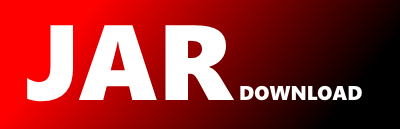
com.pulumi.azure.privatedns.kotlin.inputs.LinkServiceNatIpConfigurationArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-kotlin Show documentation
Show all versions of pulumi-azure-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azure.privatedns.kotlin.inputs
import com.pulumi.azure.privatedns.inputs.LinkServiceNatIpConfigurationArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
*
* @property name Specifies the name which should be used for the NAT IP Configuration. Changing this forces a new resource to be created.
* @property primary Is this is the Primary IP Configuration? Changing this forces a new resource to be created.
* @property privateIpAddress Specifies a Private Static IP Address for this IP Configuration.
* @property privateIpAddressVersion The version of the IP Protocol which should be used. At this time the only supported value is `IPv4`. Defaults to `IPv4`.
* @property subnetId Specifies the ID of the Subnet which should be used for the Private Link Service.
* > **NOTE:** Verify that the Subnet's `enforce_private_link_service_network_policies` attribute is set to `true`.
*/
public data class LinkServiceNatIpConfigurationArgs(
public val name: Output,
public val primary: Output,
public val privateIpAddress: Output? = null,
public val privateIpAddressVersion: Output? = null,
public val subnetId: Output,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azure.privatedns.inputs.LinkServiceNatIpConfigurationArgs =
com.pulumi.azure.privatedns.inputs.LinkServiceNatIpConfigurationArgs.builder()
.name(name.applyValue({ args0 -> args0 }))
.primary(primary.applyValue({ args0 -> args0 }))
.privateIpAddress(privateIpAddress?.applyValue({ args0 -> args0 }))
.privateIpAddressVersion(privateIpAddressVersion?.applyValue({ args0 -> args0 }))
.subnetId(subnetId.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [LinkServiceNatIpConfigurationArgs].
*/
@PulumiTagMarker
public class LinkServiceNatIpConfigurationArgsBuilder internal constructor() {
private var name: Output? = null
private var primary: Output? = null
private var privateIpAddress: Output? = null
private var privateIpAddressVersion: Output? = null
private var subnetId: Output? = null
/**
* @param value Specifies the name which should be used for the NAT IP Configuration. Changing this forces a new resource to be created.
*/
@JvmName("gygpohjmkpgaonbh")
public suspend fun name(`value`: Output) {
this.name = value
}
/**
* @param value Is this is the Primary IP Configuration? Changing this forces a new resource to be created.
*/
@JvmName("nhkbphtwckmmnkcr")
public suspend fun primary(`value`: Output) {
this.primary = value
}
/**
* @param value Specifies a Private Static IP Address for this IP Configuration.
*/
@JvmName("ksqwryiaycmwfecq")
public suspend fun privateIpAddress(`value`: Output) {
this.privateIpAddress = value
}
/**
* @param value The version of the IP Protocol which should be used. At this time the only supported value is `IPv4`. Defaults to `IPv4`.
*/
@JvmName("bpipadpmcshuhrpi")
public suspend fun privateIpAddressVersion(`value`: Output) {
this.privateIpAddressVersion = value
}
/**
* @param value Specifies the ID of the Subnet which should be used for the Private Link Service.
* > **NOTE:** Verify that the Subnet's `enforce_private_link_service_network_policies` attribute is set to `true`.
*/
@JvmName("eexskissplbaylxt")
public suspend fun subnetId(`value`: Output) {
this.subnetId = value
}
/**
* @param value Specifies the name which should be used for the NAT IP Configuration. Changing this forces a new resource to be created.
*/
@JvmName("cdymqmmkhutjaxjn")
public suspend fun name(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.name = mapped
}
/**
* @param value Is this is the Primary IP Configuration? Changing this forces a new resource to be created.
*/
@JvmName("iixvciylqtxjbngc")
public suspend fun primary(`value`: Boolean) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.primary = mapped
}
/**
* @param value Specifies a Private Static IP Address for this IP Configuration.
*/
@JvmName("exctxkipfwjckkhc")
public suspend fun privateIpAddress(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.privateIpAddress = mapped
}
/**
* @param value The version of the IP Protocol which should be used. At this time the only supported value is `IPv4`. Defaults to `IPv4`.
*/
@JvmName("vyqbtenqbirksoqy")
public suspend fun privateIpAddressVersion(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.privateIpAddressVersion = mapped
}
/**
* @param value Specifies the ID of the Subnet which should be used for the Private Link Service.
* > **NOTE:** Verify that the Subnet's `enforce_private_link_service_network_policies` attribute is set to `true`.
*/
@JvmName("obbvxtpqfqkgokyc")
public suspend fun subnetId(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.subnetId = mapped
}
internal fun build(): LinkServiceNatIpConfigurationArgs = LinkServiceNatIpConfigurationArgs(
name = name ?: throw PulumiNullFieldException("name"),
primary = primary ?: throw PulumiNullFieldException("primary"),
privateIpAddress = privateIpAddress,
privateIpAddressVersion = privateIpAddressVersion,
subnetId = subnetId ?: throw PulumiNullFieldException("subnetId"),
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy