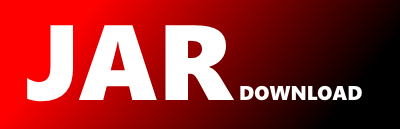
com.pulumi.azure.network.kotlin.outputs.GetApplicationGatewayResult.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-kotlin Show documentation
Show all versions of pulumi-azure-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azure.network.kotlin.outputs
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
import kotlin.collections.Map
/**
* A collection of values returned by getApplicationGateway.
* @property authenticationCertificates One or more `authentication_certificate` blocks as defined below.
* @property autoscaleConfigurations An `autoscale_configuration` block as defined below.
* @property backendAddressPools One or more `backend_address_pool` blocks as defined below.
* @property backendHttpSettings One or more `backend_http_settings` blocks as defined below.
* @property customErrorConfigurations One or more `custom_error_configuration` blocks as defined below.
* @property fipsEnabled Is FIPS enabled on the Application Gateway?
* @property firewallPolicyId The ID of the Web Application Firewall Policy which is used as an HTTP Listener for this Path Rule.
* @property forceFirewallPolicyAssociation Is the Firewall Policy associated with the Application Gateway?
* @property frontendIpConfigurations One or more `frontend_ip_configuration` blocks as defined below.
* @property frontendPorts One or more `frontend_port` blocks as defined below.
* @property gatewayIpConfigurations One or more `gateway_ip_configuration` blocks as defined below.
* @property globals A `global` block as defined below.
* @property http2Enabled Is HTTP2 enabled on the application gateway resource?
* @property httpListeners One or more `http_listener` blocks as defined below.
* @property id The provider-assigned unique ID for this managed resource.
* @property identities An `identity` block as defined below.
* @property location The Azure region where the Application Gateway exists.
* @property name Unique name of the Rewrite Rule
* @property privateEndpointConnections
* @property privateLinkConfigurations One or more `private_link_configuration` blocks as defined below.
* @property probes One or more `probe` blocks as defined below.
* @property redirectConfigurations One or more `redirect_configuration` blocks as defined below.
* @property requestRoutingRules One or more `request_routing_rule` blocks as defined below.
* @property resourceGroupName
* @property rewriteRuleSets One or more `rewrite_rule_set` blocks as defined below.
* @property skus A `sku` block as defined below.
* @property sslCertificates One or more `ssl_certificate` blocks as defined below.
* @property sslPolicies a `ssl_policy` block as defined below.
* @property sslProfiles One or more `ssl_profile` blocks as defined below.
* @property tags A mapping of tags to assign to the resource.
* @property trustedClientCertificates One or more `trusted_client_certificate` blocks as defined below.
* @property trustedRootCertificates One or more `trusted_root_certificate` blocks as defined below.
* @property urlPathMaps One or more `url_path_map` blocks as defined below.
* @property wafConfigurations A `waf_configuration` block as defined below.
* @property zones The list of Availability Zones in which this Application Gateway can use.
*/
public data class GetApplicationGatewayResult(
public val authenticationCertificates: List,
public val autoscaleConfigurations: List,
public val backendAddressPools: List,
public val backendHttpSettings: List,
public val customErrorConfigurations: List,
public val fipsEnabled: Boolean,
public val firewallPolicyId: String,
public val forceFirewallPolicyAssociation: Boolean,
public val frontendIpConfigurations: List,
public val frontendPorts: List,
public val gatewayIpConfigurations: List,
public val globals: List,
public val http2Enabled: Boolean,
public val httpListeners: List,
public val id: String,
public val identities: List,
public val location: String,
public val name: String,
public val privateEndpointConnections: List,
public val privateLinkConfigurations: List,
public val probes: List,
public val redirectConfigurations: List,
public val requestRoutingRules: List,
public val resourceGroupName: String,
public val rewriteRuleSets: List,
public val skus: List,
public val sslCertificates: List,
public val sslPolicies: List,
public val sslProfiles: List,
public val tags: Map,
public val trustedClientCertificates: List,
public val trustedRootCertificates: List,
public val urlPathMaps: List,
public val wafConfigurations: List,
public val zones: List,
) {
public companion object {
public fun toKotlin(javaType: com.pulumi.azure.network.outputs.GetApplicationGatewayResult):
GetApplicationGatewayResult = GetApplicationGatewayResult(
authenticationCertificates = javaType.authenticationCertificates().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azure.network.kotlin.outputs.GetApplicationGatewayAuthenticationCertificate.Companion.toKotlin(args0)
})
}),
autoscaleConfigurations = javaType.autoscaleConfigurations().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azure.network.kotlin.outputs.GetApplicationGatewayAutoscaleConfiguration.Companion.toKotlin(args0)
})
}),
backendAddressPools = javaType.backendAddressPools().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azure.network.kotlin.outputs.GetApplicationGatewayBackendAddressPool.Companion.toKotlin(args0)
})
}),
backendHttpSettings = javaType.backendHttpSettings().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azure.network.kotlin.outputs.GetApplicationGatewayBackendHttpSetting.Companion.toKotlin(args0)
})
}),
customErrorConfigurations = javaType.customErrorConfigurations().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azure.network.kotlin.outputs.GetApplicationGatewayCustomErrorConfiguration.Companion.toKotlin(args0)
})
}),
fipsEnabled = javaType.fipsEnabled(),
firewallPolicyId = javaType.firewallPolicyId(),
forceFirewallPolicyAssociation = javaType.forceFirewallPolicyAssociation(),
frontendIpConfigurations = javaType.frontendIpConfigurations().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azure.network.kotlin.outputs.GetApplicationGatewayFrontendIpConfiguration.Companion.toKotlin(args0)
})
}),
frontendPorts = javaType.frontendPorts().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azure.network.kotlin.outputs.GetApplicationGatewayFrontendPort.Companion.toKotlin(args0)
})
}),
gatewayIpConfigurations = javaType.gatewayIpConfigurations().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azure.network.kotlin.outputs.GetApplicationGatewayGatewayIpConfiguration.Companion.toKotlin(args0)
})
}),
globals = javaType.globals().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azure.network.kotlin.outputs.GetApplicationGatewayGlobal.Companion.toKotlin(args0)
})
}),
http2Enabled = javaType.http2Enabled(),
httpListeners = javaType.httpListeners().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azure.network.kotlin.outputs.GetApplicationGatewayHttpListener.Companion.toKotlin(args0)
})
}),
id = javaType.id(),
identities = javaType.identities().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azure.network.kotlin.outputs.GetApplicationGatewayIdentity.Companion.toKotlin(args0)
})
}),
location = javaType.location(),
name = javaType.name(),
privateEndpointConnections = javaType.privateEndpointConnections().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azure.network.kotlin.outputs.GetApplicationGatewayPrivateEndpointConnection.Companion.toKotlin(args0)
})
}),
privateLinkConfigurations = javaType.privateLinkConfigurations().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azure.network.kotlin.outputs.GetApplicationGatewayPrivateLinkConfiguration.Companion.toKotlin(args0)
})
}),
probes = javaType.probes().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azure.network.kotlin.outputs.GetApplicationGatewayProbe.Companion.toKotlin(args0)
})
}),
redirectConfigurations = javaType.redirectConfigurations().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azure.network.kotlin.outputs.GetApplicationGatewayRedirectConfiguration.Companion.toKotlin(args0)
})
}),
requestRoutingRules = javaType.requestRoutingRules().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azure.network.kotlin.outputs.GetApplicationGatewayRequestRoutingRule.Companion.toKotlin(args0)
})
}),
resourceGroupName = javaType.resourceGroupName(),
rewriteRuleSets = javaType.rewriteRuleSets().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azure.network.kotlin.outputs.GetApplicationGatewayRewriteRuleSet.Companion.toKotlin(args0)
})
}),
skus = javaType.skus().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azure.network.kotlin.outputs.GetApplicationGatewaySkus.Companion.toKotlin(args0)
})
}),
sslCertificates = javaType.sslCertificates().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azure.network.kotlin.outputs.GetApplicationGatewaySslCertificate.Companion.toKotlin(args0)
})
}),
sslPolicies = javaType.sslPolicies().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azure.network.kotlin.outputs.GetApplicationGatewaySslPolicy.Companion.toKotlin(args0)
})
}),
sslProfiles = javaType.sslProfiles().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azure.network.kotlin.outputs.GetApplicationGatewaySslProfile.Companion.toKotlin(args0)
})
}),
tags = javaType.tags().map({ args0 -> args0.key.to(args0.value) }).toMap(),
trustedClientCertificates = javaType.trustedClientCertificates().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azure.network.kotlin.outputs.GetApplicationGatewayTrustedClientCertificate.Companion.toKotlin(args0)
})
}),
trustedRootCertificates = javaType.trustedRootCertificates().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azure.network.kotlin.outputs.GetApplicationGatewayTrustedRootCertificate.Companion.toKotlin(args0)
})
}),
urlPathMaps = javaType.urlPathMaps().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azure.network.kotlin.outputs.GetApplicationGatewayUrlPathMap.Companion.toKotlin(args0)
})
}),
wafConfigurations = javaType.wafConfigurations().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azure.network.kotlin.outputs.GetApplicationGatewayWafConfiguration.Companion.toKotlin(args0)
})
}),
zones = javaType.zones().map({ args0 -> args0 }),
)
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy