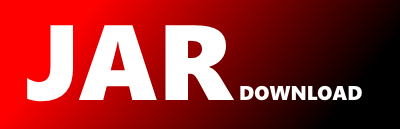
com.pulumi.azure.operationalinsights.kotlin.AnalyticsSolutionArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-kotlin Show documentation
Show all versions of pulumi-azure-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azure.operationalinsights.kotlin
import com.pulumi.azure.operationalinsights.AnalyticsSolutionArgs.builder
import com.pulumi.azure.operationalinsights.kotlin.inputs.AnalyticsSolutionPlanArgs
import com.pulumi.azure.operationalinsights.kotlin.inputs.AnalyticsSolutionPlanArgsBuilder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Pair
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.Map
import kotlin.jvm.JvmName
/**
* Manages a Log Analytics (formally Operational Insights) Solution.
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as azure from "@pulumi/azure";
* import * as random from "@pulumi/random";
* const example = new azure.core.ResourceGroup("example", {
* name: "k8s-log-analytics-test",
* location: "West Europe",
* });
* const workspace = new random.RandomId("workspace", {
* keepers: {
* group_name: example.name,
* },
* byteLength: 8,
* });
* const exampleAnalyticsWorkspace = new azure.operationalinsights.AnalyticsWorkspace("example", {
* name: pulumi.interpolate`k8s-workspace-${workspace.hex}`,
* location: example.location,
* resourceGroupName: example.name,
* sku: "PerGB2018",
* });
* const exampleAnalyticsSolution = new azure.operationalinsights.AnalyticsSolution("example", {
* solutionName: "ContainerInsights",
* location: example.location,
* resourceGroupName: example.name,
* workspaceResourceId: exampleAnalyticsWorkspace.id,
* workspaceName: exampleAnalyticsWorkspace.name,
* plan: {
* publisher: "Microsoft",
* product: "OMSGallery/ContainerInsights",
* },
* });
* ```
* ```python
* import pulumi
* import pulumi_azure as azure
* import pulumi_random as random
* example = azure.core.ResourceGroup("example",
* name="k8s-log-analytics-test",
* location="West Europe")
* workspace = random.RandomId("workspace",
* keepers={
* "group_name": example.name,
* },
* byte_length=8)
* example_analytics_workspace = azure.operationalinsights.AnalyticsWorkspace("example",
* name=workspace.hex.apply(lambda hex: f"k8s-workspace-{hex}"),
* location=example.location,
* resource_group_name=example.name,
* sku="PerGB2018")
* example_analytics_solution = azure.operationalinsights.AnalyticsSolution("example",
* solution_name="ContainerInsights",
* location=example.location,
* resource_group_name=example.name,
* workspace_resource_id=example_analytics_workspace.id,
* workspace_name=example_analytics_workspace.name,
* plan=azure.operationalinsights.AnalyticsSolutionPlanArgs(
* publisher="Microsoft",
* product="OMSGallery/ContainerInsights",
* ))
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Azure = Pulumi.Azure;
* using Random = Pulumi.Random;
* return await Deployment.RunAsync(() =>
* {
* var example = new Azure.Core.ResourceGroup("example", new()
* {
* Name = "k8s-log-analytics-test",
* Location = "West Europe",
* });
* var workspace = new Random.RandomId("workspace", new()
* {
* Keepers =
* {
* { "group_name", example.Name },
* },
* ByteLength = 8,
* });
* var exampleAnalyticsWorkspace = new Azure.OperationalInsights.AnalyticsWorkspace("example", new()
* {
* Name = workspace.Hex.Apply(hex => $"k8s-workspace-{hex}"),
* Location = example.Location,
* ResourceGroupName = example.Name,
* Sku = "PerGB2018",
* });
* var exampleAnalyticsSolution = new Azure.OperationalInsights.AnalyticsSolution("example", new()
* {
* SolutionName = "ContainerInsights",
* Location = example.Location,
* ResourceGroupName = example.Name,
* WorkspaceResourceId = exampleAnalyticsWorkspace.Id,
* WorkspaceName = exampleAnalyticsWorkspace.Name,
* Plan = new Azure.OperationalInsights.Inputs.AnalyticsSolutionPlanArgs
* {
* Publisher = "Microsoft",
* Product = "OMSGallery/ContainerInsights",
* },
* });
* });
* ```
* ```go
* package main
* import (
* "fmt"
* "github.com/pulumi/pulumi-azure/sdk/v5/go/azure/core"
* "github.com/pulumi/pulumi-azure/sdk/v5/go/azure/operationalinsights"
* "github.com/pulumi/pulumi-random/sdk/v4/go/random"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* example, err := core.NewResourceGroup(ctx, "example", &core.ResourceGroupArgs{
* Name: pulumi.String("k8s-log-analytics-test"),
* Location: pulumi.String("West Europe"),
* })
* if err != nil {
* return err
* }
* workspace, err := random.NewRandomId(ctx, "workspace", &random.RandomIdArgs{
* Keepers: pulumi.StringMap{
* "group_name": example.Name,
* },
* ByteLength: pulumi.Int(8),
* })
* if err != nil {
* return err
* }
* exampleAnalyticsWorkspace, err := operationalinsights.NewAnalyticsWorkspace(ctx, "example", &operationalinsights.AnalyticsWorkspaceArgs{
* Name: workspace.Hex.ApplyT(func(hex string) (string, error) {
* return fmt.Sprintf("k8s-workspace-%v", hex), nil
* }).(pulumi.StringOutput),
* Location: example.Location,
* ResourceGroupName: example.Name,
* Sku: pulumi.String("PerGB2018"),
* })
* if err != nil {
* return err
* }
* _, err = operationalinsights.NewAnalyticsSolution(ctx, "example", &operationalinsights.AnalyticsSolutionArgs{
* SolutionName: pulumi.String("ContainerInsights"),
* Location: example.Location,
* ResourceGroupName: example.Name,
* WorkspaceResourceId: exampleAnalyticsWorkspace.ID(),
* WorkspaceName: exampleAnalyticsWorkspace.Name,
* Plan: &operationalinsights.AnalyticsSolutionPlanArgs{
* Publisher: pulumi.String("Microsoft"),
* Product: pulumi.String("OMSGallery/ContainerInsights"),
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azure.core.ResourceGroup;
* import com.pulumi.azure.core.ResourceGroupArgs;
* import com.pulumi.random.RandomId;
* import com.pulumi.random.RandomIdArgs;
* import com.pulumi.azure.operationalinsights.AnalyticsWorkspace;
* import com.pulumi.azure.operationalinsights.AnalyticsWorkspaceArgs;
* import com.pulumi.azure.operationalinsights.AnalyticsSolution;
* import com.pulumi.azure.operationalinsights.AnalyticsSolutionArgs;
* import com.pulumi.azure.operationalinsights.inputs.AnalyticsSolutionPlanArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var example = new ResourceGroup("example", ResourceGroupArgs.builder()
* .name("k8s-log-analytics-test")
* .location("West Europe")
* .build());
* var workspace = new RandomId("workspace", RandomIdArgs.builder()
* .keepers(Map.of("group_name", example.name()))
* .byteLength(8)
* .build());
* var exampleAnalyticsWorkspace = new AnalyticsWorkspace("exampleAnalyticsWorkspace", AnalyticsWorkspaceArgs.builder()
* .name(workspace.hex().applyValue(hex -> String.format("k8s-workspace-%s", hex)))
* .location(example.location())
* .resourceGroupName(example.name())
* .sku("PerGB2018")
* .build());
* var exampleAnalyticsSolution = new AnalyticsSolution("exampleAnalyticsSolution", AnalyticsSolutionArgs.builder()
* .solutionName("ContainerInsights")
* .location(example.location())
* .resourceGroupName(example.name())
* .workspaceResourceId(exampleAnalyticsWorkspace.id())
* .workspaceName(exampleAnalyticsWorkspace.name())
* .plan(AnalyticsSolutionPlanArgs.builder()
* .publisher("Microsoft")
* .product("OMSGallery/ContainerInsights")
* .build())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* example:
* type: azure:core:ResourceGroup
* properties:
* name: k8s-log-analytics-test
* location: West Europe
* workspace:
* type: random:RandomId
* properties:
* keepers:
* group_name: ${example.name}
* byteLength: 8
* exampleAnalyticsWorkspace:
* type: azure:operationalinsights:AnalyticsWorkspace
* name: example
* properties:
* name: k8s-workspace-${workspace.hex}
* location: ${example.location}
* resourceGroupName: ${example.name}
* sku: PerGB2018
* exampleAnalyticsSolution:
* type: azure:operationalinsights:AnalyticsSolution
* name: example
* properties:
* solutionName: ContainerInsights
* location: ${example.location}
* resourceGroupName: ${example.name}
* workspaceResourceId: ${exampleAnalyticsWorkspace.id}
* workspaceName: ${exampleAnalyticsWorkspace.name}
* plan:
* publisher: Microsoft
* product: OMSGallery/ContainerInsights
* ```
*
* ## Import
* Log Analytics Solutions can be imported using the `resource id`, e.g.
* ```sh
* $ pulumi import azure:operationalinsights/analyticsSolution:AnalyticsSolution solution1 /subscriptions/00000000-0000-0000-0000-000000000000/resourceGroups/mygroup1/providers/Microsoft.OperationsManagement/solutions/solution1
* ```
* @property location Specifies the supported Azure location where the resource exists. Changing this forces a new resource to be created.
* @property plan A `plan` block as documented below.
* @property resourceGroupName The name of the resource group in which the Log Analytics solution is created. Changing this forces a new resource to be created. Note: The solution and its related workspace can only exist in the same resource group.
* @property solutionName Specifies the name of the solution to be deployed. See [here for options](https://docs.microsoft.com/azure/log-analytics/log-analytics-add-solutions).Changing this forces a new resource to be created.
* @property tags A mapping of tags to assign to the resource.
* @property workspaceName The full name of the Log Analytics workspace with which the solution will be linked. Changing this forces a new resource to be created.
* @property workspaceResourceId The full resource ID of the Log Analytics workspace with which the solution will be linked. Changing this forces a new resource to be created.
*/
public data class AnalyticsSolutionArgs(
public val location: Output? = null,
public val plan: Output? = null,
public val resourceGroupName: Output? = null,
public val solutionName: Output? = null,
public val tags: Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy