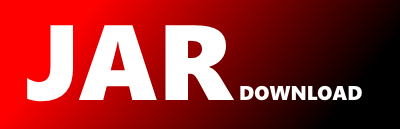
com.pulumi.azure.network.kotlin.VirtualNetworkGatewayArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-kotlin Show documentation
Show all versions of pulumi-azure-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azure.network.kotlin
import com.pulumi.azure.network.VirtualNetworkGatewayArgs.builder
import com.pulumi.azure.network.kotlin.inputs.VirtualNetworkGatewayBgpSettingsArgs
import com.pulumi.azure.network.kotlin.inputs.VirtualNetworkGatewayBgpSettingsArgsBuilder
import com.pulumi.azure.network.kotlin.inputs.VirtualNetworkGatewayCustomRouteArgs
import com.pulumi.azure.network.kotlin.inputs.VirtualNetworkGatewayCustomRouteArgsBuilder
import com.pulumi.azure.network.kotlin.inputs.VirtualNetworkGatewayIpConfigurationArgs
import com.pulumi.azure.network.kotlin.inputs.VirtualNetworkGatewayIpConfigurationArgsBuilder
import com.pulumi.azure.network.kotlin.inputs.VirtualNetworkGatewayPolicyGroupArgs
import com.pulumi.azure.network.kotlin.inputs.VirtualNetworkGatewayPolicyGroupArgsBuilder
import com.pulumi.azure.network.kotlin.inputs.VirtualNetworkGatewayVpnClientConfigurationArgs
import com.pulumi.azure.network.kotlin.inputs.VirtualNetworkGatewayVpnClientConfigurationArgsBuilder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Boolean
import kotlin.Pair
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.collections.Map
import kotlin.jvm.JvmName
/**
* Manages a Virtual Network Gateway to establish secure, cross-premises connectivity.
* > **Note:** Please be aware that provisioning a Virtual Network Gateway takes a long time (between 30 minutes and 1 hour)
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as azure from "@pulumi/azure";
* const example = new azure.core.ResourceGroup("example", {
* name: "test",
* location: "West Europe",
* });
* const exampleVirtualNetwork = new azure.network.VirtualNetwork("example", {
* name: "test",
* location: example.location,
* resourceGroupName: example.name,
* addressSpaces: ["10.0.0.0/16"],
* });
* const exampleSubnet = new azure.network.Subnet("example", {
* name: "GatewaySubnet",
* resourceGroupName: example.name,
* virtualNetworkName: exampleVirtualNetwork.name,
* addressPrefixes: ["10.0.1.0/24"],
* });
* const examplePublicIp = new azure.network.PublicIp("example", {
* name: "test",
* location: example.location,
* resourceGroupName: example.name,
* allocationMethod: "Dynamic",
* });
* const exampleVirtualNetworkGateway = new azure.network.VirtualNetworkGateway("example", {
* name: "test",
* location: example.location,
* resourceGroupName: example.name,
* type: "Vpn",
* vpnType: "RouteBased",
* activeActive: false,
* enableBgp: false,
* sku: "Basic",
* ipConfigurations: [{
* name: "vnetGatewayConfig",
* publicIpAddressId: examplePublicIp.id,
* privateIpAddressAllocation: "Dynamic",
* subnetId: exampleSubnet.id,
* }],
* vpnClientConfiguration: {
* addressSpaces: ["10.2.0.0/24"],
* rootCertificates: [{
* name: "DigiCert-Federated-ID-Root-CA",
* publicCertData: `MIIDuzCCAqOgAwIBAgIQCHTZWCM+IlfFIRXIvyKSrjANBgkqhkiG9w0BAQsFADBn
* MQswCQYDVQQGEwJVUzEVMBMGA1UEChMMRGlnaUNlcnQgSW5jMRkwFwYDVQQLExB3
* d3cuZGlnaWNlcnQuY29tMSYwJAYDVQQDEx1EaWdpQ2VydCBGZWRlcmF0ZWQgSUQg
* Um9vdCBDQTAeFw0xMzAxMTUxMjAwMDBaFw0zMzAxMTUxMjAwMDBaMGcxCzAJBgNV
* BAYTAlVTMRUwEwYDVQQKEwxEaWdpQ2VydCBJbmMxGTAXBgNVBAsTEHd3dy5kaWdp
* Y2VydC5jb20xJjAkBgNVBAMTHURpZ2lDZXJ0IEZlZGVyYXRlZCBJRCBSb290IENB
* MIIBIjANBgkqhkiG9w0BAQEFAAOCAQ8AMIIBCgKCAQEAvAEB4pcCqnNNOWE6Ur5j
* QPUH+1y1F9KdHTRSza6k5iDlXq1kGS1qAkuKtw9JsiNRrjltmFnzMZRBbX8Tlfl8
* zAhBmb6dDduDGED01kBsTkgywYPxXVTKec0WxYEEF0oMn4wSYNl0lt2eJAKHXjNf
* GTwiibdP8CUR2ghSM2sUTI8Nt1Omfc4SMHhGhYD64uJMbX98THQ/4LMGuYegou+d
* GTiahfHtjn7AboSEknwAMJHCh5RlYZZ6B1O4QbKJ+34Q0eKgnI3X6Vc9u0zf6DH8
* Dk+4zQDYRRTqTnVO3VT8jzqDlCRuNtq6YvryOWN74/dq8LQhUnXHvFyrsdMaE1X2
* DwIDAQABo2MwYTAPBgNVHRMBAf8EBTADAQH/MA4GA1UdDwEB/wQEAwIBhjAdBgNV
* HQ4EFgQUGRdkFnbGt1EWjKwbUne+5OaZvRYwHwYDVR0jBBgwFoAUGRdkFnbGt1EW
* jKwbUne+5OaZvRYwDQYJKoZIhvcNAQELBQADggEBAHcqsHkrjpESqfuVTRiptJfP
* 9JbdtWqRTmOf6uJi2c8YVqI6XlKXsD8C1dUUaaHKLUJzvKiazibVuBwMIT84AyqR
* QELn3e0BtgEymEygMU569b01ZPxoFSnNXc7qDZBDef8WfqAV/sxkTi8L9BkmFYfL
* uGLOhRJOFprPdoDIUBB+tmCl3oDcBy3vnUeOEioz8zAkprcb3GHwHAK+vHmmfgcn
* WsfMLH4JCLa/tRYL+Rw/N3ybCkDp00s0WUZ+AoDywSl0Q/ZEnNY0MsFiw6LyIdbq
* M/s/1JRtO3bDSzD9TazRVzn2oBqzSa8VgIo5C1nOnoAKJTlsClJKvIhnRlaLQqk=
* `,
* }],
* revokedCertificates: [{
* name: "Verizon-Global-Root-CA",
* thumbprint: "912198EEF23DCAC40939312FEE97DD560BAE49B1",
* }],
* },
* });
* ```
* ```python
* import pulumi
* import pulumi_azure as azure
* example = azure.core.ResourceGroup("example",
* name="test",
* location="West Europe")
* example_virtual_network = azure.network.VirtualNetwork("example",
* name="test",
* location=example.location,
* resource_group_name=example.name,
* address_spaces=["10.0.0.0/16"])
* example_subnet = azure.network.Subnet("example",
* name="GatewaySubnet",
* resource_group_name=example.name,
* virtual_network_name=example_virtual_network.name,
* address_prefixes=["10.0.1.0/24"])
* example_public_ip = azure.network.PublicIp("example",
* name="test",
* location=example.location,
* resource_group_name=example.name,
* allocation_method="Dynamic")
* example_virtual_network_gateway = azure.network.VirtualNetworkGateway("example",
* name="test",
* location=example.location,
* resource_group_name=example.name,
* type="Vpn",
* vpn_type="RouteBased",
* active_active=False,
* enable_bgp=False,
* sku="Basic",
* ip_configurations=[azure.network.VirtualNetworkGatewayIpConfigurationArgs(
* name="vnetGatewayConfig",
* public_ip_address_id=example_public_ip.id,
* private_ip_address_allocation="Dynamic",
* subnet_id=example_subnet.id,
* )],
* vpn_client_configuration=azure.network.VirtualNetworkGatewayVpnClientConfigurationArgs(
* address_spaces=["10.2.0.0/24"],
* root_certificates=[azure.network.VirtualNetworkGatewayVpnClientConfigurationRootCertificateArgs(
* name="DigiCert-Federated-ID-Root-CA",
* public_cert_data="""MIIDuzCCAqOgAwIBAgIQCHTZWCM+IlfFIRXIvyKSrjANBgkqhkiG9w0BAQsFADBn
* MQswCQYDVQQGEwJVUzEVMBMGA1UEChMMRGlnaUNlcnQgSW5jMRkwFwYDVQQLExB3
* d3cuZGlnaWNlcnQuY29tMSYwJAYDVQQDEx1EaWdpQ2VydCBGZWRlcmF0ZWQgSUQg
* Um9vdCBDQTAeFw0xMzAxMTUxMjAwMDBaFw0zMzAxMTUxMjAwMDBaMGcxCzAJBgNV
* BAYTAlVTMRUwEwYDVQQKEwxEaWdpQ2VydCBJbmMxGTAXBgNVBAsTEHd3dy5kaWdp
* Y2VydC5jb20xJjAkBgNVBAMTHURpZ2lDZXJ0IEZlZGVyYXRlZCBJRCBSb290IENB
* MIIBIjANBgkqhkiG9w0BAQEFAAOCAQ8AMIIBCgKCAQEAvAEB4pcCqnNNOWE6Ur5j
* QPUH+1y1F9KdHTRSza6k5iDlXq1kGS1qAkuKtw9JsiNRrjltmFnzMZRBbX8Tlfl8
* zAhBmb6dDduDGED01kBsTkgywYPxXVTKec0WxYEEF0oMn4wSYNl0lt2eJAKHXjNf
* GTwiibdP8CUR2ghSM2sUTI8Nt1Omfc4SMHhGhYD64uJMbX98THQ/4LMGuYegou+d
* GTiahfHtjn7AboSEknwAMJHCh5RlYZZ6B1O4QbKJ+34Q0eKgnI3X6Vc9u0zf6DH8
* Dk+4zQDYRRTqTnVO3VT8jzqDlCRuNtq6YvryOWN74/dq8LQhUnXHvFyrsdMaE1X2
* DwIDAQABo2MwYTAPBgNVHRMBAf8EBTADAQH/MA4GA1UdDwEB/wQEAwIBhjAdBgNV
* HQ4EFgQUGRdkFnbGt1EWjKwbUne+5OaZvRYwHwYDVR0jBBgwFoAUGRdkFnbGt1EW
* jKwbUne+5OaZvRYwDQYJKoZIhvcNAQELBQADggEBAHcqsHkrjpESqfuVTRiptJfP
* 9JbdtWqRTmOf6uJi2c8YVqI6XlKXsD8C1dUUaaHKLUJzvKiazibVuBwMIT84AyqR
* QELn3e0BtgEymEygMU569b01ZPxoFSnNXc7qDZBDef8WfqAV/sxkTi8L9BkmFYfL
* uGLOhRJOFprPdoDIUBB+tmCl3oDcBy3vnUeOEioz8zAkprcb3GHwHAK+vHmmfgcn
* WsfMLH4JCLa/tRYL+Rw/N3ybCkDp00s0WUZ+AoDywSl0Q/ZEnNY0MsFiw6LyIdbq
* M/s/1JRtO3bDSzD9TazRVzn2oBqzSa8VgIo5C1nOnoAKJTlsClJKvIhnRlaLQqk=
* """,
* )],
* revoked_certificates=[azure.network.VirtualNetworkGatewayVpnClientConfigurationRevokedCertificateArgs(
* name="Verizon-Global-Root-CA",
* thumbprint="912198EEF23DCAC40939312FEE97DD560BAE49B1",
* )],
* ))
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Azure = Pulumi.Azure;
* return await Deployment.RunAsync(() =>
* {
* var example = new Azure.Core.ResourceGroup("example", new()
* {
* Name = "test",
* Location = "West Europe",
* });
* var exampleVirtualNetwork = new Azure.Network.VirtualNetwork("example", new()
* {
* Name = "test",
* Location = example.Location,
* ResourceGroupName = example.Name,
* AddressSpaces = new[]
* {
* "10.0.0.0/16",
* },
* });
* var exampleSubnet = new Azure.Network.Subnet("example", new()
* {
* Name = "GatewaySubnet",
* ResourceGroupName = example.Name,
* VirtualNetworkName = exampleVirtualNetwork.Name,
* AddressPrefixes = new[]
* {
* "10.0.1.0/24",
* },
* });
* var examplePublicIp = new Azure.Network.PublicIp("example", new()
* {
* Name = "test",
* Location = example.Location,
* ResourceGroupName = example.Name,
* AllocationMethod = "Dynamic",
* });
* var exampleVirtualNetworkGateway = new Azure.Network.VirtualNetworkGateway("example", new()
* {
* Name = "test",
* Location = example.Location,
* ResourceGroupName = example.Name,
* Type = "Vpn",
* VpnType = "RouteBased",
* ActiveActive = false,
* EnableBgp = false,
* Sku = "Basic",
* IpConfigurations = new[]
* {
* new Azure.Network.Inputs.VirtualNetworkGatewayIpConfigurationArgs
* {
* Name = "vnetGatewayConfig",
* PublicIpAddressId = examplePublicIp.Id,
* PrivateIpAddressAllocation = "Dynamic",
* SubnetId = exampleSubnet.Id,
* },
* },
* VpnClientConfiguration = new Azure.Network.Inputs.VirtualNetworkGatewayVpnClientConfigurationArgs
* {
* AddressSpaces = new[]
* {
* "10.2.0.0/24",
* },
* RootCertificates = new[]
* {
* new Azure.Network.Inputs.VirtualNetworkGatewayVpnClientConfigurationRootCertificateArgs
* {
* Name = "DigiCert-Federated-ID-Root-CA",
* PublicCertData = @"MIIDuzCCAqOgAwIBAgIQCHTZWCM+IlfFIRXIvyKSrjANBgkqhkiG9w0BAQsFADBn
* MQswCQYDVQQGEwJVUzEVMBMGA1UEChMMRGlnaUNlcnQgSW5jMRkwFwYDVQQLExB3
* d3cuZGlnaWNlcnQuY29tMSYwJAYDVQQDEx1EaWdpQ2VydCBGZWRlcmF0ZWQgSUQg
* Um9vdCBDQTAeFw0xMzAxMTUxMjAwMDBaFw0zMzAxMTUxMjAwMDBaMGcxCzAJBgNV
* BAYTAlVTMRUwEwYDVQQKEwxEaWdpQ2VydCBJbmMxGTAXBgNVBAsTEHd3dy5kaWdp
* Y2VydC5jb20xJjAkBgNVBAMTHURpZ2lDZXJ0IEZlZGVyYXRlZCBJRCBSb290IENB
* MIIBIjANBgkqhkiG9w0BAQEFAAOCAQ8AMIIBCgKCAQEAvAEB4pcCqnNNOWE6Ur5j
* QPUH+1y1F9KdHTRSza6k5iDlXq1kGS1qAkuKtw9JsiNRrjltmFnzMZRBbX8Tlfl8
* zAhBmb6dDduDGED01kBsTkgywYPxXVTKec0WxYEEF0oMn4wSYNl0lt2eJAKHXjNf
* GTwiibdP8CUR2ghSM2sUTI8Nt1Omfc4SMHhGhYD64uJMbX98THQ/4LMGuYegou+d
* GTiahfHtjn7AboSEknwAMJHCh5RlYZZ6B1O4QbKJ+34Q0eKgnI3X6Vc9u0zf6DH8
* Dk+4zQDYRRTqTnVO3VT8jzqDlCRuNtq6YvryOWN74/dq8LQhUnXHvFyrsdMaE1X2
* DwIDAQABo2MwYTAPBgNVHRMBAf8EBTADAQH/MA4GA1UdDwEB/wQEAwIBhjAdBgNV
* HQ4EFgQUGRdkFnbGt1EWjKwbUne+5OaZvRYwHwYDVR0jBBgwFoAUGRdkFnbGt1EW
* jKwbUne+5OaZvRYwDQYJKoZIhvcNAQELBQADggEBAHcqsHkrjpESqfuVTRiptJfP
* 9JbdtWqRTmOf6uJi2c8YVqI6XlKXsD8C1dUUaaHKLUJzvKiazibVuBwMIT84AyqR
* QELn3e0BtgEymEygMU569b01ZPxoFSnNXc7qDZBDef8WfqAV/sxkTi8L9BkmFYfL
* uGLOhRJOFprPdoDIUBB+tmCl3oDcBy3vnUeOEioz8zAkprcb3GHwHAK+vHmmfgcn
* WsfMLH4JCLa/tRYL+Rw/N3ybCkDp00s0WUZ+AoDywSl0Q/ZEnNY0MsFiw6LyIdbq
* M/s/1JRtO3bDSzD9TazRVzn2oBqzSa8VgIo5C1nOnoAKJTlsClJKvIhnRlaLQqk=
* ",
* },
* },
* RevokedCertificates = new[]
* {
* new Azure.Network.Inputs.VirtualNetworkGatewayVpnClientConfigurationRevokedCertificateArgs
* {
* Name = "Verizon-Global-Root-CA",
* Thumbprint = "912198EEF23DCAC40939312FEE97DD560BAE49B1",
* },
* },
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-azure/sdk/v5/go/azure/core"
* "github.com/pulumi/pulumi-azure/sdk/v5/go/azure/network"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* example, err := core.NewResourceGroup(ctx, "example", &core.ResourceGroupArgs{
* Name: pulumi.String("test"),
* Location: pulumi.String("West Europe"),
* })
* if err != nil {
* return err
* }
* exampleVirtualNetwork, err := network.NewVirtualNetwork(ctx, "example", &network.VirtualNetworkArgs{
* Name: pulumi.String("test"),
* Location: example.Location,
* ResourceGroupName: example.Name,
* AddressSpaces: pulumi.StringArray{
* pulumi.String("10.0.0.0/16"),
* },
* })
* if err != nil {
* return err
* }
* exampleSubnet, err := network.NewSubnet(ctx, "example", &network.SubnetArgs{
* Name: pulumi.String("GatewaySubnet"),
* ResourceGroupName: example.Name,
* VirtualNetworkName: exampleVirtualNetwork.Name,
* AddressPrefixes: pulumi.StringArray{
* pulumi.String("10.0.1.0/24"),
* },
* })
* if err != nil {
* return err
* }
* examplePublicIp, err := network.NewPublicIp(ctx, "example", &network.PublicIpArgs{
* Name: pulumi.String("test"),
* Location: example.Location,
* ResourceGroupName: example.Name,
* AllocationMethod: pulumi.String("Dynamic"),
* })
* if err != nil {
* return err
* }
* _, err = network.NewVirtualNetworkGateway(ctx, "example", &network.VirtualNetworkGatewayArgs{
* Name: pulumi.String("test"),
* Location: example.Location,
* ResourceGroupName: example.Name,
* Type: pulumi.String("Vpn"),
* VpnType: pulumi.String("RouteBased"),
* ActiveActive: pulumi.Bool(false),
* EnableBgp: pulumi.Bool(false),
* Sku: pulumi.String("Basic"),
* IpConfigurations: network.VirtualNetworkGatewayIpConfigurationArray{
* &network.VirtualNetworkGatewayIpConfigurationArgs{
* Name: pulumi.String("vnetGatewayConfig"),
* PublicIpAddressId: examplePublicIp.ID(),
* PrivateIpAddressAllocation: pulumi.String("Dynamic"),
* SubnetId: exampleSubnet.ID(),
* },
* },
* VpnClientConfiguration: &network.VirtualNetworkGatewayVpnClientConfigurationArgs{
* AddressSpaces: pulumi.StringArray{
* pulumi.String("10.2.0.0/24"),
* },
* RootCertificates: network.VirtualNetworkGatewayVpnClientConfigurationRootCertificateArray{
* &network.VirtualNetworkGatewayVpnClientConfigurationRootCertificateArgs{
* Name: pulumi.String("DigiCert-Federated-ID-Root-CA"),
* PublicCertData: pulumi.String(`MIIDuzCCAqOgAwIBAgIQCHTZWCM+IlfFIRXIvyKSrjANBgkqhkiG9w0BAQsFADBn
* MQswCQYDVQQGEwJVUzEVMBMGA1UEChMMRGlnaUNlcnQgSW5jMRkwFwYDVQQLExB3
* d3cuZGlnaWNlcnQuY29tMSYwJAYDVQQDEx1EaWdpQ2VydCBGZWRlcmF0ZWQgSUQg
* Um9vdCBDQTAeFw0xMzAxMTUxMjAwMDBaFw0zMzAxMTUxMjAwMDBaMGcxCzAJBgNV
* BAYTAlVTMRUwEwYDVQQKEwxEaWdpQ2VydCBJbmMxGTAXBgNVBAsTEHd3dy5kaWdp
* Y2VydC5jb20xJjAkBgNVBAMTHURpZ2lDZXJ0IEZlZGVyYXRlZCBJRCBSb290IENB
* MIIBIjANBgkqhkiG9w0BAQEFAAOCAQ8AMIIBCgKCAQEAvAEB4pcCqnNNOWE6Ur5j
* QPUH+1y1F9KdHTRSza6k5iDlXq1kGS1qAkuKtw9JsiNRrjltmFnzMZRBbX8Tlfl8
* zAhBmb6dDduDGED01kBsTkgywYPxXVTKec0WxYEEF0oMn4wSYNl0lt2eJAKHXjNf
* GTwiibdP8CUR2ghSM2sUTI8Nt1Omfc4SMHhGhYD64uJMbX98THQ/4LMGuYegou+d
* GTiahfHtjn7AboSEknwAMJHCh5RlYZZ6B1O4QbKJ+34Q0eKgnI3X6Vc9u0zf6DH8
* Dk+4zQDYRRTqTnVO3VT8jzqDlCRuNtq6YvryOWN74/dq8LQhUnXHvFyrsdMaE1X2
* DwIDAQABo2MwYTAPBgNVHRMBAf8EBTADAQH/MA4GA1UdDwEB/wQEAwIBhjAdBgNV
* HQ4EFgQUGRdkFnbGt1EWjKwbUne+5OaZvRYwHwYDVR0jBBgwFoAUGRdkFnbGt1EW
* jKwbUne+5OaZvRYwDQYJKoZIhvcNAQELBQADggEBAHcqsHkrjpESqfuVTRiptJfP
* 9JbdtWqRTmOf6uJi2c8YVqI6XlKXsD8C1dUUaaHKLUJzvKiazibVuBwMIT84AyqR
* QELn3e0BtgEymEygMU569b01ZPxoFSnNXc7qDZBDef8WfqAV/sxkTi8L9BkmFYfL
* uGLOhRJOFprPdoDIUBB+tmCl3oDcBy3vnUeOEioz8zAkprcb3GHwHAK+vHmmfgcn
* WsfMLH4JCLa/tRYL+Rw/N3ybCkDp00s0WUZ+AoDywSl0Q/ZEnNY0MsFiw6LyIdbq
* M/s/1JRtO3bDSzD9TazRVzn2oBqzSa8VgIo5C1nOnoAKJTlsClJKvIhnRlaLQqk=
* `),
* },
* },
* RevokedCertificates: network.VirtualNetworkGatewayVpnClientConfigurationRevokedCertificateArray{
* &network.VirtualNetworkGatewayVpnClientConfigurationRevokedCertificateArgs{
* Name: pulumi.String("Verizon-Global-Root-CA"),
* Thumbprint: pulumi.String("912198EEF23DCAC40939312FEE97DD560BAE49B1"),
* },
* },
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azure.core.ResourceGroup;
* import com.pulumi.azure.core.ResourceGroupArgs;
* import com.pulumi.azure.network.VirtualNetwork;
* import com.pulumi.azure.network.VirtualNetworkArgs;
* import com.pulumi.azure.network.Subnet;
* import com.pulumi.azure.network.SubnetArgs;
* import com.pulumi.azure.network.PublicIp;
* import com.pulumi.azure.network.PublicIpArgs;
* import com.pulumi.azure.network.VirtualNetworkGateway;
* import com.pulumi.azure.network.VirtualNetworkGatewayArgs;
* import com.pulumi.azure.network.inputs.VirtualNetworkGatewayIpConfigurationArgs;
* import com.pulumi.azure.network.inputs.VirtualNetworkGatewayVpnClientConfigurationArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var example = new ResourceGroup("example", ResourceGroupArgs.builder()
* .name("test")
* .location("West Europe")
* .build());
* var exampleVirtualNetwork = new VirtualNetwork("exampleVirtualNetwork", VirtualNetworkArgs.builder()
* .name("test")
* .location(example.location())
* .resourceGroupName(example.name())
* .addressSpaces("10.0.0.0/16")
* .build());
* var exampleSubnet = new Subnet("exampleSubnet", SubnetArgs.builder()
* .name("GatewaySubnet")
* .resourceGroupName(example.name())
* .virtualNetworkName(exampleVirtualNetwork.name())
* .addressPrefixes("10.0.1.0/24")
* .build());
* var examplePublicIp = new PublicIp("examplePublicIp", PublicIpArgs.builder()
* .name("test")
* .location(example.location())
* .resourceGroupName(example.name())
* .allocationMethod("Dynamic")
* .build());
* var exampleVirtualNetworkGateway = new VirtualNetworkGateway("exampleVirtualNetworkGateway", VirtualNetworkGatewayArgs.builder()
* .name("test")
* .location(example.location())
* .resourceGroupName(example.name())
* .type("Vpn")
* .vpnType("RouteBased")
* .activeActive(false)
* .enableBgp(false)
* .sku("Basic")
* .ipConfigurations(VirtualNetworkGatewayIpConfigurationArgs.builder()
* .name("vnetGatewayConfig")
* .publicIpAddressId(examplePublicIp.id())
* .privateIpAddressAllocation("Dynamic")
* .subnetId(exampleSubnet.id())
* .build())
* .vpnClientConfiguration(VirtualNetworkGatewayVpnClientConfigurationArgs.builder()
* .addressSpaces("10.2.0.0/24")
* .rootCertificates(VirtualNetworkGatewayVpnClientConfigurationRootCertificateArgs.builder()
* .name("DigiCert-Federated-ID-Root-CA")
* .publicCertData("""
* MIIDuzCCAqOgAwIBAgIQCHTZWCM+IlfFIRXIvyKSrjANBgkqhkiG9w0BAQsFADBn
* MQswCQYDVQQGEwJVUzEVMBMGA1UEChMMRGlnaUNlcnQgSW5jMRkwFwYDVQQLExB3
* d3cuZGlnaWNlcnQuY29tMSYwJAYDVQQDEx1EaWdpQ2VydCBGZWRlcmF0ZWQgSUQg
* Um9vdCBDQTAeFw0xMzAxMTUxMjAwMDBaFw0zMzAxMTUxMjAwMDBaMGcxCzAJBgNV
* BAYTAlVTMRUwEwYDVQQKEwxEaWdpQ2VydCBJbmMxGTAXBgNVBAsTEHd3dy5kaWdp
* Y2VydC5jb20xJjAkBgNVBAMTHURpZ2lDZXJ0IEZlZGVyYXRlZCBJRCBSb290IENB
* MIIBIjANBgkqhkiG9w0BAQEFAAOCAQ8AMIIBCgKCAQEAvAEB4pcCqnNNOWE6Ur5j
* QPUH+1y1F9KdHTRSza6k5iDlXq1kGS1qAkuKtw9JsiNRrjltmFnzMZRBbX8Tlfl8
* zAhBmb6dDduDGED01kBsTkgywYPxXVTKec0WxYEEF0oMn4wSYNl0lt2eJAKHXjNf
* GTwiibdP8CUR2ghSM2sUTI8Nt1Omfc4SMHhGhYD64uJMbX98THQ/4LMGuYegou+d
* GTiahfHtjn7AboSEknwAMJHCh5RlYZZ6B1O4QbKJ+34Q0eKgnI3X6Vc9u0zf6DH8
* Dk+4zQDYRRTqTnVO3VT8jzqDlCRuNtq6YvryOWN74/dq8LQhUnXHvFyrsdMaE1X2
* DwIDAQABo2MwYTAPBgNVHRMBAf8EBTADAQH/MA4GA1UdDwEB/wQEAwIBhjAdBgNV
* HQ4EFgQUGRdkFnbGt1EWjKwbUne+5OaZvRYwHwYDVR0jBBgwFoAUGRdkFnbGt1EW
* jKwbUne+5OaZvRYwDQYJKoZIhvcNAQELBQADggEBAHcqsHkrjpESqfuVTRiptJfP
* 9JbdtWqRTmOf6uJi2c8YVqI6XlKXsD8C1dUUaaHKLUJzvKiazibVuBwMIT84AyqR
* QELn3e0BtgEymEygMU569b01ZPxoFSnNXc7qDZBDef8WfqAV/sxkTi8L9BkmFYfL
* uGLOhRJOFprPdoDIUBB+tmCl3oDcBy3vnUeOEioz8zAkprcb3GHwHAK+vHmmfgcn
* WsfMLH4JCLa/tRYL+Rw/N3ybCkDp00s0WUZ+AoDywSl0Q/ZEnNY0MsFiw6LyIdbq
* M/s/1JRtO3bDSzD9TazRVzn2oBqzSa8VgIo5C1nOnoAKJTlsClJKvIhnRlaLQqk=
* """)
* .build())
* .revokedCertificates(VirtualNetworkGatewayVpnClientConfigurationRevokedCertificateArgs.builder()
* .name("Verizon-Global-Root-CA")
* .thumbprint("912198EEF23DCAC40939312FEE97DD560BAE49B1")
* .build())
* .build())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* example:
* type: azure:core:ResourceGroup
* properties:
* name: test
* location: West Europe
* exampleVirtualNetwork:
* type: azure:network:VirtualNetwork
* name: example
* properties:
* name: test
* location: ${example.location}
* resourceGroupName: ${example.name}
* addressSpaces:
* - 10.0.0.0/16
* exampleSubnet:
* type: azure:network:Subnet
* name: example
* properties:
* name: GatewaySubnet
* resourceGroupName: ${example.name}
* virtualNetworkName: ${exampleVirtualNetwork.name}
* addressPrefixes:
* - 10.0.1.0/24
* examplePublicIp:
* type: azure:network:PublicIp
* name: example
* properties:
* name: test
* location: ${example.location}
* resourceGroupName: ${example.name}
* allocationMethod: Dynamic
* exampleVirtualNetworkGateway:
* type: azure:network:VirtualNetworkGateway
* name: example
* properties:
* name: test
* location: ${example.location}
* resourceGroupName: ${example.name}
* type: Vpn
* vpnType: RouteBased
* activeActive: false
* enableBgp: false
* sku: Basic
* ipConfigurations:
* - name: vnetGatewayConfig
* publicIpAddressId: ${examplePublicIp.id}
* privateIpAddressAllocation: Dynamic
* subnetId: ${exampleSubnet.id}
* vpnClientConfiguration:
* addressSpaces:
* - 10.2.0.0/24
* rootCertificates:
* - name: DigiCert-Federated-ID-Root-CA
* publicCertData: |
* MIIDuzCCAqOgAwIBAgIQCHTZWCM+IlfFIRXIvyKSrjANBgkqhkiG9w0BAQsFADBn
* MQswCQYDVQQGEwJVUzEVMBMGA1UEChMMRGlnaUNlcnQgSW5jMRkwFwYDVQQLExB3
* d3cuZGlnaWNlcnQuY29tMSYwJAYDVQQDEx1EaWdpQ2VydCBGZWRlcmF0ZWQgSUQg
* Um9vdCBDQTAeFw0xMzAxMTUxMjAwMDBaFw0zMzAxMTUxMjAwMDBaMGcxCzAJBgNV
* BAYTAlVTMRUwEwYDVQQKEwxEaWdpQ2VydCBJbmMxGTAXBgNVBAsTEHd3dy5kaWdp
* Y2VydC5jb20xJjAkBgNVBAMTHURpZ2lDZXJ0IEZlZGVyYXRlZCBJRCBSb290IENB
* MIIBIjANBgkqhkiG9w0BAQEFAAOCAQ8AMIIBCgKCAQEAvAEB4pcCqnNNOWE6Ur5j
* QPUH+1y1F9KdHTRSza6k5iDlXq1kGS1qAkuKtw9JsiNRrjltmFnzMZRBbX8Tlfl8
* zAhBmb6dDduDGED01kBsTkgywYPxXVTKec0WxYEEF0oMn4wSYNl0lt2eJAKHXjNf
* GTwiibdP8CUR2ghSM2sUTI8Nt1Omfc4SMHhGhYD64uJMbX98THQ/4LMGuYegou+d
* GTiahfHtjn7AboSEknwAMJHCh5RlYZZ6B1O4QbKJ+34Q0eKgnI3X6Vc9u0zf6DH8
* Dk+4zQDYRRTqTnVO3VT8jzqDlCRuNtq6YvryOWN74/dq8LQhUnXHvFyrsdMaE1X2
* DwIDAQABo2MwYTAPBgNVHRMBAf8EBTADAQH/MA4GA1UdDwEB/wQEAwIBhjAdBgNV
* HQ4EFgQUGRdkFnbGt1EWjKwbUne+5OaZvRYwHwYDVR0jBBgwFoAUGRdkFnbGt1EW
* jKwbUne+5OaZvRYwDQYJKoZIhvcNAQELBQADggEBAHcqsHkrjpESqfuVTRiptJfP
* 9JbdtWqRTmOf6uJi2c8YVqI6XlKXsD8C1dUUaaHKLUJzvKiazibVuBwMIT84AyqR
* QELn3e0BtgEymEygMU569b01ZPxoFSnNXc7qDZBDef8WfqAV/sxkTi8L9BkmFYfL
* uGLOhRJOFprPdoDIUBB+tmCl3oDcBy3vnUeOEioz8zAkprcb3GHwHAK+vHmmfgcn
* WsfMLH4JCLa/tRYL+Rw/N3ybCkDp00s0WUZ+AoDywSl0Q/ZEnNY0MsFiw6LyIdbq
* M/s/1JRtO3bDSzD9TazRVzn2oBqzSa8VgIo5C1nOnoAKJTlsClJKvIhnRlaLQqk=
* revokedCertificates:
* - name: Verizon-Global-Root-CA
* thumbprint: 912198EEF23DCAC40939312FEE97DD560BAE49B1
* ```
*
* ## Import
* Virtual Network Gateways can be imported using the `resource id`, e.g.
* ```sh
* $ pulumi import azure:network/virtualNetworkGateway:VirtualNetworkGateway exampleGateway /subscriptions/00000000-0000-0000-0000-000000000000/resourceGroups/myGroup1/providers/Microsoft.Network/virtualNetworkGateways/myGateway1
* ```
* @property activeActive If `true`, an active-active Virtual Network Gateway will be created. An active-active gateway requires a `HighPerformance` or an `UltraPerformance` SKU. If `false`, an active-standby gateway will be created. Defaults to `false`.
* @property bgpRouteTranslationForNatEnabled Is BGP Route Translation for NAT enabled? Defaults to `false`.
* @property bgpSettings A `bgp_settings` block which is documented below. In this block the BGP specific settings can be defined.
* @property customRoute A `custom_route` block as defined below. Specifies a custom routes address space for a virtual network gateway and a VpnClient.
* @property defaultLocalNetworkGatewayId The ID of the local network gateway through which outbound Internet traffic from the virtual network in which the gateway is created will be routed (*forced tunnelling*). Refer to the [Azure documentation on forced tunnelling](https://docs.microsoft.com/azure/vpn-gateway/vpn-gateway-forced-tunneling-rm). If not specified, forced tunnelling is disabled.
* @property dnsForwardingEnabled Is DNS forwarding enabled?
* @property edgeZone Specifies the Edge Zone within the Azure Region where this Virtual Network Gateway should exist. Changing this forces a new Virtual Network Gateway to be created.
* @property enableBgp If `true`, BGP (Border Gateway Protocol) will be enabled for this Virtual Network Gateway. Defaults to `false`.
* @property generation The Generation of the Virtual Network gateway. Possible values include `Generation1`, `Generation2` or `None`. Changing this forces a new resource to be created.
* > **NOTE:** The available values depend on the `type` and `sku` arguments - where `Generation2` is only value for a `sku` larger than `VpnGw2` or `VpnGw2AZ`.
* @property ipConfigurations One or more (up to 3) `ip_configuration` blocks documented below.
* An active-standby gateway requires exactly one `ip_configuration` block,
* an active-active gateway requires exactly two `ip_configuration` blocks whereas
* an active-active zone redundant gateway with P2S configuration requires exactly three `ip_configuration` blocks.
* @property ipSecReplayProtectionEnabled Is IP Sec Replay Protection enabled? Defaults to `true`.
* @property location The location/region where the Virtual Network Gateway is located. Changing this forces a new resource to be created.
* @property name The name of the Virtual Network Gateway. Changing this forces a new resource to be created.
* @property policyGroups One or more `policy_group` blocks as defined below.
* @property privateIpAddressEnabled Should private IP be enabled on this gateway for connections? Changing this forces a new resource to be created.
* @property remoteVnetTrafficEnabled Is remote vnet traffic that is used to configure this gateway to accept traffic from other Azure Virtual Networks enabled? Defaults to `false`.
* @property resourceGroupName The name of the resource group in which to create the Virtual Network Gateway. Changing this forces a new resource to be created.
* @property sku Configuration of the size and capacity of the virtual network gateway. Valid options are `Basic`, `Standard`, `HighPerformance`, `UltraPerformance`, `ErGw1AZ`, `ErGw2AZ`, `ErGw3AZ`, `VpnGw1`, `VpnGw2`, `VpnGw3`, `VpnGw4`,`VpnGw5`, `VpnGw1AZ`, `VpnGw2AZ`, `VpnGw3AZ`,`VpnGw4AZ` and `VpnGw5AZ` and depend on the `type`, `vpn_type` and `generation` arguments. A `PolicyBased` gateway only supports the `Basic` SKU. Further, the `UltraPerformance` SKU is only supported by an `ExpressRoute` gateway.
* > **NOTE:** To build a UltraPerformance ExpressRoute Virtual Network gateway, the associated Public IP needs to be SKU "Basic" not "Standard"
* > **NOTE:** Not all SKUs (e.g. `ErGw1AZ`) are available in all regions. If you see `StatusCode=400 -- Original Error: Code="InvalidGatewaySkuSpecifiedForGatewayDeploymentType"` please try another region.
* @property tags A mapping of tags to assign to the resource.
* @property type The type of the Virtual Network Gateway. Valid options are `Vpn` or `ExpressRoute`. Changing the type forces a new resource to be created.
* @property virtualWanTrafficEnabled Is remote vnet traffic that is used to configure this gateway to accept traffic from remote Virtual WAN networks enabled? Defaults to `false`.
* @property vpnClientConfiguration A `vpn_client_configuration` block which is documented below. In this block the Virtual Network Gateway can be configured to accept IPSec point-to-site connections.
* @property vpnType The routing type of the Virtual Network Gateway. Valid options are `RouteBased` or `PolicyBased`. Defaults to `RouteBased`. Changing this forces a new resource to be created.
*/
public data class VirtualNetworkGatewayArgs(
public val activeActive: Output? = null,
public val bgpRouteTranslationForNatEnabled: Output? = null,
public val bgpSettings: Output? = null,
public val customRoute: Output? = null,
public val defaultLocalNetworkGatewayId: Output? = null,
public val dnsForwardingEnabled: Output? = null,
public val edgeZone: Output? = null,
public val enableBgp: Output? = null,
public val generation: Output? = null,
public val ipConfigurations: Output>? = null,
public val ipSecReplayProtectionEnabled: Output? = null,
public val location: Output? = null,
public val name: Output? = null,
public val policyGroups: Output>? = null,
public val privateIpAddressEnabled: Output? = null,
public val remoteVnetTrafficEnabled: Output? = null,
public val resourceGroupName: Output? = null,
public val sku: Output? = null,
public val tags: Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy