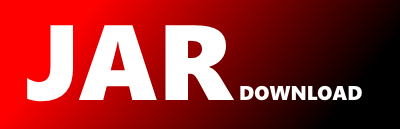
com.pulumi.azure.network.kotlin.outputs.GetGatewayConnectionResult.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-kotlin Show documentation
Show all versions of pulumi-azure-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azure.network.kotlin.outputs
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
import kotlin.collections.Map
/**
* A collection of values returned by getGatewayConnection.
* @property authorizationKey The authorization key associated with the
* Express Route Circuit. This field is present only if the type is an
* ExpressRoute connection.
* @property connectionProtocol
* @property dpdTimeoutSeconds The dead peer detection timeout of this connection in seconds.
* @property egressBytesTransferred
* @property enableBgp If `true`, BGP (Border Gateway Protocol) is enabled
* for this connection.
* @property expressRouteCircuitId The ID of the Express Route Circuit
* (i.e. when `type` is `ExpressRoute`).
* @property expressRouteGatewayBypass If `true`, data packets will bypass ExpressRoute Gateway for data forwarding. This is only valid for ExpressRoute connections.
* @property id The provider-assigned unique ID for this managed resource.
* @property ingressBytesTransferred
* @property ipsecPolicies (Optional) A `ipsec_policy` block which is documented below.
* Only a single policy can be defined for a connection. For details on
* custom policies refer to [the relevant section in the Azure documentation](https://docs.microsoft.com/azure/vpn-gateway/vpn-gateway-ipsecikepolicy-rm-powershell).
* @property localAzureIpAddressEnabled Use private local Azure IP for the connection.
* @property localNetworkGatewayId The ID of the local network gateway
* when a Site-to-Site connection (i.e. when `type` is `IPsec`).
* @property location The location/region where the connection is
* located.
* @property name
* @property peerVirtualNetworkGatewayId The ID of the peer virtual
* network gateway when a VNet-to-VNet connection (i.e. when `type`
* is `Vnet2Vnet`).
* @property privateLinkFastPathEnabled If `true`, data packets will bypass the Express Route gateway when accessing private-links.
* This is only valid for ExpressRoute connections, on the conditions described in [the relevant section in the Azure documentation](https://learn.microsoft.com/en-us/azure/expressroute/expressroute-howto-linkvnet-arm#fastpath-virtual-network-peering-user-defined-routes-udrs-and-private-link-support-for-expressroute-direct-connections)
* @property resourceGroupName
* @property resourceGuid
* @property routingWeight The routing weight.
* @property sharedKey The shared IPSec key.
* @property tags A mapping of tags to assign to the resource.
* @property trafficSelectorPolicies One or more `traffic_selector_policy` blocks which are documented below.
* A `traffic_selector_policy` allows to specify a traffic selector policy proposal to be used in a virtual network gateway connection.
* For details about traffic selectors refer to [the relevant section in the Azure documentation](https://docs.microsoft.com/azure/vpn-gateway/vpn-gateway-connect-multiple-policybased-rm-ps).
* @property type The type of connection. Valid options are `IPsec`
* (Site-to-Site), `ExpressRoute` (ExpressRoute), and `Vnet2Vnet` (VNet-to-VNet).
* @property usePolicyBasedTrafficSelectors If `true`, policy-based traffic
* selectors are enabled for this connection. Enabling policy-based traffic
* selectors requires an `ipsec_policy` block.
* @property virtualNetworkGatewayId The ID of the Virtual Network Gateway
* in which the connection is created.
*/
public data class GetGatewayConnectionResult(
public val authorizationKey: String,
public val connectionProtocol: String,
public val dpdTimeoutSeconds: Int,
public val egressBytesTransferred: Int,
public val enableBgp: Boolean,
public val expressRouteCircuitId: String,
public val expressRouteGatewayBypass: Boolean,
public val id: String,
public val ingressBytesTransferred: Int,
public val ipsecPolicies: List,
public val localAzureIpAddressEnabled: Boolean,
public val localNetworkGatewayId: String,
public val location: String,
public val name: String,
public val peerVirtualNetworkGatewayId: String,
public val privateLinkFastPathEnabled: Boolean,
public val resourceGroupName: String,
public val resourceGuid: String,
public val routingWeight: Int,
public val sharedKey: String,
public val tags: Map,
public val trafficSelectorPolicies: List,
public val type: String,
public val usePolicyBasedTrafficSelectors: Boolean,
public val virtualNetworkGatewayId: String,
) {
public companion object {
public fun toKotlin(javaType: com.pulumi.azure.network.outputs.GetGatewayConnectionResult):
GetGatewayConnectionResult = GetGatewayConnectionResult(
authorizationKey = javaType.authorizationKey(),
connectionProtocol = javaType.connectionProtocol(),
dpdTimeoutSeconds = javaType.dpdTimeoutSeconds(),
egressBytesTransferred = javaType.egressBytesTransferred(),
enableBgp = javaType.enableBgp(),
expressRouteCircuitId = javaType.expressRouteCircuitId(),
expressRouteGatewayBypass = javaType.expressRouteGatewayBypass(),
id = javaType.id(),
ingressBytesTransferred = javaType.ingressBytesTransferred(),
ipsecPolicies = javaType.ipsecPolicies().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azure.network.kotlin.outputs.GetGatewayConnectionIpsecPolicy.Companion.toKotlin(args0)
})
}),
localAzureIpAddressEnabled = javaType.localAzureIpAddressEnabled(),
localNetworkGatewayId = javaType.localNetworkGatewayId(),
location = javaType.location(),
name = javaType.name(),
peerVirtualNetworkGatewayId = javaType.peerVirtualNetworkGatewayId(),
privateLinkFastPathEnabled = javaType.privateLinkFastPathEnabled(),
resourceGroupName = javaType.resourceGroupName(),
resourceGuid = javaType.resourceGuid(),
routingWeight = javaType.routingWeight(),
sharedKey = javaType.sharedKey(),
tags = javaType.tags().map({ args0 -> args0.key.to(args0.value) }).toMap(),
trafficSelectorPolicies = javaType.trafficSelectorPolicies().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azure.network.kotlin.outputs.GetGatewayConnectionTrafficSelectorPolicy.Companion.toKotlin(args0)
})
}),
type = javaType.type(),
usePolicyBasedTrafficSelectors = javaType.usePolicyBasedTrafficSelectors(),
virtualNetworkGatewayId = javaType.virtualNetworkGatewayId(),
)
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy