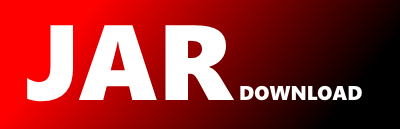
com.pulumi.azure.network.kotlin.inputs.ApplicationGatewayUrlPathMapArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-kotlin Show documentation
Show all versions of pulumi-azure-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azure.network.kotlin.inputs
import com.pulumi.azure.network.inputs.ApplicationGatewayUrlPathMapArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
*
* @property defaultBackendAddressPoolId The ID of the Default Backend Address Pool.
* @property defaultBackendAddressPoolName The Name of the Default Backend Address Pool which should be used for this URL Path Map. Cannot be set if `default_redirect_configuration_name` is set.
* @property defaultBackendHttpSettingsId The ID of the Default Backend HTTP Settings Collection.
* @property defaultBackendHttpSettingsName The Name of the Default Backend HTTP Settings Collection which should be used for this URL Path Map. Cannot be set if `default_redirect_configuration_name` is set.
* @property defaultRedirectConfigurationId The ID of the Default Redirect Configuration.
* @property defaultRedirectConfigurationName The Name of the Default Redirect Configuration which should be used for this URL Path Map. Cannot be set if either `default_backend_address_pool_name` or `default_backend_http_settings_name` is set.
* > **NOTE:** Both `default_backend_address_pool_name` and `default_backend_http_settings_name` or `default_redirect_configuration_name` should be specified.
* @property defaultRewriteRuleSetId
* @property defaultRewriteRuleSetName The Name of the Default Rewrite Rule Set which should be used for this URL Path Map. Only valid for v2 SKUs.
* @property id The ID of the Rewrite Rule Set
* @property name The Name of the URL Path Map.
* @property pathRules One or more `path_rule` blocks as defined above.
*/
public data class ApplicationGatewayUrlPathMapArgs(
public val defaultBackendAddressPoolId: Output? = null,
public val defaultBackendAddressPoolName: Output? = null,
public val defaultBackendHttpSettingsId: Output? = null,
public val defaultBackendHttpSettingsName: Output? = null,
public val defaultRedirectConfigurationId: Output? = null,
public val defaultRedirectConfigurationName: Output? = null,
public val defaultRewriteRuleSetId: Output? = null,
public val defaultRewriteRuleSetName: Output? = null,
public val id: Output? = null,
public val name: Output,
public val pathRules: Output>,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azure.network.inputs.ApplicationGatewayUrlPathMapArgs =
com.pulumi.azure.network.inputs.ApplicationGatewayUrlPathMapArgs.builder()
.defaultBackendAddressPoolId(defaultBackendAddressPoolId?.applyValue({ args0 -> args0 }))
.defaultBackendAddressPoolName(defaultBackendAddressPoolName?.applyValue({ args0 -> args0 }))
.defaultBackendHttpSettingsId(defaultBackendHttpSettingsId?.applyValue({ args0 -> args0 }))
.defaultBackendHttpSettingsName(defaultBackendHttpSettingsName?.applyValue({ args0 -> args0 }))
.defaultRedirectConfigurationId(defaultRedirectConfigurationId?.applyValue({ args0 -> args0 }))
.defaultRedirectConfigurationName(defaultRedirectConfigurationName?.applyValue({ args0 -> args0 }))
.defaultRewriteRuleSetId(defaultRewriteRuleSetId?.applyValue({ args0 -> args0 }))
.defaultRewriteRuleSetName(defaultRewriteRuleSetName?.applyValue({ args0 -> args0 }))
.id(id?.applyValue({ args0 -> args0 }))
.name(name.applyValue({ args0 -> args0 }))
.pathRules(
pathRules.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
).build()
}
/**
* Builder for [ApplicationGatewayUrlPathMapArgs].
*/
@PulumiTagMarker
public class ApplicationGatewayUrlPathMapArgsBuilder internal constructor() {
private var defaultBackendAddressPoolId: Output? = null
private var defaultBackendAddressPoolName: Output? = null
private var defaultBackendHttpSettingsId: Output? = null
private var defaultBackendHttpSettingsName: Output? = null
private var defaultRedirectConfigurationId: Output? = null
private var defaultRedirectConfigurationName: Output? = null
private var defaultRewriteRuleSetId: Output? = null
private var defaultRewriteRuleSetName: Output? = null
private var id: Output? = null
private var name: Output? = null
private var pathRules: Output>? = null
/**
* @param value The ID of the Default Backend Address Pool.
*/
@JvmName("krglynydbhkekbea")
public suspend fun defaultBackendAddressPoolId(`value`: Output) {
this.defaultBackendAddressPoolId = value
}
/**
* @param value The Name of the Default Backend Address Pool which should be used for this URL Path Map. Cannot be set if `default_redirect_configuration_name` is set.
*/
@JvmName("nipihgipwsghlnuo")
public suspend fun defaultBackendAddressPoolName(`value`: Output) {
this.defaultBackendAddressPoolName = value
}
/**
* @param value The ID of the Default Backend HTTP Settings Collection.
*/
@JvmName("kaokrnakqvymcasa")
public suspend fun defaultBackendHttpSettingsId(`value`: Output) {
this.defaultBackendHttpSettingsId = value
}
/**
* @param value The Name of the Default Backend HTTP Settings Collection which should be used for this URL Path Map. Cannot be set if `default_redirect_configuration_name` is set.
*/
@JvmName("khflwxikgmbucgtq")
public suspend fun defaultBackendHttpSettingsName(`value`: Output) {
this.defaultBackendHttpSettingsName = value
}
/**
* @param value The ID of the Default Redirect Configuration.
*/
@JvmName("gkknujcvslboacda")
public suspend fun defaultRedirectConfigurationId(`value`: Output) {
this.defaultRedirectConfigurationId = value
}
/**
* @param value The Name of the Default Redirect Configuration which should be used for this URL Path Map. Cannot be set if either `default_backend_address_pool_name` or `default_backend_http_settings_name` is set.
* > **NOTE:** Both `default_backend_address_pool_name` and `default_backend_http_settings_name` or `default_redirect_configuration_name` should be specified.
*/
@JvmName("nohsxfpbxbqwoyth")
public suspend fun defaultRedirectConfigurationName(`value`: Output) {
this.defaultRedirectConfigurationName = value
}
/**
* @param value
*/
@JvmName("htifinuwxudkygmy")
public suspend fun defaultRewriteRuleSetId(`value`: Output) {
this.defaultRewriteRuleSetId = value
}
/**
* @param value The Name of the Default Rewrite Rule Set which should be used for this URL Path Map. Only valid for v2 SKUs.
*/
@JvmName("pdenyddaalpsauvm")
public suspend fun defaultRewriteRuleSetName(`value`: Output) {
this.defaultRewriteRuleSetName = value
}
/**
* @param value The ID of the Rewrite Rule Set
*/
@JvmName("jpdnoipwaxiitsaw")
public suspend fun id(`value`: Output) {
this.id = value
}
/**
* @param value The Name of the URL Path Map.
*/
@JvmName("noiwaieyxkwoyxln")
public suspend fun name(`value`: Output) {
this.name = value
}
/**
* @param value One or more `path_rule` blocks as defined above.
*/
@JvmName("grtvxydnbcuuscjn")
public suspend fun pathRules(`value`: Output>) {
this.pathRules = value
}
@JvmName("vkmqjmpegbsmgdws")
public suspend fun pathRules(vararg values: Output) {
this.pathRules = Output.all(values.asList())
}
/**
* @param values One or more `path_rule` blocks as defined above.
*/
@JvmName("xbqkgdtinyyytocs")
public suspend fun pathRules(values: List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy