*/
@JvmName("chfvjkmtcsrboxff")
public suspend fun enableInternetSecurity(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.enableInternetSecurity = mapped
}
/**
* @param value The ID of the Express Route Circuit Peering that this Express Route Connection connects with. Changing this forces a new resource to be created.
*/
@JvmName("irmacjoeumdejcsn")
public suspend fun expressRouteCircuitPeeringId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.expressRouteCircuitPeeringId = mapped
}
/**
* @param value Specified whether Fast Path is enabled for Virtual Wan Firewall Hub. Defaults to `false`.
*/
@JvmName("lbsvsgjftfpokofo")
public suspend fun expressRouteGatewayBypassEnabled(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.expressRouteGatewayBypassEnabled = mapped
}
/**
* @param value The ID of the Express Route Gateway that this Express Route Connection connects with. Changing this forces a new resource to be created.
*/
@JvmName("hsgtirsmjhrfennn")
public suspend fun expressRouteGatewayId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.expressRouteGatewayId = mapped
}
/**
* @param value The name which should be used for this Express Route Connection. Changing this forces a new resource to be created.
*/
@JvmName("pxnnpdlncacdrdyc")
public suspend fun name(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.name = mapped
}
/**
* @param value Bypass the Express Route gateway when accessing private-links. When enabled `express_route_gateway_bypass_enabled` must be set to `true`. Defaults to `false`.
*/
@JvmName("njleiwjdhymehpbk")
public suspend fun privateLinkFastPathEnabled(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.privateLinkFastPathEnabled = mapped
}
/**
* @param value A `routing` block as defined below.
*/
@JvmName("llcivjysqvbslpmk")
public suspend fun routing(`value`: ExpressRouteConnectionRoutingArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.routing = mapped
}
/**
* @param argument A `routing` block as defined below.
*/
@JvmName("fuadaihyjtclldjw")
public suspend
fun routing(argument: suspend ExpressRouteConnectionRoutingArgsBuilder.() -> Unit) {
val toBeMapped = ExpressRouteConnectionRoutingArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.routing = mapped
}
/**
* @param value The routing weight associated to the Express Route Connection. Possible value is between `0` and `32000`. Defaults to `0`.
*/
@JvmName("pyngnmsxowgdvmiw")
public suspend fun routingWeight(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.routingWeight = mapped
}
internal fun build(): ExpressRouteConnectionArgs = ExpressRouteConnectionArgs(
authorizationKey = authorizationKey,
enableInternetSecurity = enableInternetSecurity,
expressRouteCircuitPeeringId = expressRouteCircuitPeeringId,
expressRouteGatewayBypassEnabled = expressRouteGatewayBypassEnabled,
expressRouteGatewayId = expressRouteGatewayId,
name = name,
privateLinkFastPathEnabled = privateLinkFastPathEnabled,
routing = routing,
routingWeight = routingWeight,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy