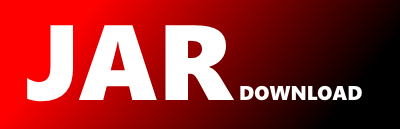
com.pulumi.azure.network.kotlin.NetworkSecurityRule.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-kotlin Show documentation
Show all versions of pulumi-azure-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azure.network.kotlin
import com.pulumi.core.Output
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
/**
* Builder for [NetworkSecurityRule].
*/
@PulumiTagMarker
public class NetworkSecurityRuleResourceBuilder internal constructor() {
public var name: String? = null
public var args: NetworkSecurityRuleArgs = NetworkSecurityRuleArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend NetworkSecurityRuleArgsBuilder.() -> Unit) {
val builder = NetworkSecurityRuleArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): NetworkSecurityRule {
val builtJavaResource = com.pulumi.azure.network.NetworkSecurityRule(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return NetworkSecurityRule(builtJavaResource)
}
}
/**
* Manages a Network Security Rule.
* > **NOTE on Network Security Groups and Network Security Rules:** This provider currently
* provides both a standalone Network Security Rule resource, and allows for Network Security Rules to be defined in-line within the Network Security Group resource.
* At this time you cannot use a Network Security Group with in-line Network Security Rules in conjunction with any Network Security Rule resources. Doing so will cause a conflict of rule settings and will overwrite rules.
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as azure from "@pulumi/azure";
* const example = new azure.core.ResourceGroup("example", {
* name: "example-resources",
* location: "West Europe",
* });
* const exampleNetworkSecurityGroup = new azure.network.NetworkSecurityGroup("example", {
* name: "acceptanceTestSecurityGroup1",
* location: example.location,
* resourceGroupName: example.name,
* });
* const exampleNetworkSecurityRule = new azure.network.NetworkSecurityRule("example", {
* name: "test123",
* priority: 100,
* direction: "Outbound",
* access: "Allow",
* protocol: "Tcp",
* sourcePortRange: "*",
* destinationPortRange: "*",
* sourceAddressPrefix: "*",
* destinationAddressPrefix: "*",
* resourceGroupName: example.name,
* networkSecurityGroupName: exampleNetworkSecurityGroup.name,
* });
* ```
* ```python
* import pulumi
* import pulumi_azure as azure
* example = azure.core.ResourceGroup("example",
* name="example-resources",
* location="West Europe")
* example_network_security_group = azure.network.NetworkSecurityGroup("example",
* name="acceptanceTestSecurityGroup1",
* location=example.location,
* resource_group_name=example.name)
* example_network_security_rule = azure.network.NetworkSecurityRule("example",
* name="test123",
* priority=100,
* direction="Outbound",
* access="Allow",
* protocol="Tcp",
* source_port_range="*",
* destination_port_range="*",
* source_address_prefix="*",
* destination_address_prefix="*",
* resource_group_name=example.name,
* network_security_group_name=example_network_security_group.name)
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Azure = Pulumi.Azure;
* return await Deployment.RunAsync(() =>
* {
* var example = new Azure.Core.ResourceGroup("example", new()
* {
* Name = "example-resources",
* Location = "West Europe",
* });
* var exampleNetworkSecurityGroup = new Azure.Network.NetworkSecurityGroup("example", new()
* {
* Name = "acceptanceTestSecurityGroup1",
* Location = example.Location,
* ResourceGroupName = example.Name,
* });
* var exampleNetworkSecurityRule = new Azure.Network.NetworkSecurityRule("example", new()
* {
* Name = "test123",
* Priority = 100,
* Direction = "Outbound",
* Access = "Allow",
* Protocol = "Tcp",
* SourcePortRange = "*",
* DestinationPortRange = "*",
* SourceAddressPrefix = "*",
* DestinationAddressPrefix = "*",
* ResourceGroupName = example.Name,
* NetworkSecurityGroupName = exampleNetworkSecurityGroup.Name,
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-azure/sdk/v5/go/azure/core"
* "github.com/pulumi/pulumi-azure/sdk/v5/go/azure/network"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* example, err := core.NewResourceGroup(ctx, "example", &core.ResourceGroupArgs{
* Name: pulumi.String("example-resources"),
* Location: pulumi.String("West Europe"),
* })
* if err != nil {
* return err
* }
* exampleNetworkSecurityGroup, err := network.NewNetworkSecurityGroup(ctx, "example", &network.NetworkSecurityGroupArgs{
* Name: pulumi.String("acceptanceTestSecurityGroup1"),
* Location: example.Location,
* ResourceGroupName: example.Name,
* })
* if err != nil {
* return err
* }
* _, err = network.NewNetworkSecurityRule(ctx, "example", &network.NetworkSecurityRuleArgs{
* Name: pulumi.String("test123"),
* Priority: pulumi.Int(100),
* Direction: pulumi.String("Outbound"),
* Access: pulumi.String("Allow"),
* Protocol: pulumi.String("Tcp"),
* SourcePortRange: pulumi.String("*"),
* DestinationPortRange: pulumi.String("*"),
* SourceAddressPrefix: pulumi.String("*"),
* DestinationAddressPrefix: pulumi.String("*"),
* ResourceGroupName: example.Name,
* NetworkSecurityGroupName: exampleNetworkSecurityGroup.Name,
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azure.core.ResourceGroup;
* import com.pulumi.azure.core.ResourceGroupArgs;
* import com.pulumi.azure.network.NetworkSecurityGroup;
* import com.pulumi.azure.network.NetworkSecurityGroupArgs;
* import com.pulumi.azure.network.NetworkSecurityRule;
* import com.pulumi.azure.network.NetworkSecurityRuleArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var example = new ResourceGroup("example", ResourceGroupArgs.builder()
* .name("example-resources")
* .location("West Europe")
* .build());
* var exampleNetworkSecurityGroup = new NetworkSecurityGroup("exampleNetworkSecurityGroup", NetworkSecurityGroupArgs.builder()
* .name("acceptanceTestSecurityGroup1")
* .location(example.location())
* .resourceGroupName(example.name())
* .build());
* var exampleNetworkSecurityRule = new NetworkSecurityRule("exampleNetworkSecurityRule", NetworkSecurityRuleArgs.builder()
* .name("test123")
* .priority(100)
* .direction("Outbound")
* .access("Allow")
* .protocol("Tcp")
* .sourcePortRange("*")
* .destinationPortRange("*")
* .sourceAddressPrefix("*")
* .destinationAddressPrefix("*")
* .resourceGroupName(example.name())
* .networkSecurityGroupName(exampleNetworkSecurityGroup.name())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* example:
* type: azure:core:ResourceGroup
* properties:
* name: example-resources
* location: West Europe
* exampleNetworkSecurityGroup:
* type: azure:network:NetworkSecurityGroup
* name: example
* properties:
* name: acceptanceTestSecurityGroup1
* location: ${example.location}
* resourceGroupName: ${example.name}
* exampleNetworkSecurityRule:
* type: azure:network:NetworkSecurityRule
* name: example
* properties:
* name: test123
* priority: 100
* direction: Outbound
* access: Allow
* protocol: Tcp
* sourcePortRange: '*'
* destinationPortRange: '*'
* sourceAddressPrefix: '*'
* destinationAddressPrefix: '*'
* resourceGroupName: ${example.name}
* networkSecurityGroupName: ${exampleNetworkSecurityGroup.name}
* ```
*
* ## Import
* Network Security Rules can be imported using the `resource id`, e.g.
* ```sh
* $ pulumi import azure:network/networkSecurityRule:NetworkSecurityRule rule1 /subscriptions/00000000-0000-0000-0000-000000000000/resourceGroups/mygroup1/providers/Microsoft.Network/networkSecurityGroups/mySecurityGroup/securityRules/rule1
* ```
*/
public class NetworkSecurityRule internal constructor(
override val javaResource: com.pulumi.azure.network.NetworkSecurityRule,
) : KotlinCustomResource(javaResource, NetworkSecurityRuleMapper) {
/**
* Specifies whether network traffic is allowed or denied. Possible values are `Allow` and `Deny`.
*/
public val access: Output
get() = javaResource.access().applyValue({ args0 -> args0 })
/**
* A description for this rule. Restricted to 140 characters.
*/
public val description: Output?
get() = javaResource.description().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* CIDR or destination IP range or * to match any IP. Tags such as `VirtualNetwork`, `AzureLoadBalancer` and `Internet` can also be used. Besides, it also supports all available Service Tags like ‘Sql.WestEurope‘, ‘Storage.EastUS‘, etc. You can list the available service tags with the CLI: ```shell az network list-service-tags --location westcentralus```. For further information please see [Azure CLI - az network list-service-tags](https://docs.microsoft.com/cli/azure/network?view=azure-cli-latest#az-network-list-service-tags). This is required if `destination_address_prefixes` is not specified.
*/
public val destinationAddressPrefix: Output?
get() = javaResource.destinationAddressPrefix().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* List of destination address prefixes. Tags may not be used. This is required if `destination_address_prefix` is not specified.
*/
public val destinationAddressPrefixes: Output>?
get() = javaResource.destinationAddressPrefixes().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 -> args0 })
}).orElse(null)
})
/**
* A List of destination Application Security Group IDs
*/
public val destinationApplicationSecurityGroupIds: Output?
get() = javaResource.destinationApplicationSecurityGroupIds().applyValue({ args0 ->
args0.map({ args0 -> args0 }).orElse(null)
})
/**
* Destination Port or Range. Integer or range between `0` and `65535` or `*` to match any. This is required if `destination_port_ranges` is not specified.
*/
public val destinationPortRange: Output?
get() = javaResource.destinationPortRange().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* List of destination ports or port ranges. This is required if `destination_port_range` is not specified.
*/
public val destinationPortRanges: Output>?
get() = javaResource.destinationPortRanges().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 -> args0 })
}).orElse(null)
})
/**
* The direction specifies if rule will be evaluated on incoming or outgoing traffic. Possible values are `Inbound` and `Outbound`.
*/
public val direction: Output
get() = javaResource.direction().applyValue({ args0 -> args0 })
/**
* The name of the security rule. This needs to be unique across all Rules in the Network Security Group. Changing this forces a new resource to be created.
*/
public val name: Output
get() = javaResource.name().applyValue({ args0 -> args0 })
/**
* The name of the Network Security Group that we want to attach the rule to. Changing this forces a new resource to be created.
*/
public val networkSecurityGroupName: Output
get() = javaResource.networkSecurityGroupName().applyValue({ args0 -> args0 })
/**
* Specifies the priority of the rule. The value can be between 100 and 4096. The priority number must be unique for each rule in the collection. The lower the priority number, the higher the priority of the rule.
*/
public val priority: Output
get() = javaResource.priority().applyValue({ args0 -> args0 })
/**
* Network protocol this rule applies to. Possible values include `Tcp`, `Udp`, `Icmp`, `Esp`, `Ah` or `*` (which matches all).
*/
public val protocol: Output
get() = javaResource.protocol().applyValue({ args0 -> args0 })
/**
* The name of the resource group in which to create the Network Security Rule. Changing this forces a new resource to be created.
*/
public val resourceGroupName: Output
get() = javaResource.resourceGroupName().applyValue({ args0 -> args0 })
/**
* CIDR or source IP range or * to match any IP. Tags such as `VirtualNetwork`, `AzureLoadBalancer` and `Internet` can also be used. This is required if `source_address_prefixes` is not specified.
*/
public val sourceAddressPrefix: Output?
get() = javaResource.sourceAddressPrefix().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* List of source address prefixes. Tags may not be used. This is required if `source_address_prefix` is not specified.
*/
public val sourceAddressPrefixes: Output>?
get() = javaResource.sourceAddressPrefixes().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 -> args0 })
}).orElse(null)
})
/**
* A List of source Application Security Group IDs
*/
public val sourceApplicationSecurityGroupIds: Output?
get() = javaResource.sourceApplicationSecurityGroupIds().applyValue({ args0 ->
args0.map({ args0 -> args0 }).orElse(null)
})
/**
* Source Port or Range. Integer or range between `0` and `65535` or `*` to match any. This is required if `source_port_ranges` is not specified.
*/
public val sourcePortRange: Output?
get() = javaResource.sourcePortRange().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* List of source ports or port ranges. This is required if `source_port_range` is not specified.
*/
public val sourcePortRanges: Output>?
get() = javaResource.sourcePortRanges().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 -> args0 })
}).orElse(null)
})
}
public object NetworkSecurityRuleMapper : ResourceMapper {
override fun supportsMappingOfType(javaResource: Resource): Boolean =
com.pulumi.azure.network.NetworkSecurityRule::class == javaResource::class
override fun map(javaResource: Resource): NetworkSecurityRule = NetworkSecurityRule(
javaResource
as com.pulumi.azure.network.NetworkSecurityRule,
)
}
/**
* @see [NetworkSecurityRule].
* @param name The _unique_ name of the resulting resource.
* @param block Builder for [NetworkSecurityRule].
*/
public suspend fun networkSecurityRule(
name: String,
block: suspend NetworkSecurityRuleResourceBuilder.() -> Unit,
): NetworkSecurityRule {
val builder = NetworkSecurityRuleResourceBuilder()
builder.name(name)
block(builder)
return builder.build()
}
/**
* @see [NetworkSecurityRule].
* @param name The _unique_ name of the resulting resource.
*/
public fun networkSecurityRule(name: String): NetworkSecurityRule {
val builder = NetworkSecurityRuleResourceBuilder()
builder.name(name)
return builder.build()
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy