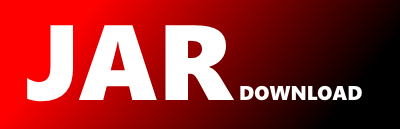
com.pulumi.azure.network.kotlin.inputs.VpnGatewayConnectionVpnLinkIpsecPolicyArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-kotlin Show documentation
Show all versions of pulumi-azure-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azure.network.kotlin.inputs
import com.pulumi.azure.network.inputs.VpnGatewayConnectionVpnLinkIpsecPolicyArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
*
* @property dhGroup The DH Group used in IKE Phase 1 for initial SA. Possible values are `None`, `DHGroup1`, `DHGroup2`, `DHGroup14`, `DHGroup24`, `DHGroup2048`, `ECP256`, `ECP384`.
* @property encryptionAlgorithm The IPSec encryption algorithm (IKE phase 1). Possible values are `AES128`, `AES192`, `AES256`, `DES`, `DES3`, `GCMAES128`, `GCMAES192`, `GCMAES256`, `None`.
* @property ikeEncryptionAlgorithm The IKE encryption algorithm (IKE phase 2). Possible values are `DES`, `DES3`, `AES128`, `AES192`, `AES256`, `GCMAES128`, `GCMAES256`.
* @property ikeIntegrityAlgorithm The IKE integrity algorithm (IKE phase 2). Possible values are `MD5`, `SHA1`, `SHA256`, `SHA384`, `GCMAES128`, `GCMAES256`.
* @property integrityAlgorithm The IPSec integrity algorithm (IKE phase 1). Possible values are `MD5`, `SHA1`, `SHA256`, `GCMAES128`, `GCMAES192`, `GCMAES256`.
* @property pfsGroup The Pfs Group used in IKE Phase 2 for the new child SA. Possible values are `None`, `PFS1`, `PFS2`, `PFS14`, `PFS24`, `PFS2048`, `PFSMM`, `ECP256`, `ECP384`.
* @property saDataSizeKb The IPSec Security Association (also called Quick Mode or Phase 2 SA) payload size in KB for the site to site VPN tunnel.
* @property saLifetimeSec The IPSec Security Association (also called Quick Mode or Phase 2 SA) lifetime in seconds for the site to site VPN tunnel.
*/
public data class VpnGatewayConnectionVpnLinkIpsecPolicyArgs(
public val dhGroup: Output,
public val encryptionAlgorithm: Output,
public val ikeEncryptionAlgorithm: Output,
public val ikeIntegrityAlgorithm: Output,
public val integrityAlgorithm: Output,
public val pfsGroup: Output,
public val saDataSizeKb: Output,
public val saLifetimeSec: Output,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azure.network.inputs.VpnGatewayConnectionVpnLinkIpsecPolicyArgs =
com.pulumi.azure.network.inputs.VpnGatewayConnectionVpnLinkIpsecPolicyArgs.builder()
.dhGroup(dhGroup.applyValue({ args0 -> args0 }))
.encryptionAlgorithm(encryptionAlgorithm.applyValue({ args0 -> args0 }))
.ikeEncryptionAlgorithm(ikeEncryptionAlgorithm.applyValue({ args0 -> args0 }))
.ikeIntegrityAlgorithm(ikeIntegrityAlgorithm.applyValue({ args0 -> args0 }))
.integrityAlgorithm(integrityAlgorithm.applyValue({ args0 -> args0 }))
.pfsGroup(pfsGroup.applyValue({ args0 -> args0 }))
.saDataSizeKb(saDataSizeKb.applyValue({ args0 -> args0 }))
.saLifetimeSec(saLifetimeSec.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [VpnGatewayConnectionVpnLinkIpsecPolicyArgs].
*/
@PulumiTagMarker
public class VpnGatewayConnectionVpnLinkIpsecPolicyArgsBuilder internal constructor() {
private var dhGroup: Output? = null
private var encryptionAlgorithm: Output? = null
private var ikeEncryptionAlgorithm: Output? = null
private var ikeIntegrityAlgorithm: Output? = null
private var integrityAlgorithm: Output? = null
private var pfsGroup: Output? = null
private var saDataSizeKb: Output? = null
private var saLifetimeSec: Output? = null
/**
* @param value The DH Group used in IKE Phase 1 for initial SA. Possible values are `None`, `DHGroup1`, `DHGroup2`, `DHGroup14`, `DHGroup24`, `DHGroup2048`, `ECP256`, `ECP384`.
*/
@JvmName("dxjdgohrgwkvaosb")
public suspend fun dhGroup(`value`: Output) {
this.dhGroup = value
}
/**
* @param value The IPSec encryption algorithm (IKE phase 1). Possible values are `AES128`, `AES192`, `AES256`, `DES`, `DES3`, `GCMAES128`, `GCMAES192`, `GCMAES256`, `None`.
*/
@JvmName("midivejapjukfhtf")
public suspend fun encryptionAlgorithm(`value`: Output) {
this.encryptionAlgorithm = value
}
/**
* @param value The IKE encryption algorithm (IKE phase 2). Possible values are `DES`, `DES3`, `AES128`, `AES192`, `AES256`, `GCMAES128`, `GCMAES256`.
*/
@JvmName("ixnckakcgsnypsdb")
public suspend fun ikeEncryptionAlgorithm(`value`: Output) {
this.ikeEncryptionAlgorithm = value
}
/**
* @param value The IKE integrity algorithm (IKE phase 2). Possible values are `MD5`, `SHA1`, `SHA256`, `SHA384`, `GCMAES128`, `GCMAES256`.
*/
@JvmName("yffmbeqqklerfoib")
public suspend fun ikeIntegrityAlgorithm(`value`: Output) {
this.ikeIntegrityAlgorithm = value
}
/**
* @param value The IPSec integrity algorithm (IKE phase 1). Possible values are `MD5`, `SHA1`, `SHA256`, `GCMAES128`, `GCMAES192`, `GCMAES256`.
*/
@JvmName("bdknaakueildxhfo")
public suspend fun integrityAlgorithm(`value`: Output) {
this.integrityAlgorithm = value
}
/**
* @param value The Pfs Group used in IKE Phase 2 for the new child SA. Possible values are `None`, `PFS1`, `PFS2`, `PFS14`, `PFS24`, `PFS2048`, `PFSMM`, `ECP256`, `ECP384`.
*/
@JvmName("jctymvxfaafpfulj")
public suspend fun pfsGroup(`value`: Output) {
this.pfsGroup = value
}
/**
* @param value The IPSec Security Association (also called Quick Mode or Phase 2 SA) payload size in KB for the site to site VPN tunnel.
*/
@JvmName("phuwpvvulfssnrwq")
public suspend fun saDataSizeKb(`value`: Output) {
this.saDataSizeKb = value
}
/**
* @param value The IPSec Security Association (also called Quick Mode or Phase 2 SA) lifetime in seconds for the site to site VPN tunnel.
*/
@JvmName("mvxucivaspxrujat")
public suspend fun saLifetimeSec(`value`: Output) {
this.saLifetimeSec = value
}
/**
* @param value The DH Group used in IKE Phase 1 for initial SA. Possible values are `None`, `DHGroup1`, `DHGroup2`, `DHGroup14`, `DHGroup24`, `DHGroup2048`, `ECP256`, `ECP384`.
*/
@JvmName("pxnjgldmyaqurgkh")
public suspend fun dhGroup(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.dhGroup = mapped
}
/**
* @param value The IPSec encryption algorithm (IKE phase 1). Possible values are `AES128`, `AES192`, `AES256`, `DES`, `DES3`, `GCMAES128`, `GCMAES192`, `GCMAES256`, `None`.
*/
@JvmName("sufkuwfwajooqrgh")
public suspend fun encryptionAlgorithm(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.encryptionAlgorithm = mapped
}
/**
* @param value The IKE encryption algorithm (IKE phase 2). Possible values are `DES`, `DES3`, `AES128`, `AES192`, `AES256`, `GCMAES128`, `GCMAES256`.
*/
@JvmName("jfngybcavjrvevlc")
public suspend fun ikeEncryptionAlgorithm(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.ikeEncryptionAlgorithm = mapped
}
/**
* @param value The IKE integrity algorithm (IKE phase 2). Possible values are `MD5`, `SHA1`, `SHA256`, `SHA384`, `GCMAES128`, `GCMAES256`.
*/
@JvmName("cpqhepewgwvaxlct")
public suspend fun ikeIntegrityAlgorithm(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.ikeIntegrityAlgorithm = mapped
}
/**
* @param value The IPSec integrity algorithm (IKE phase 1). Possible values are `MD5`, `SHA1`, `SHA256`, `GCMAES128`, `GCMAES192`, `GCMAES256`.
*/
@JvmName("ntbixddvallnsqrb")
public suspend fun integrityAlgorithm(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.integrityAlgorithm = mapped
}
/**
* @param value The Pfs Group used in IKE Phase 2 for the new child SA. Possible values are `None`, `PFS1`, `PFS2`, `PFS14`, `PFS24`, `PFS2048`, `PFSMM`, `ECP256`, `ECP384`.
*/
@JvmName("dtcidjyfmwcgqhvo")
public suspend fun pfsGroup(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.pfsGroup = mapped
}
/**
* @param value The IPSec Security Association (also called Quick Mode or Phase 2 SA) payload size in KB for the site to site VPN tunnel.
*/
@JvmName("ijncbcywlqnjyuaw")
public suspend fun saDataSizeKb(`value`: Int) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.saDataSizeKb = mapped
}
/**
* @param value The IPSec Security Association (also called Quick Mode or Phase 2 SA) lifetime in seconds for the site to site VPN tunnel.
*/
@JvmName("eobnjysdwpitsbhm")
public suspend fun saLifetimeSec(`value`: Int) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.saLifetimeSec = mapped
}
internal fun build(): VpnGatewayConnectionVpnLinkIpsecPolicyArgs =
VpnGatewayConnectionVpnLinkIpsecPolicyArgs(
dhGroup = dhGroup ?: throw PulumiNullFieldException("dhGroup"),
encryptionAlgorithm = encryptionAlgorithm ?: throw PulumiNullFieldException("encryptionAlgorithm"),
ikeEncryptionAlgorithm = ikeEncryptionAlgorithm ?: throw
PulumiNullFieldException("ikeEncryptionAlgorithm"),
ikeIntegrityAlgorithm = ikeIntegrityAlgorithm ?: throw
PulumiNullFieldException("ikeIntegrityAlgorithm"),
integrityAlgorithm = integrityAlgorithm ?: throw PulumiNullFieldException("integrityAlgorithm"),
pfsGroup = pfsGroup ?: throw PulumiNullFieldException("pfsGroup"),
saDataSizeKb = saDataSizeKb ?: throw PulumiNullFieldException("saDataSizeKb"),
saLifetimeSec = saLifetimeSec ?: throw PulumiNullFieldException("saLifetimeSec"),
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy